A C++ Standard Template Library (STL) cheat sheet provides a quick reference to essential containers and algorithms for efficient programming.
Here's a simple code snippet demonstrating the use of a vector and an algorithm from the STL:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {5, 3, 8, 1};
std::sort(numbers.begin(), numbers.end());
for(int num : numbers) {
std::cout << num << " ";
}
return 0;
}
What is STL?
The Standard Template Library (STL) is a powerful library in C++ that provides a collection of template classes and functions. It allows programmers to use and manage various data structures and algorithms without having to code them from scratch. By utilizing STL, developers can focus on implementing the unique features of their applications rather than reinventing the wheel.
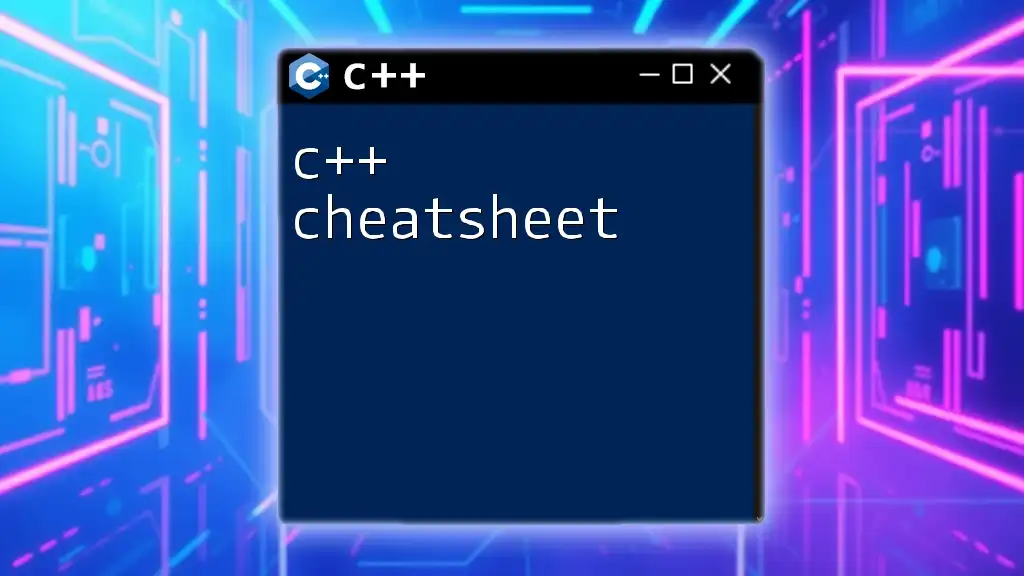
Benefits of Using STL
Using STL comes with several significant advantages:
-
Code Reusability: STL provides a set of pre-built data structures (such as vectors, lists, and maps) and algorithms (like sorting and searching) which can be reused in different programs, promoting efficient programming.
-
Performance: STL is designed for high performance with algorithms optimized for efficiency, which can lead to faster runtime compared to custom implementations.
-
Functionality: STL encompasses a wide range of utilities for data handling, making it easier for programmers to manipulate complex data in a straightforward manner.
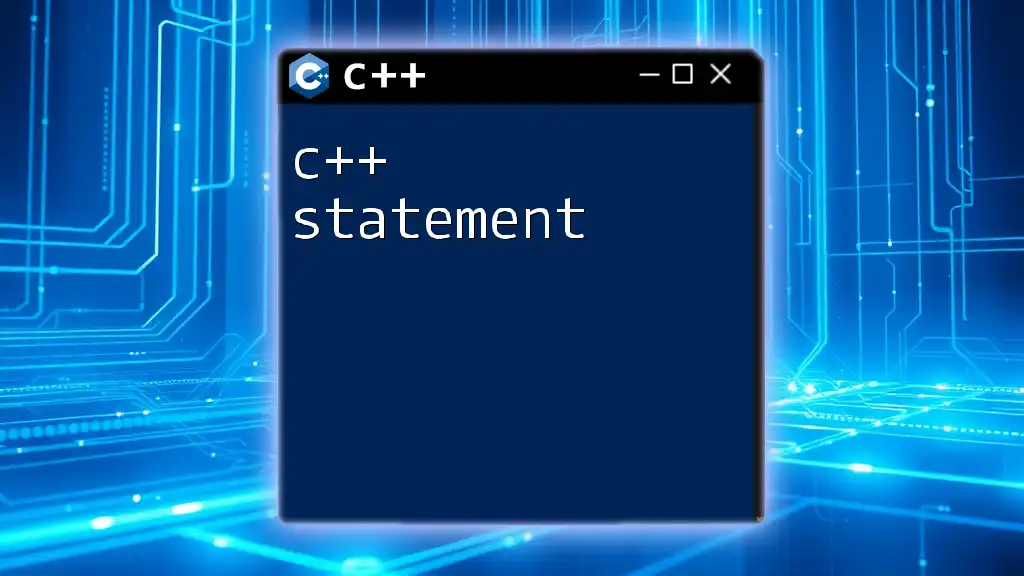
Core Components of STL
Containers
Containers in STL store and manage data. There are three main types of containers:
-
Sequential Containers: These store elements in a linear order. Common examples include:
- Vector: Dynamic array that can resize automatically.
