Fixed-point notation in C++ is a way of representing numbers that maintains a fixed number of digits after the decimal point, allowing for precise decimal calculations without the complications of floating-point arithmetic.
Here’s a simple example demonstrating fixed-point arithmetic using integers to represent cents:
#include <iostream>
int main() {
int priceCents = 1999; // price in cents, representing $19.99
int taxCents = 250; // tax in cents, representing $2.50
int totalCents = priceCents + taxCents;
std::cout << "Total price: $" << totalCents / 100 << "." << totalCents % 100 << std::endl;
return 0;
}
What is Fixed Point Notation?
Fixed point notation provides a way to represent real numbers with a fixed number of digits after the decimal point. Unlike floating point notation, which can vary in precision and range, fixed point representation offers a consistent method to handle decimal values. This is particularly important in systems where resources are limited, such as embedded systems, where performance and memory usage are critical.
Understanding fixed point notation is essential because it allows programmers and engineers to optimize calculations while ensuring determinism in the representation of decimal values.
Advantages and Disadvantages
Advantages:
- Ease of Implementation in Hardware: Fixed point arithmetic is often easier to implement in hardware compared to floating point arithmetic, resulting in faster processing speeds.
- Performance: Operations such as addition, subtraction, and multiplication typically execute faster because they require simpler circuitry.
- Predictable Precision and Range: The precision and range are determined by the fixed number of bits allocated to the integer and fractional parts, making it easier to manage in certain applications.
Disadvantages:
- Limited Range: Fixed point numbers cannot represent very large or very small numbers as efficiently as floating point numbers.
- Scaling Requirements: To avoid overflow or inaccuracies, careful scaling of numbers is essential, particularly in operations that may exceed the limits of the fixed range.
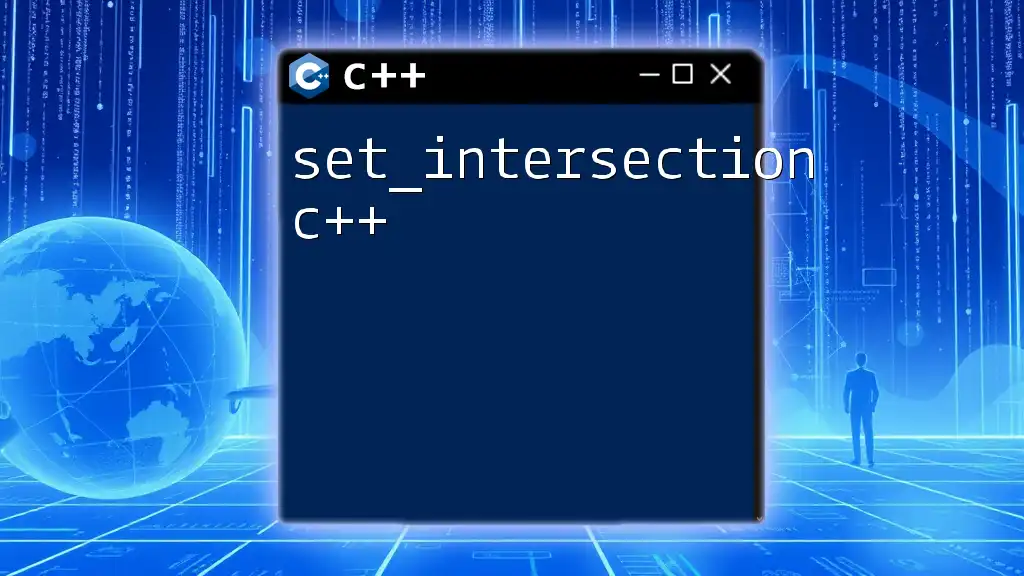
Understanding Fixed Point Representation
Fixed point numbers consist of two distinct parts: the integer part and the fractional part. This structure allows for an organized and predictable way to perform arithmetic operations.
Fixed Point Representation Formats
The Q format (denoted as Qm.n) is commonly used for fixed point notation, where m represents the number of bits used for the integer part, and n represents the number of bits used for the fractional part. For example, in a Q15.16 representation:
- The first 15 bits are allocated for the integer portion.
- The last 16 bits represent the fractional portion.
This format allows the representation of a wide range of numbers while maintaining a consistent precision.
