In C++, regular expressions can be used for pattern matching within strings, allowing you to search, replace, and validate text easily.
Here’s a simple example of using regular expressions in C++ to check if a string contains only digits:
#include <iostream>
#include <regex>
int main() {
std::string input = "12345";
std::regex pattern("^[0-9]+$");
if (std::regex_match(input, pattern)) {
std::cout << "The string contains only digits." << std::endl;
} else {
std::cout << "The string contains non-digit characters." << std::endl;
}
return 0;
}
Understanding Regular Expressions
What are Regular Expressions?
Regular expressions, often abbreviated as regex, are special sequences of characters that form a search pattern. They are widely used in programming to perform string searching and manipulation tasks. Regular expressions can validate input, search strings for specific patterns, and even perform complex text replacements. The power of regex lies in its ability to represent complex search criteria using a concise syntax.
How Regular Expressions Work in C++
In C++, the `<regex>` library provides comprehensive support for regular expressions. This library includes several classes to facilitate regex operations, namely:
- `std::regex`: This class represents a compiled regular expression pattern.
- `std::smatch`: A class used for holding the matched results of a regex operation.
- `std::sregex_iterator`: An iterator that helps iterate over matches in a string.
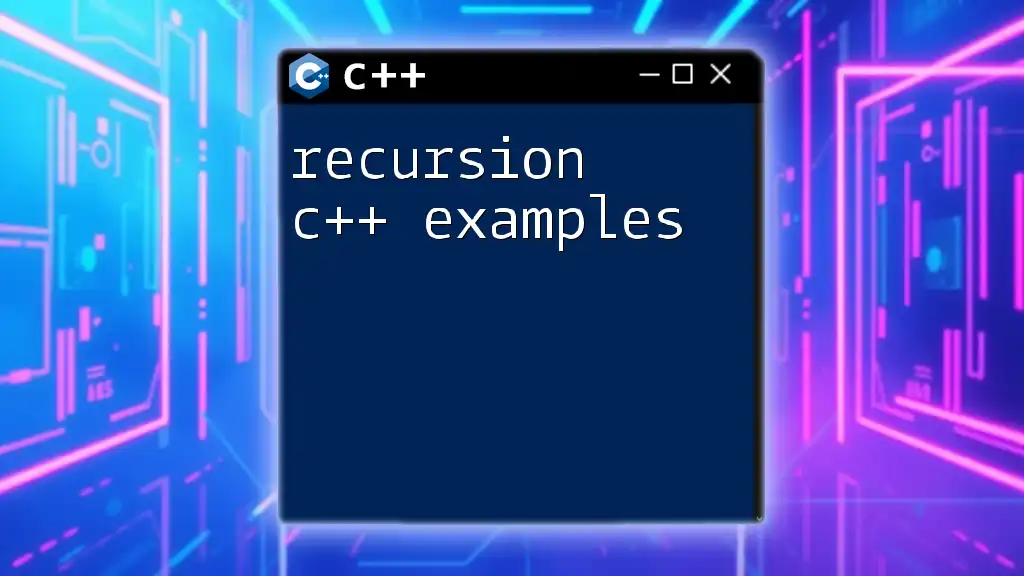
Basic Concepts of Regular Expressions
Pattern Matching
In regex, you can match literal characters as well as utilize special characters called metacharacters. For example:
- `.` matches any character.
- `^` asserts the start of a line.
- `$` asserts the end of a line.
Here’s a simple regex pattern that matches the word "Hello":
std::regex pattern("Hello");
Character Classes
Character classes allow matching a specific set of characters. For example:
- `[abc]` matches any of the characters 'a', 'b', or 'c'.
- `[^abc]` matches any character except 'a', 'b', or 'c'.
- `[0-9]` matches any digit between 0 and 9.
std::regex digits("[0-9]");
Quantifiers
Quantifiers specify how many times a character or group must appear in a string. Examples of quantifiers include:
- `*`: Matches zero or more times.
- `+`: Matches one or more times.
- `?`: Matches zero or one time.
- `{n}`: Matches exactly n times.
- `{n,}`: Matches at least n times.
- `{n,m}`: Matches between n and m times.
For instance, the regex pattern `a{2,4}` matches 'aa', 'aaa', or 'aaaa'.
