The error "invalid operands to binary expression" in C++ occurs when you attempt to perform an operation on data types that are incompatible with each other, such as trying to add a string and an integer.
Here's a code snippet demonstrating this error:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, ";
int num = 42;
// This line will cause an "invalid operands to binary expression" error
std::cout << str + num << std::endl;
return 0;
}
Understanding Binary Expressions in C++
What is a Binary Expression?
In C++, a binary expression refers to an expression that contains two operands and one operator. The operands can be constants, variables, or function invocations, and common operators include arithmetic symbols (+, -, *, /), relational symbols (<, >, ==), and logical symbols (&&, ||).
The operands are the values that the operator acts upon. For example, in the expression `3 + 4`, the operator is `+`, and the operands are `3` and `4`. Understanding how binary expressions work is fundamental, as they often serve as the backbone for more complex expressions in C++ programming.
Importance of Type Compatibility
Type compatibility is a crucial aspect of binary expressions in C++. When performing operations, C++ requires that the types of the operands are compatible with the operator being used.
For example, adding an integer to a double is permitted, as C++ will automatically convert the integer to a double. Thus, the expression `5 + 2.0` is evaluated correctly and yields `7.0`. However, attempts to add incompatible types, such as an integer and a string, result in compilation errors.
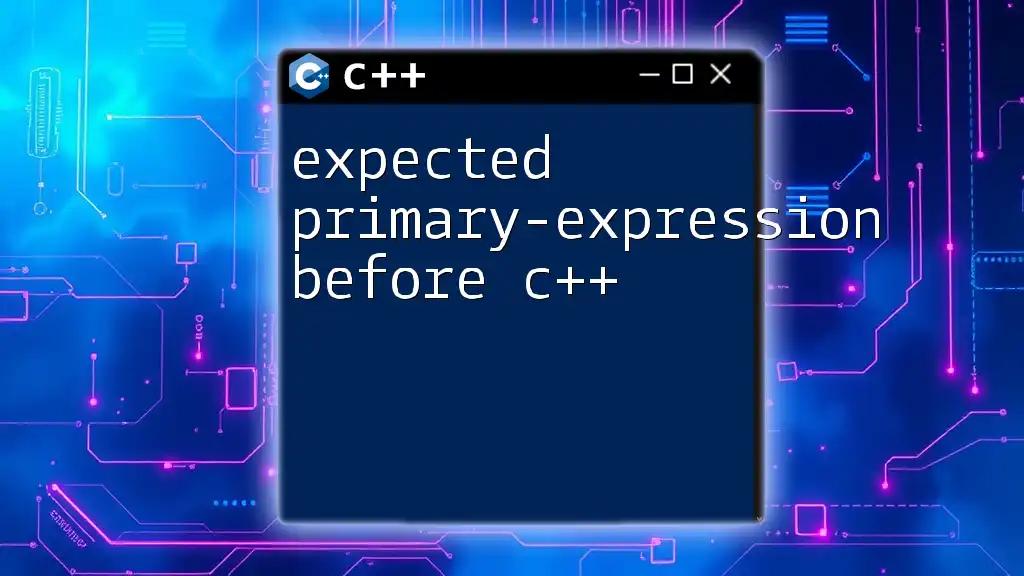
Common Causes of "Invalid Operands to Binary Expression" Error
Mismatched Data Types
One of the most common causes of the "invalid operands to binary expression" error in C++ is when two operands of incompatible types are used in an expression. For instance, if you attempt to add a character and an integer, the compiler throws an error.
char c = 'A';
int num = 5;
auto result = c + num; // This works due to type promotion.
In the example above, while adding a character and an integer might seem valid (because characters can be promoted to their ASCII values), it's essential to be cautious. The actual intention of the code might not be clear, leading to potential logic errors.
Using Non-Overloaded Operators
C++ allows operators to be overloaded, which means you can define custom behaviors for existing operators based on the context of the operands. However, when trying to use a standard operator with objects that have not been overloaded for that operation, you may encounter the "invalid operands to binary expression" error.
For example:
class MyClass {
public:
int value;
MyClass(int v) : value(v) {}
};
MyClass obj1(10);
MyClass obj2(20);
auto sum = obj1 + obj2; // Error: invalid operands to binary expression.
In this case, the addition operator (`+`) has not been defined for `MyClass`. To resolve this issue, we must overload the operator.
MyClass operator+(const MyClass& a, const MyClass& b) {
return MyClass(a.value + b.value);
}
Pointer and Non-Pointer Misalignment
Another common scenario leading to the invalid operands error is the misalignment between pointers and non-pointer types. It's crucial to distinguish between pointer and non-pointer types in C++. An attempt to perform arithmetic on these mismatched types results in errors.
int* ptr = nullptr;
int value = 10;
auto result = ptr + value; // Error: invalid operands to binary expression.
In this snippet, attempting to add an integer to a null pointer will yield an error. To fix this, ensure that you are performing arithmetic on compatible types:
int arr[3] = {1, 2, 3};
int* ptr = arr;
int result = *(ptr + 1); // This is valid and retrieves the second element in the array.
Using Invalid or Uninitialized Variables
Using uninitialized or invalid variables can also lead to the invalid operands to binary expression error. In C++, it is imperative to initialize variables before use.
int a; // Uninitialized variable.
int b = 5;
auto result = a + b; // Error: invalid operands to binary expression.
In the example above, since `a` was not initialized, the compiler raises an error when attempting to use it in arithmetic operations. Always ensure that your variables are initialized properly:
int a = 0; // Now initialized.
int b = 5;
auto result = a + b; // This is valid.