Scaling and Precision
Scaling is a critical aspect of using fixed point notation. When performing arithmetic, the values must be scaled accordingly to manage precision and prevent overflow. For instance, if you have a binary number in a Qm.n format, multiplying it by a scaling factor before the operation and adjusting back after the operation ensures that the result remains valid within the fixed point bounds.
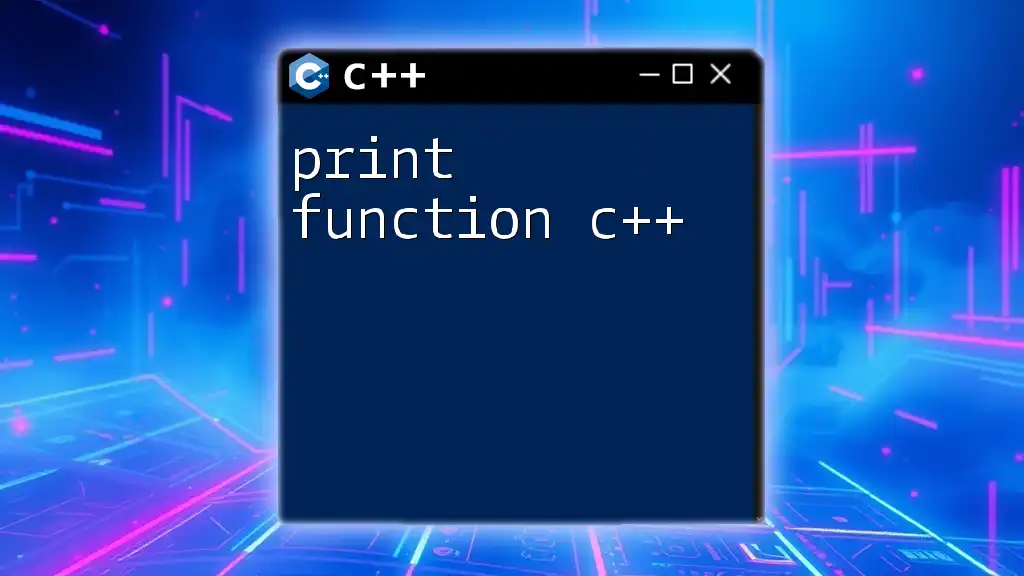
Implementing Fixed Point Arithmetic in C++
When working with fixed point numbers in C++, you can implement operations such as addition, subtraction, multiplication, and division. Let's go over each of these basic arithmetic operations.
Basic Arithmetic Operations
- Addition involves simply adding the integer values, but attention should be paid to overflow.
- Subtraction is similar and requires subtracting the integer values.
- Multiplication can be more complex since you must account for the scaling of both operands to maintain precision.
- Division involves careful shifting to maintain the correct format.
Code Snippets for Basic Operations
Addition Example
To illustrate the addition of two fixed point values, consider the following function:
int fixedPointAdd(int a, int b) {
return a + b; // Assume `a` and `b` are in Qm.n format.
}
Multiplication Example
The multiplication of two fixed point numbers requires a right shift to maintain the scale. Here's an example:
int fixedPointMultiply(int a, int b, int n) {
return (a * b) >> n; // Right shift by `n` after multiplication to adjust scale.
}
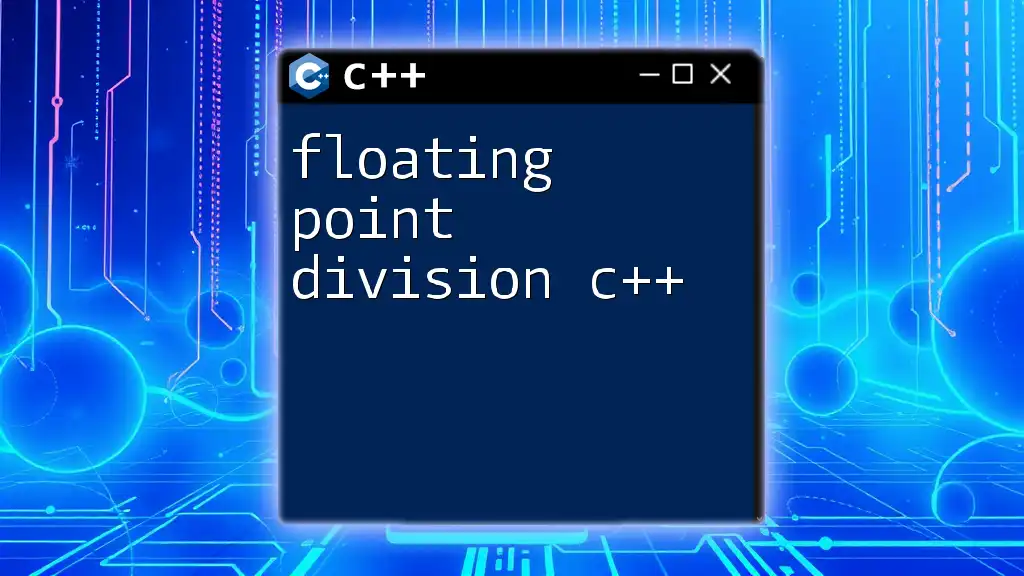
Handling Overflow and Underflow
Understanding how to handle overflow and underflow is crucial when performing arithmetic in fixed point notation. Overflow occurs when the result of an operation exceeds the maximum value that can be represented, while underflow happens when it goes below the minimum.
