To print quotation marks in C++, you can use the escape character `\` before the quotation marks to prevent them from being interpreted as string delimiters.
Here’s an example:
#include <iostream>
int main() {
std::cout << "He said, \"Hello, world!\"";
return 0;
}
Understanding Quotation Marks in C++
What Are Quotation Marks?
Quotation marks are critical components in programming languages, including C++. They are used to define strings and characters. In C++, single quotes (`'`) denote character literals, while double quotes (`"`) denote string literals. Understanding these differences is vital for mastering string manipulation in your C++ programs.
Importance of Quotation Marks in C++
Quotation marks play a crucial role in determining how your program interprets certain characters. When you enclose text in double quotes, C++ treats it as a string, allowing you to perform various operations on that text. Conversely, single quotes are reserved for single characters, such as letters or symbols, which can be utilized in character manipulation, comparisons, and other logical operations.
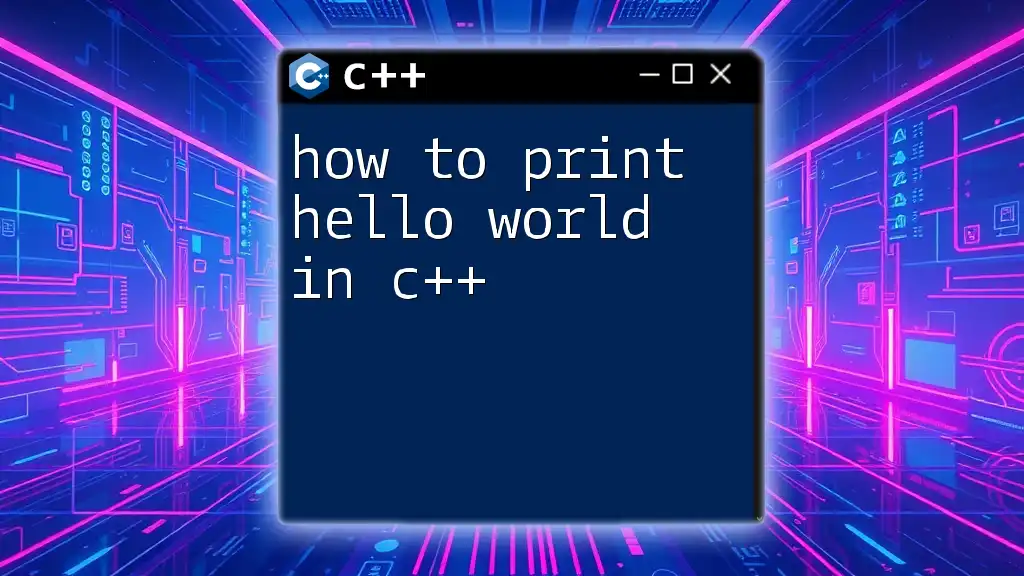
Escape Sequences in C++
What Are Escape Sequences?
Escape sequences are combinations of characters that allow you to perform tasks that would otherwise be impossible with regular characters. In C++, an escape sequence begins with a backslash (`\`). It enables you to include special characters in strings, including quotation marks, newline characters, tabs, and more.
Printing Special Characters with Escape Sequences
By understanding escape sequences, you can effectively manage special characters in your strings. This is especially useful when you want to include quotation marks within strings without terminating the string itself. This leads us to the next section: how to print double quotation marks.
