An AVL tree is a self-balancing binary search tree where the heights of the two child subtrees of any node differ by at most one, ensuring O(log n) time complexity for search, insertion, and deletion. Here’s a basic implementation in C++:
#include <iostream>
using namespace std;
class Node {
public:
int key, height;
Node *left, *right;
Node(int val) : key(val), height(1), left(nullptr), right(nullptr) {}
};
int height(Node *N) {
if (N == nullptr) return 0;
return N->height;
}
int getBalance(Node *N) {
if (N == nullptr) return 0;
return height(N->left) - height(N->right);
}
// Additional functions for rotations and insertions would follow here...
int main() {
// Code to create and manipulate the AVL tree
}
What is an AVL Tree?
An AVL Tree, named after its inventors Georgy Adelson-Velsky and Evgenii Landis, is a type of self-balancing binary search tree. In this tree, the heights of the two child subtrees of any node differ by at most one, ensuring that operations like insertion, deletion, and lookup remain efficient.
Why Use AVL Trees?
The self-balancing property of AVL trees means that they automatically keep themselves balanced after any update, unlike traditional binary search trees (BSTs) where the performance can degrade to linear time in the case of unbalanced trees. AVL trees offer:
- Faster Lookups: By maintaining balance, AVL trees guarantee log(n) time complexity for lookup operations.
- Consistent Performance: All key operations are ensured to be fast, promoting efficiency in applications where lookups are frequent.
- Ideal Use Cases: AVL trees are often used in situations where read operations outweigh write operations, such as databases and memory management systems.
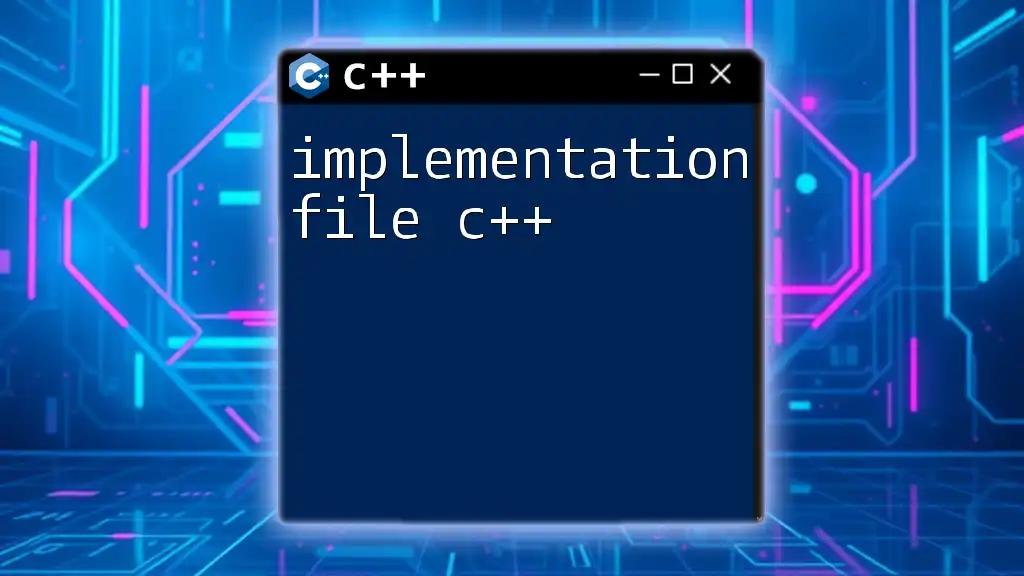
Understanding the Basics of AVL Trees
Before diving deeper into AVL implementation in C++, it's crucial to understand the fundamental concepts behind AVL trees.
Key Terminology
- Node: Each element in the tree that contains a key and pointers to its child nodes.
- Height: The height of a node is the number of edges on the longest path from that node to a leaf.
- Balance Factor: For any node, the difference between the heights of its left and right subtrees. It must be within the range of -1 to 1.
