In C++, you can declare a 2D vector using the following syntax:
#include <vector>
std::vector<std::vector<int>> vec(3, std::vector<int>(4)); // 3 rows and 4 columns
Understanding Vectors in C++
What is a Vector?
In C++, a vector is a sequence container that encapsulates dynamic size arrays. Unlike conventional arrays, vectors can grow and shrink at runtime, which makes them highly flexible for managing collections of data.
Advantages of Using Vectors
Using vectors comes with several advantages:
- Dynamic Sizing: Vectors automatically adjust their size as elements are added or removed, providing significant ease of use.
- Automatic Memory Management: With vectors, memory allocation and deallocation are handled automatically, which reduces the risk of memory leaks.
- Performance: Vectors typically offer better performance than static arrays, especially when it comes to insertion and deletion operations.
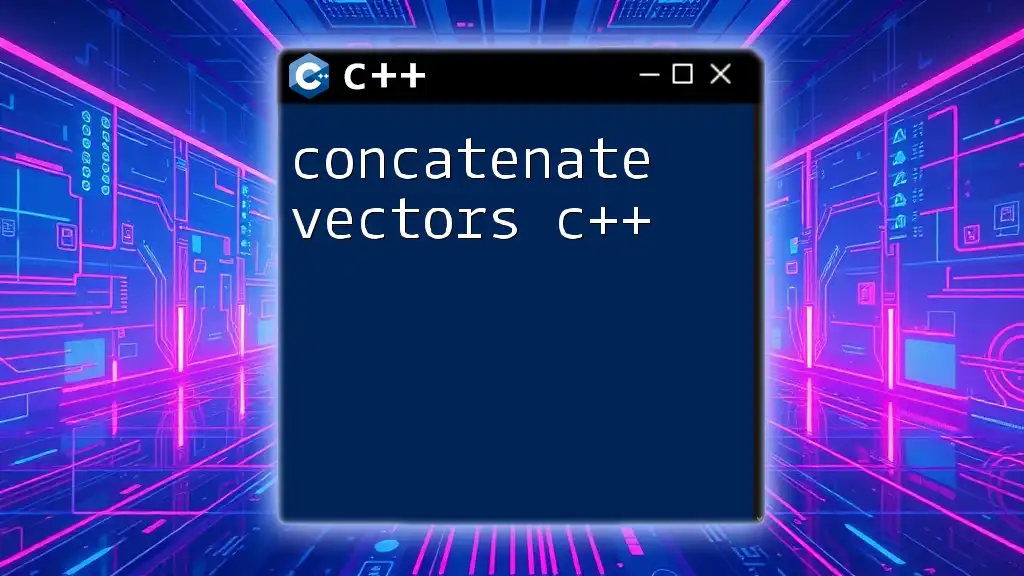
Declaring a 2D Vector
When you want to store a collection of items organized in rows and columns, you'll need to declare a 2D vector in C++. Let's dive into how you can do that effectively.
Syntax for Declaring a 2D Vector
To declare a 2D vector, you can use the following syntax:
std::vector<std::vector<int>> vec;
This statement establishes a 2D vector named `vec` that can store integers.
Size and Initial Values
It's often useful to declare a 2D vector with specific sizes. For instance, if you wanted to create a 3x4 grid (3 rows and 4 columns) initialized to zero, you can do so like this:
std::vector<std::vector<int>> vec(3, std::vector<int>(4));
This creates a 2D vector with three rows, each containing four integer elements initialized to zero.
Specifying Data Types
C++ vectors can hold various data types. You can declare a 2D vector for double data types, for example:
std::vector<std::vector<double>> vecDouble(2, std::vector<double>(3));
Here, we've created a 2D vector with 2 rows and 3 columns, where each element is initialized to zero as well.
