In this post, we'll cover essential interview questions related to multithreading in C++, along with a concise code snippet demonstrating the use of threads using the C++11 standard.
#include <iostream>
#include <thread>
void function_to_run() {
std::cout << "Hello from the thread!" << std::endl;
}
int main() {
std::thread myThread(function_to_run);
myThread.join(); // Wait for the thread to finish
return 0;
}
Understanding Multithreading in C++
What is Multithreading?
Multithreading is a programming capability that allows multiple threads to exist within a single process, executing concurrently. This parallel execution enables better resource utilization and application responsiveness, especially in modern multi-core processors. As applications grow increasingly complex, understanding multithreading is vital for optimizing performance and managing tasks efficiently.
Key Concepts in Multithreading
Threads
A thread is the smallest unit of processing that can be scheduled by an operating system. Threads are not independent; they share the same data space, which allows for efficient communication. However, this also introduces challenges, such as managing access to shared data.
Concurrency vs. Parallelism
It's essential to understand the difference between concurrency and parallelism:
- Concurrency refers to the ability of a system to manage multiple tasks at once. It may involve switching between tasks without executing them at the same time.
- Parallelism, on the other hand, refers to the simultaneous execution of multiple tasks, typically on different cores.
Using real-world analogies can be helpful. For instance, if a chef prepares multiple dishes one after another, that’s concurrency. However, if two chefs are cooking simultaneously, that’s parallelism.
C++ Multithreading Basics
The C++ Thread Library
The inclusion of the `<thread>` library in C++11 dramatically enhanced multithreading capabilities. It simplifies the creation and management of threads. A simple example of creating a thread is as follows:
#include <iostream>
#include <thread>
void hello() {
std::cout << "Hello from thread!" << std::endl;
}
int main() {
std::thread t(hello);
t.join(); // Wait for the thread to finish
return 0;
}
In this example, the `hello` function is run in a separate thread, demonstrating how easy it is to invoke multithreading using C++.

Common Multithreading Interview Questions
Basic Level Questions
What is the difference between a thread and a process?
The distinction between a thread and a process is fundamental. A process is an independent entity with its own memory space, while a thread is a smaller unit that runs within a process, sharing the same memory.
To illustrate, consider an application as a building (the process) with several rooms acting as threads. While all rooms can function independently, they still share the building's infrastructure (memory).
How can you create a thread in C++?
There are several ways to create threads in C++. Using the `std::thread` class is one of the most common approaches. For instance, you can pass a function or a lambda expression to initialize a thread.
Intermediate Level Questions
What are race conditions, and how can they be avoided?
A race condition occurs when two or more threads attempt to modify shared data concurrently, leading to unpredictable results. For example, if two threads increment a shared variable simultaneously without proper synchronization, the final value may not be as expected.
To prevent race conditions, you can utilize mutexes (mutual exclusions). A mutex locks a section of code so that only one thread can access it at a time. Here’s an example:
#include <mutex>
std::mutex mtx; // Mutex for critical section
int counter = 0;
void safe_increment() {
std::lock_guard<std::mutex> lock(mtx); // Lock the mutex
++counter; // Critical section
}
This function ensures that increments to `counter` happen safely across different threads.
Explain the purpose of mutexes in multithreading.
Mutexes serve as a locking mechanism to prevent multiple threads from accessing critical sections of code simultaneously. When a thread locks a mutex, other threads attempting to lock that mutex will be blocked until it is unlocked. This is crucial for maintaining data integrity when multiple threads interact with shared resources.
Advanced Level Questions
What is deadlock, and how can it be prevented?
A deadlock occurs when two or more threads are unable to proceed because they are each waiting for the other to release resources. An example is when Thread A holds Lock 1 and waits for Lock 2, while Thread B holds Lock 2 and waits for Lock 1.
To prevent deadlocks, consider the following strategies:
- Lock Ordering: Always acquire locks in a consistent order across threads.
- Timeouts: Implement timeout mechanisms to avoid indefinite waiting.
