Learning C before C++ can provide a solid foundation in programming concepts and syntax, making the transition to C++ smoother and more intuitive. Here's an example code snippet in C to illustrate basic syntax:
#include <stdio.h>
int main() {
printf("Hello, World!\n");
return 0;
}
Understanding C and C++
What is C?
C is a procedural programming language that has been around since the early 1970s. It was developed at Bell Labs by Dennis Ritchie and is known for its efficiency and flexibility. Key features of the C programming language include:
- Low-level access: C allows fine control over system resources and memory.
- Structured programming: Promotes clear and logical program flow through functions.
- Portability: C code can be easily run on various hardware architectures without significant changes.
C is widely used in systems programming, embedded systems, and software application development. It serves as a foundational language that many other modern programming languages build upon.
What is C++?
C++ was developed by Bjarne Stroustrup, also at Bell Labs, in the early 1980s as an extension of C. It introduces object-oriented programming (OOP) features to the efficient and robust foundation set by C. Major features that differentiate C++ from its predecessor include:
- Classes and Objects: C++ supports OOP, allowing developers to encapsulate data and methods.
- Inheritance: Enables new classes to derive properties and behaviors from existing ones, promoting code reusability.
- Polymorphism: Allows functions to operate on different data types, enhancing flexibility.
C++ is a versatile language often used in large-scale applications, game development, and systems where performance and efficiency are crucial.

Comparison of C and C++
Programming Paradigms
C: Programming in C focuses on the sequential execution of code. It excels in procedural programming, where functions are essential units.
C++: Leveraging OOP, C++ allows developers to create classes that define the blueprints for objects—this represents a significant shift in thinking. Understand core OOP concepts such as:
-
Classes and Objects:
class Dog { public: void bark() { std::cout << "Woof!" << std::endl; } }; Dog myDog; myDog.bark(); // Output: Woof!
-
Inheritance:
class Animal { public: void eat() { std::cout << "Eating..." << std::endl; } }; class Dog : public Animal { public: void bark() { std::cout << "Woof!" << std::endl; } }; Dog myDog; myDog.eat(); // Output: Eating... myDog.bark(); // Output: Woof!
Syntax and Language Features
C and C++ share a similar syntax, but C++ introduces several enhancements that facilitate complex programming tasks:
-
Costumes of C++ code allow for cleaner organization compared to C. For example, function overloading in C++ can create multiple functions with the same name but different parameters.
-
Template Programming: C++ supports generic programming through templates that facilitate code reusability.
Memory Management
In C, memory management is manual, requiring developers to use functions like `malloc` and `free` to allocate and deallocate memory. This can lead to issues like memory leaks if not handled meticulously.
int* arr = (int*)malloc(10 * sizeof(int)); // Allocate memory
free(arr); // Deallocate memory
In contrast, C++ introduces constructors and destructors along with `new` and `delete`, allowing for more streamlined memory management. Consider the following example:
class MyClass {
public:
MyClass() { /* Constructor */ }
~MyClass() { /* Destructor */ }
};
MyClass* obj = new MyClass(); // Allocate
delete obj; // Deallocate

