The error "expected primary-expression before 'c++'" typically occurs in C++ when the compiler encounters an unexpected token or expression, often due to a syntax issue or misplacement of code.
Here's a code snippet that illustrates a common scenario that could lead to this error:
#include <iostream>
int main() {
int x = 10;
int y = 20;
// Missing semicolon or incorrect expression leads to an error
if (x > y)
std::cout << "x is greater than y" // Error here: expected ';'
else
std::cout << "y is greater than or equal to x";
return 0;
}
Understanding the Error Message
What is "expected primary-expression before 'c++'"?
The error message "expected primary-expression before 'c++'" indicates that the C++ compiler encountered an unexpected input in your code. Compilers are stringent regarding syntax, and this specific error often arises from issues with expressions, whether due to a formatting error, a missing symbol, or an improperly declared variable. Understanding the root causes of this message will help programmers debug their code efficiently.
Common Reasons for the Error
Several scenarios can lead to the "expected primary-expression before c++" error, including syntax mistakes or logic issues. For C++ developers, being aware of these common problems is crucial for maintaining clean and functional code.
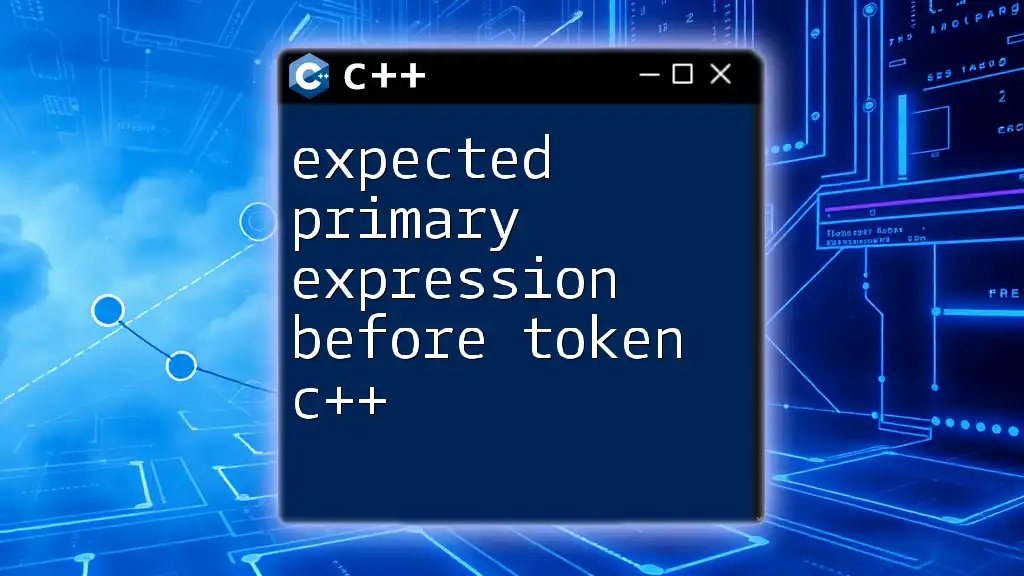
Analyzing Common Scenarios
Missing Semicolons
In C++, each statement must end with a semicolon. When you omit this crucial symbol, the compiler gets confused and generates an error message.
For instance:
int main()
{
int a = 5
int b = a + 10; // Error: expected primary-expression before 'c++'
return 0;
}
In this case, the missing semicolon after `int a = 5` leads to an inability for the compiler to interpret the subsequent line correctly.
Misplaced or Missing Parentheses
Parentheses play a crucial role in defining the order of operations and grouping expressions in C++. If you incorrectly place or forget to include them, it could easily trigger the error.
Consider the example below:
int main()
{
int a = 5;
int b = (a + 10; // Error: expected primary-expression before 'c++'
return 0;
}
Here, the missing closing parenthesis results in the compiler being unable to parse the operation correctly, leading to the reported error.
Incorrect Variable or Function Names
The scope of declared variables is vital in C++. If a variable or function is used before it has been declared, the compiler will not recognize it, creating the error.
For example:
int main()
{
int a = 5;
int b = c + 10; // Error: expected primary-expression before 'c++' - 'c' not declared
return 0;
}
In this instance, since `c` was never declared, the compiler throws the error when attempting to execute the operation.