Detailed Examples of the Error
Example 1: Mismatched Types
Let's take a closer look at a valid operation compared to an invalid one.
int main() {
int a = 5;
double b = 3.2;
auto result = a + b; // This is valid in C++, producing result as 8.2
}
Key Point: In this case, C++ will perform automatic type conversion to handle the operation correctly, yet relying on implicit conversions can lead to unintended outcomes if the types are not carefully considered.
Example 2: Pointer Arithmetic
The following snippet demonstrates how using pointers incorrectly can lead to compilation errors:
int main() {
int value = 10;
int* ptr = nullptr;
// Using ptr in arithmetic leads to an invalid expression
// int result = ptr + value; // Uncommenting this generates an error
}
Resolution: To prevent such errors, ensure that pointers are valid before using them in arithmetic.
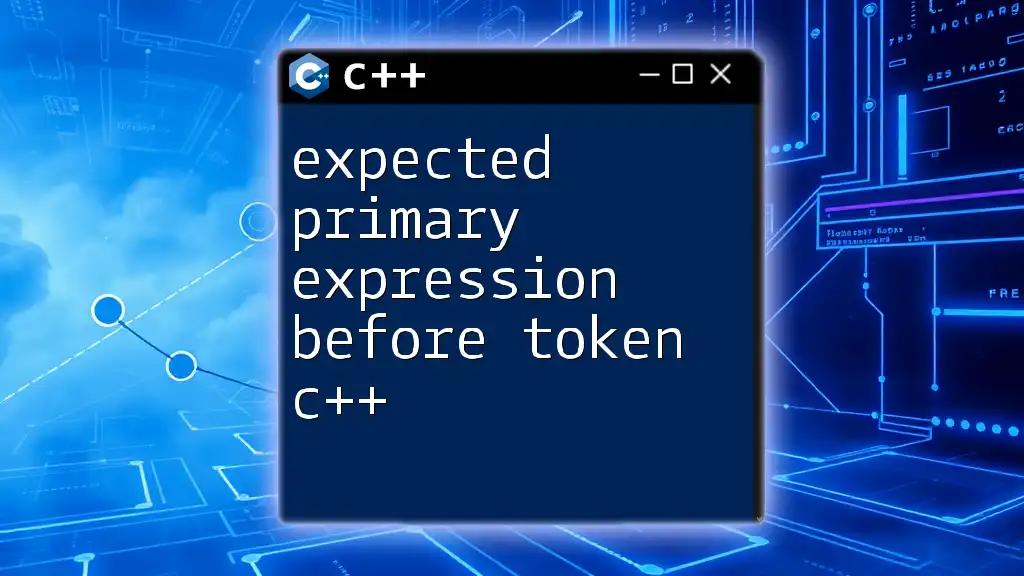
How to Debug the "Invalid Operands to Binary Expression" Error
Reading Compiler Error Messages
Interpreting compiler error messages can often feel overwhelming, but it's essential. When compiling code, the "invalid operands to binary expression" error provides a clear indication that there are incompatible types being used. Referring to the line number and context of the error message can guide you toward the problematic expression.
Using Compiler Options for Better Diagnostics
Using certain compiler flags can enhance your debugging experience. For instance, the `-Wall` and `-Wextra` flags in `g++` alert you to additional potential issues within your code, including type mismatches.
g++ -Wall -Wextra your_code.cpp
Debugging Techniques
When debugging, it’s prudent to isolate the error by stripping down complex expressions to their basic components. This method allows you to identify the exact source of the incompatibility within your code.
Refactor larger binary expressions into smaller parts, and test each part individually. This process helps clarify which parts of your code are causing the compile-time errors.

Best Practices to Avoid This Error
Consistent Data Typing
Maintain consistent data typing throughout your codebase. This practice reduces the risk of encountering the "invalid operands to binary expression" error. By using the same types in expressions, not only do you guard against potential errors, but your code also becomes more readable and maintainable.
Thorough Testing and Validation
Incorporate testing frameworks such as Google Test or Catch2 to systematically validate your code before deployment. Testing helps identify issues in your code early on, ensuring type mismatches are addressed.
Embracing Modern C++ Features
Modern C++ features, such as `std::variant` and `std::optional`, promote safer programming practices. These features assist in managing types in a more controlled manner, preventing many type-related errors.
#include <variant>
std::variant<int, double> getValue(bool isInt) {
if (isInt) return 42; // int
return 3.14; // double
}
// Usage of std::variant ensures type safety
By leveraging these features, you can prevent the common errors associated with binary expressions significantly.

Conclusion
Understanding the common causes of the "invalid operands to binary expression" error, alongside effective debugging techniques, allows you to write more robust C++ code. By maintaining consistent data typing, employing thorough testing strategies, and embracing modern C++ features, you can minimize errors and enhance your programming effectiveness. As you navigate through your programming journey, remember to always prioritize clarity and practicality in your code expressions!