Should You Learn C Before C++?
Advantages of Learning C First
- Foundation in Programming: Learning C enhances your understanding of fundamental programming concepts like loops, conditionals, and functions.
- Understanding Memory Management: C trains you to comprehend the importance of memory allocation and deallocation, which is pivotal even in C++.
- Proficiency with Lower-Level Operations: Since C provides a closer experience to hardware, you’ll gain insights into how software interacts with computer systems.
Advantages of Learning C++ First
- Immediate Access to OOP Concepts: Starting with C++ offers you access to modern programming paradigms, allowing you to create more complex and maintainable programs from the outset.
- Enhanced Standard Library: C++ has a richer standard library, such as the Standard Template Library (STL), which simplifies common programming tasks.
- More Relevant for Certain Fields: Many contemporary applications, especially in game development and large systems, lean towards C++, making it a valuable career choice.
Real-World Use Cases
C: Commonly found in operating systems, embedded systems, and applications where performance is critical—examples include Linux kernel and firmware development.
C++: Widely utilized in applications requiring heavy computational efficiency, such as video games (e.g., Unreal Engine) and large-scale systems (e.g., Adobe applications).
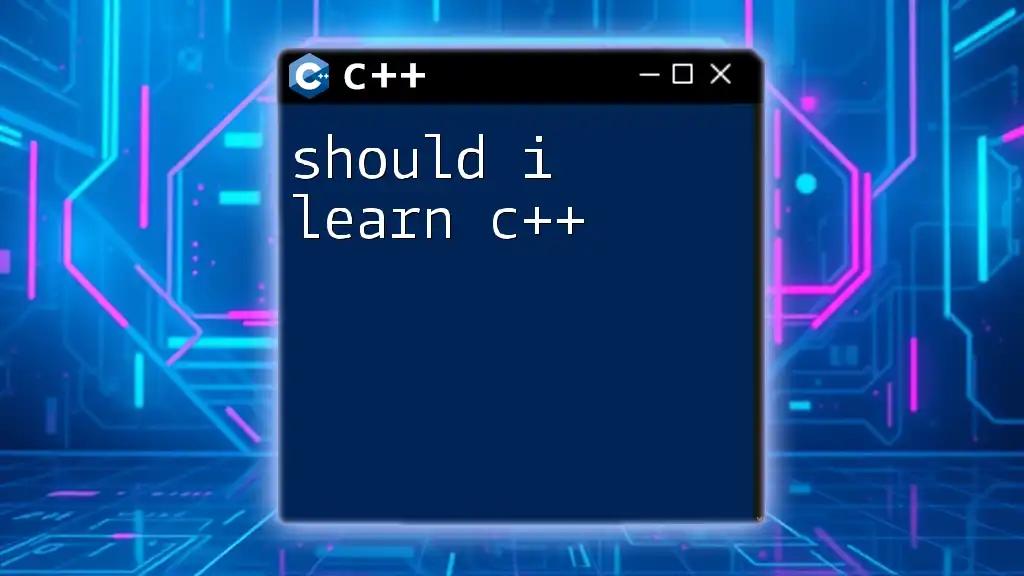
Transitioning from C to C++
Learning Curve
If you start with C, you may find transitioning to C++ much smoother as many fundamental principles carry over. For instance, the understanding of functions, loops, and conditional statements will ease the learning of object-oriented programming in C++.
Key Concepts Inherits from C to C++
Many core concepts from C—such as data types, operators, and control structures—remain relevant in C++. When moving from C to C++, you will recognize that:
- Structs from C can easily evolve into classes in C++.
- Basic input-output operations can be enhanced with C++'s stream objects.
Here's a bridging example:
// C code using struct
struct Point {
int x;
int y;
};
// C++ code using class
class Point {
public:
int x;
int y;
void display() {
std::cout << "Point(" << x << ", " << y << ")" << std::endl;
}
};

Conclusion
In conclusion, deciding should I learn C before C++ depends on your learning style, goals, and the specific applications you aim to develop. Each language has its merits, but starting with C can build a strong foundation that pays dividends when transitioning to C++. However, if you wish to dive into modern programming concepts right away, starting with C++ will provide a more relevant context for many contemporary programming tasks.

Additional Resources
Explore various books, online courses, and community forums dedicated to both C and C++ to deepen your understanding and connect with fellow learners.
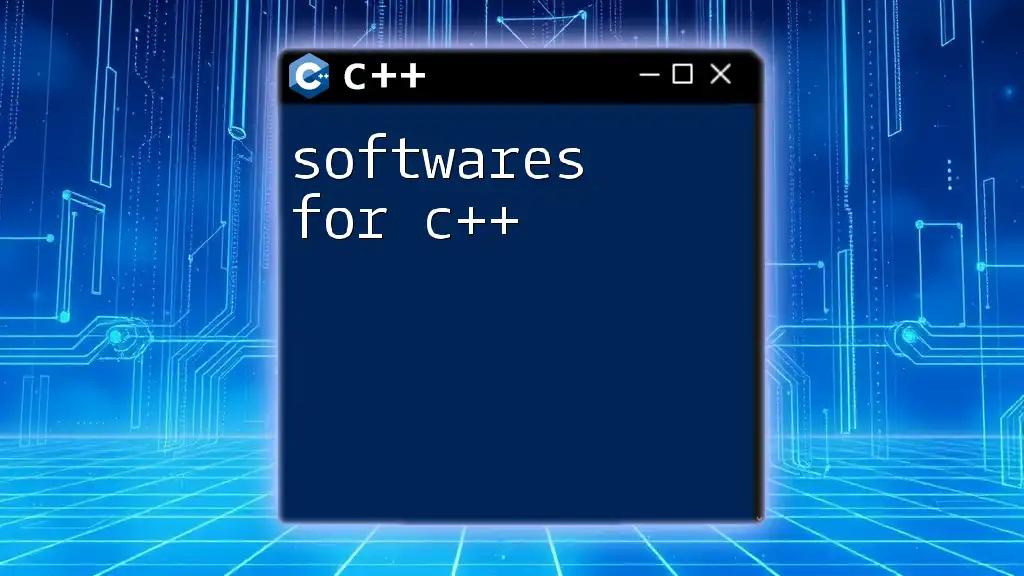
Call to Action
Share your experiences about learning C or C++. Are you leaning towards one over the other? Feel free to sign up for our courses designed to help you master both languages effectively!