Type Mismatches
C++ is a statically typed language, meaning that types are checked at compile time. If a mismatch occurs between the expected and actual types, this will lead to the "expected primary-expression before 'c++'" error.
An example is seen here:
int main()
{
int a = 5;
double b = "10"; // Error: expected primary-expression before 'c++' - incompatible types
return 0;
}
The variable `b` is supposed to store a numeric value, but attempting to assign a string ("10") causes a type conflict.
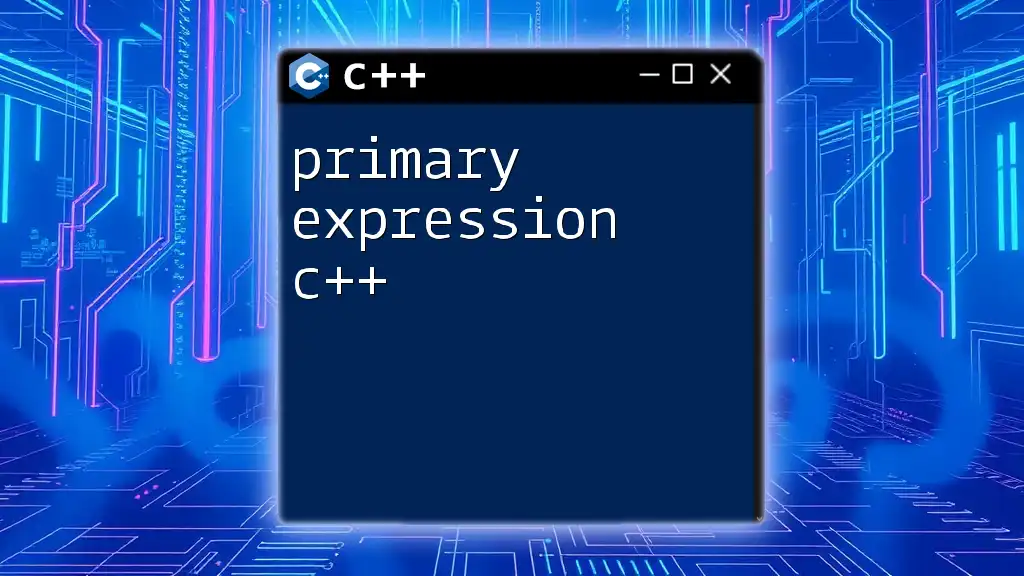
Debugging Techniques
Using Compiler Messages
Compilers provide feedback through error messages, which are invaluable for debugging. While the messages may seem cryptic at times, properly interpreting these can lead to quick solutions. Always refer back to the official documentation regarding error codes and messages to gain a comprehensive understanding.
Step-by-Step Debugging
A systematic approach to debugging can be immensely helpful. When your code produces the "expected primary-expression before 'c++'" error, consider isolating the lines of code leading up to that point. By commenting out lines or breaking down complex expressions, you can pinpoint the issue more effectively.
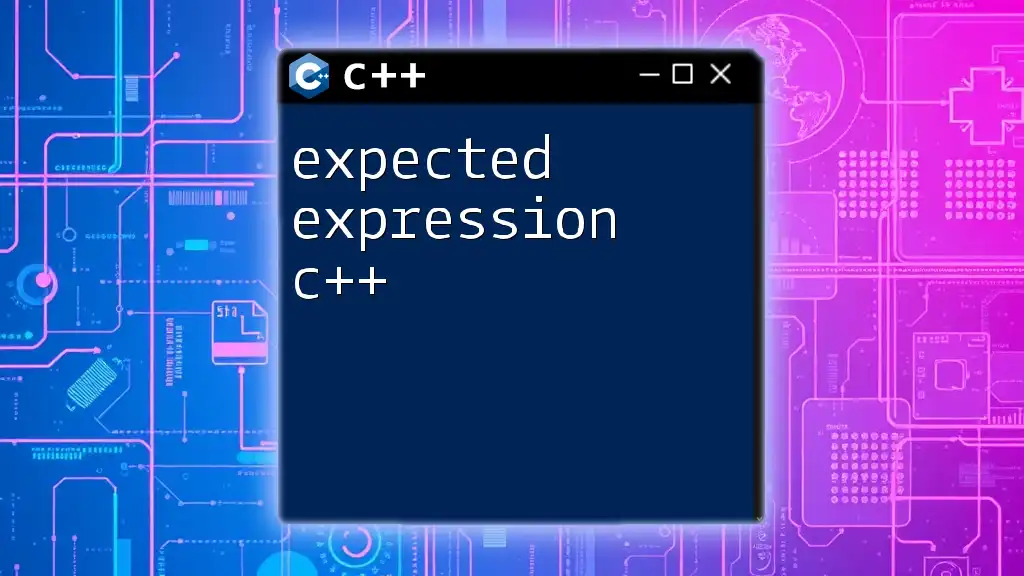
Best Practices to Avoid the Error
Code Structuring
Good coding practices can minimize unnecessary errors. Use consistent indentation and meaningful comments to make your code more readable and maintainable. This helps both you and others who may work with your code in understanding its logic and identifying potential problems.