Structure of an AVL Tree
An AVL tree consists of nodes that point to their child nodes. Each node contains a value, pointers to the left and right children, and possibly its height for balance calculations. In C++, this can be represented as follows:
class TreeNode {
public:
int value;
TreeNode* left;
TreeNode* right;
int height;
TreeNode(int val) : value(val), left(nullptr), right(nullptr), height(1) {}
};
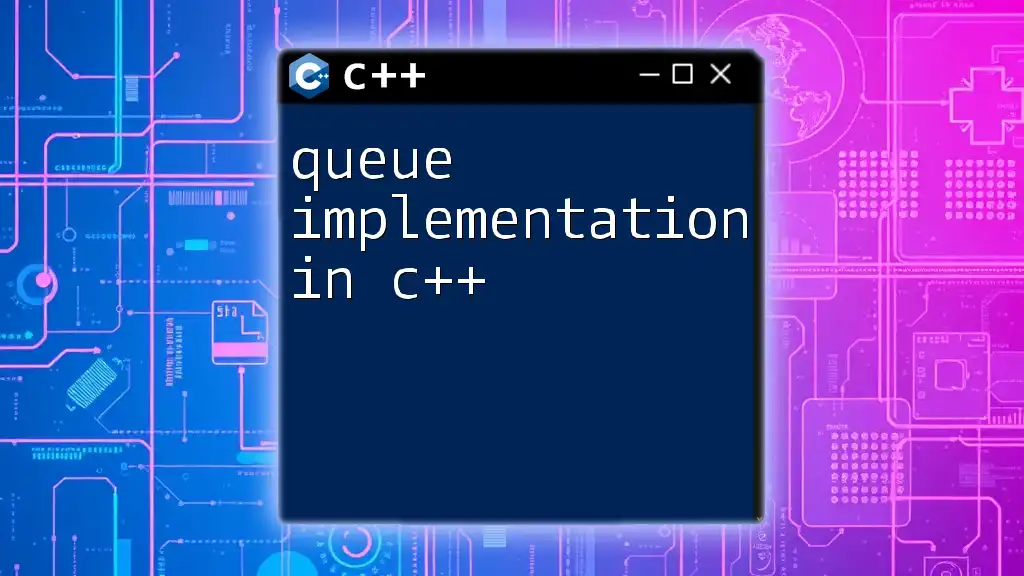
AVL Tree Operations
The core of any AVL implementation in C++ involves executing three key operations: insertion, deletion, and searching.
Insertion in AVL Tree
Inserting a new node into an AVL tree involves placing it in the correct location while ensuring the tree remains balanced. The insertion algorithm includes:
- Standard BST Insertion: Insert the node like in a binary search tree.
- Update Height: After insertion, update the height of the ancestor nodes.
- Check Balance: For each ancestor node, check its balance factor. If the factor is outside the range [-1, 1], rotate the tree to balance it.
Code Snippet: Insertion Logic
TreeNode* insert(TreeNode* node, int value) {
if (node == nullptr)
return new TreeNode(value);
if (value < node->value)
node->left = insert(node->left, value);
else if (value > node->value)
node->right = insert(node->right, value);
else
return node; // Duplicate values are not allowed
node->height = 1 + std::max(height(node->left), height(node->right));
int balance = getBalance(node);
// Left Left Case
if (balance > 1 && value < node->left->value)
return rightRotate(node);
// Right Right Case
if (balance < -1 && value > node->right->value)
return leftRotate(node);
// Left Right Case
if (balance > 1 && value > node->left->value) {
node->left = leftRotate(node->left);
return rightRotate(node);
}
// Right Left Case
if (balance < -1 && value < node->right->value) {
node->right = rightRotate(node->right);
return leftRotate(node);
}
return node;
}
Deletion in AVL Tree
Removing a node from an AVL tree is similar to the deletion operation in a binary search tree but requires additional steps to balance the tree afterward.
- Standard BST Deletion: Remove the node as in a binary search tree.
- Update Height: Update heights of ancestor nodes.
- Check Balance: Similar to the insertion process, check and balance the tree if necessary.
Code Snippet: Deletion Logic
TreeNode* remove(TreeNode* node, int value) {
if (node == nullptr)
return node;
if (value < node->value)
node->left = remove(node->left, value);
else if (value > node->value)
node->right = remove(node->right, value);
else {
// Node with only one child or no child
if ((node->left == nullptr) || (node->right == nullptr)) {
TreeNode* temp = node->left ? node->left : node->right;
if (temp == nullptr) {
temp = node;
node = nullptr;
} else
*node = *temp; // Copy the contents of the non-empty child
delete temp;
} else {
// Node with two children: Get the inorder successor (smallest in the right subtree)
TreeNode* temp = minValueNode(node->right);
node->value = temp->value;
node->right = remove(node->right, temp->value);
}
}
if (node == nullptr)
return node;
node->height = 1 + std::max(height(node->left), height(node->right));
int balance = getBalance(node);
// Balance the tree
// Similar balancing logic to insertion
...
return node;
}
Searching in AVL Tree
Searching in an AVL tree is efficient due to its self-balancing nature. The search logic follows standard binary search tree principles:
- If the target value is less than the current node's value, traverse left.