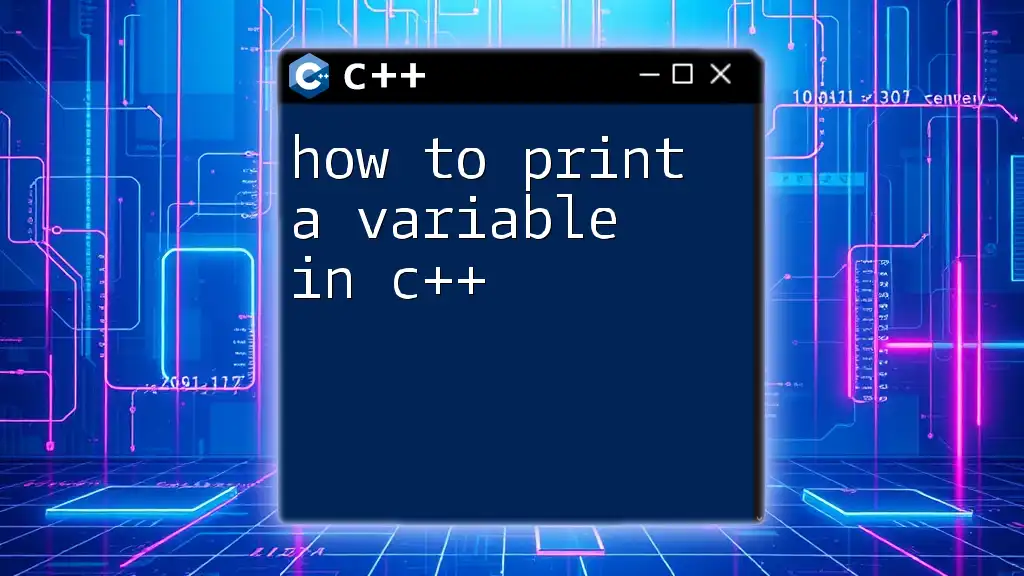
How to Print Double Quotation Marks
Using Escape Characters
To print double quotation marks inside a string, you can utilize the backslash as an escape character. This tells C++ that what follows should be treated differently, allowing you to include quotation marks without breaking the string.
Here’s an example:
#include <iostream>
int main() {
std::cout << "He said, \"Hello, World!\" to everyone." << std::endl;
return 0;
}
In this example, when run, the output will be:
He said, "Hello, World!" to everyone.
Explanation: The backslash (`\`) before the double quotation marks prevents C++ from interpreting them as the end of the string. Instead, they are printed as part of the output.
Using `std::string` to Handle Quotes
If you want to manage more complex strings or maintain clarity, consider using `std::string`. It allows for easier manipulation and storage of strings containing quotation marks.
Here's how you could achieve that:
#include <iostream>
#include <string>
int main() {
std::string quote = "She said, \"The quick brown fox jumps over the lazy dog.\"";
std::cout << quote << std::endl;
return 0;
}
Explanation: This code stores a complex quote in a `std::string` variable. By using the escape sequence, the quotation marks are correctly included in the output, making it easier to read and maintain.
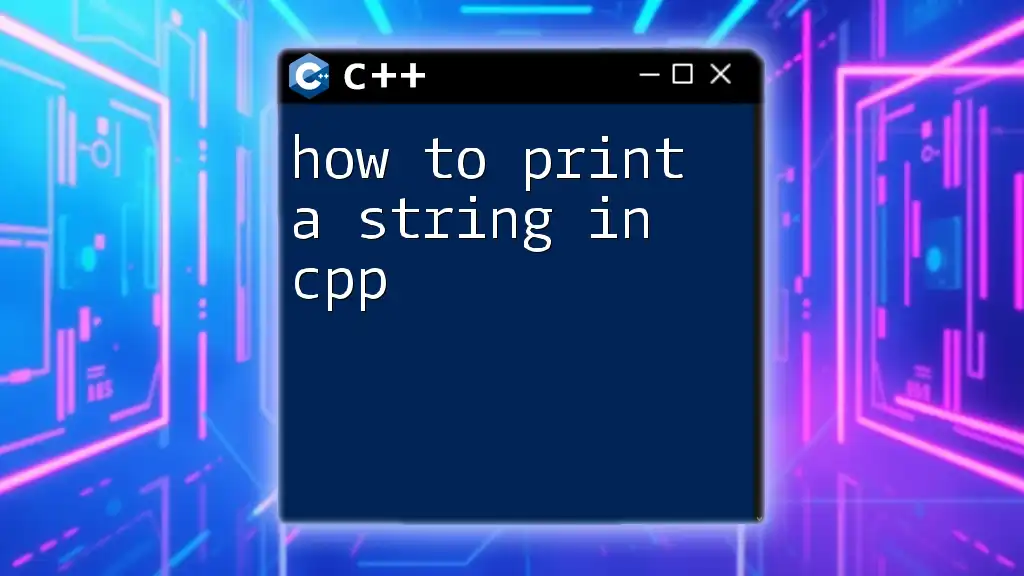
How to Print Single Quotation Marks
Direct Printing of Single Quotes
Interestingly, single quotation marks can be printed directly without needing escape characters in strings, as they're not treated as a string delimiter.
