Game engines that use C++ leverage the language's performance and flexibility, enabling developers to create efficient and complex gaming applications.
Here's a simple example of a C++ class used in a game engine:
class Player {
public:
void Move(float x, float y) {
position.x += x;
position.y += y;
}
private:
struct {
float x, y;
} position;
};
Understanding Game Engines
What is a Game Engine?
A game engine is a software framework designed for the development of video games. It provides developers with the necessary tools and functionalities to create, design, and manage games efficiently. The core components of a game engine typically include:
- Graphics Rendering: Handles visual output, allowing for stunning graphics and animations.
- Physics Simulation: Simulates real-world physics to create lifelike interactions.
- Audio Management: Manages sound effects and music, enhancing the gaming experience.
- AI: Implements artificial intelligence for in-game characters and systems.
- Networking: Facilitates online capabilities, enabling multiplayer experiences.
Why Use C++ in Game Development?
C++ has become the industry standard for many game engines due to its performance, control, and flexibility. Here are some key reasons to use C++:
- Performance Benefits: C++ is a highly efficient language, allowing for low-level memory manipulation and fast execution speeds. This is crucial for resource-intensive applications like games.
- Control and Flexibility: C++ provides developers with a greater degree of control over system resources and hardware, which is essential for optimizing game performance.
- Industry Standard for AAA Development: Many established companies prefer C++ due to its widespread adoption in professional game development.
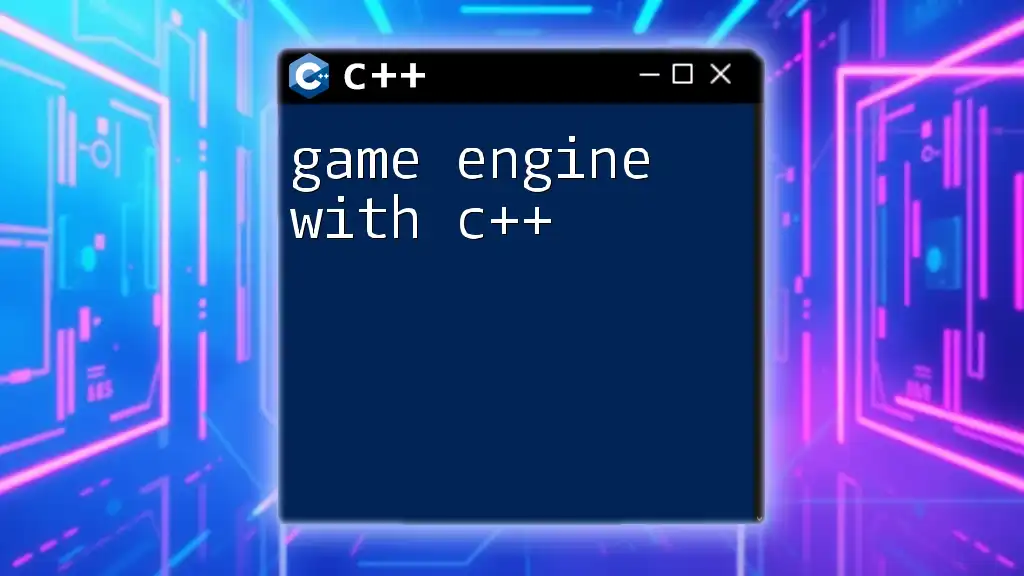
Popular Game Engines That Use C++
Unreal Engine
Overview
Unreal Engine, developed by Epic Games, is one of the most popular game engines on the market. It has a rich history, starting from its first release in 1998, and has evolved significantly. The engine is known for its:
- High-quality graphics and realism.
- Blueprints visual scripting system, which allows developers to create games without deep coding knowledge.
C++ in Unreal Engine
Unreal Engine leverages C++ to provide additional flexibility and performance beyond what Blueprints can offer. Developers can create custom classes and game mechanics directly in C++.
Code Example: Basic Class Creation
#include "GameFramework/Actor.h"
#include "MyActor.generated.h"
UCLASS()
class MYGAME_API AMyActor : public AActor {
GENERATED_BODY()
public:
AMyActor();
virtual void BeginPlay() override;
virtual void Tick(float DeltaTime) override;
};
Here, we define a basic actor class, which is the foundation for most game entities in Unreal Engine. The `BeginPlay()` and `Tick()` functions allow you to implement game logic and behavior during the game's runtime.