To prevent these issues:
- Clamping Values: Ensure that results do not exceed predefined limits.
- Using Larger Representations: If your application frequently encounters overflow, consider using a larger fixed point representation or a different format altogether.
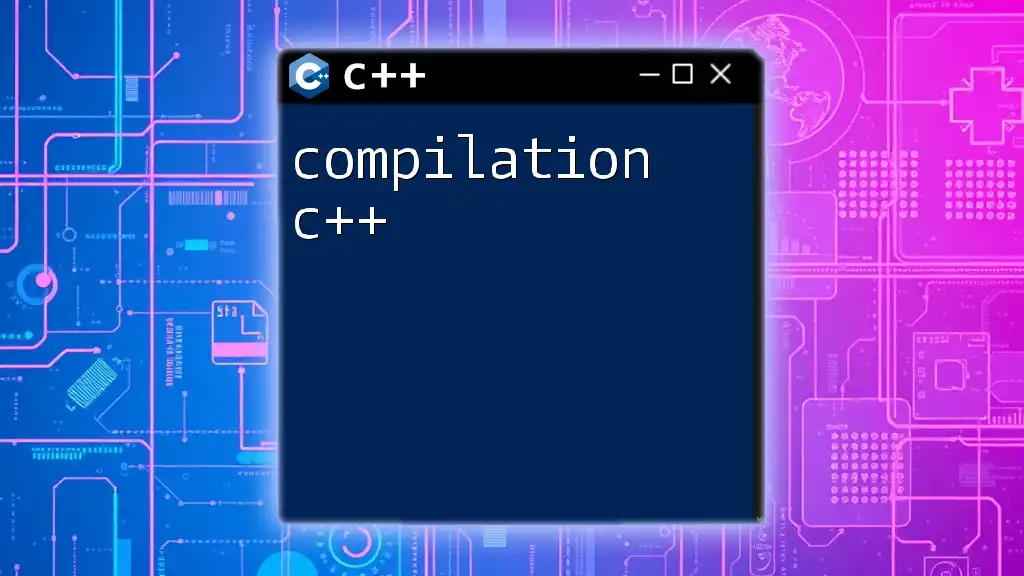
Using Fixed Point in C++
Creating a custom representation of fixed point numbers can enhance readability and manage complexity. A simple class can encapsulate this functionality.
Example Fixed Point Class
Here’s a basic implementation of a fixed point class:
class FixedPoint {
public:
FixedPoint(int value) : value(value << fraction_bits) {}
// Method for addition
FixedPoint operator+(const FixedPoint &other) {
return FixedPoint((value + other.value) >> fraction_bits);
}
private:
int value; // Internal representation
static const int fraction_bits = 16; // Number of fractional bits
};
In this example, the class uses a left shift to account for the fractional bits upon construction, enabling straightforward addition through operator overloading.
