Dependency injection in C++ is a design pattern that allows a class to receive its dependencies from an external source rather than creating them internally, promoting better modularity and easier testing. Here's a simple code snippet to illustrate dependency injection using constructor injection:
#include <iostream>
// Interface
class IService {
public:
virtual void serve() = 0;
};
// Implementation of IService
class Service : public IService {
public:
void serve() override {
std::cout << "Service Called!" << std::endl;
}
};
// Client that depends on IService
class Client {
private:
IService* service;
public:
Client(IService* svc) : service(svc) {} // Dependency Injection via Constructor
void doSomething() {
service->serve();
}
};
int main() {
Service service;
Client client(&service);
client.doSomething(); // Output: Service Called!
return 0;
}
What is Dependency Injection?
Dependency injection (DI) is a software design pattern that allows a class to receive its dependencies from an external source rather than creating them internally. This decoupling provides greater flexibility, maintainability, and testability in software development. Instead of a class relying on concrete implementations of its dependencies, it declares the dependencies it requires and allows an external framework or container to provide them.
Importance in Software Design
Dependency injection plays a critical role in software design by fostering loose coupling among components, making them more interchangeable and easier to test. When classes are not tightly bound to their dependencies, developers can swap implementations, facilitating changes and improvements without impacting the entire system. This architectural choice aligns well with SOLID principles, particularly the Dependency Inversion Principle, promoting a cleaner and more modular codebase.
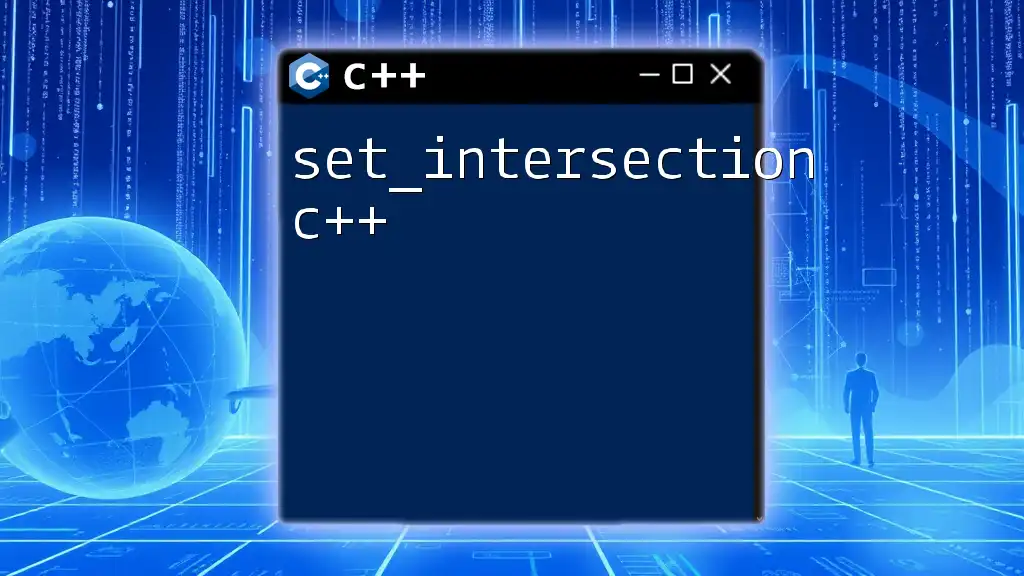
Benefits of Dependency Injection in C++
Promotes Code Reusability
One of the primary benefits of using dependency injection in C++ is that it enhances code reusability. By decoupling components, developers can reuse various parts of the application in different contexts, and this modularity decreases the chances of code duplication. For example, if a service class depends on multiple data repository implementations, changing the repository used does not require a significant rework of the service class itself.
Enhances Testability
Unit testing becomes simpler and more effective with dependency injection. By allowing dependencies to be injected, you can easily substitute concrete implementations with mocks or stubs during tests, leading to faster and more reliable test runs. This capability becomes even more critical in C++ due to its compiled nature, where changing code for tests may result in extensive refactoring.
Encourages Loose Coupling
Loose coupling is a design principle that ensures that changes to one component minimally impact other components. Dependency injection promotes this by ensuring that classes do not need to be aware of the construction details of their dependencies. This brings about improved maintainability and flexibility, as changing one class or component does not necessitate changes to others that depend on it.
