In C++, the error message "expected an identifier" usually indicates that the compiler was expecting a name for a variable, function, or other identifier but found something else instead, often due to syntax issues.
#include <iostream>
int main() {
int 123number; // This will cause the "expected an identifier" error
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ Syntax
The Role of Identifiers
In C++, identifiers are essentially the names used to identify variables, functions, classes, and other user-defined items. As a programmer, it's imperative to understand how to properly name these identifiers to avoid confusion and errors in your code.
Rules for Naming Identifiers
- Starting Character: An identifier must begin with either a letter (A-Z, a-z) or an underscore (_).
- Allowed Characters: After the first character, an identifier can consist of letters, digits (0-9), and underscores.
- Case Sensitivity: Identifiers in C++ are case-sensitive, meaning `MyVariable` and `myvariable` would be considered two distinct identifiers.
Common Syntax Errors in C++
Errors related to identifiers often stem from misunderstandings of the fundamental C++ syntax. Being aware of general syntax errors can help you steer clear of more specific problems, including the dreaded "expected an identifier" error, which frequently occurs at compile time.
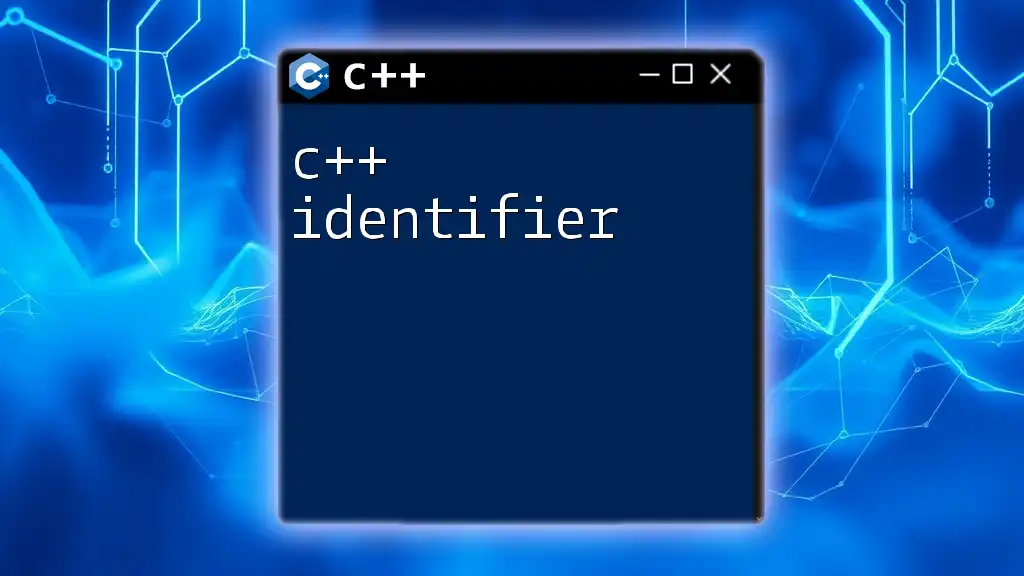
What Does "Expected an Identifier" Mean?
Analyzing the Error Message
When you encounter the error message stating "expected an identifier", it signifies that the C++ compiler is expecting a valid identifier at a particular point in your code but instead finds something else—be it a keyword, symbol, or incomplete syntax. Understanding why this error occurs is crucial for effective debugging.
Common Scenarios Leading to the Error
Unintended Keywords
One prevalent situation that results in the "expected an identifier" error is the use of reserved keywords as identifiers. C++ has a set of keywords that cannot be used as variable or function names because they have predefined meanings in the language.
Example:
int return = 5; // Error: expected an identifier
In the code above, `return` is a keyword used to exit a function and cannot be employed as a variable name.
Incorrect Syntax Structure
Sometimes the error arises from a faulty syntax structure, such as missing or misplacing symbols.
Example of a Method Declaration Leading to the Error:
void myFunction( // Error: expected an identifier
The compiler does not find a valid identifier because the function parameters are not correctly indicated.
Variable Declarations Gone Wrong
Another common mistake is forgetting to declare the data type when initializing a variable.
