The error "expected primary expression before token" in C++ typically occurs when the compiler encounters an unexpected symbol or token that does not conform to the expected syntax for an expression.
Here’s a code snippet demonstrating a common scenario that triggers this error:
#include <iostream>
int main() {
int x = 10;
// Intentional typo: missing semicolon leads to unexpected token
if (x > 5)
std::cout << "x is greater than 5" // missing semicolon here
return 0;
}
Understanding the Error
What is a Primary Expression?
In C++, a primary expression serves as the basis for expressions in the language and can include a variety of basic constructs. These constructs typically consist of simple elements such as:
- Variables: Names that hold data (e.g., `int x;`)
- Constants: Literal values (e.g., `5`, `true`, `3.14`)
- Parenthetical expressions: Expressions enclosed in parentheses (e.g., `(x + y)`)
Understanding what constitutes a primary expression is essential because the error "expected primary expression before token" typically arises when the compiler anticipates one of these elements but encounters something unexpected instead.
The Meaning of the Error Message
When you see "expected primary expression before token," it signifies that the compiler encountered a token (a character, keyword, or symbol) when it was expecting a valid primary expression. The part of the message "before token" indicates the specific token that the compiler stumbled upon.
Common scenarios include:
- Misplaced commas or semicolons
- Incorrect identifiers or undeclared variables
- Syntax being incorrect, preventing the compiler from interpreting the structure as intended
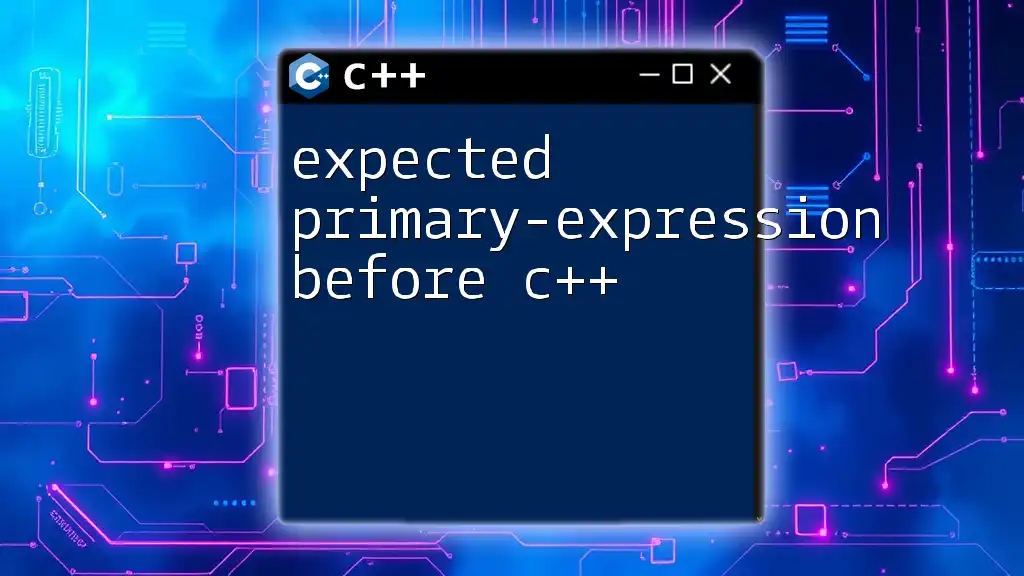
Common Causes of the Error
Missing or Incorrect Syntax
One of the most frequent causes of this error is simple syntactical mistakes. Consider the following example:
int x = 5 // Error: expected primary expression before '5'
In this snippet, the missing semicolon at the end of the line leads to confusion during compilation. The compiler expects a proper termination of the variable declaration, but instead, it encounters the numeric literal ‘5’ without the requisite punctuation.
Fix: Ensure every statement ends with a semicolon. The corrected line should read:
int x = 5;
Using Incorrect Identifiers
The error can also crop up when you use identifiers that have not been declared in the current scope. For instance:
int x = y + 5; // Error: expected primary expression before 'y'
Here, `y` is not declared before it is used in the expression, prompting the compiler to throw an error. In C++, each variable must be declared before you use it in any calculation or operation.
Fix: Declare the variable `y` before using it. For example:
int y = 10;
int x = y + 5; // No error now
Misplaced Operators
You might also encounter the error due to misplaced operators. For instance, consider:
int result = + 5; // Error: expected primary expression before '5'
In this example, the unary plus operator is incorrectly placed without a valid preceding operand. The compiler is expecting a primary expression before encountering the number `5`.
Fix: Ensure that the operator has something to operate upon. The correct use would be:
int value = 5;
int result = +value; // Correct usage
Parentheses Mismatch
Another common cause of the "expected primary expression before token" error is mismatched parentheses. For example:
int a = (b + 3; // Error: expected primary expression
In this case, the open parenthesis is not followed by a corresponding closing parenthesis, leading the compiler to get confused regarding the expression's validity.