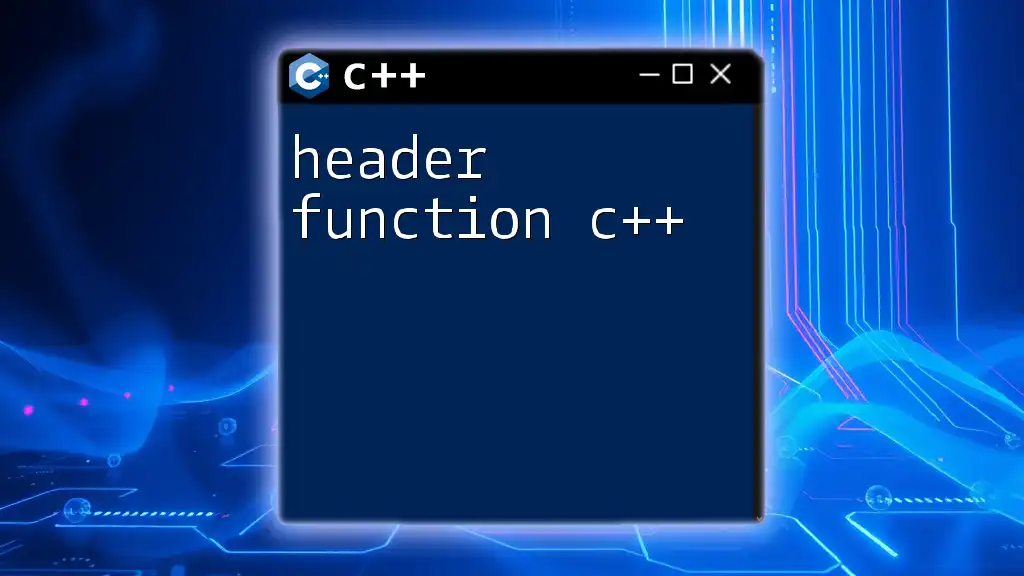
Understanding the Basics
Key Concepts of Dependency Injection
-
Dependencies: These are the objects that a class requires to function correctly. For example, if a class `Car` requires an instance of `Engine`, then `Engine` is a dependency for `Car`.
-
Containers: A DI container is a framework that manages the creation and lifetime of dependencies. It assembles these dependencies before they are needed, essentially providing a central point of control.
-
Injection: This refers to how the dependencies are provided to the class. There are three primary methods of dependency injection: Constructor Injection, Setter Injection, and Interface Injection.
Constructor Injection
Constructor injection is a method in which dependencies are provided through a class constructor. This approach is often favored because it makes dependencies explicit and immutable once constructed.
Code Example:
class Engine {
public:
void start() {}
};
class Car {
private:
Engine& engine; // Engine dependency
public:
Car(Engine& eng) : engine(eng) {}
void drive() {
engine.start();
// Logic to drive the car
}
};
In this example, `Car` receives its `Engine` dependency via the constructor, allowing for flexibility and clear separation of concerns.
Setter Injection
Setter injection involves passing dependencies via setter methods after the object is constructed. While this method provides greater flexibility for changes, it may lead to a situation where the object can be in an incomplete state.
Code Example:
class Car {
private:
Engine* engine; // Pointer to Engine dependency
public:
void setEngine(Engine* eng) {
engine = eng;
}
void drive() {
if (engine) {
engine->start();
// Logic to drive the car
}
}
};
In this case, the `Car` class can accept an `Engine` instance after construction, but it is important to ensure that `setEngine` is called before invoking `drive`.
Interface Injection
Interface injection involves defining an interface that must be implemented to receive dependencies. This method is less common in C++, as it often requires additional boilerplate code.
Code Example:
class IEngine {
public:
virtual void start() = 0; // Pure virtual function
};
class Engine : public IEngine {
public:
void start() override {}
};
class Car {
private:
IEngine* engine; // Pointer to engine interface
public:
void setEngine(IEngine* eng) {
engine = eng;
}
void drive() {
if (engine) {
engine->start();
// Logic to drive the car
}
}
};
While this method allows for different types of engines to be injected, it comes with additional complexity that isn't always necessary.