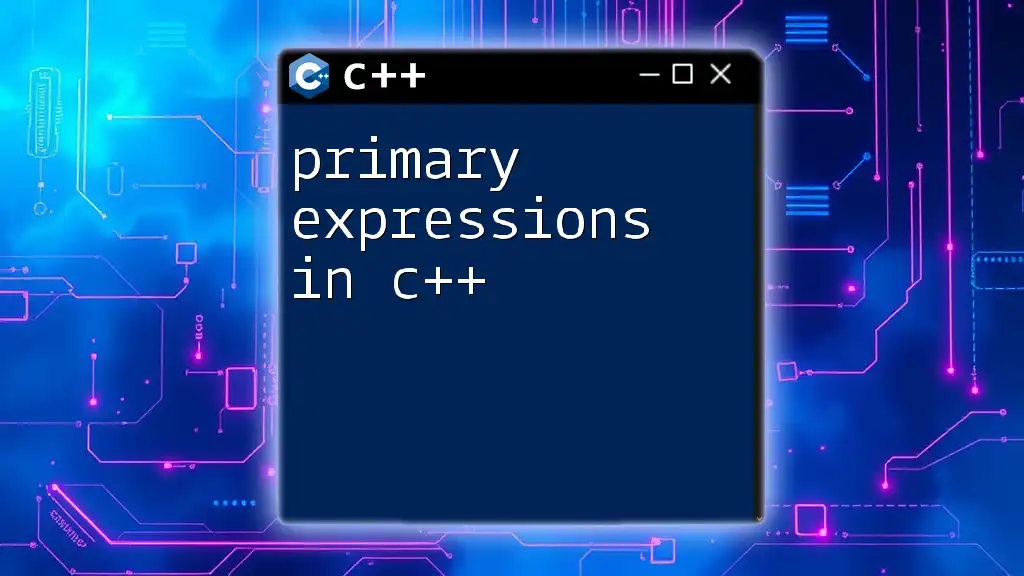
Advanced Regular Expressions
Anchors
Anchors are used to specify the position of a match. The `^` anchor indicates the start of a string, while the `$` anchor signifies the end.
For example, the regex pattern `^Hello$` will only match the string "Hello" if it appears at the start and end of the line.
Groups and Backreferences
Grouping allows you to create sub-patterns within a regex expression. This is done using parentheses `()`. Backreferences, denoted by `\n` where n is the group number, allow you to refer back to previously captured groups within the regex.
For example:
std::regex pattern("(\\d{3})-(\\d{2})-(\\d{4})"); // Matches format 123-45-6789
Lookaheads and Lookbehinds
Lookaheads and lookbehinds add another layer of capability to regex. A lookahead `(?=...)` will match a group only if it is followed by another group, while a lookbehind `(?<=...)` asserts that a group must precede another.
For instance, the regex pattern `(?<=\s)\w+` will match a word that is preceded by a space.
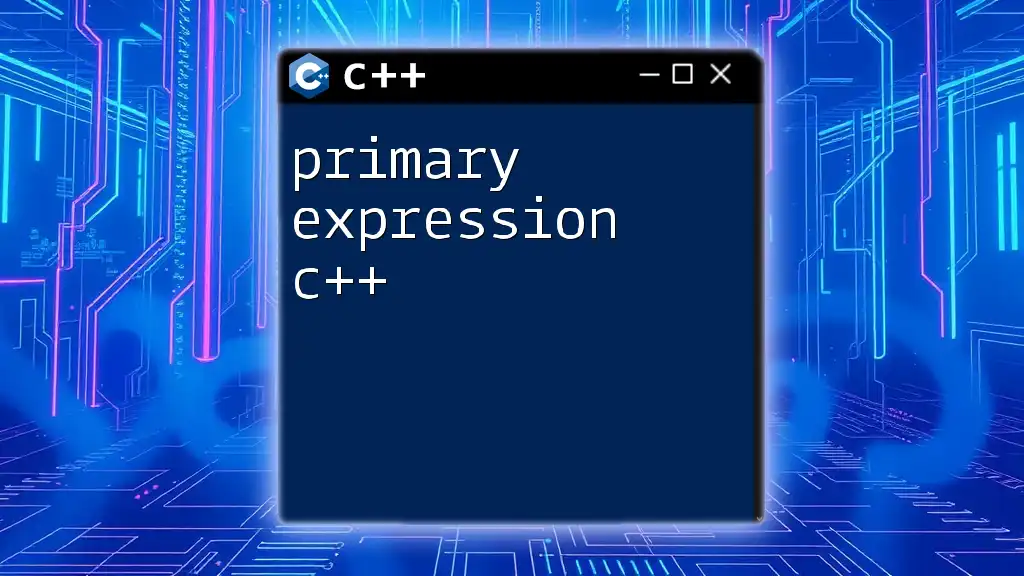
Using Regular Expressions in C++
Setting Up the Environment
To use regular expressions in C++, include the `<regex>` header. The following snippet shows a basic structure for utilizing regex in a C++ application:
#include <regex>
#include <iostream>
#include <string>
Example 1: Email Validation
Validating an email address is a common use case for regex. Here’s a sample regex pattern for this purpose:
std::regex emailPattern(R"((\w+)(\.\w+)*@(\w+)(\.\w+)+)");
The following code snippet validates an email address using this pattern:
std::string email = "example@domain.com";
if (std::regex_match(email, emailPattern)) {
std::cout << "Valid email!" << std::endl;
} else {
