The Visitor Design Pattern in C++ allows you to separate an algorithm from the objects on which it operates, promoting ease of extension for new operations without altering the existing object structure.
#include <iostream>
#include <vector>
class Visitor; // Forward declaration
class Element {
public:
virtual void accept(Visitor &v) = 0; // Accept method
};
class ConcreteElementA : public Element {
public:
void accept(Visitor &v) override;
void operationA() { std::cout << "Operation A" << std::endl; }
};
class ConcreteElementB : public Element {
public:
void accept(Visitor &v) override;
void operationB() { std::cout << "Operation B" << std::endl; }
};
class Visitor {
public:
virtual void visit(ConcreteElementA &element) = 0;
virtual void visit(ConcreteElementB &element) = 0;
};
void ConcreteElementA::accept(Visitor &v) {
v.visit(*this); // Visitor calls visit for this element
}
void ConcreteElementB::accept(Visitor &v) {
v.visit(*this); // Visitor calls visit for this element
}
class ConcreteVisitor : public Visitor {
public:
void visit(ConcreteElementA &element) override {
element.operationA(); // Perform operation on Element A
}
void visit(ConcreteElementB &element) override {
element.operationB(); // Perform operation on Element B
}
};
int main() {
ConcreteElementA elementA;
ConcreteElementB elementB;
ConcreteVisitor visitor;
elementA.accept(visitor); // Visit Element A
elementB.accept(visitor); // Visit Element B
return 0;
}
What is the Visitor Pattern?
The Visitor Design Pattern is a behavioral design pattern that enables you to separate an algorithm from the object structure on which it operates. This is particularly beneficial when you want to add new operations to existing object structures without altering their classes.
By encapsulating operations within visitor classes, the pattern facilitates extensibility, allowing you to introduce and manage new operations easily. The primary essence of this pattern lies in allowing operations to "visit" various types of objects, enabling the addition of new operations without modifying the objects themselves.
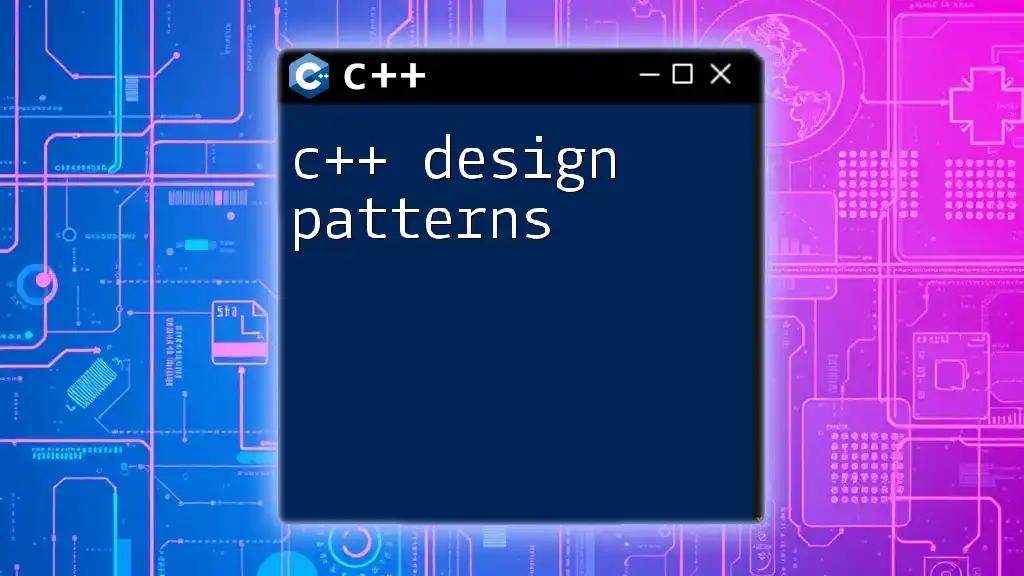
Why Use the Visitor Pattern in C++?
The Visitor Pattern is beneficial for several reasons:
- Separation of Concerns: It separates the operations performed on the objects from the object structure itself, promoting cleaner code and better organization.