Here’s an example:
#include <iostream>
int main() {
std::cout << "It's a beautiful day!" << std::endl;
return 0;
}
In this case, you will see the output:
It's a beautiful day!
Discussion: The single quote (`'`) in this string does not interfere with the surrounding double quotes, showing how different types of quotation marks can coexist in C++ strings seamlessly.
Escape Sequence for Single Quotation Marks
While single quotes can often be printed directly, employing escape sequences can enhance clarity, particularly when the context grows confusing. Here’s how it can work:
#include <iostream>
int main() {
std::cout << "He\'s going to the store." << std::endl;
return 0;
}
Notes: Even though the single quote in this example could be directly placed in the string, the escape sequence can be particularly useful for maintaining consistency within your code or when constructing strings dynamically where contexts might fluctuate.
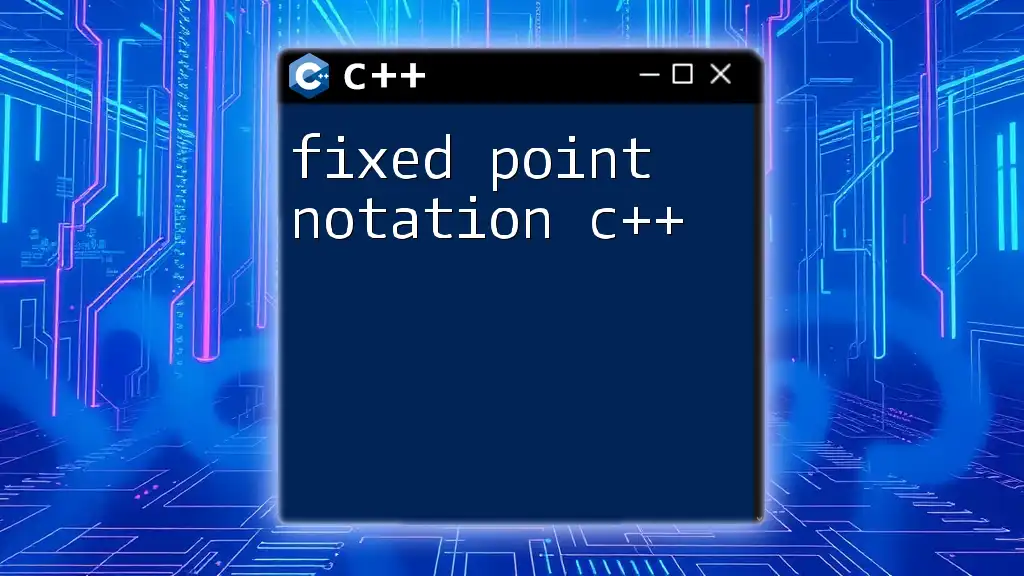
Best Practices for Using Quotation Marks in C++
General Tips
When working with quotation marks, it is essential to consider context. Use double quotes for strings that contain multiple characters and single quotes for single characters. This distinction helps maintain clarity and prevents logical errors in your code.
Readability and Maintainability
Focus on writing code that is not only functional but also easy to read and maintain. Favor using `std::string` for quotes that require multiple characters or nested quotation marks. Utilize escape sequences judiciously to avoid confusion and ensure that your intentions as a programmer are clearly conveyed.