- List: Doubly linked list used for frequent insertions and deletions.
- Deque: Double-ended queue that allows insertion and deletion from both ends.
-
Associative Containers: These store elements in key-value pairs, allowing fast access based on keys. Examples include:
- Set: Collection of unique keys.
- Map: Collection of key-value pairs where keys are unique.
- Multiset and Multimap: Allow duplicate keys.
-
Unordered Containers: These use hash tables for faster access. Examples include:
- Unordered Set: Similar to set but does not maintain order.
- Unordered Map: Similar to map but does not maintain order.
Iterators
Iterators provide a standard way of accessing elements in containers without exposing the underlying structure. They can be classified into several types:
- Input Iterators: Allow reading elements in a forward direction.
- Output Iterators: Allow writing elements in a forward direction.
- Forward Iterators: Allow both reading and writing in a forward direction.
- Bidirectional Iterators: Allow reading and writing in both forward and backward directions.
- Random Access Iterators: Provide the ability to access elements at arbitrary positions in constant time.
Algorithms
STL includes a rich set of algorithms for manipulating data. Some commonly used algorithms are:
- Sorting Algorithms: Use `sort()` for sorting elements in a container.
- Searching Algorithms: Use `find()` for locating an element and `binary_search()` for searching in a sorted container.
- Modification Algorithms: Use `copy()` to replicate elements from one container to another and `transform()` to apply a function on elements.
- Numeric Algorithms: Use `accumulate()` to sum up values in a container or `inner_product()` for computing dot products of two ranges.
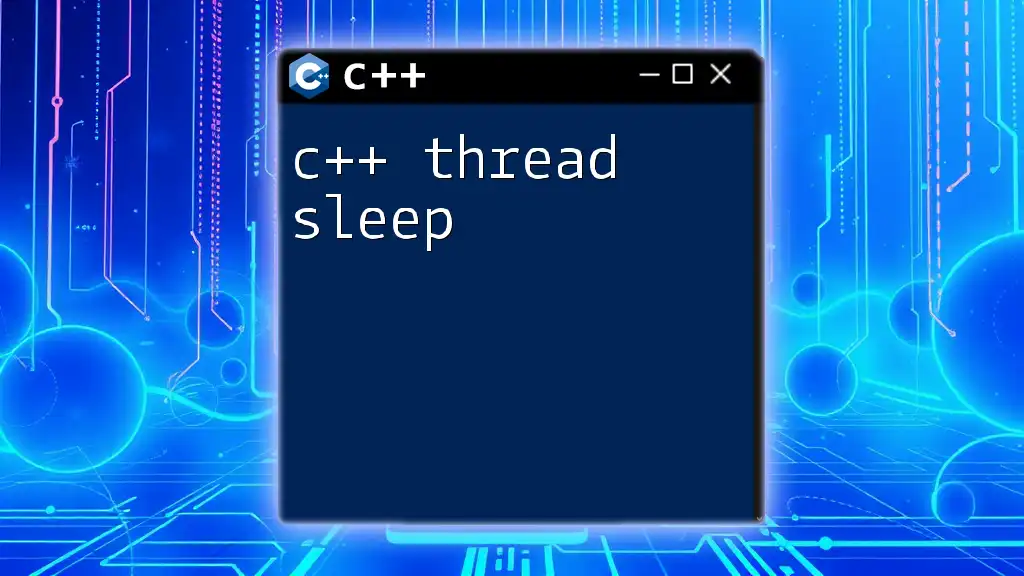
Using STL in C++
Basic Operations with Containers
Vectors
Vectors are flexible and dynamically sized array containers. Here's how to declare and use them:
Declaration:
#include <vector>
std::vector<int> numbers;
Adding Elements: You can add an element to the end of the vector using `push_back()`:
numbers.push_back(10);
Accessing Elements: You can access elements in a vector using the index operator:
int firstNumber = numbers[0]; // Accessing first element
Maps
Maps are associative containers that store elements as key-value pairs.