- Extensibility: When you need to add new functionalities, you can do so by designing a new visitor rather than modifying existing objects, thereby adhering to the Open/Closed Principle.
- Centralization of Operations: By centralizing multiple operations on an object structure, you enhance manageability and readability of your code.
However, it is essential to avoid using this pattern when the object structure is subject to frequent changes. In such cases, modifying visitors may lead to increased complexity and maintenance difficulties.
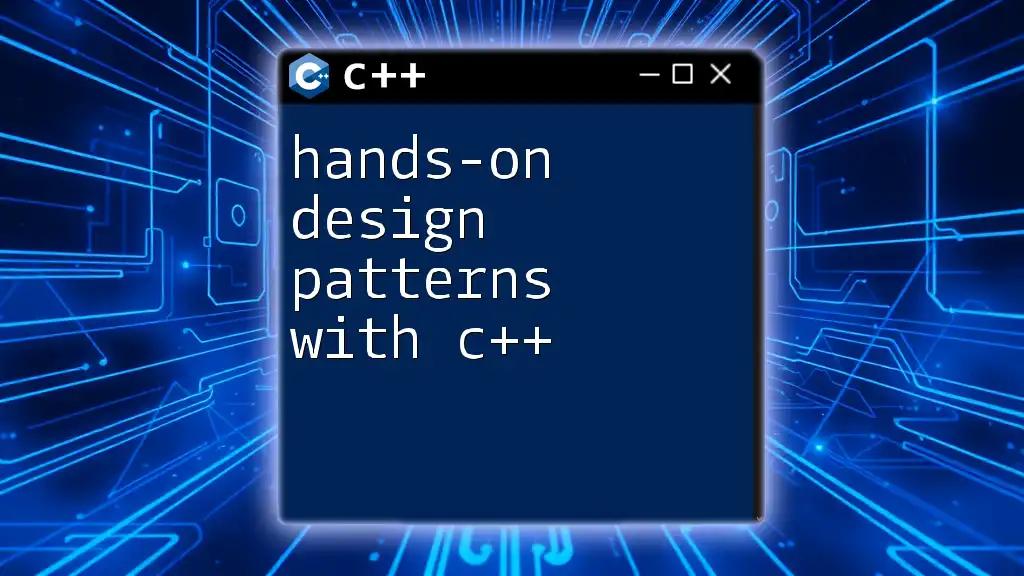
Components of the Visitor Pattern
The Visitor Pattern consists of several key components:
-
Visitor: An interface that declares a visit method for each type of Concrete Element in the object structure.
-
Element: An interface or abstract class that declares an accept operation, which takes a visitor as an argument.
-
Concrete Visitor: A class that implements the Visitor interface, defining specific operations on the elements.
-
Concrete Element: A class that implements the Element interface, enabling it to accept visitors.
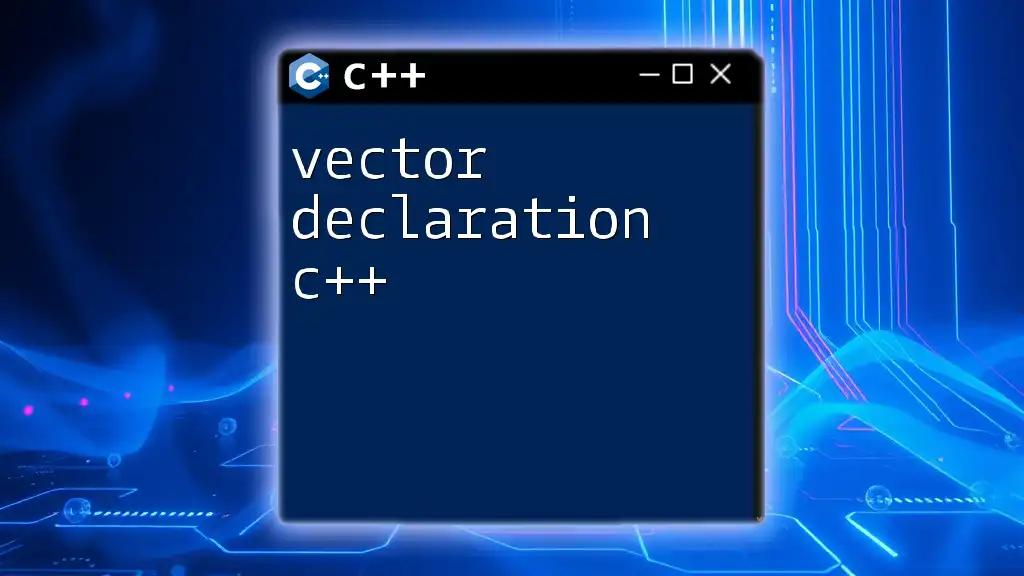
How Does the Visitor Pattern Work?