Example of Faulty Declaration:
myVar = 10; // Error: expected an identifier
Here, `myVar` is undefined prior to assignment, leading to confusion for the compiler.
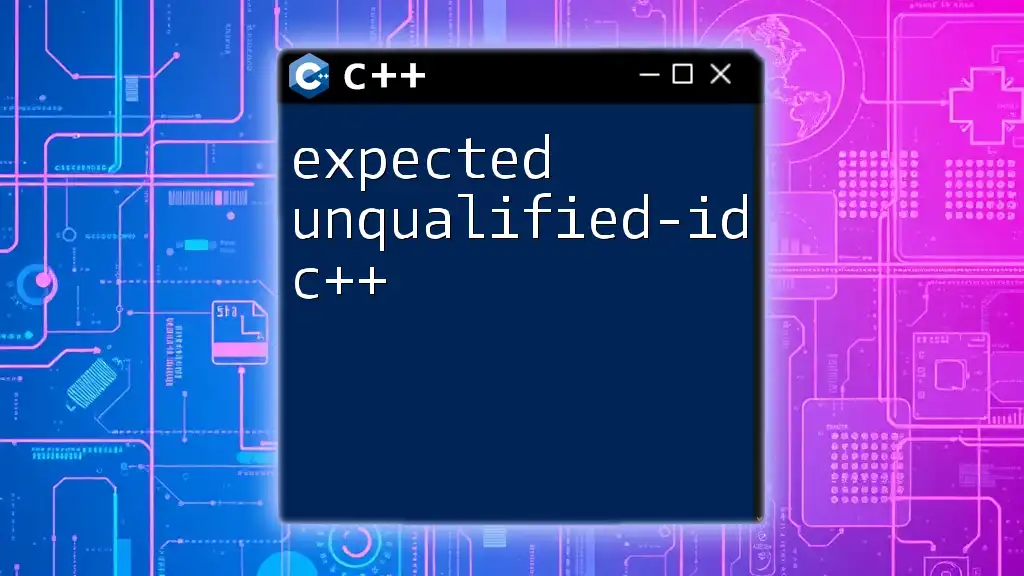
How to Troubleshoot the "Expected an Identifier" Error
Step-by-Step Debugging
If you encounter this error, consider the following tips for finding the source of the issue:
- Read the Error Message: The compiler provides a message along with the line number, which hints at where the problem might lie.
- Isolate the Line: Comment out adjacent lines of code to narrow it down to the exact line causing the problem.
- Inspect Surrounding Code: Often, the error in question may not be on the same line but could result from improperly closed braces or missing semicolons in previous lines.
Common Fixes for the Error
Revising Identifiers
If you find that a reserved keyword is in use, simply rename the identifier to something unique that follows C++ rules.
Example of Fixing an Identifier Issue:
int myReturnValue = 5; // Corrected the identifier
Correcting Syntax Issues
To resolve syntax errors, focus on ensuring that your function declarations and statements adhere to proper formatting standards.
Example of Correct Syntax:
void myFunction(); // Correctly declared function
Taking the time to ensure your syntax is correct can save you from confusing errors down the line.