Pros and Cons
Advantages of using Unreal Engine include its powerful graphics capabilities and a large community for support. However, developers may face challenges such as a steeper learning curve and complex project setup.
Unity
Overview
Unity is widely recognized for its versatility and user-friendly interface. It supports a variety of genres, including 2D and 3D games, and is known for cross-platform compatibility, which allows developers to publish their games on multiple platforms with minimal changes.
C++ in Unity (Native Plugins)
While Unity primarily uses C#, developers can incorporate C++ through native plugins. This allows for performance-critical code to be executed in C++, enhancing overall performance.
Code Example: Simple Unity Plugin
extern "C" {
void UnityPluginLoad();
}
void UnityPluginLoad() {
// Initialization code here
}
This simple code snippet demonstrates how to declare a function that Unity will call when loading the plugin. By writing performance-critical components in C++, you can significantly boost your application's efficiency.
Pros and Cons
Unity's strengths lie in its asset store and vast resources for learning. However, C++ integration can be cumbersome, and some developers may find it challenging to maintain a hybrid codebase.
CryEngine
Overview
CryEngine is celebrated for its impressive rendering capabilities and is often used for developing visually stunning games. It has targeted a niche of developers focused on realistic graphics, particularly in first-person shooters.
C++ Usage in CryEngine
CryEngine facilitates extensive use of C++ for gameplay scripting. Its architecture allows developers to create highly customized game mechanics.
Code Example: Getting Started with CryEngine C++
#include <CryEntitySystem/IEntity.h>
void MyEntityScript::OnStart() {
IEntity* entity = GetEntity();
entity->SetName("MyEntity");
}
In this example, we define a script for an entity, demonstrating how to create and name game objects using C++.
Pros and Cons
The benefits of CryEngine include its visually stunning output and advanced graphics technology. However, it may pose challenges like limited community support compared to its competitors.
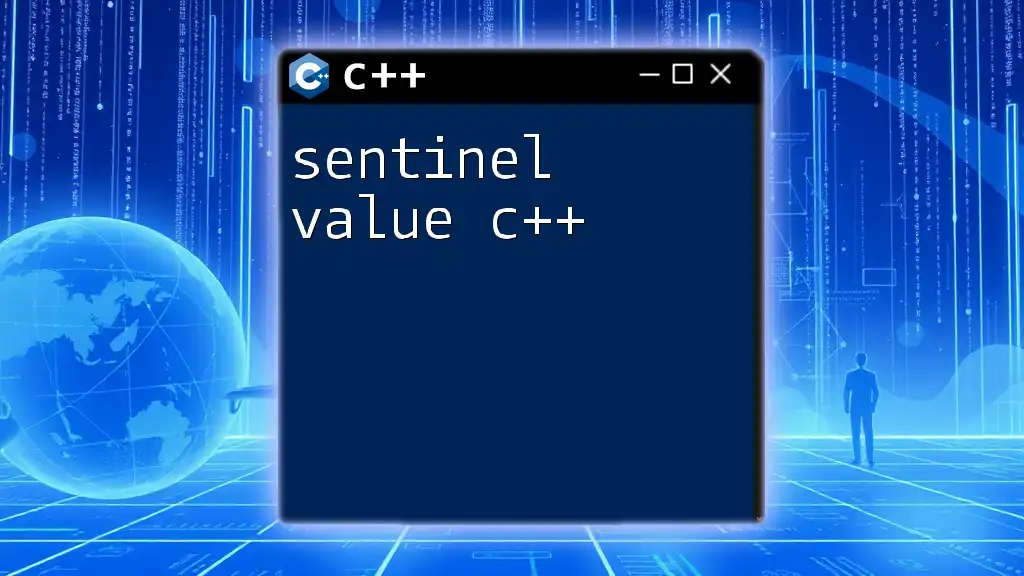
Other Notable Game Engines
Godot Engine
Overview
Godot is an open-source game engine that has gained popularity for its lightweight design and flexibility. It supports 2D and 3D development and is particularly favored by indie developers.
