C++ POSIX threads, commonly known as pthreads, provide a standardized interface for creating and managing threads in C++ applications to enable parallel programming.
Here’s a simple example of using POSIX threads to create and run a thread in C++:
#include <iostream>
#include <pthread.h>
void* threadFunction(void* arg) {
std::cout << "Hello from the thread!" << std::endl;
return nullptr;
}
int main() {
pthread_t thread;
pthread_create(&thread, nullptr, threadFunction, nullptr);
pthread_join(thread, nullptr);
return 0;
}
What are POSIX Threads?
POSIX threads, often referred to as pthreads, are a standardized C library that allows the creation and management of threads in C and C++. They provide a powerful means of implementing parallelism and concurrency in applications by enabling multiple threads to run concurrently within the same process. This becomes essential in modern programming, where performance and responsiveness are critical.
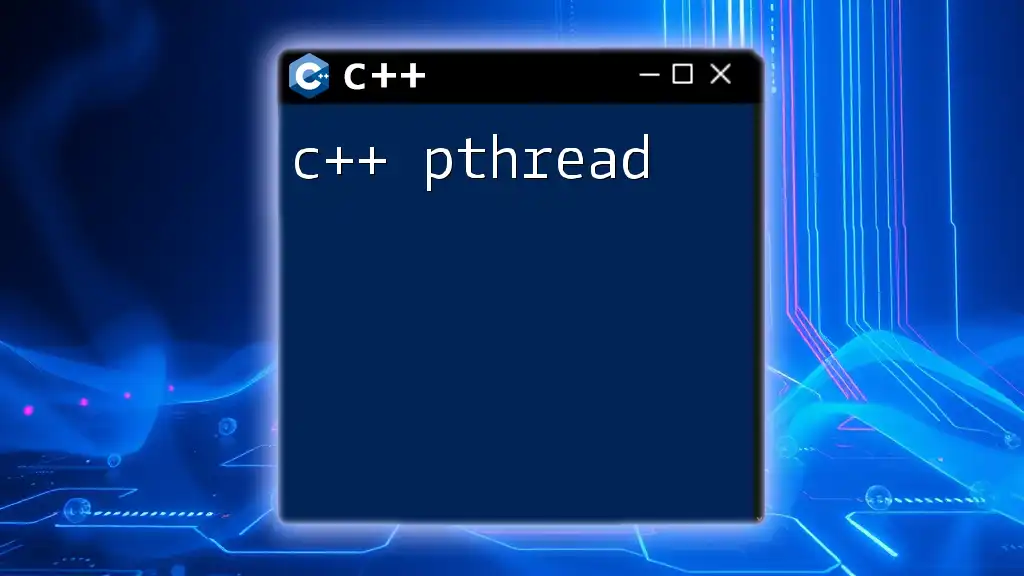
Benefits of Using POSIX Threads in C++
Utilizing C++ POSIX threads offers several notable advantages:
- Improved Performance: By allowing concurrent execution of routines, programs can utilize multiple CPU cores more effectively, resulting in increased performance.
- Better Resource Management: Threads share the same memory space, allowing for more efficient use of resources compared to multiple processes, which require separate memory allocation.
- Cross-Platform Compatibility: POSIX threads are supported on numerous operating systems, making applications more portable.
