In C++, you can convert a `std::string` to a character array using the `c_str()` method, which returns a pointer to a null-terminated character array representation of the string.
#include <iostream>
#include <string>
int main() {
std::string myString = "Hello, World!";
const char* charArray = myString.c_str();
std::cout << charArray << std::endl;
return 0;
}
Understanding Strings in C++
What is a C++ String?
In C++, a `std::string` is a part of the Standard Template Library (STL) and offers a dynamic way to manage sequences of characters. Unlike C-style strings, which are arrays of characters that end with a null character (`'\0'`), C++ strings handle memory allocation and freeing automatically. This encapsulation provides many advantages, such as eliminating buffer overflows and making string manipulation significantly easier.
What is a Char Array?
A char array, or C-style string, is a contiguous block of memory containing characters, terminating with a null character. Char arrays are essential in scenarios where performance is critical, or for interfacing with legacy code and APIs that do not utilize C++ strings.
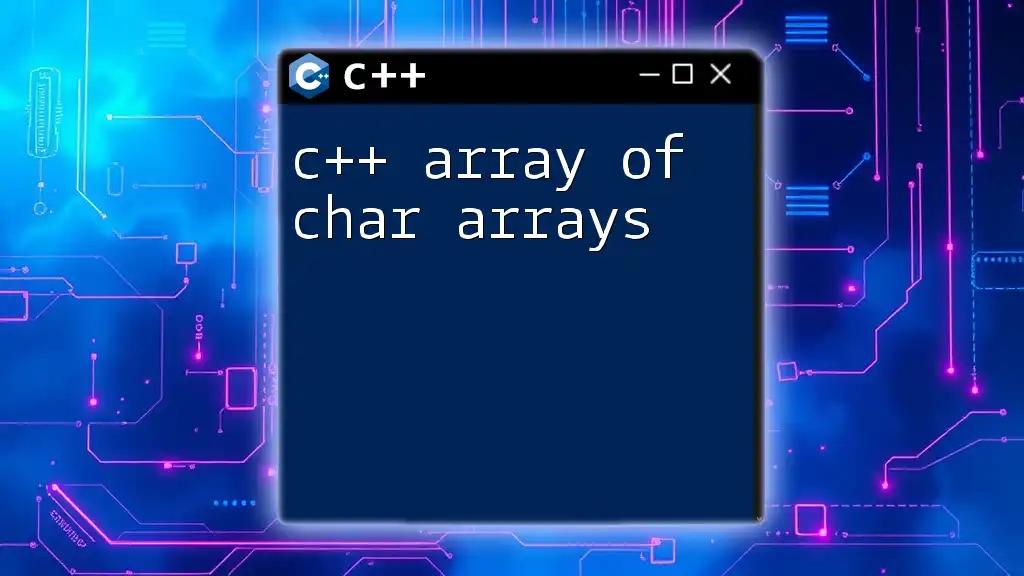
Converting String to Char Array in C++
Converting C++ strings to char arrays is a common requirement, especially when dealing with APIs or functions that expect C-style strings. Below, we explore several methods for performing this conversion effectively.
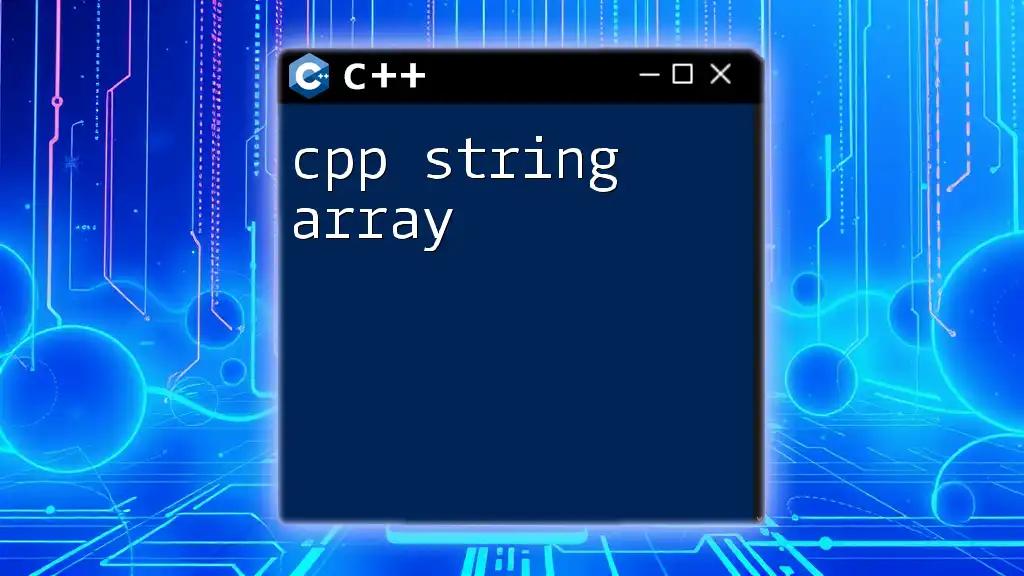
Method 1: Using the `c_str()` Function
How the `c_str()` Function Works
The `c_str()` member function of the `std::string` class returns a constant pointer to the underlying character array, enabling easy access to the string data in C-style format. This function provides a convenient way to get a `const char*` pointer without worrying about memory management, as it automatically ensures the null terminator is correctly placed at the end.