C++ in Godot
Godot incorporates C++ through GDNative, enabling developers to write performance-heavy scripts in C++ without being constrained by GDScript.
Code Example: GDNative C++ Script
#include <Godot.hpp>
using namespace godot;
class MyScript : public Reference {
GODOT_CLASS(MyScript, Reference)
public:
static void _register_methods() {
register_method("_ready", &MyScript::_ready);
}
void _ready() {
Godot::print("Hello, Godot with C++!");
}
};
This code snippet shows how to create a simple C++ class that integrates with Godot, demonstrating the ease of access to the engine's methods.
Torque3D
Overview
Torque3D is a versatile engine aimed at independent developers. It offers an extensive set of tools for creating both 2D and 3D games.
C++ Capabilities in Torque3D
Torque3D allows developers to implement extensive gameplay logic using C++.
Code Example: Simple Torque3D Function
function MyGame::startGame(%this) {
echo("Game Started!");
}
This function highlights how easily you can initiate game logic with simple C++ methods in Torque3D.
Panda3D
Overview
Panda3D is unique for its educational applications, making it an excellent choice for those interested in learning game development. Its user-friendly interface and powerful features make it easy to start.
C++ with Panda3D
Panda3D offers C++ interfaces for developers who wish to harness low-level performance without sacrificing ease of use.
Code Example: Simple Scene Setup
#include "pandaFramework.h"
#include "pandaSystem.h"
int main(int argc, char* argv[]) {
PandaFramework framework;
framework.open_framework(argc, argv);
framework.set_window_title("My Panda3D Game");
WindowFramework* window = framework.open_window();
return (framework.main_loop());
}
This code sets up a basic Panda3D application, emphasizing the straightforward nature of creating game windows and initiating game loops.
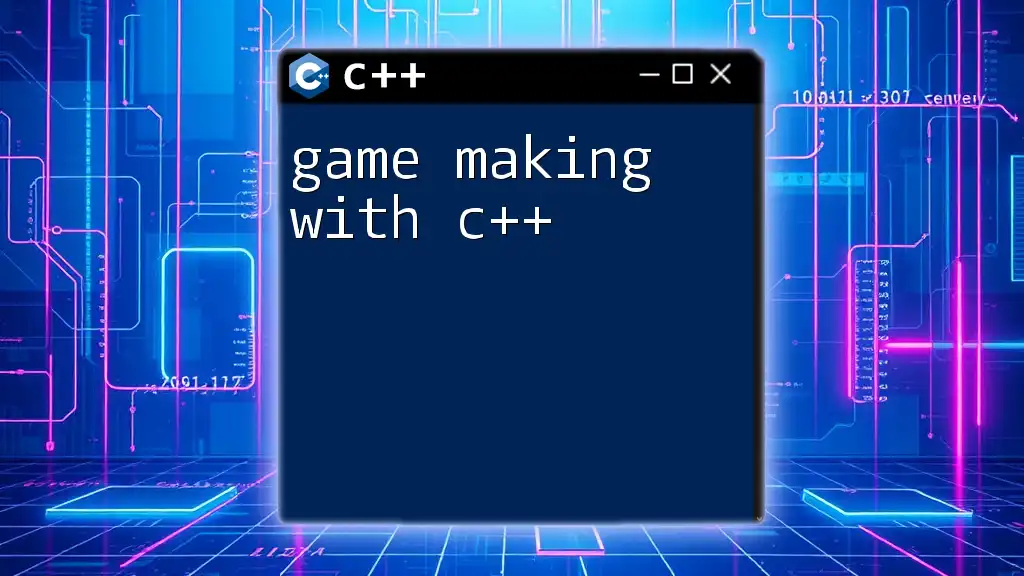
Conclusion
In conclusion, the world of game engines that use C++ is diverse and rich with opportunities for developers. Each engine has its unique strengths, whether it's Unreal Engine's high-quality graphics, Unity's versatility, or CryEngine's realism. Understanding these platforms and their integration with C++ can significantly enhance a developer's ability to create compelling games.