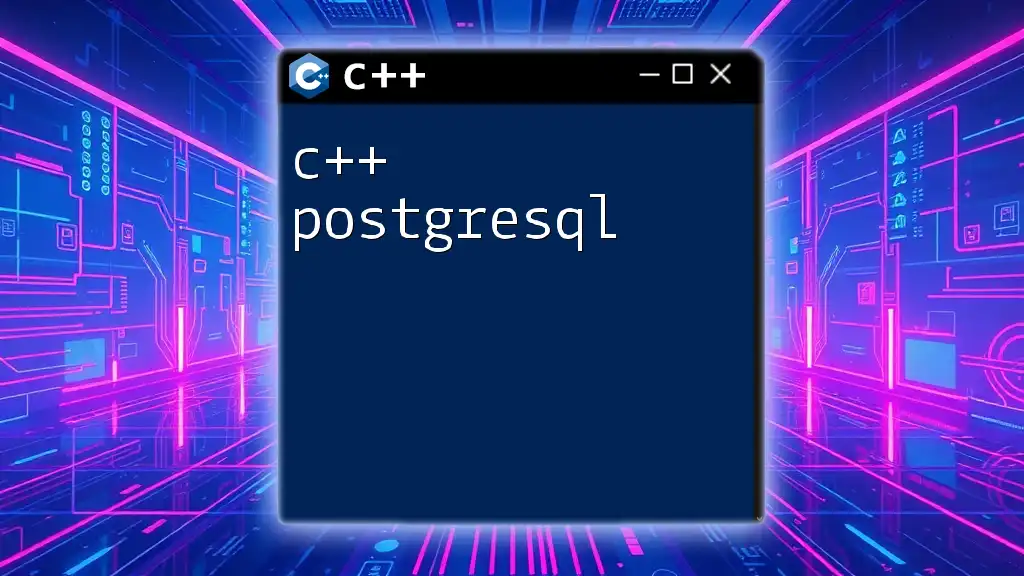
Setting Up Your C++ Environment for POSIX Threads
Tools and Libraries Required
To start using POSIX threads in C++, you’ll need the following:
- GCC (GNU Compiler Collection): Ensure you have GCC installed, which includes support for POSIX threads. This can be done via package managers in Linux or downloaded from the internet for other operating systems.
- Linking POSIX Libraries: When compiling your C++ code that uses pthreads, make sure to link the pthread library. This is done using the `-pthread` flag in your compile command:
g++ -pthread -o my_program my_program.cpp
Creating Your First POSIX Threads Program
To illustrate how to create a basic POSIX thread, consider the following C++ program:
#include <pthread.h>
#include <iostream>
void* helloThread(void* arg) {
std::cout << "Hello from thread!" << std::endl;
return nullptr;
}
int main() {
pthread_t thread;
pthread_create(&thread, nullptr, helloThread, nullptr);
pthread_join(thread, nullptr);
return 0;
}
In this example:
- We first include the necessary header `<pthread.h>`.
- The function `helloThread` prints a simple message and serves as our thread's function.
- The `pthread_create` function is called to create a thread, passing the thread ID and the function to execute.
- `pthread_join` is used to wait for the thread to finish executing before the main program exits, ensuring proper cleanup.
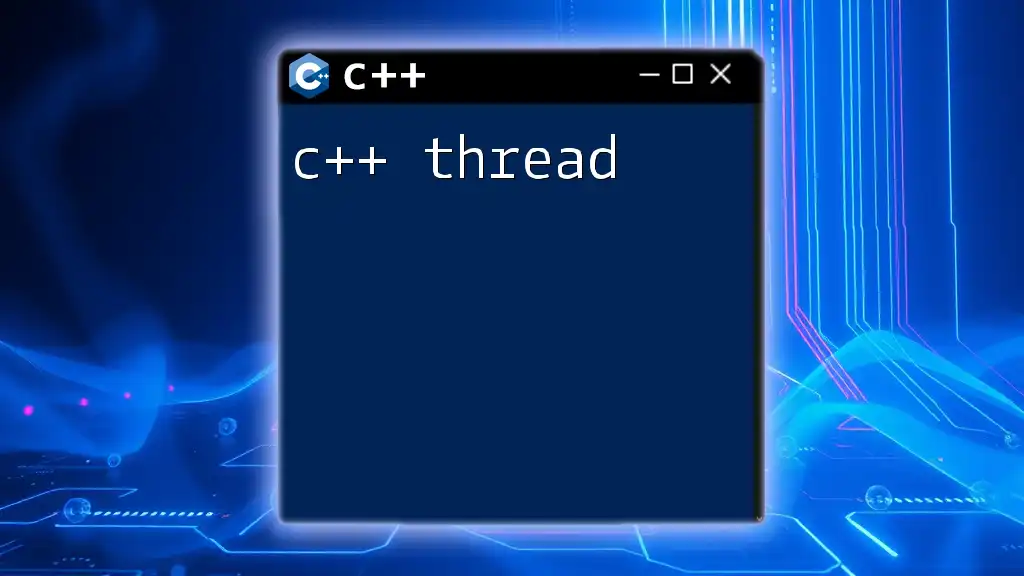
Understanding Thread Management