- If it's greater, traverse right.
- If it matches, return the node.
Code Snippet: Searching Logic
TreeNode* find(TreeNode* node, int value) {
if (node == nullptr || node->value == value)
return node;
if (value < node->value)
return find(node->left, value);
return find(node->right, value);
}
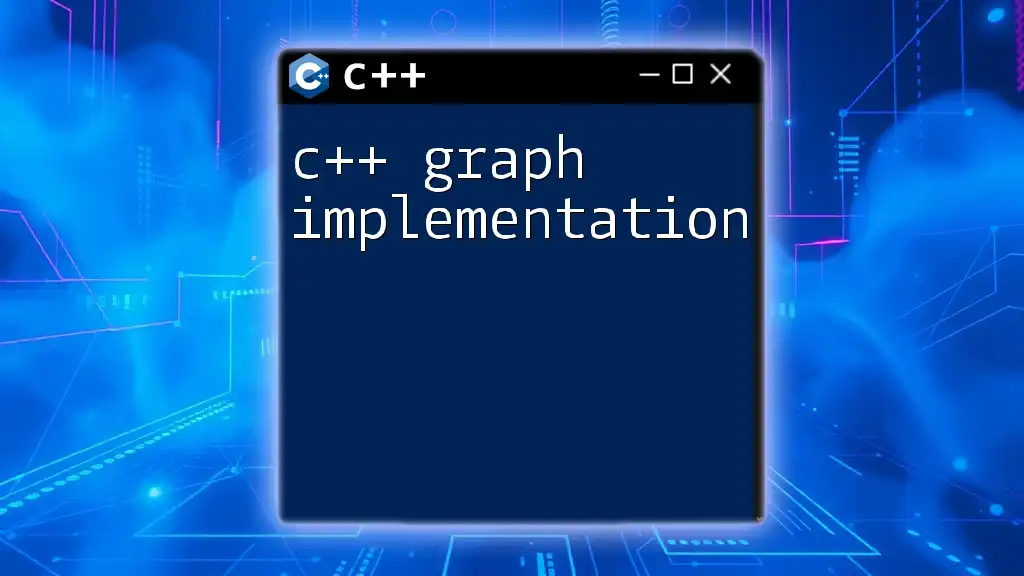
Balancing the AVL Tree
One of the core features of an AVL tree is its balancing mechanism. This involves rotations to maintain the balance factor of each node.
Understanding Rotations in AVL Trees
There are four types of rotations that are used to rebalance an AVL tree:
- Left Rotation: Used when a right-heavy tree needs to be balanced.
- Right Rotation: Applies to left-heavy scenarios.
- Left-Right Rotation: Combination of left then right rotation is necessary.
- Right-Left Rotation: A combination for cases requiring a balance from the right subtree.
Balance Factor Calculation
The balance factor for a node is computed as the difference between the heights of the left and right subtrees:
int getBalance(TreeNode* node) {
if (node == nullptr)
return 0;
return height(node->left) - height(node->right);
}
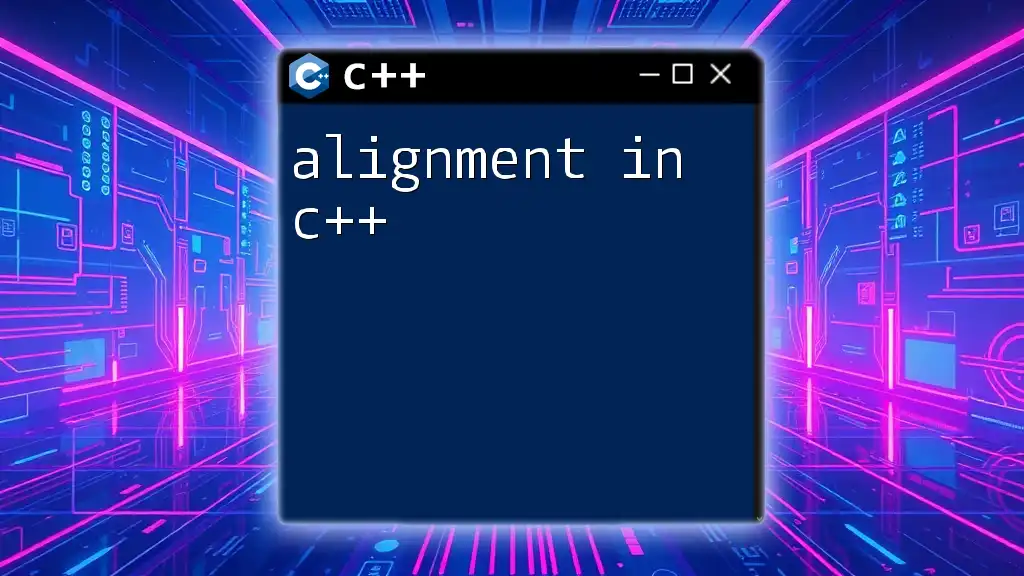
Full AVL Tree Implementation in C++
The complete implementation of an AVL tree in C++ encapsulates all the operations discussed. Below is an example of a simplified AVL tree class.
