The design and evolution of C++ reflects its transition from a simple enhancement of C to a powerful multi-paradigm programming language that supports procedural, object-oriented, and generic programming, exemplified by features like classes and templates.
#include <iostream>
#include <vector>
class Example {
public:
void display(const std::vector<int>& numbers) {
for (const auto& num : numbers) {
std::cout << num << " ";
}
std::cout << std::endl;
}
};
int main() {
Example example;
example.display({1, 2, 3, 4, 5});
return 0;
}
The Birth of C++
The design and evolution of C++ began in the early 1980s amidst the growing needs for more sophisticated programming paradigms. Prior to C++, the prevalent language was C, which was primarily procedural. However, the industry was increasingly leaning towards Object-Oriented Programming (OOP) to enhance code reusability and modularity.
Historical Context of C++
As software systems became more complex, the limitations of C became evident. Engineers required features that allowed them to model real-world entities more intuitively. This led to the birth of OOP, which provided a foundation for encapsulating data and behaviors together. Bjarne Stroustrup saw this need and began working on what would become C++. In this early stage, C++ was simply an extension of C, adding OOP features like classes and objects.
Bjarne Stroustrup and the Foundations of C++
Bjarne Stroustrup, a Danish computer scientist, began developing C++ in 1979 while working on his doctoral thesis. His vision was to create a language that combined the performance and efficiency of C with the capabilities of OOP. The result was a language that retained C's syntax but introduced class concepts for encapsulation, allowing developers to create new data types.

Key Design Principles of C++
The design and evolution of C++ is marked by several key principles that facilitate diverse programming styles and enhance performance.
Multi-Paradigm Approach
One of the defining features of C++ is its multi-paradigm approach. It supports:
- Procedural Programming: Developers can write straightforward procedural code.
- Object-Oriented Programming (OOP): The ability to define classes and objects lets programmers use real-world modeling.
- Generic Programming: Allows the creation of templates that work with any data type.
This versatility makes C++ suitable for a wide range of applications, from system programming to game development.
Resource Management and Performance
C++ was designed with a strong emphasis on resource management and performance. The language enables programmers to manage resources, such as memory, efficiently.
Throwing light on this principle, we can observe how constructors and destructors play a crucial role in manual memory management.
class Resource {
public:
Resource() {
// Allocate resources
}
~Resource() {
// Free resources
}
};
In this code, the constructor allocates resources upon object instantiation, while the destructor deallocates them, preventing memory leaks.
Backward Compatibility with C
A critical aspect of C++ is its backward compatibility with C. This feature allows developers to port existing C codebases to C++ without extensive rewrites. By maintaining a familiar syntax and core features, C++ successfully bridges the gap between the two languages. However, this also posed challenges, as many low-level features in C could lead to programming errors that are harder to catch.