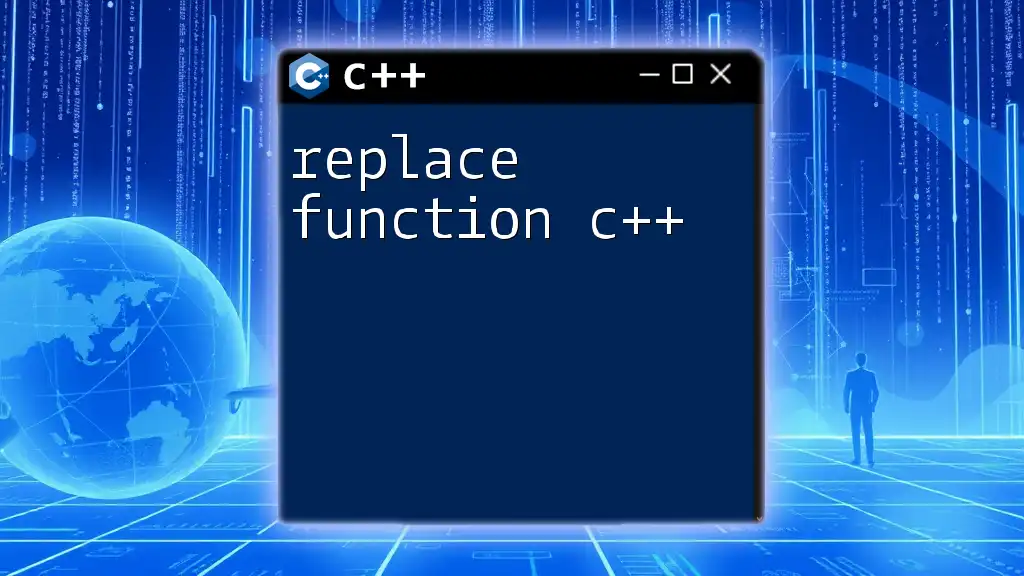
Implementing Dependency Injection in C++
Setting Up Your Project
Before implementing dependency injection, it is essential to set up your project. Choose a suitable Integrated Development Environment (IDE) or text editor and ensure that you have a C++ compiler installed. Libraries like Boost.DI can simplify the process.
Creating Dependency Injection Containers
Manual DI Container
A manual DI container involves creating a simple class to manage dependencies. It typically includes registration methods for each dependency and the ability to resolve them.
Code Example:
class DIContainer {
private:
std::map<std::string, std::function<void*()>> services;
public:
template<typename T>
void registerService(const std::string& name) {
services[name] = []() { return new T(); };
}
template<typename T>
T* resolve(const std::string& name) {
return static_cast<T*>(services[name]());
}
};
In this practical example, the `DIContainer` can register services and resolve them based on names, offering a degree of flexibility.
Using a DI Framework
Using a dependency injection framework like Boost.DI can significantly streamline the creation and management of dependencies. Boost.DI provides a more sophisticated approach to handling scopes, lifetimes, and creating more complex graphs of dependencies.
Code Example:
#include <boost/di.hpp>
namespace di = boost::di;
class Engine {
public:
void start() {}
};
class Car {
public:
Car(std::shared_ptr<Engine> engine) : engine_(engine) {}
void drive() {
engine_->start();
// Logic to drive the car
}
private:
std::shared_ptr<Engine> engine_;
};
int main() {
auto injector = di::make_injector(
di::bind<Engine>().to<Engine>(),
di::bind<Car>().to<Car>()
);
auto car = injector.create<std::shared_ptr<Car>>();
car->drive();
return 0;
}
This example demonstrates how easy it is to integrate dependencies using the Boost.DI framework, allowing for flexible and maintainable code.