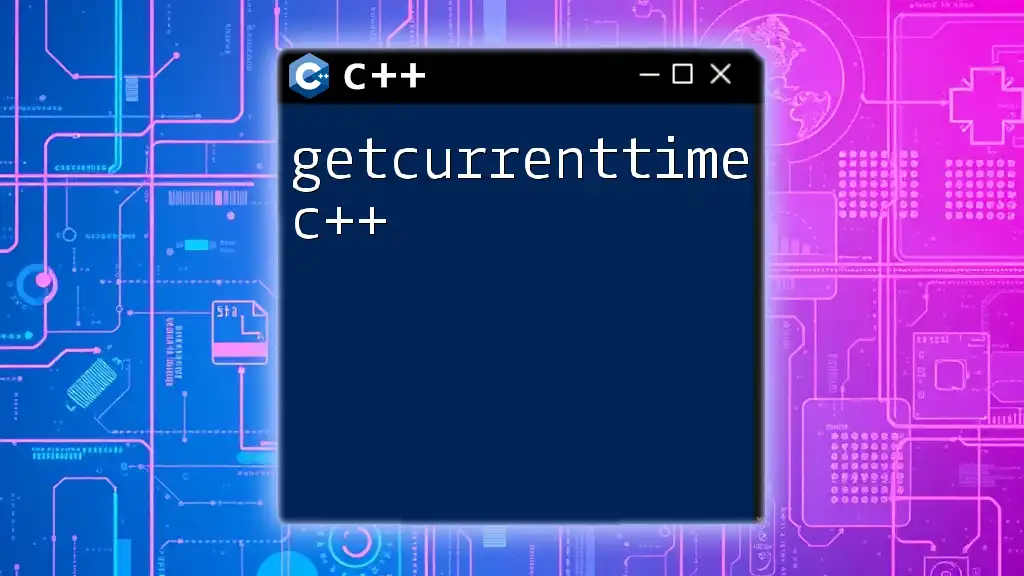
Resources and Further Learning
Several resources are available for learning more about game development and C++ integration. Online tutorials, books, and community forums provide substantial support for both newbies and experienced developers seeking to expand their knowledge.
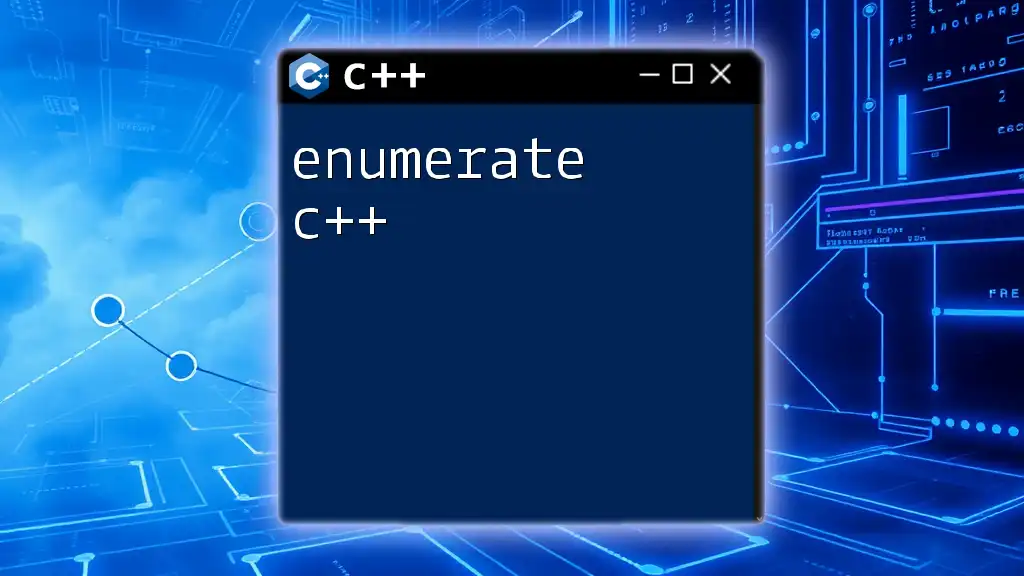
Call to Action
If you're interested in diving deeper into game development with C++, consider enrolling in our courses or subscribing to our newsletter for the latest guides and resources!