Creating Threads
There are several ways to create threads using POSIX. Here’s an example of creating multiple threads:
void* threadFunction(void* id) {
std::cout << "Thread ID: " << *(int*)id << std::endl;
return nullptr;
}
int main() {
pthread_t threads[5];
int threadIDs[5];
for (int i = 0; i < 5; ++i) {
threadIDs[i] = i;
pthread_create(&threads[i], nullptr, threadFunction, (void*)&threadIDs[i]);
}
for (int i = 0; i < 5; ++i) {
pthread_join(threads[i], nullptr);
}
return 0;
}
In this program:
- We define a `threadFunction` to print its thread ID.
- A loop creates five threads, each receiving a unique ID.
- We then join each thread in a second loop to ensure the main thread waits for their completion.
Destroying Threads
When working with threads, it’s important to manage their lifecycle properly. This involves releasing resources after they are no longer needed. You can explicitly terminate a thread by using `pthread_exit()` if a thread needs to end early. However, the proper joining of threads using `pthread_join()` is the recommended approach, as it ensures that resources are cleaned up appropriately.
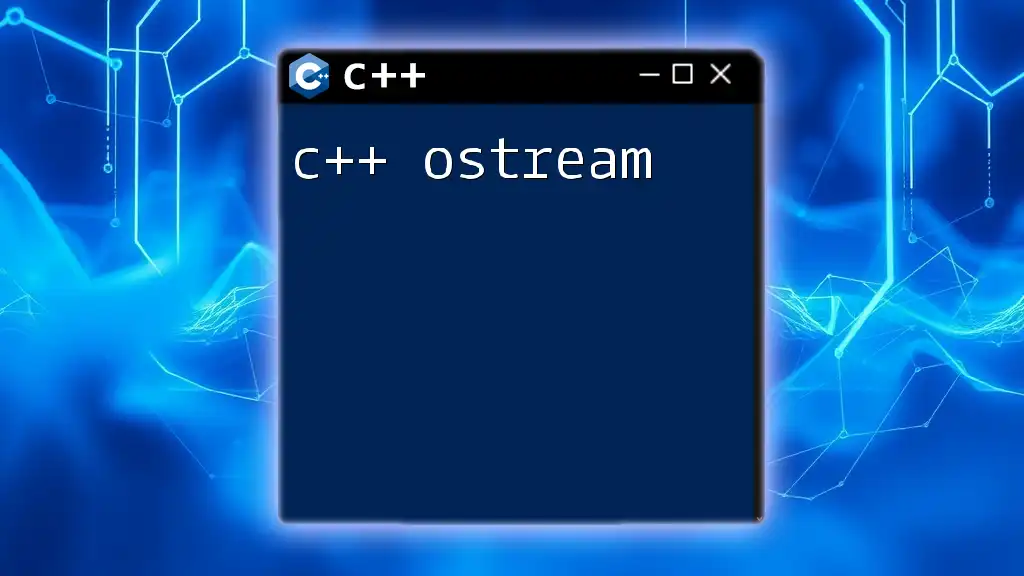
Synchronization Mechanisms
Why Synchronization is Necessary
In multithreaded applications, synchronization is crucial to prevent race conditions, where two or more threads attempt to modify shared resources simultaneously. Such situations can lead to unpredictable behavior and bugs that are difficult to trace.