Example usage of `c_str()`:
#include <iostream>
#include <string>
int main() {
std::string cppString = "Hello, World!";
const char* charArray = cppString.c_str();
std::cout << "C++ String: " << cppString << std::endl;
std::cout << "Char Array: " << charArray << std::endl;
return 0;
}
In this example, the output demonstrates how the C++ string is displayed alongside its corresponding char array representation.
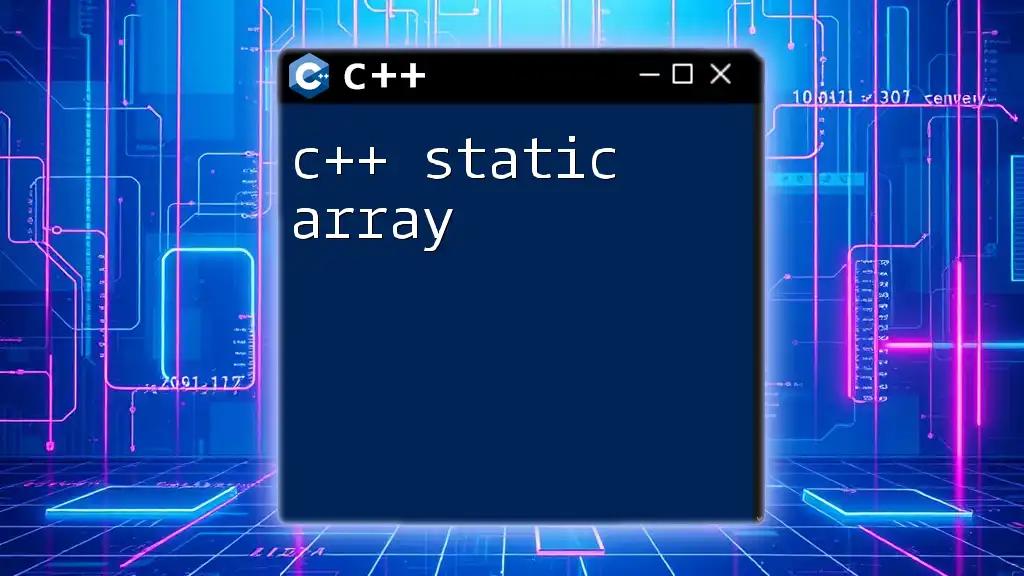
Method 2: Using the `data()` Function
Understanding the `data()` Function
Similar to `c_str()`, the `data()` function returns a pointer to the underlying character array of a C++ string. The main difference between the two is that `data()` was originally designed to provide access to the internal data without a guarantee of the null terminator being present in pre-C++11 versions. Starting from C++11, `data()` behaves similar to `c_str()` by ensuring a null-terminated output.
Example usage of `data()`:
#include <iostream>
#include <string>
int main() {
std::string cppString = "Hello, World!";
const char* charArray = cppString.data();
std::cout << "C++ String: " << cppString << std::endl;
std::cout << "Char Array: " << charArray << std::endl;
return 0;
}
This piece of code demonstrates how straightforward the `data()` function is to use; it effectively retrieves the character array representation without any complex operations.