Regular Code Reviews
Encouraging regular peer code reviews plays a crucial role in catching errors early. Different perspectives can reveal possible mistakes that may be overlooked during personal coding sessions.
Leveraging IDE Features
Modern Integrated Development Environments (IDEs) like Visual Studio, Code::Blocks, and CLion come equipped with various features that assist in identifying errors, including syntax highlighting, code completion, and runtime analysis. Familiarizing yourself with these tools can greatly enhance your coding efficiency and error resolution.
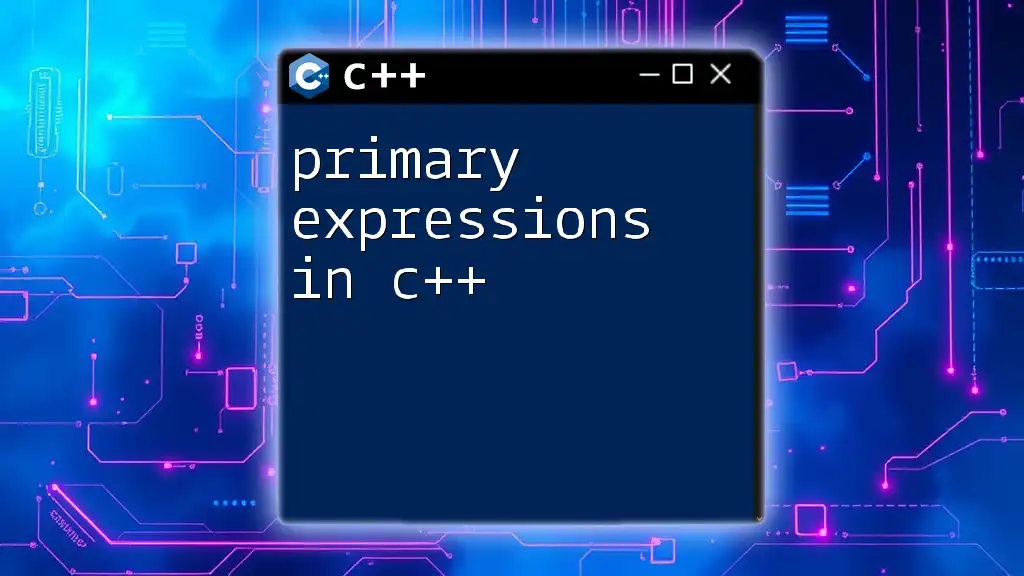
Additional Resources
Recommended Books and Tutorials
Books specifically covering C++ syntax and common pitfalls are a great way to deepen your understanding. Resources like "The C++ Programming Language" by Bjarne Stroustrup and online tutorials at platforms like Udemy or Codecademy can be invaluable.
Community and Support
Engaging with C++ communities, such as forums, Reddit threads, or GitHub discussions, can provide support and insight. Don’t hesitate to seek help or clarification on complex issues; collaboration often leads to quicker problem resolution.