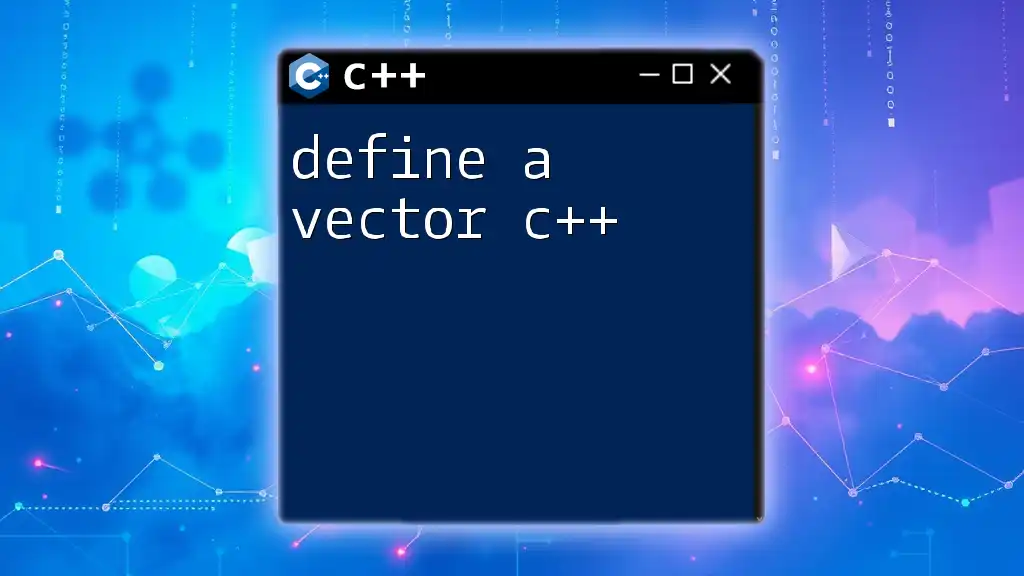
Accessing and Modifying Elements in a 2D Vector
Once you have declared a 2D vector, you'll likely want to access and modify its elements.
Accessing Elements
To access elements in a 2D vector, you can use two indices — one for the row and another for the column. For example:
int value = vec[1][2]; // Accessing the element at row 1, column 2
This retrieves the value contained in the second row and third column.
Modifying Elements
You can use nested loops to modify the elements of a 2D vector. For example, to populate the matrix with the product of its row and column indices, you could do the following:
for (size_t i = 0; i < vec.size(); ++i) {
for (size_t j = 0; j < vec[i].size(); ++j) {
vec[i][j] = i * j; // Example modification
}
}
This snippet iteratively fills each position in the 2D vector based on the product of its indices.
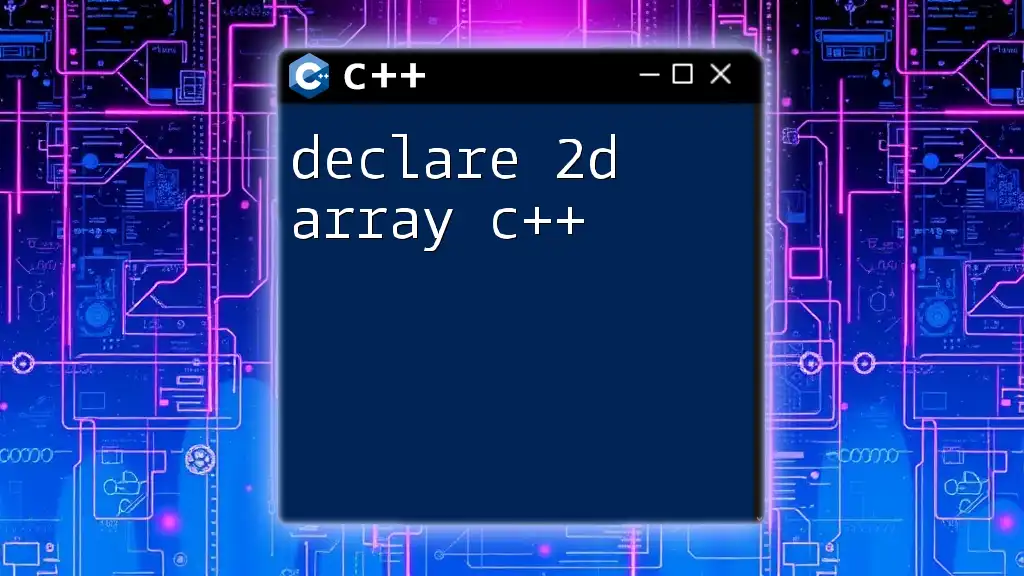
Common Operations on 2D Vectors
Understanding how to manipulate 2D vectors is vital for effective programming in C++.
Resizing a 2D Vector
If you need to change the size of your 2D vector dynamically, C++ provides the `resize` method. Here's how you can increase the size of a 2D vector to have 5 rows and 4 columns:
vec.resize(5, std::vector<int>(4));
This command modifies `vec` to add two more rows, initializing any new elements to zero.
Iterating through a 2D Vector
Iterating through a 2D vector can be seamlessly achieved using range-based for loops, making your code more readable. Here’s an example:
for (const auto& row : vec) {
for (int val : row) {
std::cout << val << " ";
}
std::cout << std::endl;
}
This code will print each row of the vector on a new line, providing a clear representation of your data.
