In C++, the shift left operator (`<<`) is used to perform a bitwise left shift on a binary number, effectively multiplying the number by two for each shift, as shown in the following code snippet:
#include <iostream>
int main() {
int num = 5; // binary: 0101
int result = num << 1; // result: 1010 (binary), which is 10 in decimal
std::cout << "Result after shifting left: " << result << std::endl;
return 0;
}
What is the Left Shift Operator in C++?
Definition of Left Shift
The left shift operator in C++ is represented by `<<`. This operator allows you to shift all bits in a binary number to the left by a specified number of positions. Each left shift effectively multiplies the number by 2 for every position shifted, making it a powerful tool for bit manipulation.
Syntax of the Left Shift Operator
The general syntax for using the left shift operator in C++ is as follows:
int result = value << numberOfPositions;
Here, `value` is the number we want to shift, and `numberOfPositions` specifies how many places we want to shift the bits to the left.
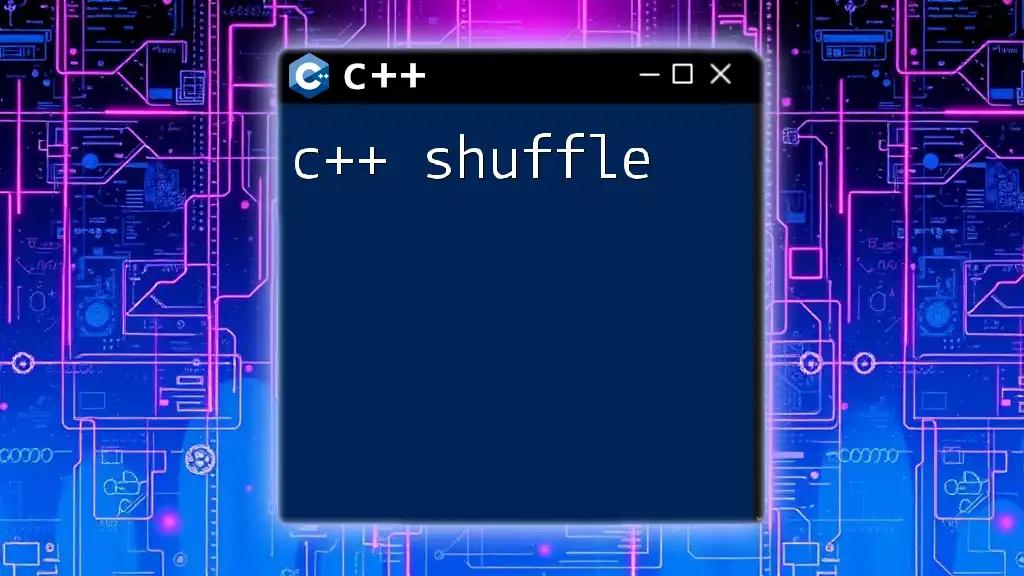
How the Left Shift Works
Understanding Bit Manipulation
To fully grasp the `c++ shift left`, it helps to visualize how numbers are represented in binary. Each bit in a binary number represents an increasing power of 2. For example, the number 5 in binary is represented as `00000101`. When we perform a left shift on this number:
// Original Value: 5 (Binary: 00000101)
int num = 5;
int shifted = num << 1; // Result: 10 (Binary: 00001010)
In this code, the original binary value `00000101` is shifted one place to the left, producing `00001010`, which equals the decimal number `10`.
Effects of Left Shift on Positive and Negative Numbers
When performing a left shift on positive integers, the operation behaves as expected — each shift multiplies the number by 2.
However, left shifting negative numbers can lead to unexpected outcomes due to representation in two's complement form. For instance, look at this example:
// Positive Shift
int posNum = 2; // Binary: 00000010
int posResult = posNum << 2; // Result: 8 (Binary: 00001000)
// Negative Shift
int negNum = -2; // Binary: 11111110 (for a 2-complement system)
int negResult = negNum << 1; // Result: -4, Binary: 11111100
In this example, the left shift of `2` (binary `00000010`) by two positions yields `8`, while the left shift of `-2` keeps its sign but changes its magnitude.