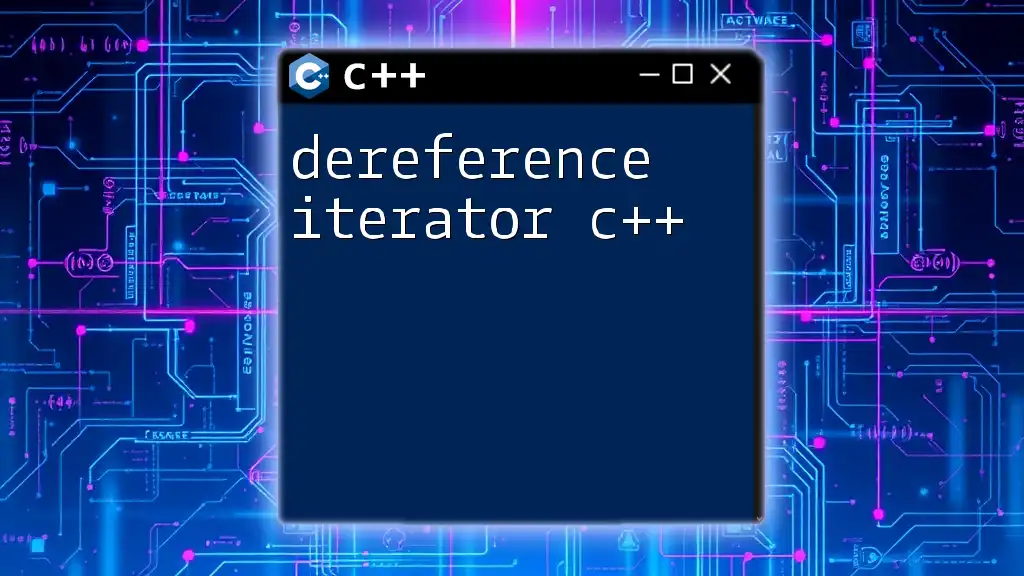
Advanced Topics in Dependency Injection
Lifecycle Management
Managing the lifecycle of dependencies is crucial in ensuring resources are effectively utilized. There are two main lifecycle patterns: Singleton and Prototype.
Singleton vs. Prototype
-
Singleton: An instance is created once and shared across the application. This is ideal when only one instance is needed, like a logging service.
-
Prototype: A fresh instance is created every time it is requested. This is useful for objects that maintain state independently, such as user sessions.
When choosing between these patterns, consider the nature of the dependencies and their usage within your application.
Scoping and Context
It’s essential to understand the importance of context within dependency injection. The context determines the lifecycle and scope of the injected dependencies. For example, if a request-based application requires dependencies tied to a session or user request, managing these scopes becomes pertinent to avoid unintended consequences.
Code Example: Managing scopes effectively
class User {
public:
User(std::string name) : name_(name) {}
private:
std::string name_;
};
class Session {
public:
void addUser(std::shared_ptr<User> user) {
users_.push_back(user);
}
private:
std::vector<std::shared_ptr<User>> users_;
};
In this code snippet, we can inject a user context and manage sessions effectively, which ensures proper lifecycle management according to the requirements of the application.
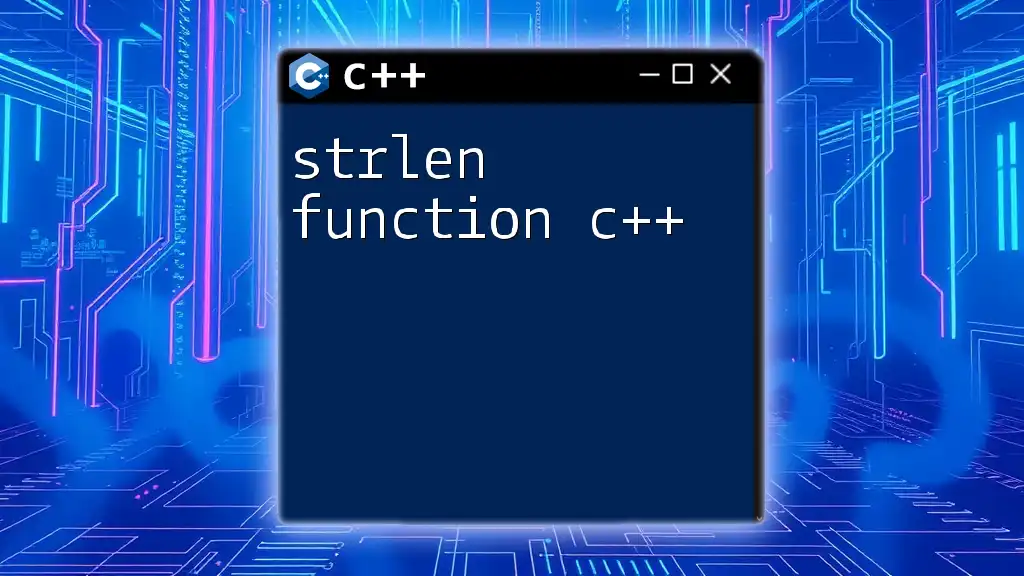
Testing with Dependency Injection
Unit Testing
Dependency injection significantly enhances unit testing capabilities. By injecting mocks and stubs, you can test classes in isolation without relying on actual implementations.