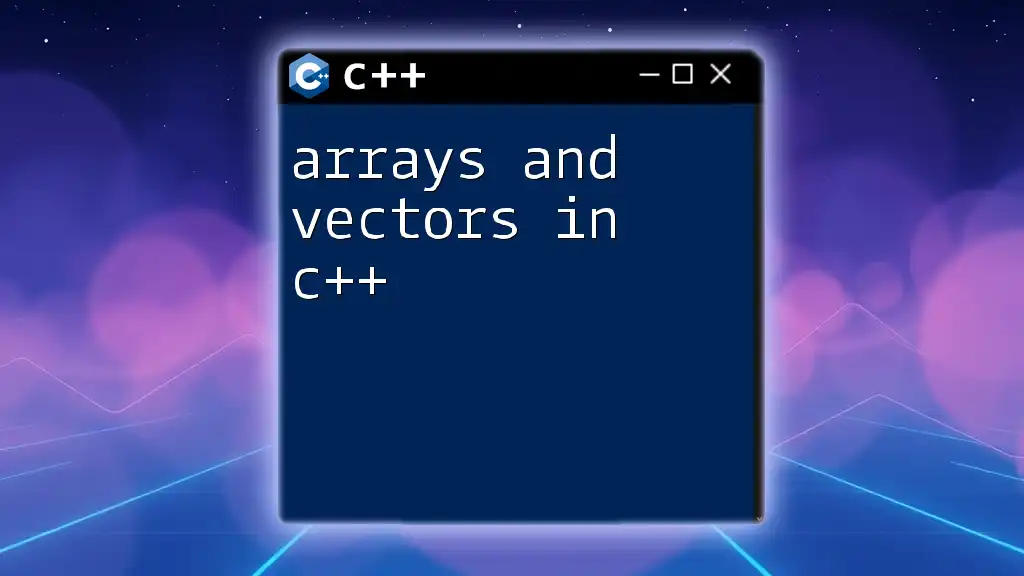
Use Cases for 2D Vectors
Representing Matrices
2D vectors are ideal for representing matrices in mathematical computations. With their dynamic size, programmers can perform operations like matrix addition, multiplication, and transposition easily.
Game Development Use Cases
In game development, 2D vectors can represent game maps, where each cell in the grid might correspond to a different terrain type or entity. This allows for flexible game designs that can adapt based on player actions or evolving game states.
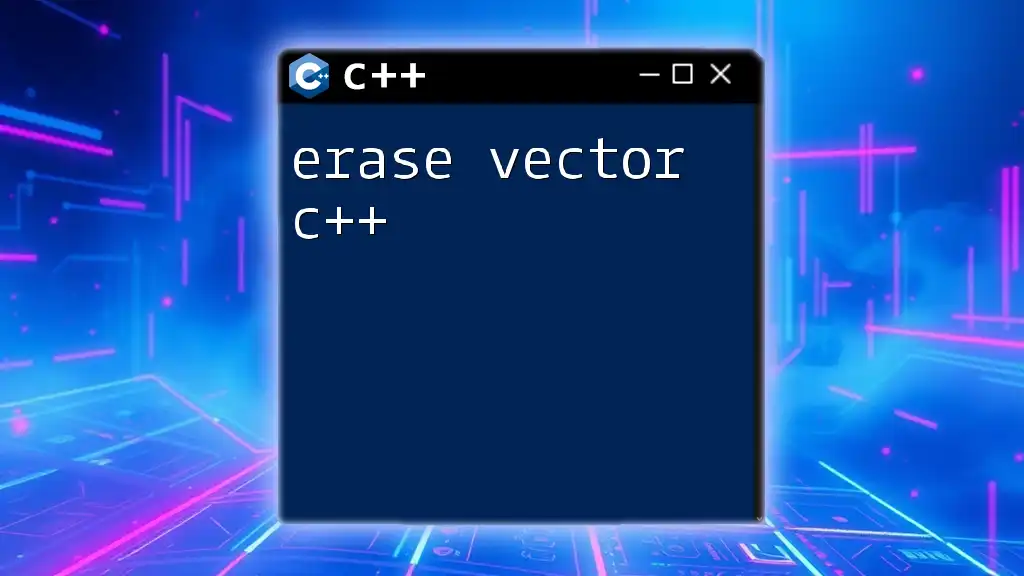
Performance Considerations
Memory Overhead
One downside of 2D vectors is that they incur some memory overhead due to dynamic allocation. Each row is a separate vector, leading to some extra space usage. Understanding your specific use case will help you decide when to use a static array instead.