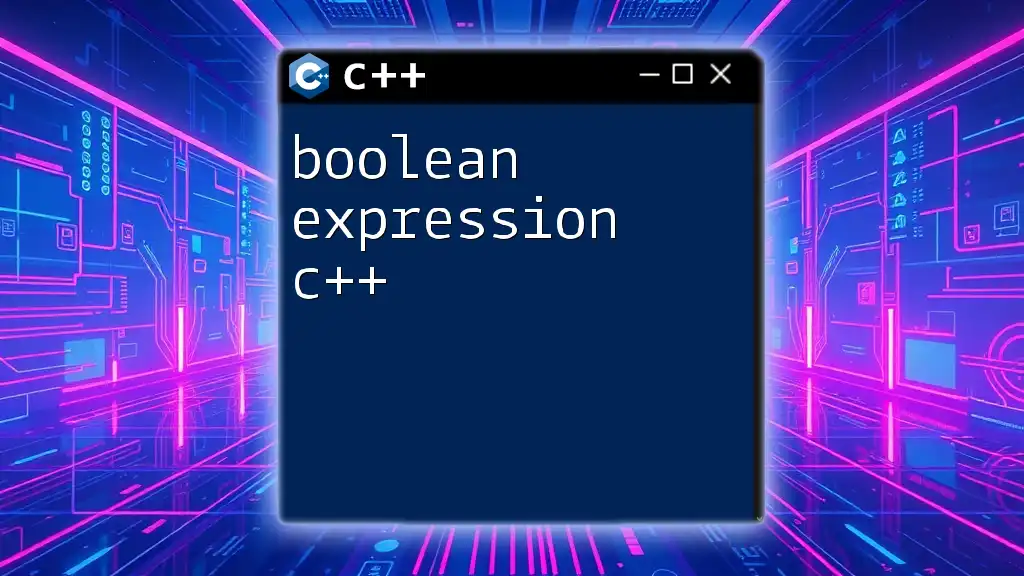
Conclusion
The "expected primary-expression before 'c++'" error can be frustrating, but understanding its root causes and common scenarios will empower you to troubleshoot more effectively. By implementing best practices in coding and taking advantage of community resources, you can tackle this error head-on, ensuring a smoother development experience.
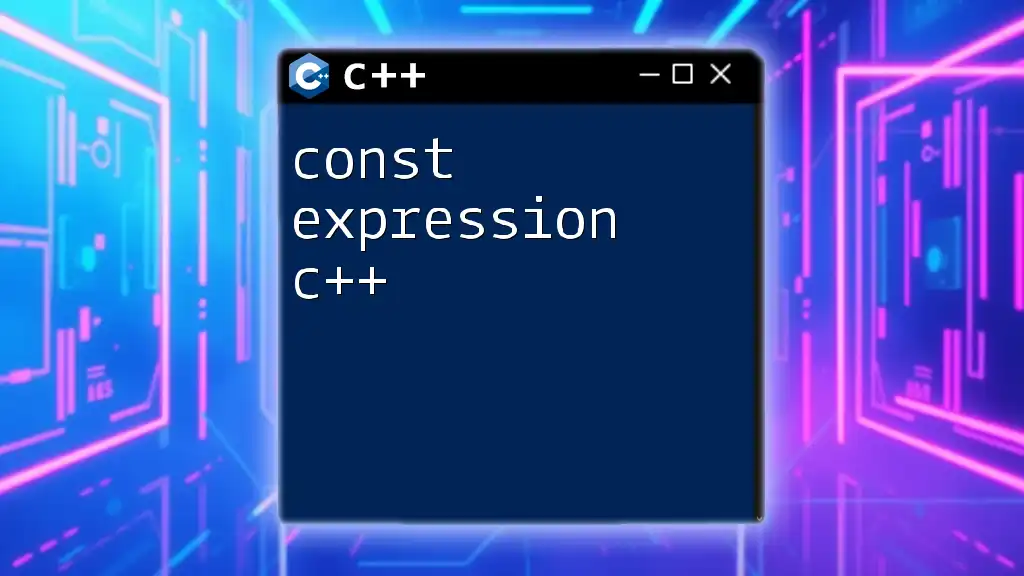
Call to Action
We encourage our readers to share your experiences with the "expected primary-expression before 'c++'" error! Have you come across it in your projects? What strategies worked for you in resolving it? Let’s foster a community of learning by sharing knowledge and insights.