- Isolate Dependencies: Inject mock implementations in place of real ones to validate behaviors without external side effects.
- Control the Environment: Specify exactly what dependencies a class has and how they behave during tests.
Mocking Dependencies
To facilitate unit testing, mocking frameworks (like Google Mock) can conveniently create mock versions of dependencies, which can be injected where necessary.
Code Example:
class MockEngine : public IEngine {
public:
MOCK_METHOD(void, start, (), (override));
};
// Test case example
TEST(CarTest, Drive_CallsEngineStart) {
MockEngine mockEngine;
Car car;
car.setEngine(&mockEngine);
// Expect Engine::start to be called
EXPECT_CALL(mockEngine, start()).Times(1);
car.drive();
}
By utilizing mocking, you can create robust tests that validate the behavior of your code while minimizing dependencies on external systems.
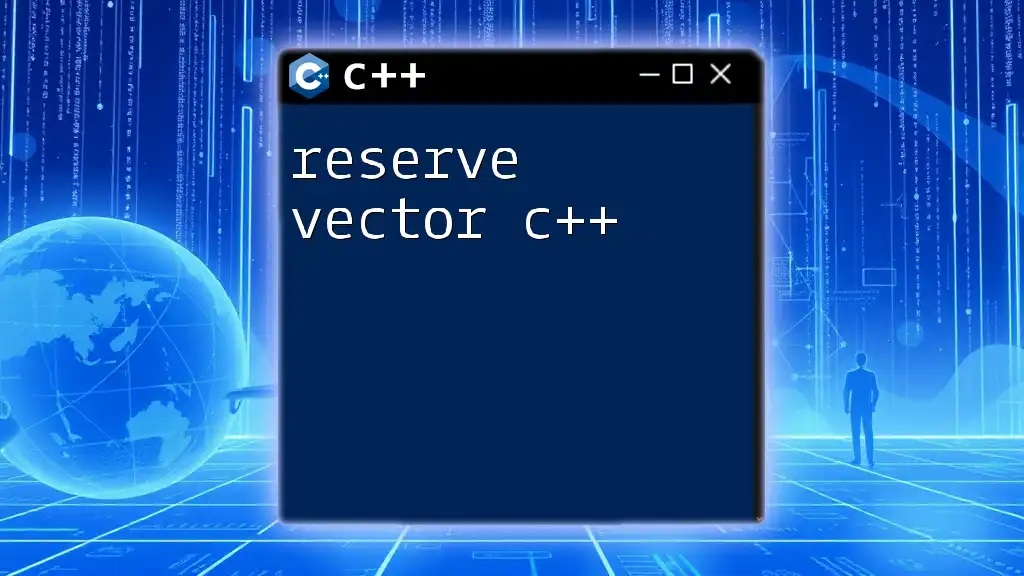
Best Practices for Dependency Injection
Keep It Simple
Avoid over-engineering your DI setup. While it may be tempting to implement sophisticated frameworks, simplicity often leads to better maintainability. Reflect on whether you genuinely need a DI container for your project or if manual injection would suffice.
Follow SOLID Principles
Implement DI practices in harmony with SOLID principles. Specifically:
- Single Responsibility Principle: Each class should have one reason to change. DI helps in ensuring classes focus on their responsibilities.
- Dependency Inversion Principle: Depend on abstractions rather than concrete implementations, a fundamental tenet in DI.
Understanding When to Use Dependency Injection
Recognize situations where dependency injection is most beneficial. If a class requires multiple dependencies that change frequently or if it needs to be extensively tested, DI is a fitting choice. Conversely, applying DI in simple classes that don’t need to be tested could lead to unnecessary complexity.