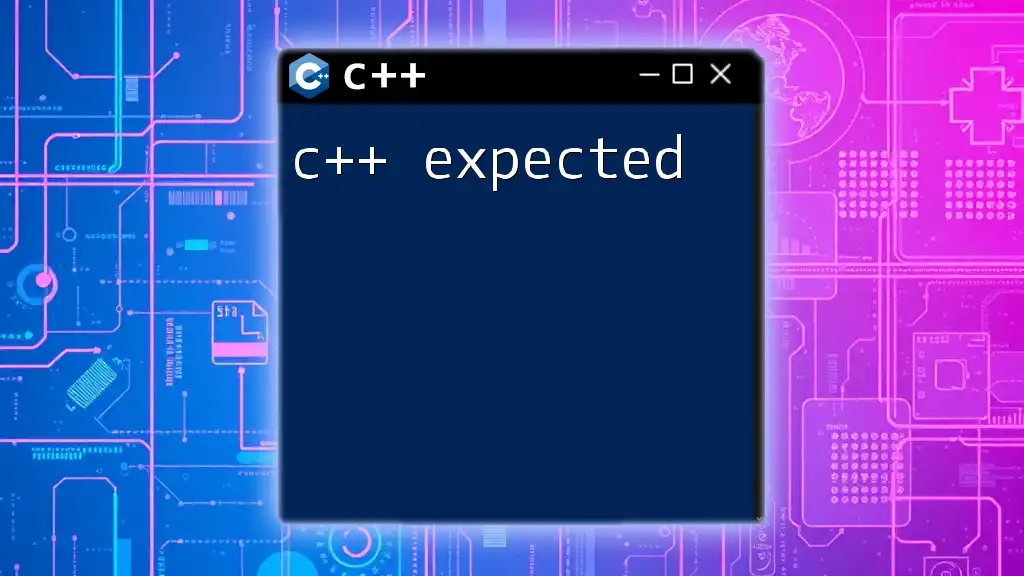
Preventing Future Errors
Best Practices in C++ Programming
- Consistent Naming Conventions: Follow standard naming conventions, such as using camelCase for variable names. This makes your code more readable and helps avoid naming conflicts.
- Use Comments for Clarity: Adding comments can also clarify your intent, making it easier to remember what certain identifiers represent when you revisit your code.
- Code Formatting: Maintain consistent indentation and spacing. Visually organized code is significantly easier to debug.
Leverage IDE Tools
Utilizing Integrated Development Environments (IDEs) can be incredibly beneficial for catching errors before you compile. Many modern IDEs offer features like:
- Syntax highlighting: Visual cues that differentiate keywords, variables, and comments.
- Code linting: This assists with spotting potential issues even before compiling your code.
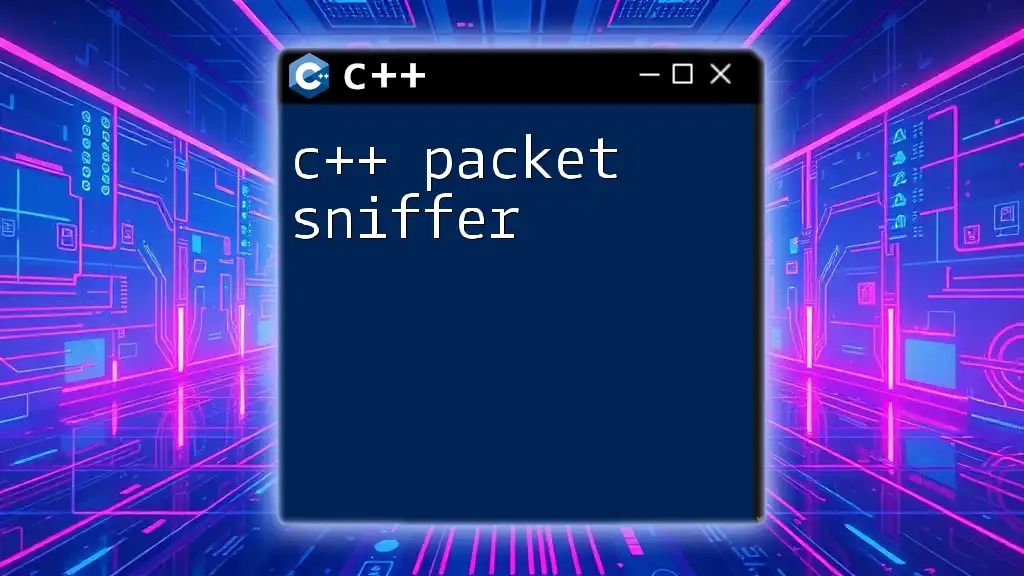
Conclusion
Understanding the "expected an identifier" error in C++ is crucial for beginner and experienced programmers alike. Recognizing the common causes and applying effective debugging techniques can significantly enhance your coding experience and efficiency. As you continue to practice, you will improve your skills in identifying and fixing these common syntax errors, leading to cleaner and more robust code.