Speed Implications
While vectors tend to be performant for many operations, it’s essential to recognize that they may introduce slight overhead compared to static arrays. For applications that require high performance, particularly in tight loops or with numerous elements, static arrays might sometimes be a better option.
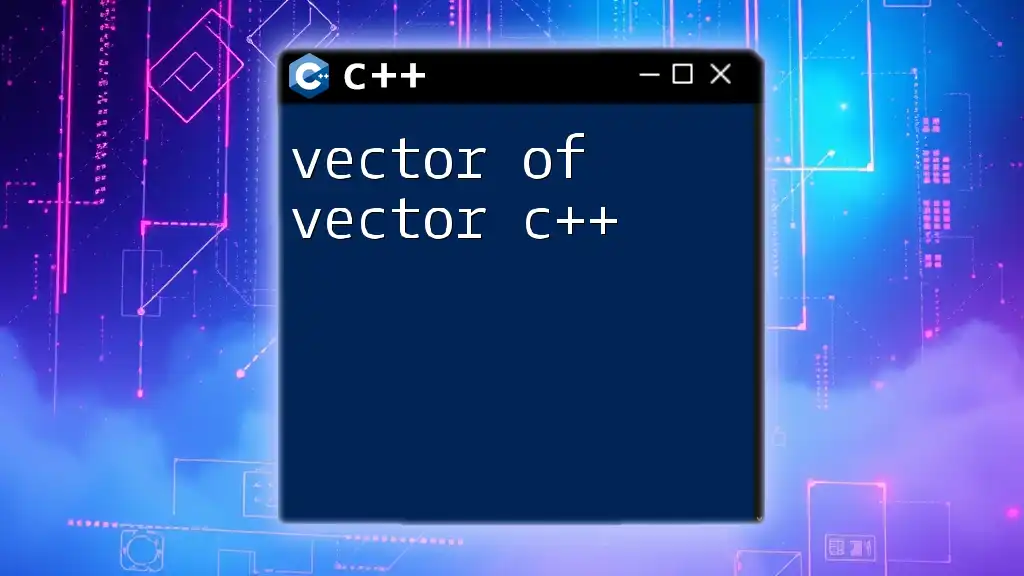
Conclusion
The ability to declare a 2D vector in C++ opens up a range of possibilities for dynamic data management. With flexibility in size and ease of access to elements, it's a valuable tool in any programmer's library. I encourage readers to experiment with the examples provided and explore the numerous functionalities available with 2D vectors. Happy coding!
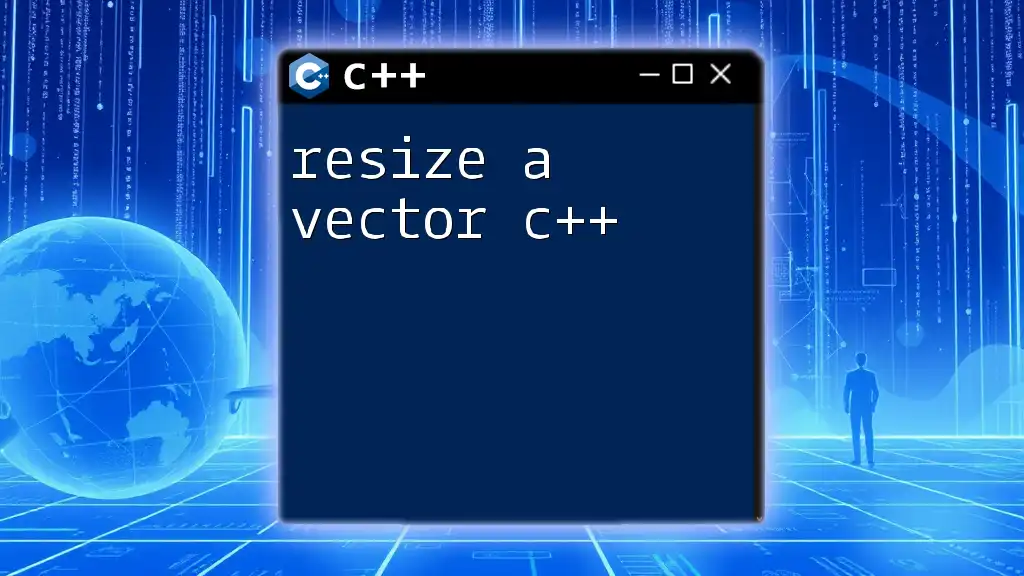
References
For further reading, I recommend exploring online resources, textbooks, and documentation that delve deeper into C++ vectors and best practices. Engaging with the programming community, through forums or contributions on platforms like Stack Overflow, can also be beneficial for expanding your knowledge.