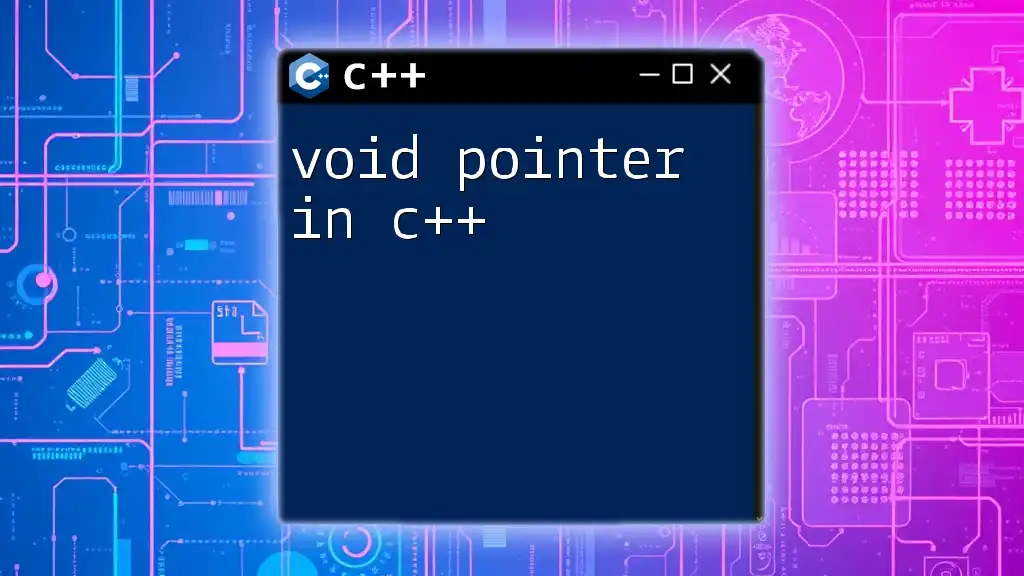
Real-World Applications of Fixed Point Notation
Fixed point notation finds its place in various real-world applications, particularly in sectors where computational resources are constrained.
Industry Examples
- Robotics Control Systems: In robotics, fixed point arithmetic can be leveraged to perform calculations related to position and movement reliably and predictably.
- Audio Processing: High-performance real-time audio processing can benefit from fixed point arithmetic by reducing computational demands while ensuring accurate sound reproduction.
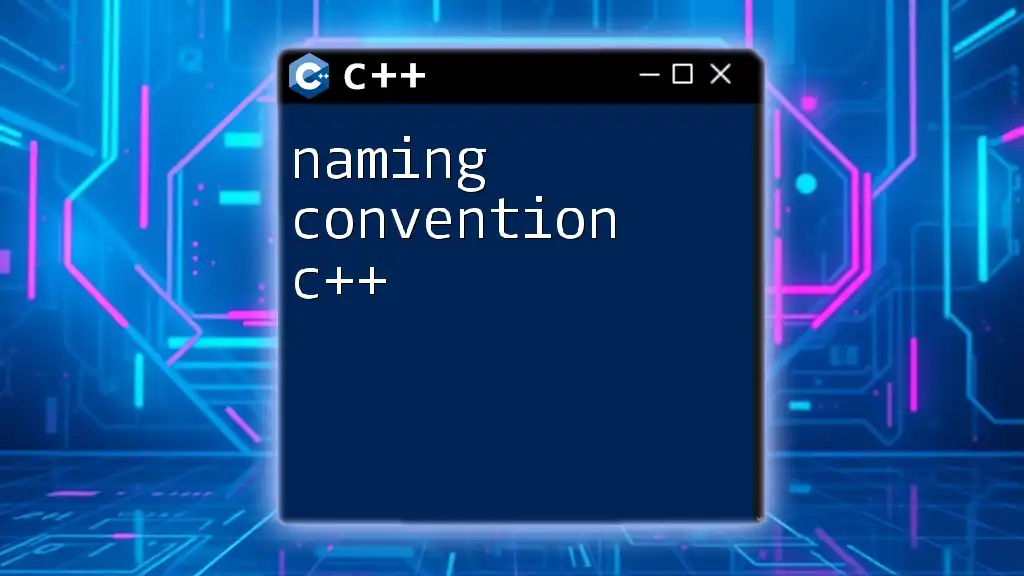
Best Practices for Using Fixed Point Notation
To effectively utilize fixed point notation in C++, consider the following best practices:
- Choose the Right Format: Select the format that best fits the range and precision requirements of your application.
- Constantly Monitor Precision: Be vigilant about potential precision issues, particularly during complex calculations.
- Test Rigorously: Implement and test your code against edge cases to ensure stability and performance.