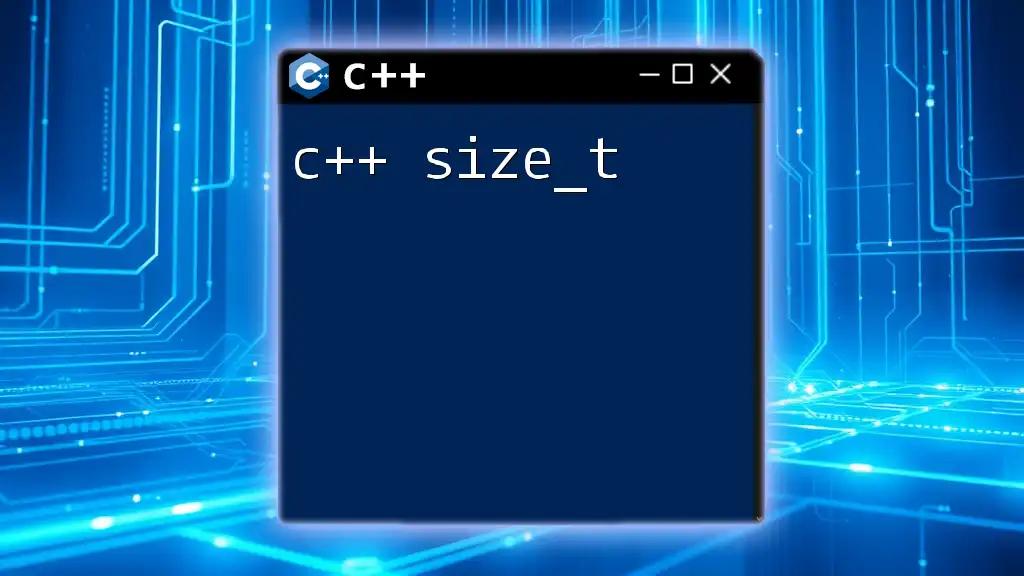
Practical Uses of Left Shift in C++
Multiplying by Powers of Two
One of the most common applications of the left shift operation is multiplying by powers of two. Each shift to the left effectively equates to multiplying the number by 2. For example:
int base = 3;
int multiplied = base << 4; // Equivalent to base * 16 (2^4)
In this case, shifting `3` four times to the left gives us `48`, since `3 * 16 = 48`. This simple yet effective technique is widely used in performance-sensitive applications where multiplication operations may slow down the execution.
Performance Optimizations
Using the left shift operator can yield performance benefits over more conventional multiplication methods. Multiplying a number by a constant value like `16` using traditional multiplication involves more processing than a simple left shift. For clarity, see the comparison below:
// Manual multiplication
int manualMult = base * 16;
// Using left shift
int shiftMult = base << 4; // More efficient
By using `shiftMult`, you may reduce computational overhead, making your code not just shorter but faster.
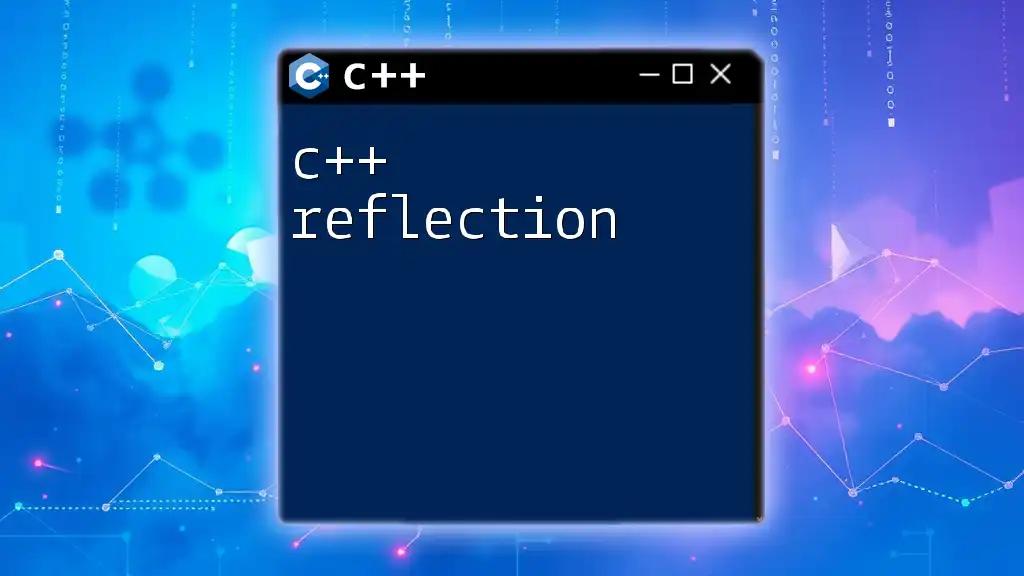
Common Mistakes and Pitfalls
Over-shifting and Under-shifting
A significant risk in performing left shifts is over-shifting. If you attempt to shift bits beyond the limits of the data type, it could result in undefined behavior or overflow. For example:
int largeNum = INT_MAX;
int overflowResult = largeNum << 1; // Undefined behavior
In this case, shifting `INT_MAX` left could potentially exceed the bounds of the `int` data type, leading to unexpected results.