- Resource Allocation Graphs: Use algorithms that detect and prevent cycles in resource allocation.
How does the C++ Standard Library handle thread management?
The C++ Standard Library provides comprehensive tools for thread management, from creating threads with `std::thread` to synchronizing them with mutexes and condition variables. These features simplify multithreading significantly and handle many underlying complexities for the developer.
Describe Condition Variables and their usage.
Condition variables are synchronization primitives that block a thread when a specified condition is not met. They enable threads to wait until they are notified by another thread. This is particularly useful in producer-consumer scenarios. Here's how a condition variable is used:
#include <condition_variable>
#include <queue>
std::queue<int> q;
std::mutex mtx;
std::condition_variable cv;
void producer() {
while (true) {
std::unique_lock<std::mutex> lock(mtx);
q.push(1); // Produce an item
cv.notify_one(); // Notify one consumer
}
}
void consumer() {
while (true) {
std::unique_lock<std::mutex> lock(mtx);
cv.wait(lock, [] { return !q.empty(); }); // Wait for an item
q.pop(); // Consume item
}
}
In this example, the producer thread adds items to a queue and signals the consumer thread when an item is available. The consumer waits if the queue is empty.

Tips for Answering Multithreading Questions in Interviews
Understand the Fundamentals
To effectively tackle multithreading interview questions, having a solid grasp of core concepts such as threads, processes, and concurrency is crucial. This foundational knowledge will give you the confidence to address more advanced queries.
Provide Code Examples
Illustrating your answers with code examples is key. Visual demonstrations can clarify concepts you explain verbally, making it easier for interviewers to see your understanding.
Communicate Effectively
Lastly, focus on clear communication. When explaining complex concepts during interviews, aim to break down the information into digestible pieces. This will not only showcase your knowledge but also your ability to convey ideas clearly.
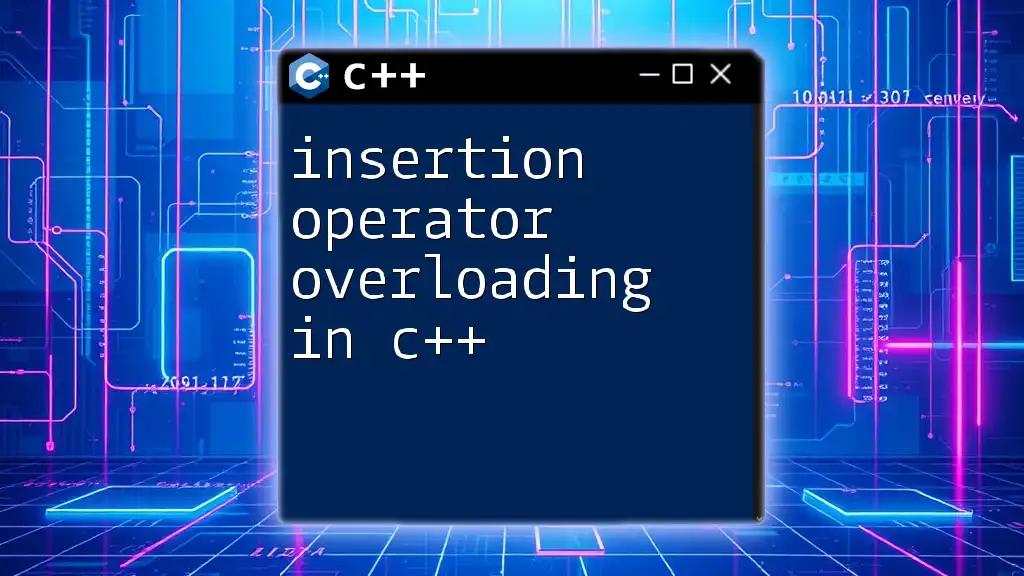
Conclusion
Mastering multithreading in C++ is an essential aspect of becoming a proficient developer. Understanding various concepts and preparing for interview questions on multithreading in C++ can set you apart in the job market. Continuous practice and exploration of practical scenarios will further solidify your expertise in this vital area of programming.