At the core of the Visitor Pattern is double dispatch, which allows a call to be resolved at runtime based on both the object being operated on and the type of operation being invoked. This is achieved through the accept and visit methods.
Here's how the flow of control works:
- The client calls the accept method on the Concrete Element.
- The accept method calls the visit method on the Concrete Visitor, passing `this` as an argument.
- The visit method in the Concrete Visitor uses the type of the Concrete Element to execute the specific operation.
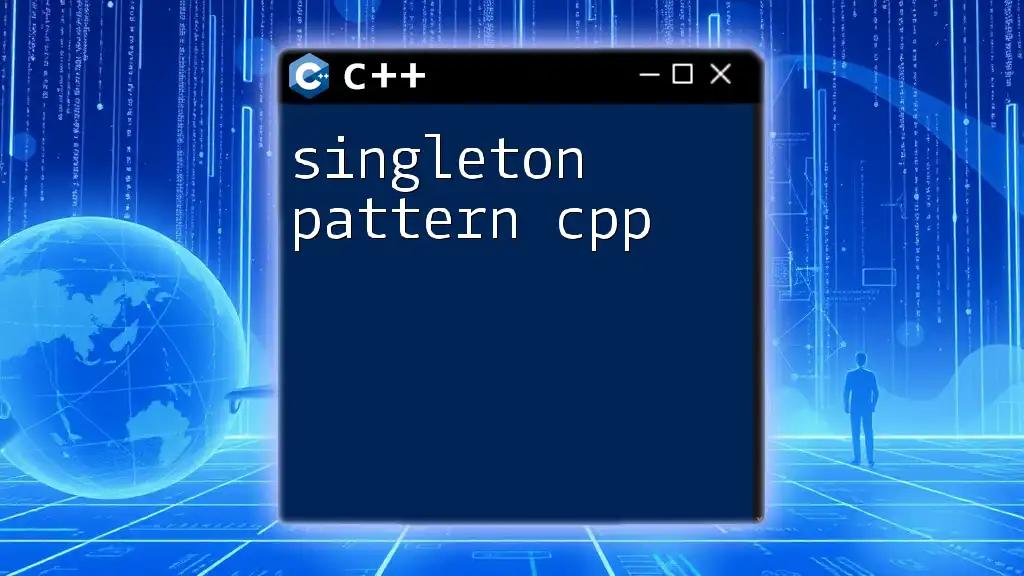
Implementing the Visitor Pattern in C++
Step-by-Step Implementation
Setting up the base classes for elements To implement the Visitor Pattern in C++, we start by declaring the base Element class:
class Shape {
public:
virtual void accept(class ShapeVisitor &v) = 0; // Abstract accept method
};
Creating the Visitor Interface Next, we define the Visitor interface that contains the visit methods for each Concrete Element:
class ShapeVisitor {
public:
virtual void visit(class Circle &c) = 0; // Visit Circle
virtual void visit(class Square &s) = 0; // Visit Square
};
Defining Concrete Elements
Now, let's create Concrete Element classes that inherit from the Shape interface:
class Circle : public Shape {
public:
void accept(ShapeVisitor &v) override {
v.visit(*this); // Accepts a visitor
}
// Additional Circle-specific methods...