Mutexes
A mutex (short for mutual exclusion) is a common synchronization mechanism to protect shared data. Here’s a simple example:
#include <pthread.h>
#include <iostream>
pthread_mutex_t lock;
void* increment(void* count) {
pthread_mutex_lock(&lock);
// Logic to increment the shared resource
pthread_mutex_unlock(&lock);
return nullptr;
}
int main() {
pthread_mutex_init(&lock, nullptr);
// Create threads that call increment
pthread_mutex_destroy(&lock);
return 0;
}
In this code:
- We declare a mutex variable `lock`.
- Before accessing a shared resource in `increment`, we lock the mutex using `pthread_mutex_lock()`.
- After the critical section is executed, the mutex is unlocked to allow other threads to access it.
Condition Variables
Condition variables facilitate communication between threads, enabling them to wait for certain conditions to occur. Here’s an example illustrating their use:
pthread_cond_t cond;
pthread_mutex_t mutex;
void* threadFunc(void* arg) {
pthread_mutex_lock(&mutex);
// Wait for a condition
pthread_cond_wait(&cond, &mutex);
pthread_mutex_unlock(&mutex);
return nullptr;
}
In this example, threads will wait on the condition variable until it is signaled. This is particularly useful for applications that require threads to coordinate their actions based on variable states.
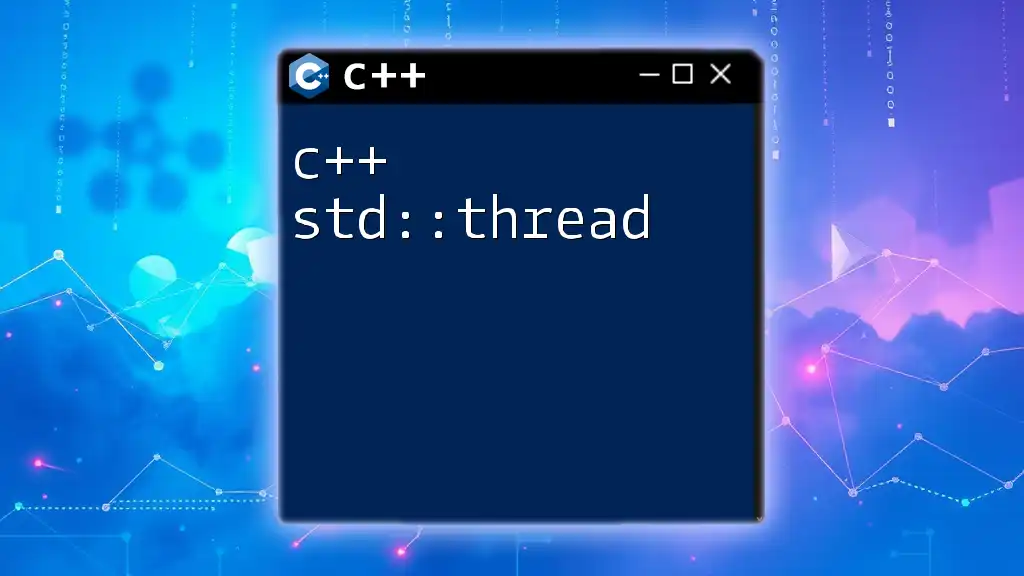
Thread Attributes and Management
Customizing Thread Attributes
The attributes of threads can be customized using the `pthread_attr_t` type. For example, to set a custom stack size:
pthread_attr_t attr;
pthread_attr_init(&attr);
pthread_attr_setstacksize(&attr, 1024 * 1024);
pthread_create(&thread, &attr, helloThread, nullptr);
pthread_attr_destroy(&attr);
This code initializes thread attributes, sets a custom stack size (1 MB), and creates a thread with those attributes. It’s important to remember to destroy the attributes afterward to free up resources.
Setting Thread Schedules
POSIX threads allow you to specify scheduling policies (such as FIFO and Round Robin) to control how threads are scheduled for execution. This plays a crucial role in applications where thread priorities can affect overall responsiveness.