Method 3: Manual Conversion
Step-by-Step Manual Conversion Process
In some situations, you may need more control over the conversion process, necessitating a manual copy from a C++ string to a char array. This technique offers fine-tuned memory management, especially if you intend to modify the contents of your char array.
Here's how to manually convert a C++ string to a char array:
#include <iostream>
#include <string>
#include <cstring> // Required for strcpy
int main() {
std::string cppString = "Hello, World!";
char charArray[cppString.length() + 1]; // +1 for the null terminator
std::strcpy(charArray, cppString.c_str()); // Manual copy to char array
std::cout << "C++ String: " << cppString << std::endl;
std::cout << "Char Array: " << charArray << std::endl;
return 0;
}
In this code, we allocate a char array that is large enough to hold the original string along with a terminating null character. By using `std::strcpy`, we safely copy the contents, ensuring that all characters are transferred correctly.
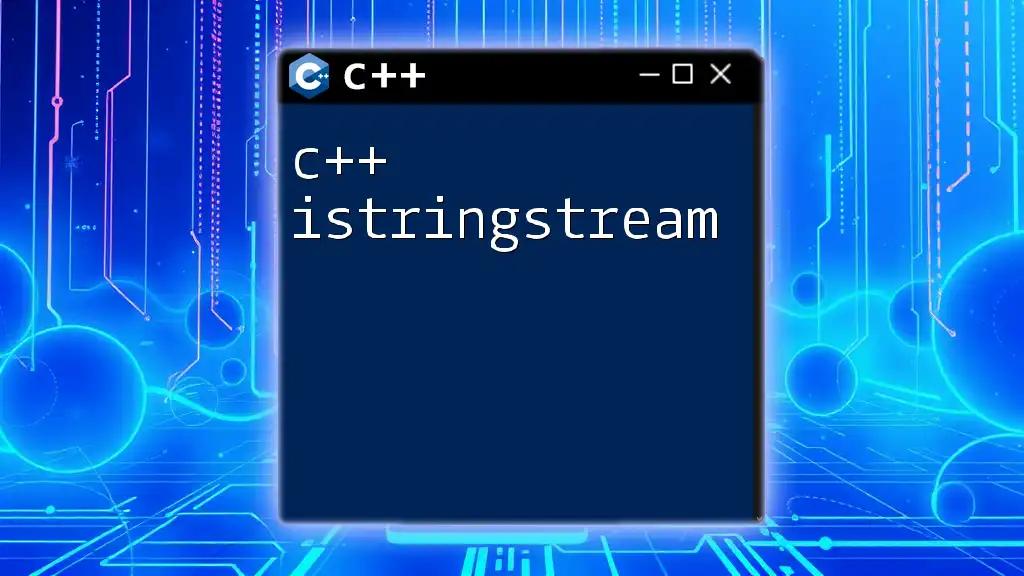
Best Practices for Conversion
When deciding between `c_str()`, `data()`, and manual conversion, consider the use case:
- Use `c_str()` when you need a const pointer to pass to functions that expect a C-style string, especially when you don't intend to modify the contents.
- Use `data()` similarly, with the reassurance that it is safe for C++11 and later versions.
- Manual conversion is best when you require direct modifications of the char array.
It’s crucial to remember that conversion methods like `c_str()` and `data()` return pointers to the internal data of the string; hence, they’re valid only as long as the original string exists. Therefore, treat them as transient and avoid altering their contents.

Common Errors and Troubleshooting
Common Pitfalls
One major mistake is attempting to modify a char array obtained via `c_str()`. Since the returned pointer points to constant data, attempting to modify it can lead to undefined behavior. Always ensure you create a separate copy if modification is necessary.
Another pitfall is accessing uninitialized char arrays. Ensure you properly initialize arrays or utilize library functions that guarantee populated arrays to avoid segmentation faults or garbage values.
Error Handling Tips
To effectively troubleshoot issues related to string conversion, consider these strategies:
- Print Debugging: Use print statements to examine the contents of strings and char arrays before and after conversion.
- Utilize Assertions: C++ assertions can help verify whether pointers are valid or if the expected sizes match.
- Memory Analysis Tools: Software tools that track memory usage can spot leaks or buffer overflows early.