};
class Square : public Shape {
public:
void accept(ShapeVisitor &v) override {
v.visit(*this); // Accepts a visitor
}
// Additional Square-specific methods...
};
Creating Concrete Visitors
Now, let's create a Concrete Visitor that implements operations on these elements. For example, an `AreaCalculator` visitor that calculates the area of Shapes:
class AreaCalculator : public ShapeVisitor {
public:
void visit(Circle &c) override {
// Area calculation for Circle
double area = 3.14 * c.getRadius() * c.getRadius();
std::cout << "Area of Circle: " << area << std::endl;
}
void visit(Square &s) override {
// Area calculation for Square
double area = s.getSide() * s.getSide();
std::cout << "Area of Square: " << area << std::endl;
}
};

Example Use Case of the Visitor Pattern
Case Study: Shape Visitor
In this case study, let's model a scenario where we need to handle multiple operations on various shapes such as calculating their areas or drawing them.
-
Shape Interface We start with our Shape interface as previously defined.
-
Circle and Square Classes Here’s how we define the `Circle` and `Square` classes and their methods:
class Circle : public Shape {
private:
double radius;
public:
Circle(double r) : radius(r) {}
double getRadius() { return radius; }
void accept(ShapeVisitor &v) override {
v.visit(*this);
}
};
class Square : public Shape {
private:
double side;
public:
Square(double s) : side(s) {}
double getSide() { return side; }
void accept(ShapeVisitor &v) override {
v.visit(*this);
}
};
-
Visitor Interface for Shapes As previously defined, our `ShapeVisitor` with the visit methods.
-
Concrete Visitor implementation Here’s the `AreaCalculator` visitor:
class AreaCalculator : public ShapeVisitor {
public:
void visit(Circle &c) override {
double area = 3.14 * c.getRadius() * c.getRadius();
std::cout << "Area of Circle: " << area << std::endl;
}
void visit(Square &s) override {
double area = s.getSide() * s.getSide();
std::cout << "Area of Square: " << area << std::endl;
}
};
Testing the Visitor Pattern Implementation
Here’s how you might set up a simple main function to demonstrate the use of the Visitor pattern:
int main() {
Circle circle(5);
Square square(4);
AreaCalculator areaCalculator;
circle.accept(areaCalculator); // Calculate area of Circle
square.accept(areaCalculator); // Calculate area of Square
return 0;
}
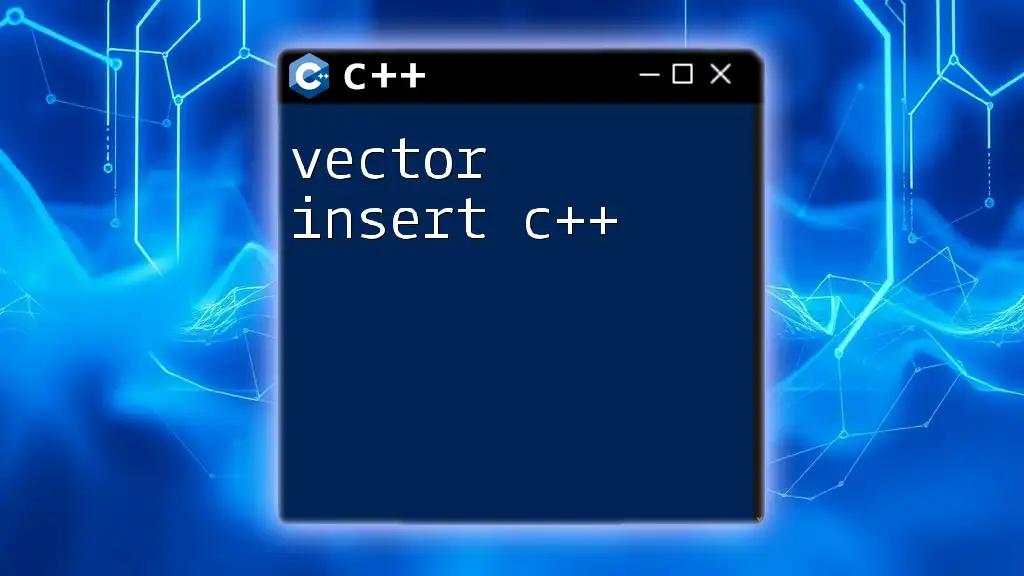
Advantages and Disadvantages of the Visitor Pattern in C++
Pros of Using the Visitor Pattern
- Improved Maintainability and Extensibility: The pattern enables adding new operations easily without modifying existing codes.