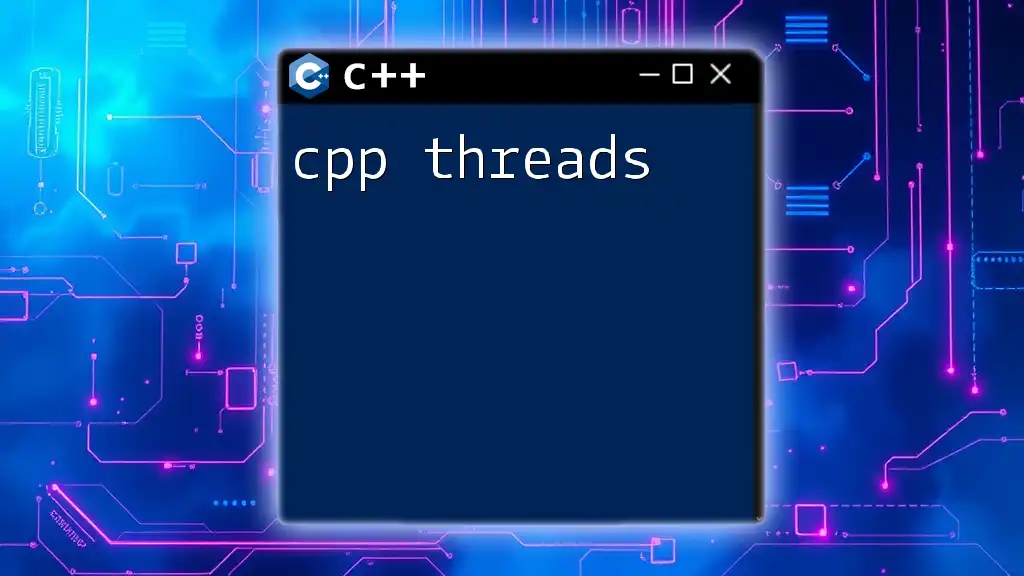
Error Handling in POSIX Threads
Common Errors and Their Solutions
Working with C++ POSIX threads can sometimes lead to errors, such as failing to create a thread or unsuccessful mutex locking. It is vital to check and handle these errors accordingly.
Using Return Codes for Error Management
Most POSIX thread functions return an integer error code. It’s best practice to check these return values to ensure operations like thread creation, joining, and mutex operations succeed. For example:
int result = pthread_create(&thread, nullptr, threadFunction, nullptr);
if (result != 0) {
std::cerr << "Error creating thread: " << strerror(result) << std::endl;
}
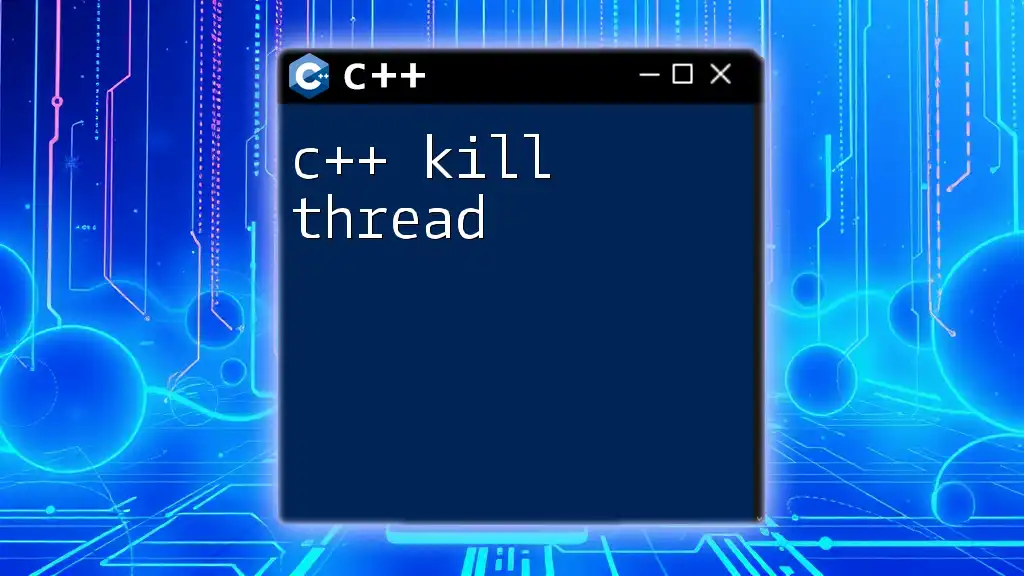
Best Practices for Using POSIX Threads in C++
Writing Efficient Threaded Code
To maximize performance when using POSIX threads, consider the following best practices:
- Keep Threads Lightweight: Only create threads when necessary, as each thread consumes system resources.