Misunderstanding Sign Bits
When working with signed integers, it's crucial to understand that shifting bits may alter the representation and the result's sign. For instance, left-shifting a negative number might cause the sign bit to flip, leading to complications in how the value is interpreted.
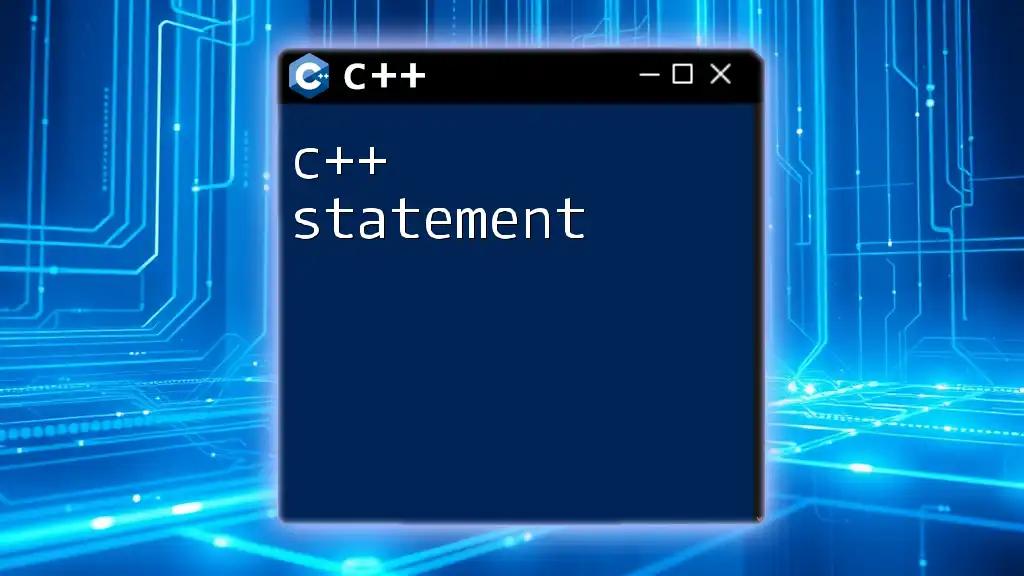
Conclusion
In summary, the c++ shift left operator is a robust tool, essential for manipulating binary data efficiently. Understanding its mechanics offers significant advantages in both performance and clarity when handling integer values. Through a mix of practice and exploration, embrace the intricate world of bit manipulation, and maximize your coding efficiency.
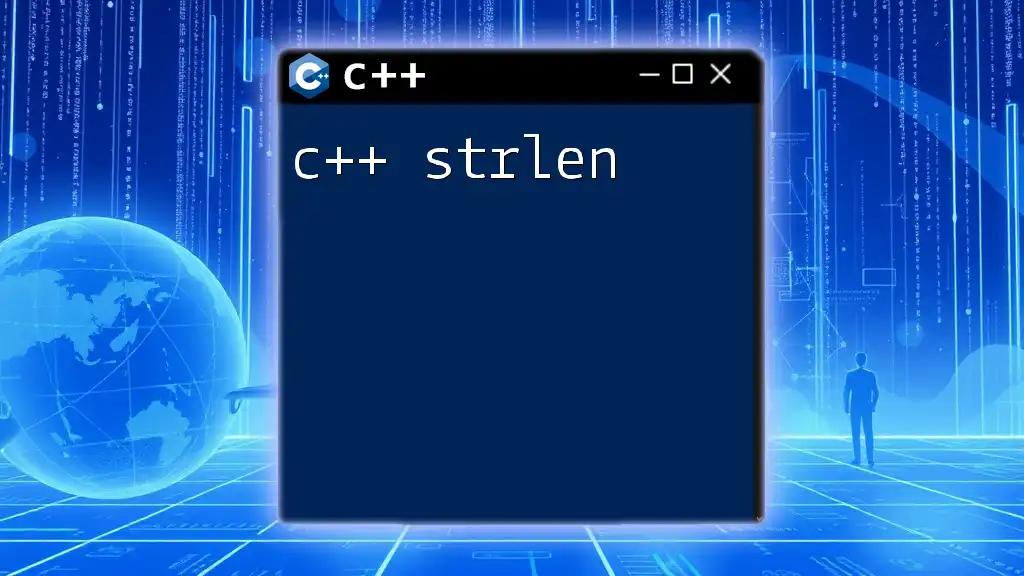
Additional Resources
For further reading on C++ and sharpening your understanding of bitwise operations, consider exploring online tutorials, coding exercises on platforms like LeetCode or HackerRank, and comprehensive C++ programming books that delve deeper into these topics.