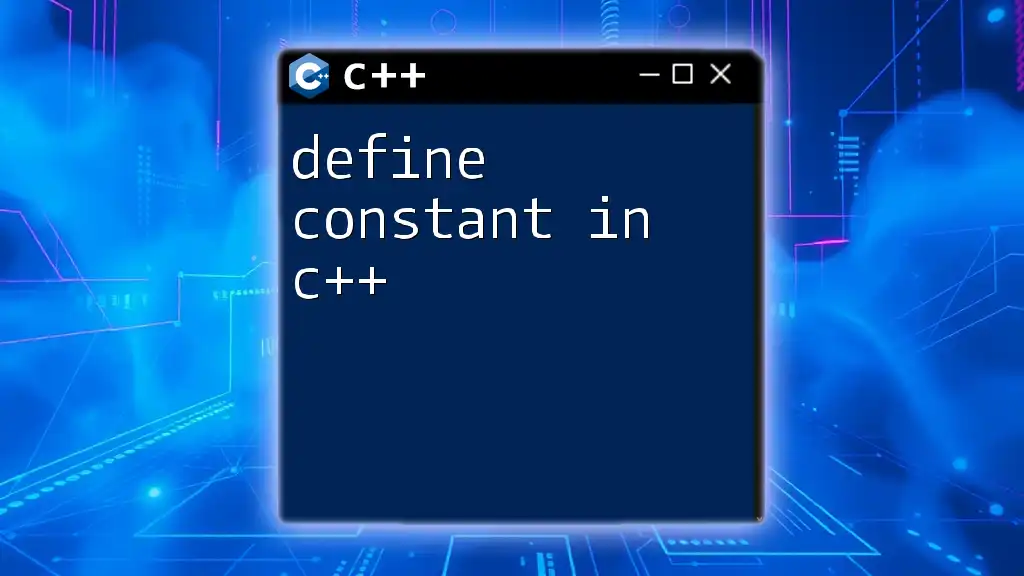
Conclusion
Mastering fixed point notation in C++ is vital for developing efficient applications, especially in systems where performance and resource management are paramount. Understanding how to represent, manipulate, and optimize fixed point arithmetic will empower you to write cleaner, faster, and more reliable code. To further enhance your skills, engage with various examples and projects that challenge your understanding and application of fixed point notation.
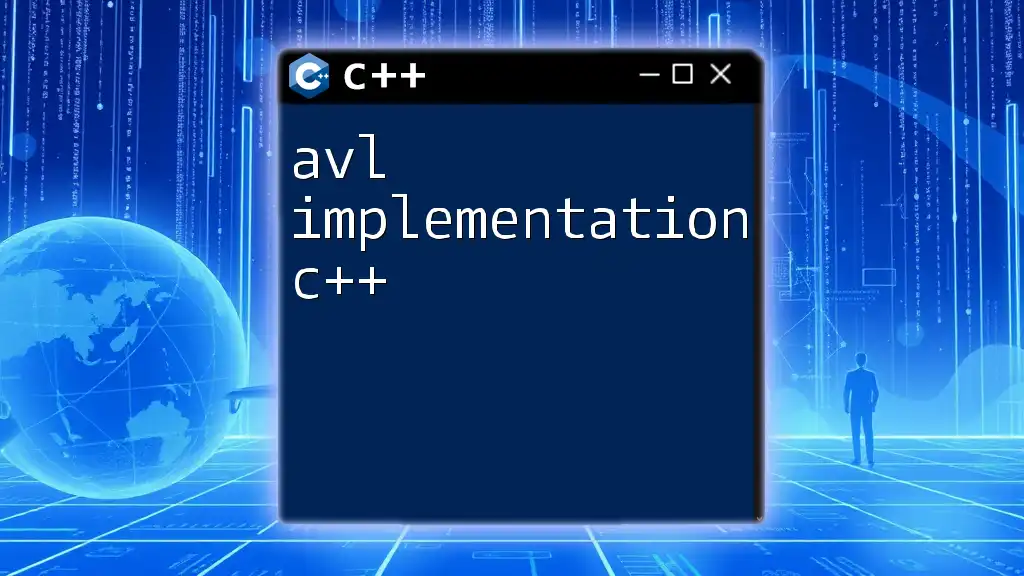
Additional Resources
For further reading materials and comprehensive tutorials, consider exploring online resources that dive deeper into the nuances of fixed point arithmetic and its application in C++. Engaging with community forums can provide additional insights and best practices as well.