- Clarity of Responsibilities: It gives clear responsibility by segregating operations from the object structure.
Cons of Using the Visitor Pattern
- Increased Complexity: The pattern introduces additional classes and relationships which might complicate the design.
- Difficulties in Adding New Elements: For new elements, all existing visitors need to be updated to handle them, which can lead to an extensive maintenance burden.

Real-World Applications of the Visitor Design Pattern
Examples in Software Engineering
- Parsing Compilers: The Visitor pattern is widely used in compiler design where various visitors can evaluate expressions, check syntax, etc.
- Graphical User Interface Elements: In UI frameworks, the Visitor can be used to perform different operations on various UI components, facilitating easy updates and modifications.
- Object-Oriented Design Patterns: Many design patterns can leverage the Visitor design pattern to provide more elaborate capabilities for object manipulation.
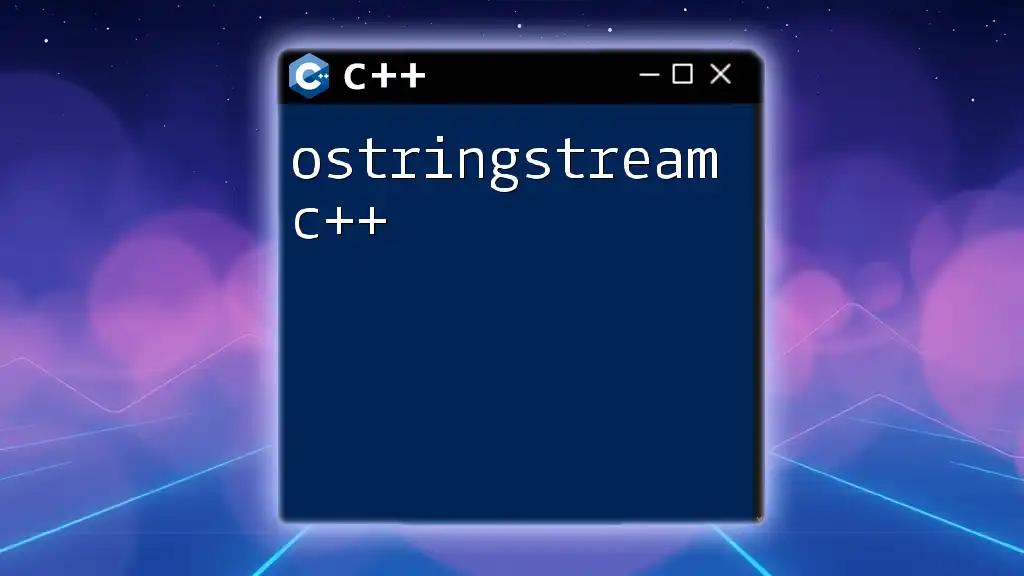
Conclusion
The Visitor Design Pattern in C++ presents a powerful means of separating operations from the structure of objects and alerts developers to consider extensibility when designing systems. By allowing new operations to be added easily without altering the existing object structure, it fosters a cleaner, more organized programming environment. However, caution is warranted to judge when to apply this pattern, particularly in contexts where changes to object structures are frequent. By navigating its advantages and disadvantages, programmers can make informed decisions about employing this design pattern effectively.

References
Books and Resources for Further Learning
- "Design Patterns: Elements of Reusable Object-Oriented Software" by Erich Gamma et al.
- "Head First Design Patterns" by Eric Freeman and Bert Bates.
- Online tutorials and resources on Object-Oriented Design Patterns in C++.