Fix: Always ensure that every opening parenthesis has a matching closing parenthesis. The corrected line should read:
int a = (b + 3); // No error now
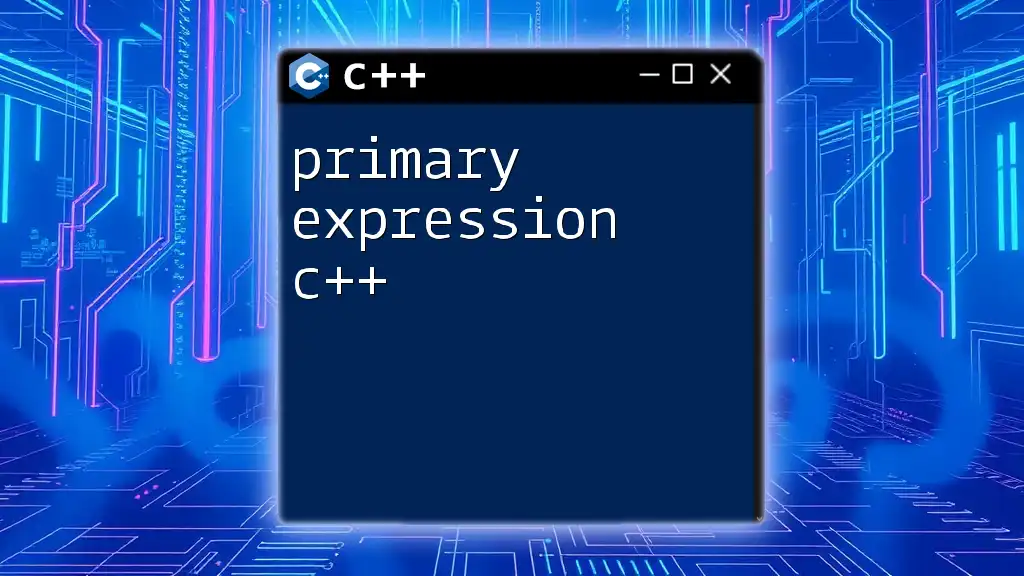
Debugging the Error
Step-by-Step Troubleshooting
When faced with an "expected primary expression before token" error, it’s crucial to approach debugging systematically:
- Identify the Line Causing the Issue: Your compiler will point out the specific line where the error occurred.
- Understand the Context: Look at the preceding lines for any potential issues, as the error may be a result of earlier syntax problems.
- Examine the Token: Focus on the token mentioned in the error message to understand what the compiler was expecting.
Common Tools to Use
Utilizing the right tools can streamline your debugging process:
- IDE Features: Most modern IDEs highlight errors and provide insights that can be beneficial in resolving issues.
- Linters and Static Analysis Tools: These can help identify code quality issues even before compilation, serving as a preventative measure.
- Online Resources: Platforms like StackOverflow and official C++ documentation provide a wealth of information for troubleshooting common errors.
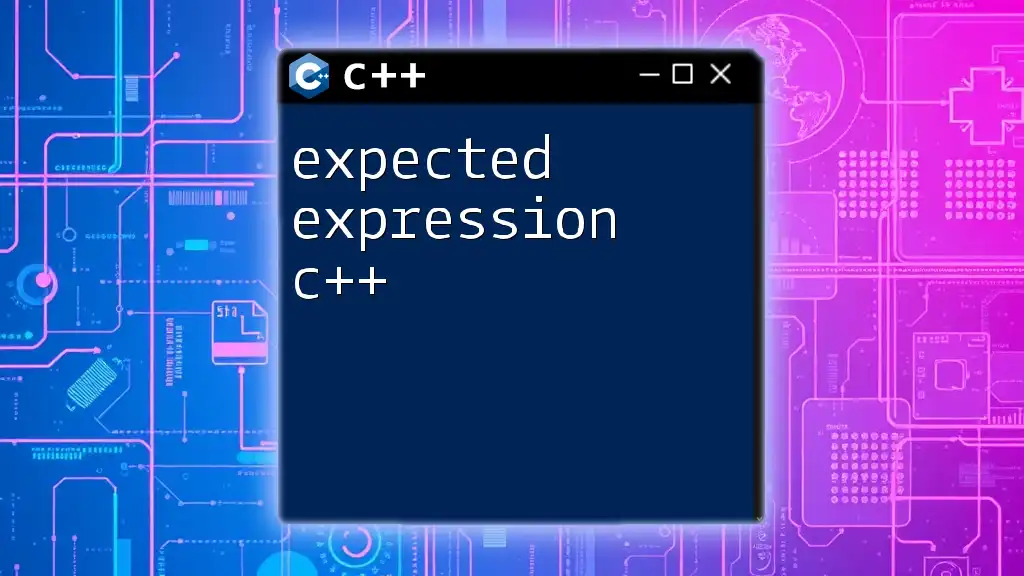
How to Prevent Future Errors
Best Practices in C++ Coding
Preventing errors is often easier than fixing them. By implementing coding best practices, you can significantly reduce the likelihood of encountering syntax-related errors.
- Write clean and readable code: This helps not only in identifying syntax errors but also increases overall code maintainability.
- Conduct regular code reviews: Peer feedback can catch issues that you might overlook.