std::cout << "Invalid email!" << std::endl;
}
Example 2: String Replacement
Regex can also be utilized for string replacement. For instance, to remove all digits from a string, you can use the following pattern:
std::regex digitPattern("[0-9]");
std::string str = "abc123def456";
str = std::regex_replace(str, digitPattern, "");
std::cout << str; // Output: "abcdef"
Example 3: Extracting Data from Text
Another practical use of regex is extracting specific data, such as phone numbers. Here’s a regex pattern that matches a North American phone number format:
std::regex phonePattern(R"(\(\d{3}\)\s\d{3}-\d{4})");
std::string text = "Call me at (123) 456-7890.";
std::smatch match;
if (std::regex_search(text, match, phonePattern)) {
std::cout << "Found phone number: " << match[0] << std::endl;
}
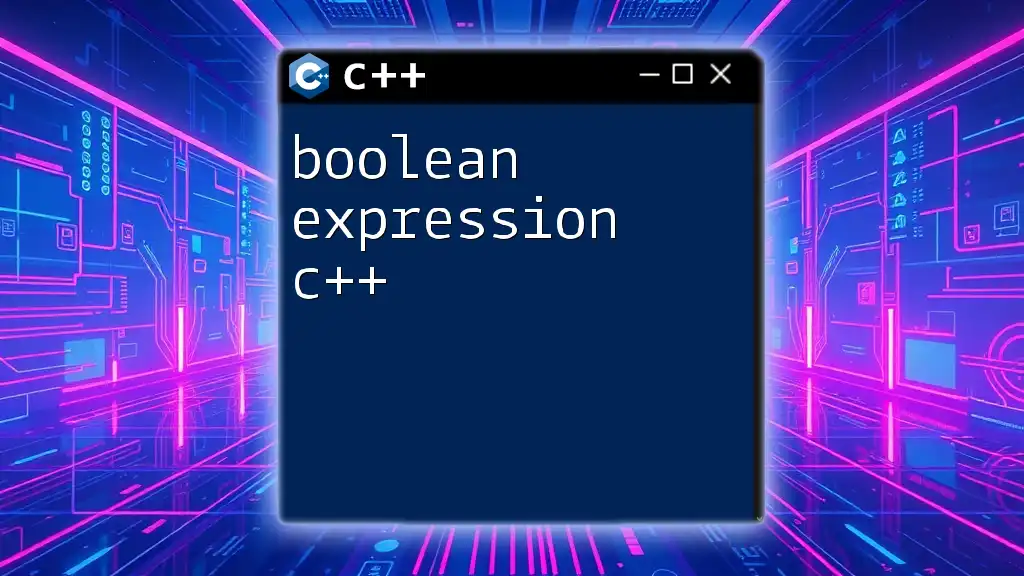
Best Practices for Using Regular Expressions
Writing Readable Regex Patterns
To maintain readability, ensure that complex regex patterns are well-documented. Use comments and whitespace (where allowed) to clarify your intentions, particularly in intricate patterns.
Performance Considerations
Regex operations can be demanding on performance, especially with large datasets. To optimize regex performance, consider pre-compiling your regex patterns and using simple patterns where possible.
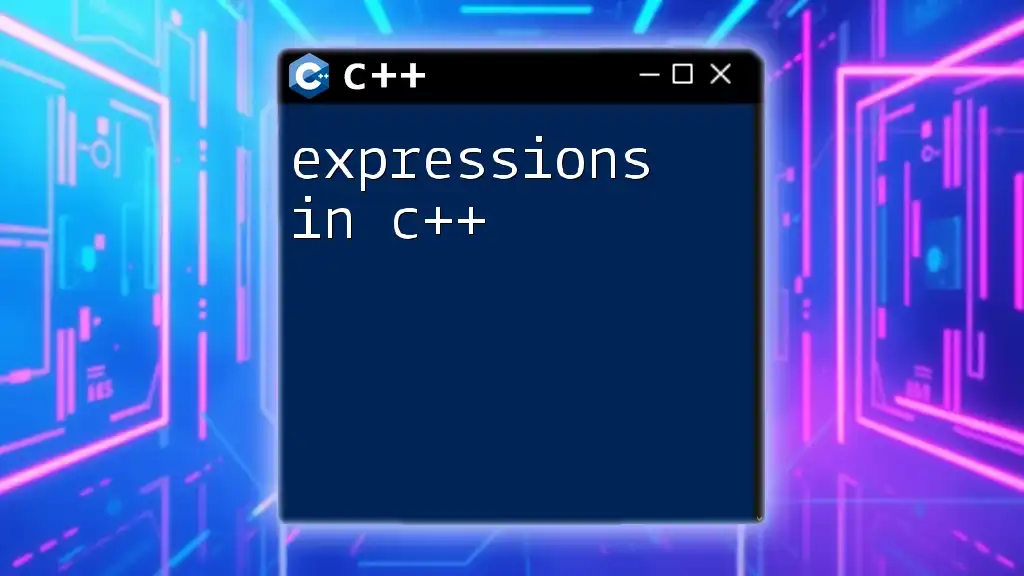
Conclusion
Regular expressions in C++ serve as a powerful tool for string manipulation, allowing developers to implement complex searching, validation, and data extraction with ease. By mastering regex patterns, you can significantly enhance your programming efficiency and capabilities. Dive into practice with the examples provided and explore further applications in your projects to solidify your understanding.