The `friend` keyword in C++ allows a function or another class to access the private and protected members of the class in which it is declared.
class MyClass {
private:
int secretNumber;
public:
MyClass() : secretNumber(42) {}
friend void revealSecret(MyClass& obj);
};
void revealSecret(MyClass& obj) {
std::cout << "The secret number is: " << obj.secretNumber << std::endl;
}
Understanding Compatibility in C++
The friend keyword c++ serves a specific purpose in C++ programming. It allows a certain function or class to access the private and protected members of another class. This capability enhances the flexibility of C++ by granting controlled access to encapsulated data while preserving the benefits of encapsulation.

Why Use the `friend` Keyword?
Access Control in C++
C++ uses access specifiers like `public`, `private`, and `protected` to define the visibility of class members. The friend keyword c++ effectively bypasses these access controls, allowing a friend function or class to access even the most restricted members. This is particularly useful when a certain function or class needs to perform operations on another class without exposing all members to the outside world.
Encapsulation and Friendships
Using friend in C++ is about balancing encapsulation with functionality. On one hand, friends can access private data which might otherwise remain hidden; on the other, this can lead to less secure code if overused. Hence, while the friend keyword c++ provides great power, it should be wielded judiciously.

Types of Friends in C++
Friend Functions
What is a friend function? A friend function is a standalone function that has special access rights to a class’s private and protected members. Unlike member functions, it is not associated with any object of the class, yet it can access the private data.
For instance, consider the example:
class Box {
private:
double length;
public:
Box(double l) : length(l) {}
friend void printLength(Box b);
};
void printLength(Box b) {
std::cout << "Length of Box: " << b.length << std::endl;
}
In this example, the function `printLength` is a friend of the `Box` class. It can access the private member `length`, allowing it to perform its intended function seamlessly.
Friend Classes
A friend class takes the concept a step further: an entire class is declared as a friend of another class. This grants access to all private and protected members of the class it befriends.
Consider the following example:
class Box {
private:
double length;
public:
Box(double l) : length(l) {}
friend class BoxPrinter;
};
class BoxPrinter {
public:
void printLength(Box b) {
std::cout << "Length of Box: " << b.length << std::endl;
}
};
Here, `BoxPrinter` can access the private member `length` of `Box`. This capability is particularly useful when one class needs to manage or manipulate another class’s data closely.
Friend Function Overloading
One interesting aspect of friend functions is that they can be overloaded just like regular functions. The key point to remember is that overloaded friend functions can still access private members of the class they befriend.
For example:
class Box {
private:
double length;
public:
Box(double l) : length(l) {}
friend void printLength(Box b);
friend void printLength(Box b, const std::string &prefix);
};
void printLength(Box b) {
std::cout << "Length: " << b.length << std::endl;
}
void printLength(Box b, const std::string &prefix) {
std::cout << prefix << " Length: " << b.length << std::endl;
}
In this code, both versions of `printLength` can access the private member `length`, showcasing how the friend keyword c++ allows for flexibility in function design.

Common Misconceptions About `friend`
One common misconception is that the use of friend keyword c++ inherently breaks encapsulation. While it does allow access to private members, it's about controlled access. If used wisely, it can help maintain clear and manageable code.
Another myth is the belief that friend should be avoided at all costs. It’s crucial to understand when and where to utilize it effectively. For instance, if class A needs to interact frequently with class B, declaring A as a friend enables efficient communication without exposing its members to all external classes.

Performance Considerations
Regarding performance, it’s important to note that friend functions can improve efficiency in scenarios where access to private members is required. A friend keyword c++ function may execute faster in some situations since it can access data directly without needing additional getter functions. However, the performance gain is often minimal and should not be the driving factor behind using friends.

Best Practices for Using `friend`
When considering the friend keyword c++, adhere to several best practices:
-
Use sparingly: Only declare a function or class as a friend when absolutely necessary. Overusing friends leads to tight coupling between classes, making maintenance more challenging.
-
Keep friend declarations minimal: Do not declare every class as friends. Instead, limit friendship to a few necessary classes that require access.
-
Maintain readability and clarity: Clearly document the reasons why certain classes or functions are declared as friends. This communication helps future developers understand your design decisions.
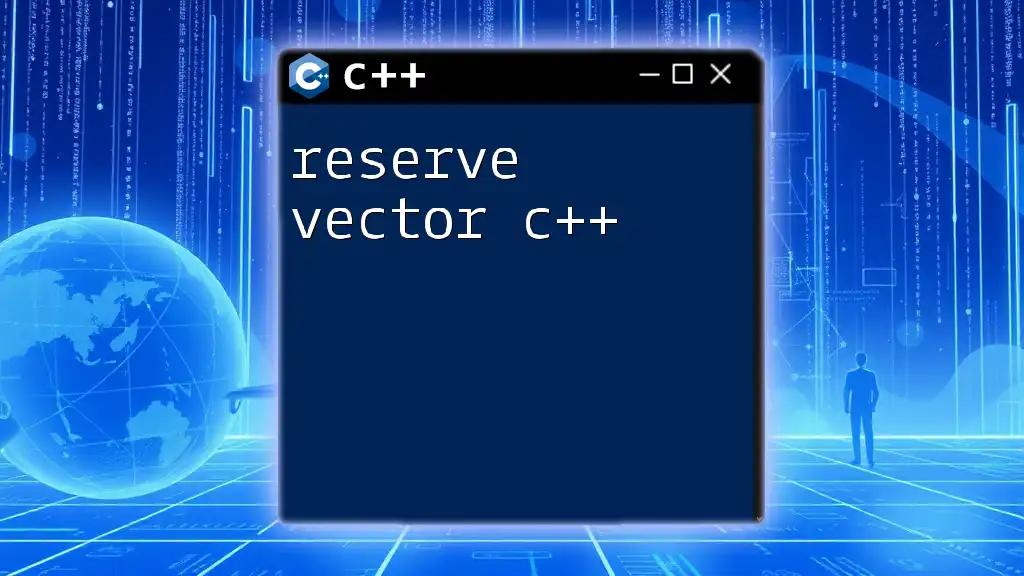
Conclusion
Understanding the friend keyword c++ is crucial for developing efficient and maintainable C++ code. It allows selective access to class members, enabling functionality without compromising encapsulation. However, due care should be taken to avoid excessive use.
As you advance in C++ programming, exploring the practical applications of the friend keyword c++ and how it meshes with good object-oriented practices will greatly enhance your coding proficiency.

Frequently Asked Questions (FAQs)
What is the difference between a friend function and a member function?
A key difference is that a friend function is not associated with any object of the class, while a member function is explicitly tied to an instance of a class. While both have access to class members, the scopes of their usage differ significantly.
Can a class be a friend of itself?
Yes, a class can declare itself as its own friend. This is often used when you want to grant certain member functions access to other private members.
Are friend functions inherited in derived classes?
No, friend functions are not inherited by derived classes. Each class defines its own set of friends, and these privileges do not carry over to subclasses. This ensures that privacy controls remain intact in the class hierarchy.