- Participate in pair programming: Collaborating closely with another developer can uncover mistakes in real-time.
Learning Resources
To improve your C++ skills and understanding of compiler errors, consider exploring various resources:
- Books: Look for titles that focus not just on C++ syntax but also on common pitfalls and advanced concepts.
- Courses: Online platforms offer comprehensive C++ courses that include practical coding assignments.
- Communities: Join forums and user groups that focus on C++ programming. Sharing knowledge with peers can be invaluable.
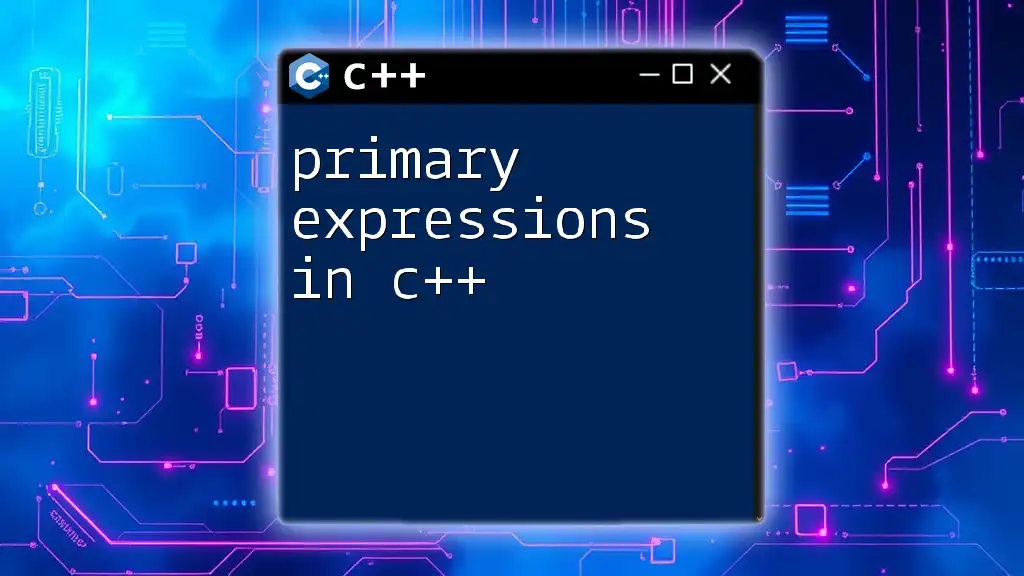
Conclusion
Understanding the error "expected primary expression before token c++" is crucial for any programmer venturing into C++. This error message can often seem cryptic, but by delving into its components and recognizing common causes, you'll become more adept at debugging your code.
As you code, remember that practice makes perfect. The more you debug and solve C++ issues, the more proficient you will become. Embrace the learning process and foster a mindset of continuous improvement, as this will serve you well throughout your programming journey.
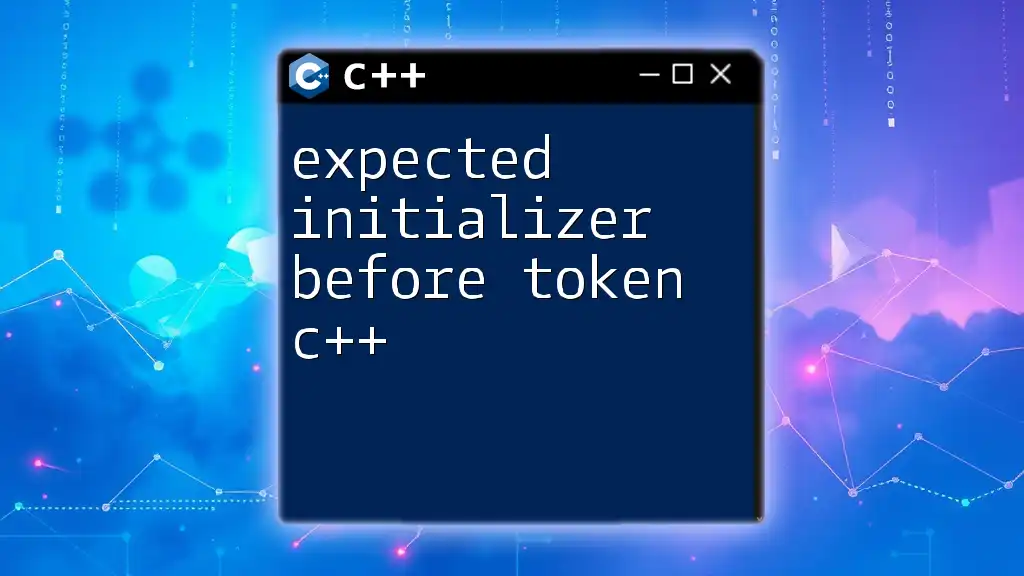
Additional Resources
For further assistance in your C++ journey, consider exploring:
- Official Documentation: Your first stop for understanding language constructs.
- Code Examples Repository: A collection of sample codes that can help illustrate various concepts.
- Contact Information: For inquiries or further support regarding C++ concepts or the services offered by your company.