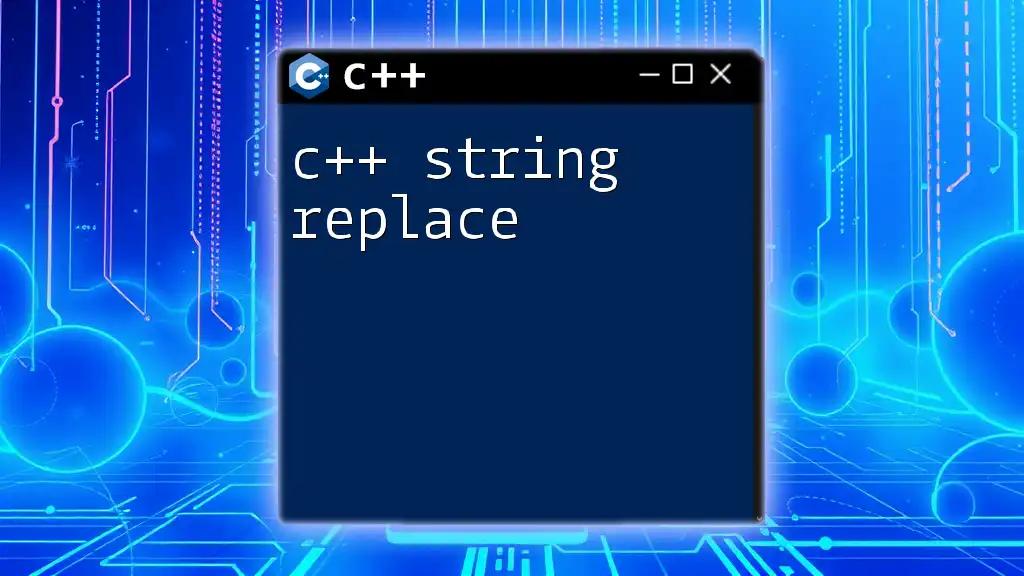
Conclusion
Converting a C++ string to a char array is a fundamental skill in C++ programming that opens a door to working with more traditional C-style string functions and libraries. By understanding the methods of conversion and when to use them, you can effectively manipulate string data in your applications.
Embrace the various techniques discussed, practice using them, and enhance your C++ string manipulation skills to bring your coding prowess to the next level. Happy coding!
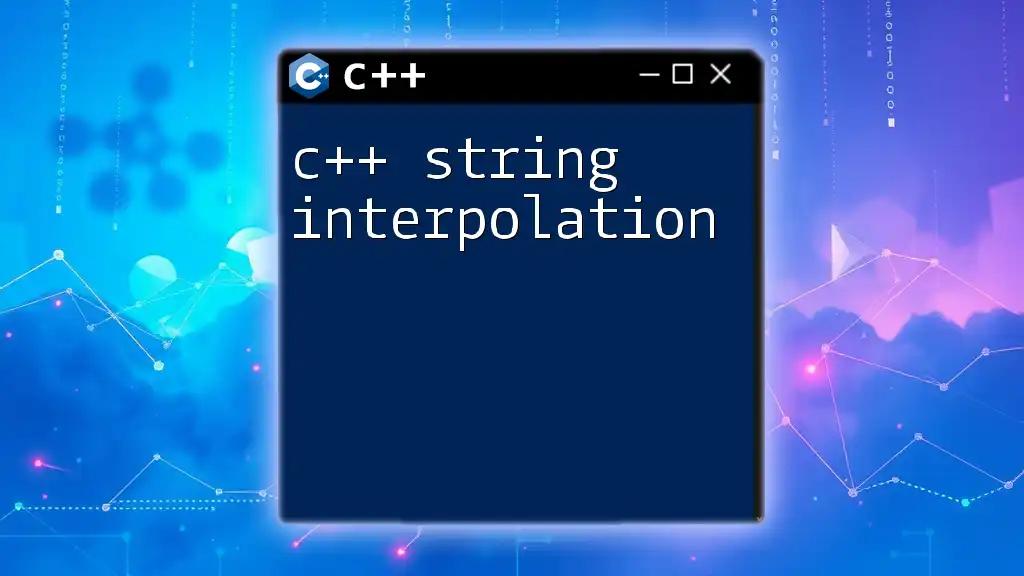
Additional Resources
For further learning, explore the official C++ documentation, online coding platforms, and tutorials that delve deeper into string manipulation. Engaging with these resources will enhance your understanding and facilitate more complex string-related tasks in C++.