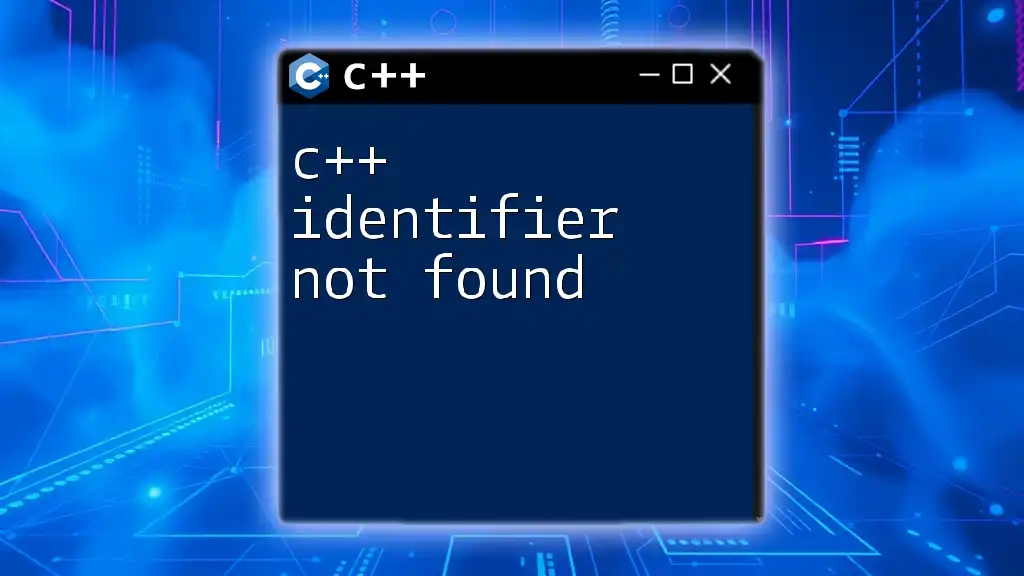
Additional Resources
For further reading and resources to strengthen your C++ skills, consider checking out different online platforms that provide coding challenges and create a supportive learning community. Continuous engagement allows for constant growth and improvement in your coding abilities.
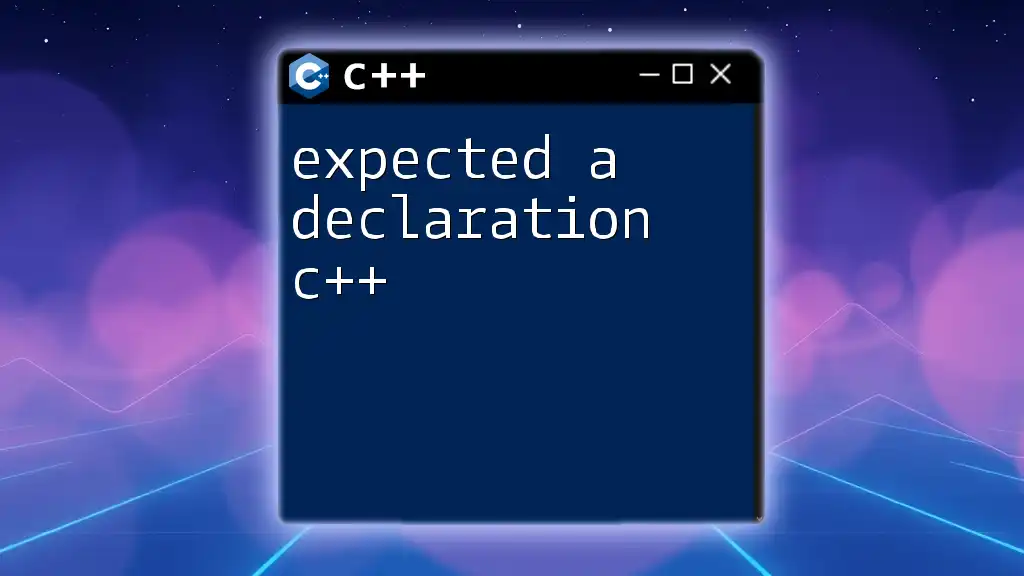
Call to Action
Feel free to share your experiences with the "expected an identifier" error or any other C++ issues you’ve encountered. Join our upcoming course to delve deeper into C++ commands and learn how to navigate errors quickly and efficiently!