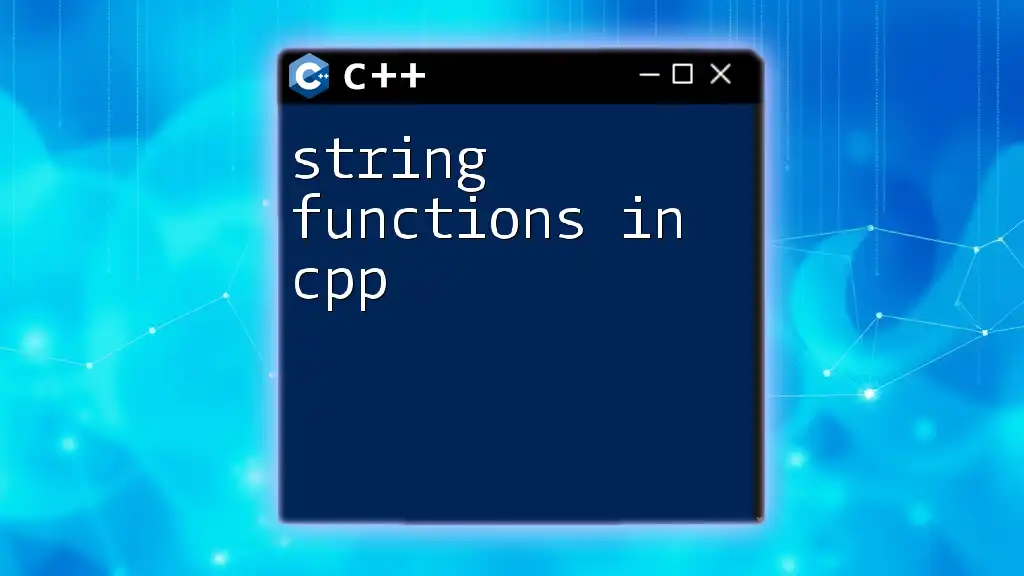
Conclusion
Recap of Key Points
In summary, understanding how to print quotation marks in C++ is vital for effectively working with strings and characters. By mastering escape sequences and the correct use of single and double quotes, you can write clearer and more functional code.
Encouragement to Practice
I encourage you to experiment with these concepts in your own code. Try crafting strings that incorporate both types of quotation marks and utilize escape sequences creatively. As you practice, you'll grow more comfortable with these fundamental but crucial aspects of C++ programming.
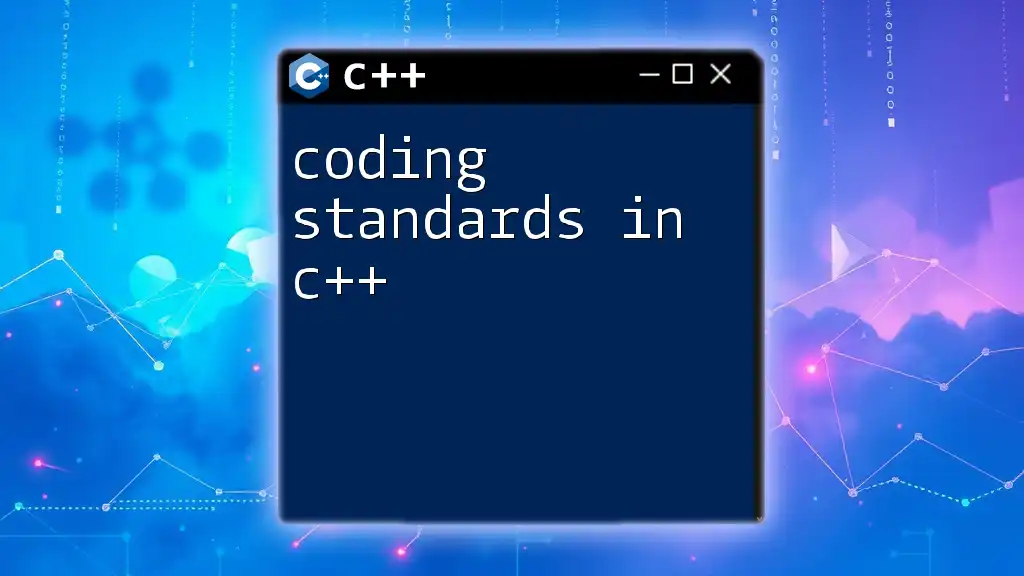
Additional Resources
Further Reading on C++
To reinforce your understanding, consider checking out official documentation and tutorials that delve deeper into C++ string handling, escape sequences, and related topics.
Community Engagement
Feel free to leave comments or questions about printing quotation marks or any other C++ subjects. Engaging with the community can greatly enhance your learning process and provide additional insights.