Declaration:
#include <map>
std::map<std::string, int> age;
Inserting Key-Value Pairs: You can insert a new key-value pair like so:
age["Alice"] = 30; // Assigning value 30 to the key "Alice"
Accessing Values: To retrieve a value associated with a specific key:
int alicesAge = age["Alice"];
STL Iterators and Their Usage
Using Iterators with Vectors
Iterators can be used to traverse through containers. Here’s how you can iterate through a vector:
for (std::vector<int>::iterator it = numbers.begin(); it != numbers.end(); ++it) {
std::cout << *it << " "; // Outputting each element
}
Using Iterators with Maps
You can use iterators to go through a map as follows:
for (const auto& pair : age) {
std::cout << pair.first << ": " << pair.second << "\n"; // Outputting key-value pairs
}
Practical Examples
Example 1: Sorting a Vector
Sorting a vector is straightforward with STL. Here’s a simple implementation:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {4, 1, 3, 2};
std::sort(vec.begin(), vec.end()); // Sorting the vector
for (const auto& num : vec) {
std::cout << num << " "; // Outputting sorted elements
}
return 0;
}
Example 2: Counting Occurrences using Map
You can use a map to count occurrences of words in a string:
#include <iostream>
#include <map>
#include <string>
#include <sstream>
int main() {
std::map<std::string, int> countWords;
std::string text = "hello world hello";
std::string word;
std::istringstream stream(text);
while (stream >> word) {
countWords[word]++; // Incrementing the count of each word
}
for (const auto& pair : countWords) {
std::cout << pair.first << ": " << pair.second << "\n"; // Outputting each word and its count
}
return 0;
}
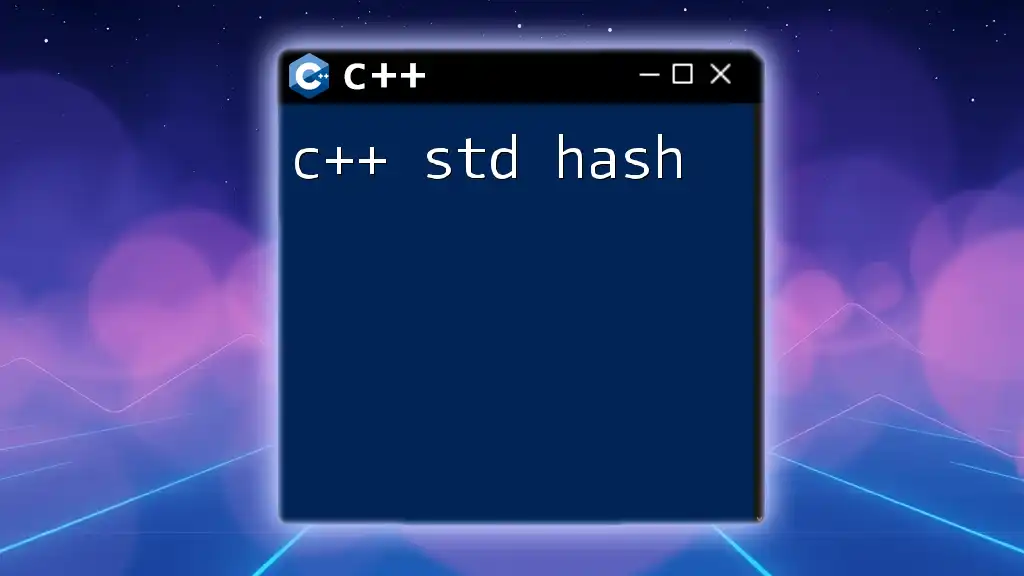
Conclusion
In summary, understanding the C++ STL is crucial for writing efficient and maintainable code. By leveraging its components—containers, iterators, and algorithms—you can significantly improve your coding effectiveness.
This guide serves as a C++ STL Cheat Sheet, providing essential insights into STL's components and operations. As you advance in your programming journey, consider exploring these tools further by building projects and experimenting with different features.
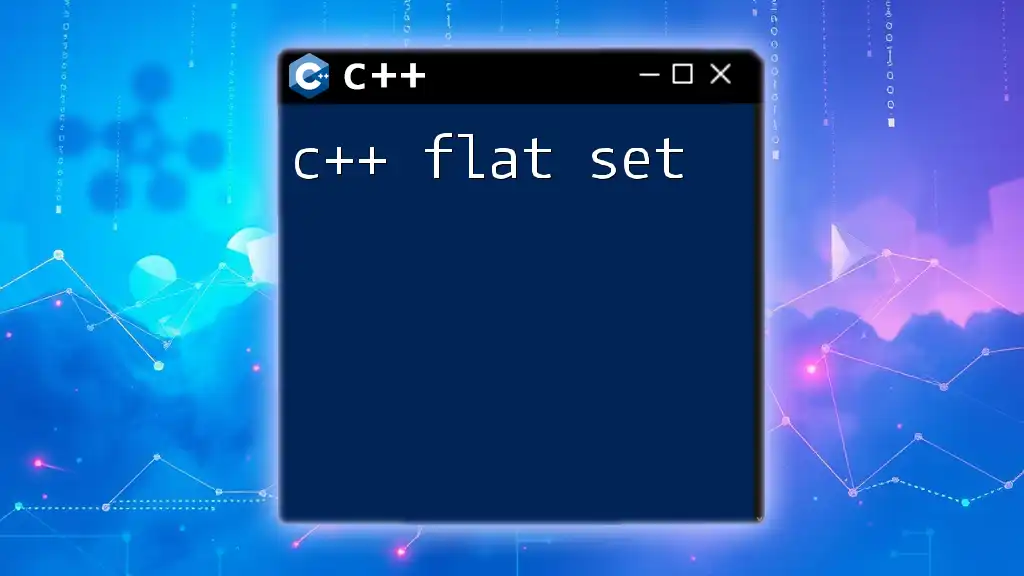
Additional Resources
For further learning, consider exploring the official C++ documentation on STL features and seek tutorials that provide coding exercises and challenges. Engaging with communities that focus on C++ can also enhance your skills and understanding of STL.