Major Evolutionary Milestones
The design and evolution of C++ can be marked by significant milestones that shaped its trajectory.
C++98: The First Standardization
C++98 saw the first official standardization of the language, introducing key features like:
- Templates: Enabling generic programming, allowing functions and classes to operate with any data type.
- The Standard Template Library (STL): Providing a rich set of libraries, including algorithms and data structures, to improve code efficiency.
C++03: Minor Updates and Fixes
C++03 brought minor updates and fixes to the language but no major new features. The primary focus was on resolving issues and enhancing existing functionality.
C++11: A New Era of C++
C++11 represented a significant overhaul of the language, introducing numerous features that modernized C++.
Key Features:
- Lambda Expressions: Allowing concise function definitions, particularly useful for functional programming paradigms.
- Smart Pointers: Providing automated memory management through shared_ptr and unique_ptr.
An example of a lambda expression is as follows:
auto add = [](int a, int b) {
return a + b;
};
This code snippet illustrates the use of lambda expressions to create anonymous functions.
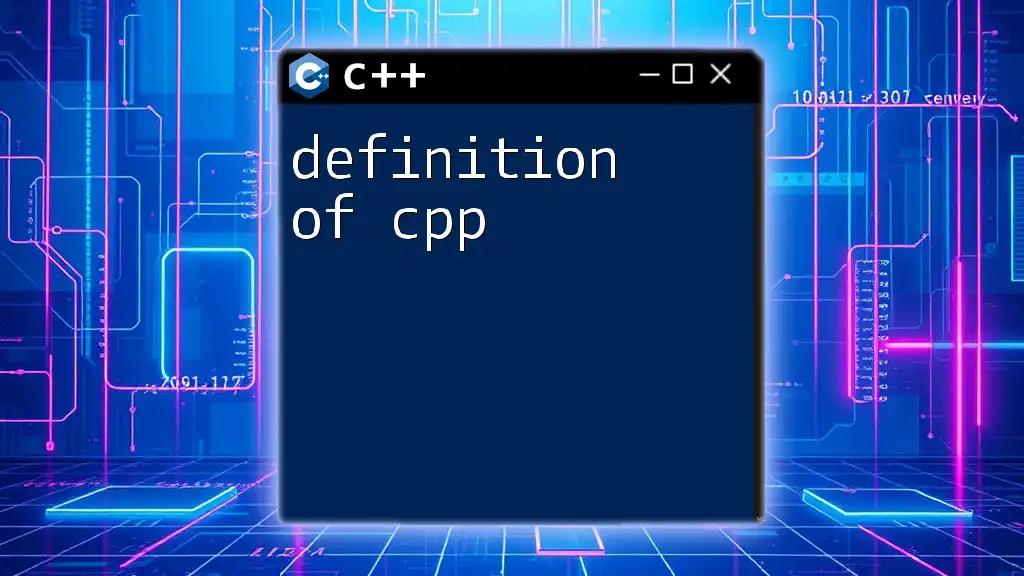
Innovations in Recent Standards
The design and evolution of C++ continued to evolve with newer standards introducing even more innovations.
C++14: Incremental Improvements
While not as extensive as C++11, C++14 made meaningful contributions, including generic lambda expressions and relaxed constexpr, allowing for greater compile-time computation.
C++17: Introduction of Parallel Algorithms
C++17 focused on performance improvements by introducing parallel algorithms, making it easier for developers to optimize their applications. For example, using `std::for_each` in parallel:
#include <execution>
std::for_each(std::execution::par, data.begin(), data.end(), [](int& n) { n *= 2; });
This snippet showcases how to apply algorithms in parallel, enhancing performance for large datasets.
C++20: Concepts, Ranges, and Coroutines
With the introduction of C++20, the language leveraged new ways to improve code quality and efficiency:
- Concepts: Enabling constraints on template types, offering clearer error messages and better code documentation.
- Ranges: Providing a more intuitive way to work with sequences of data.
- Coroutines: Allowing asynchronous programming more naturally, which is crucial for modern applications.

The Role of the Community and Library Development
The design and evolution of C++ has been significantly influenced by community contributions and the development of libraries.
Standardization Process
The ISO C++ Committee, composed of members from industry and academia, plays a crucial role in evolving the language standard. The collaborative effort leads to a thorough review process that ensures improvements meet the needs of programmers.
Open Source Contributions and Libraries
The rise of open-source libraries has been pivotal in extending C++'s capabilities. Prominent libraries such as Boost and Qt provide an extensive range of additional functionalities that enhance productivity and code quality. For example, using Boost for graph algorithms can simplify complex tasks:
#include <boost/graph/adjacency_list.hpp>
using Graph = boost::adjacency_list<>;
Here, the Boost library offers a powerful data structure for graph handling, showcasing C++'s extensibility through community efforts.

The Future of C++
Looking ahead, the design and evolution of C++ poses exciting opportunities. Key trends include:
- Increasing Focus on Compile-Time Programming: C++ has always influenced compile-time operations, but future enhancements are expected to expand this further.
- Enhanced Support for Functional and Concurrent Programming: As applications become more concurrent in design, C++ will evolve to facilitate better support for functional programming paradigms and concurrent execution.
C++23 and Beyond
Anticipated new features in C++23 and beyond are intriguing, as discussions within the community continue to highlight potential innovations such as improved language usability and further enhancements to algorithms and data structures.

Conclusion
The design and evolution of C++ has been a remarkable journey driven by the need for efficient, flexible, and powerful programming paradigms. From its initial inception by Bjarne Stroustrup to its current state as a multi-paradigm language, C++ continues to adapt and grow. As developers embrace its capabilities and push the boundaries of its design, the future promises to be as rich and varied as its past. Embracing C++ in your skill set can open countless doors in the ever-evolving landscape of software development.