- Avoid Excessive Locking: Locking should be minimized to avoid bottlenecks—that is, only use locks around critical sections of code.
- Utilize Thread Pools: To manage thread creation and destruction more efficiently, consider using a thread pool which reuses threads for multiple tasks.
Debugging Threaded Applications
Debugging multithreaded applications can be challenging. Tools like gdb (GNU Debugger) or Valgrind can assist in tracking down race conditions and thread mismanagement. It’s also beneficial to use logging to trace thread activity, ensuring that all outputs are thread-safe.
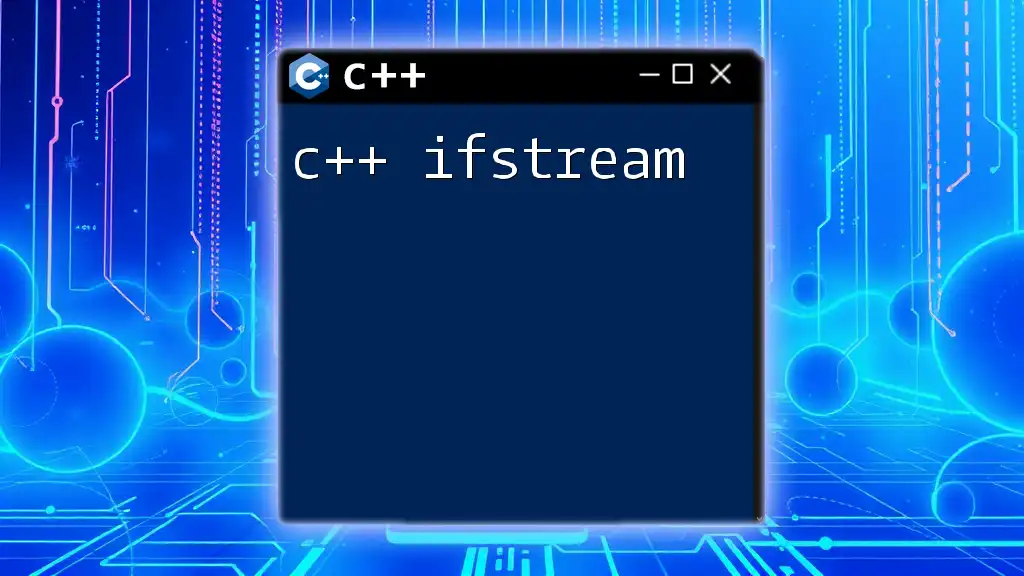
Conclusion
The application of C++ POSIX threads provides a robust framework for developing concurrent applications. Understanding the various aspects of threading—creation, management, synchronization, and error handling—is critical for writing high-performance multithreaded applications.