Code Snippet: Complete AVL Tree Class
class AVLTree {
private:
TreeNode* root;
// Private methods for insert, remove, and rotations
public:
AVLTree() : root(nullptr) {}
void insert(int value) {
root = insert(root, value);
}
void remove(int value) {
root = remove(root, value);
}
TreeNode* find(int value) {
return find(root, value);
}
// Additional utility methods for minValueNode, rotation operations, etc.
};
Performance Analysis
The AVL tree ensures that all key operations run in O(log n) time due to its self-balancing nature:
- Insertion: O(log n)
- Deletion: O(log n)
- Searching: O(log n)
In terms of space complexity, AVL trees use O(n) space for storing n nodes and maintaining pointers.
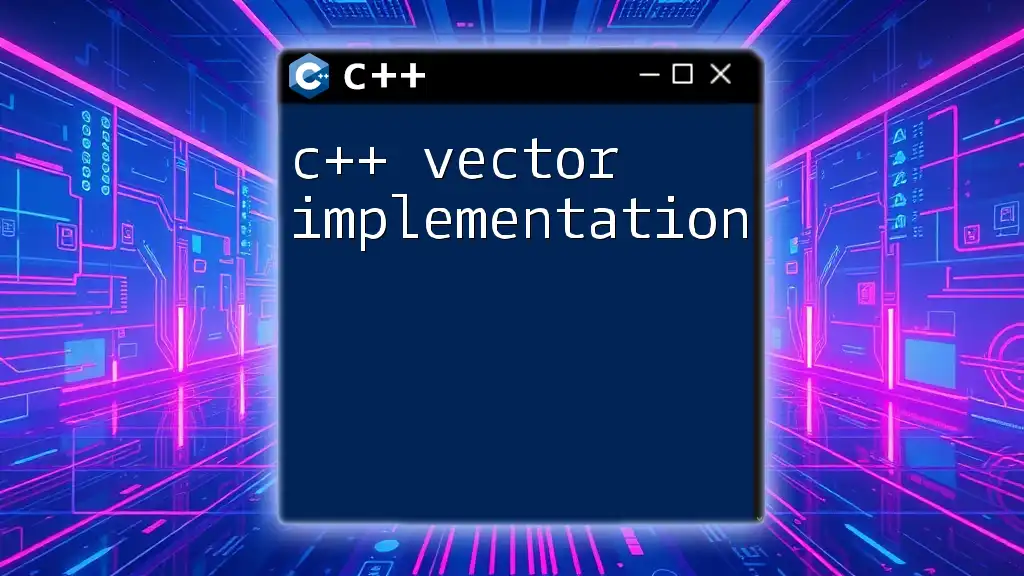
Common Use Cases for AVL Trees
AVL trees find their way into various applications in computer science, such as:
- Database Indexing: Where fast query performance is crucial.
- Memory Management Systems: To manage blocks of memory efficiently.
- Scheduling Algorithms: In operating systems for maintaining ordered events.