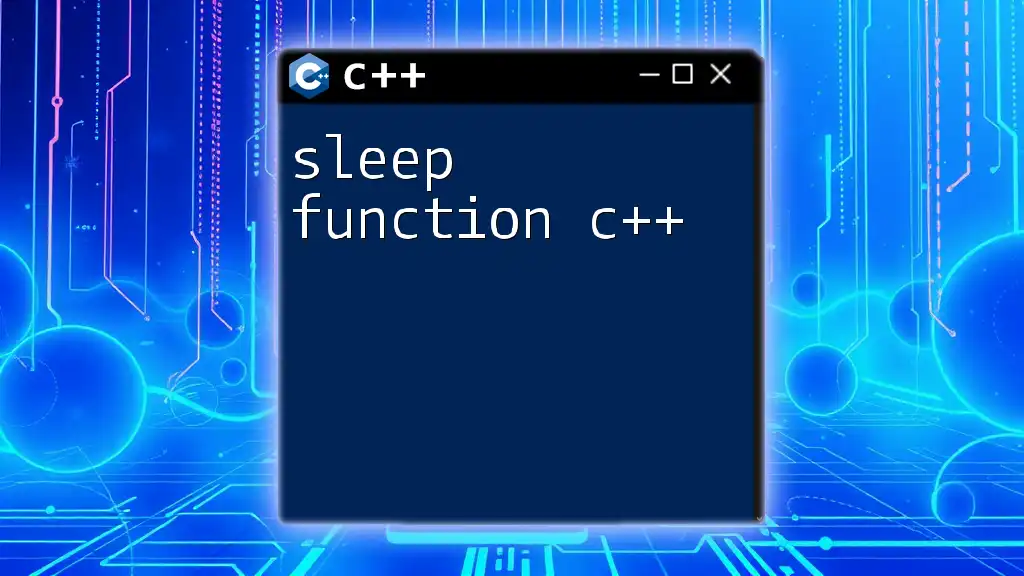
Conclusion
Dependency injection in C++ provides a robust framework to build modular, testable, and maintainable code. By embracing DI, you empower your application with flexibility, better resource management, and the ability to evolve smoothly over time.
Encouraging readers to integrate these concepts into their projects can unlock a new level of architecture sophistication, enhancing their programming skills and application performance.
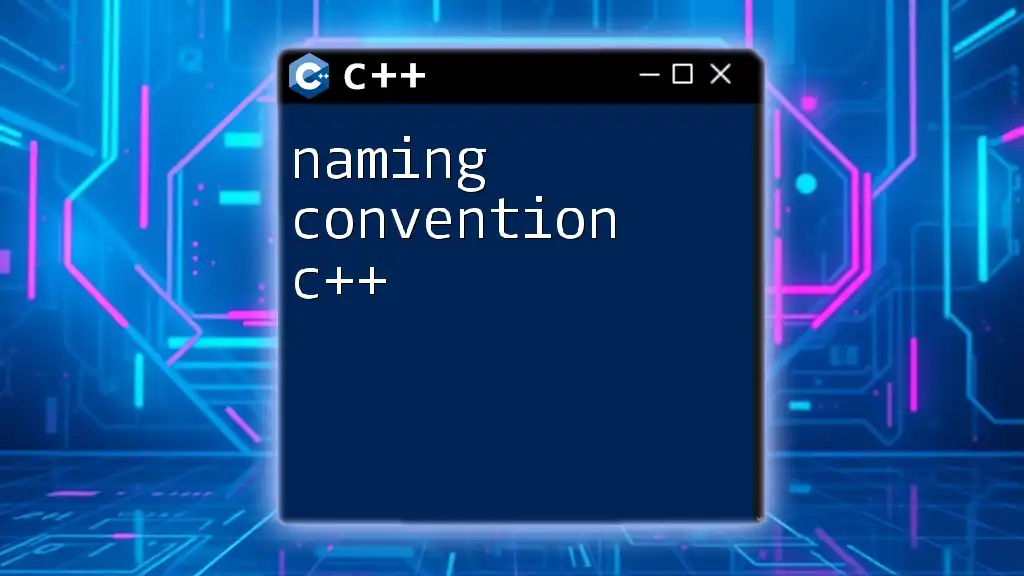
Additional Resources
Recommended Books and Articles
- "Clean Code" by Robert C. Martin: A guide on writing clean and maintainable code.
- "Dependency Injection in .NET" by Mark Seemann: Although targeted at .NET, the principles are applicable across languages.
Online Courses and Tutorials
- Coursera and Pluralsight offer C++ courses that delve deeper into design patterns including dependency injection. Check out their libraries for more detailed learning opportunities.