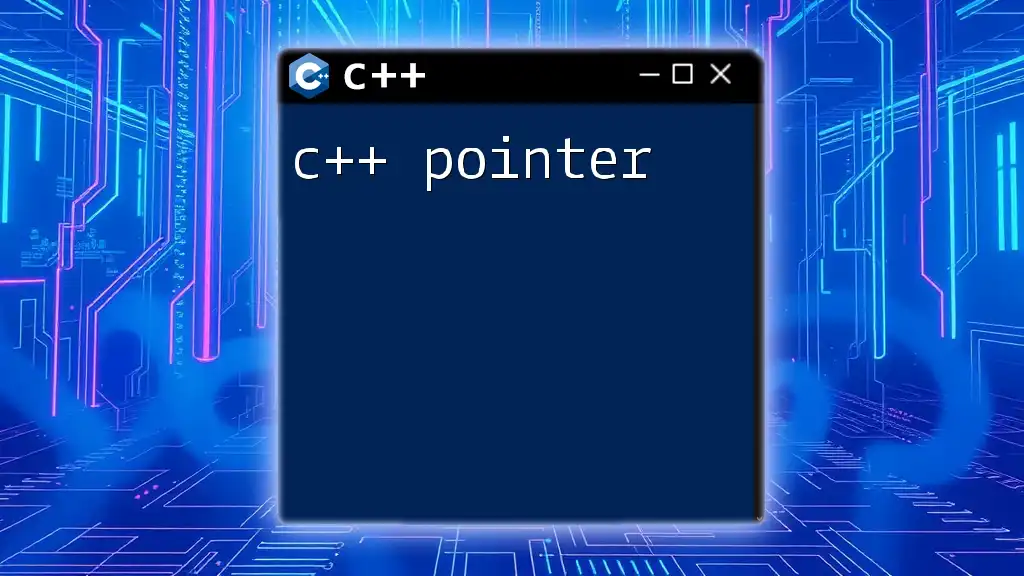
Resources for Further Learning
To expand your understanding of C++ POSIX threads, consider reviewing the official POSIX documentation and exploring various C++ resources online. Community forums and coding platforms can also provide valuable insights and peer support.
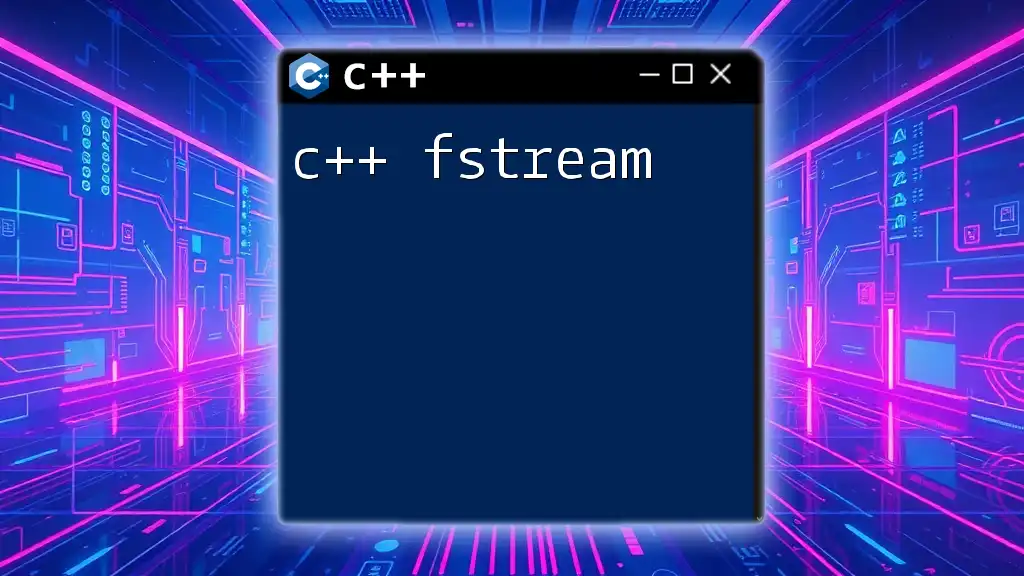
FAQs about POSIX Threads in C++
The use of POSIX threads raises common inquiries, including questions about best practices, cross-platform compatibility, and effective debugging strategies. It is beneficial to engage with communities focused on threading in C++ to deepen your knowledge.