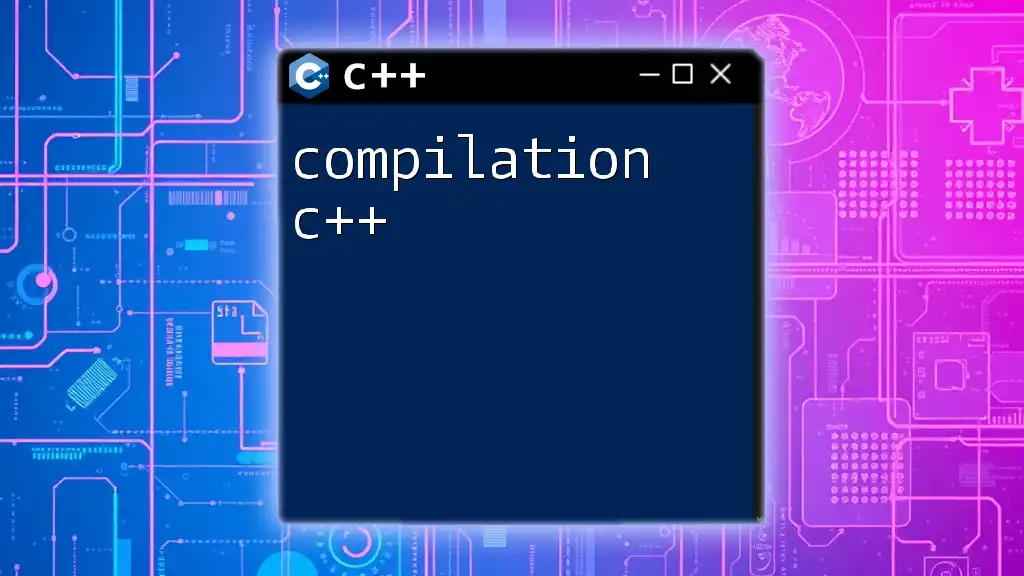
Conclusion
Understanding AVL trees and their implementation in C++ is a vital skill for developers, particularly those dealing with data structures and algorithms. The self-balancing property ensures that operations remain efficient, making AVL trees a preferred choice in many applications.
Further Learning Resources
For those looking to deepen their understanding, consider exploring:
- Books: "Introduction to Algorithms" by Cormen et al.
- Online Courses: Platforms like Coursera or Udacity offer specialized courses on data structures and algorithms.
- Practice: Engaging in coding challenges and implementing AVL trees in various scenarios can solidify your understanding.
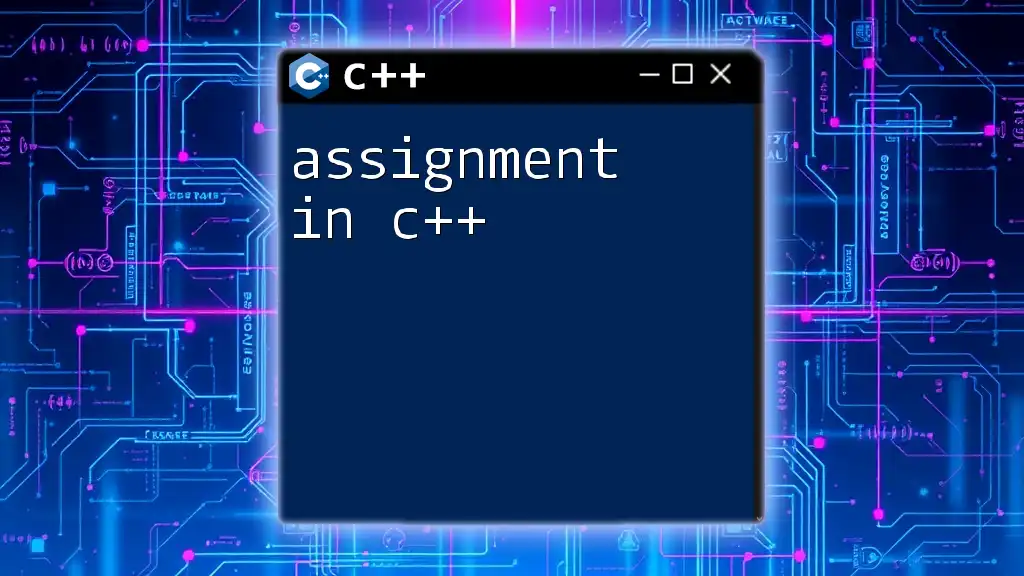
Call to Action
We invite readers to share their experiences with AVL trees! Have you implemented one in your projects? What challenges did you face? Comment below with your thoughts or questions. Let’s continue learning and exploring advanced C++ programming together!