The `override` keyword in C++ is used to indicate that a virtual function is intended to override a base class's virtual function, providing better code readability and enabling the compiler to produce an error if the function does not correctly override any base class function.
class Base {
public:
virtual void show() { std::cout << "Base class show()" << std::endl; }
};
class Derived : public Base {
public:
void show() override { std::cout << "Derived class show()" << std::endl; }
};
What is the Override Keyword?
The c++ override keyword is a specifier used in C++ to indicate that a function in a derived class is intended to override a virtual function in its base class. By using the `override` keyword, programmers can ensure that the function signatures match and that the derived class's function truly overrides the base class function. This not only helps in maintaining the integrity of object-oriented programming principles but also enhances code readability and maintainability.
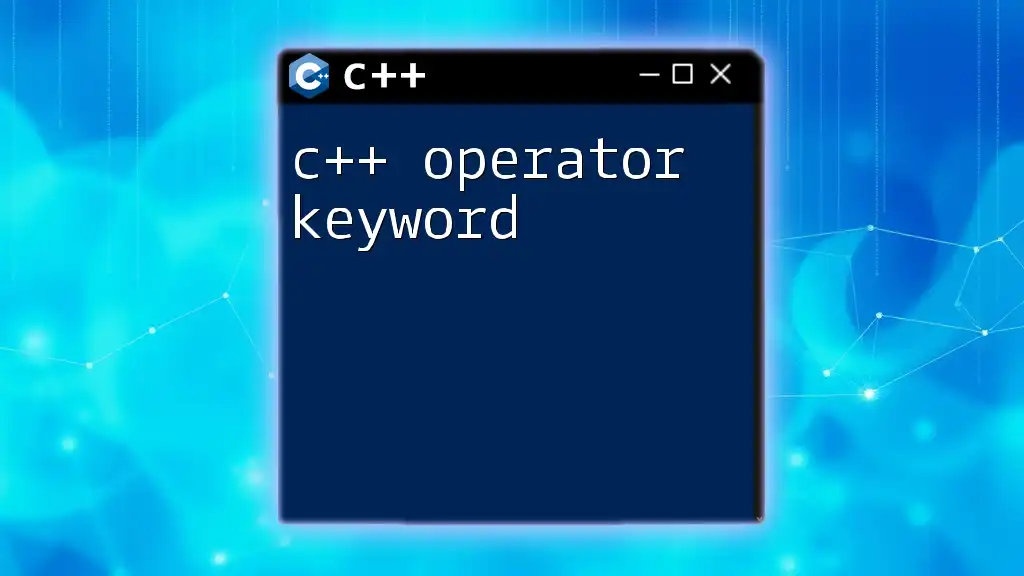
Understanding Function Overriding
In the context of object-oriented programming (OOP), function overriding occurs when a derived class provides its own implementation of a method that is already defined in its base class. The process relies on the polymorphic behavior of C++, where the type of the object determines which method to invoke at runtime.
Consider the following example demonstrating basic function overriding:
class Base {
public:
virtual void display() {
std::cout << "Base Display" << std::endl;
}
};
class Derived : public Base {
public:
void display() override { // Using the override keyword
std::cout << "Derived Display" << std::endl;
}
};
In this example, we have a `Base` class with a virtual method `display()`. The `Derived` class overrides this method. When a `Derived` object calls `display()`, it outputs "Derived Display," demonstrating the principle of overriding.
Benefits of Function Overriding
Using function overriding provides several advantages:
- Increased code flexibility: The ability to alter behavior by overriding methods makes the code more adaptable to changes.
- Enhanced readability and maintainability: Specifying `override` clarifies intentions and helps maintainers immediately understand the relationship between the derived and base classes.
- Simplified use of polymorphism: This allows functions to utilize base class pointers or references that can lead to derived class implementations being executed, which is crucial for utilizing polymorphic behavior effectively.
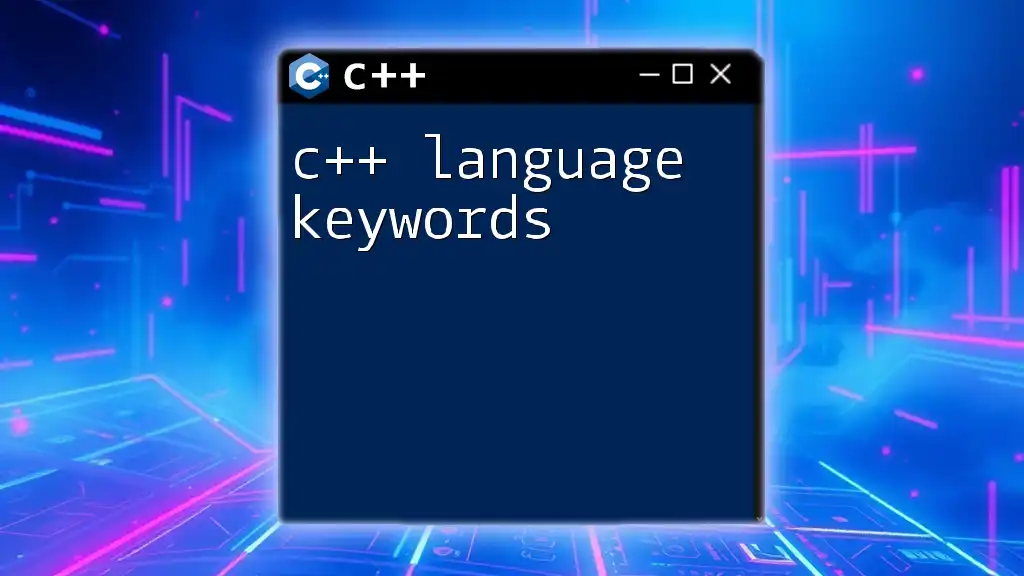
The Syntax of the Override Keyword
The syntax for using the c++ override keyword is straightforward. For a derived class to properly override a virtual function from a base class, it needs to match the base class function's signature precisely.
Consider this example illustrating correct and incorrect usage:
class Base {
public:
virtual void show() {
std::cout << "Base Show" << std::endl;
}
};
class Derived : public Base {
public:
void show() override; // Correct Usage
// void show(int) override; // Incorrect Usage - Error
};
When using the `override` keyword, it's essential to ensure that the derived function signature matches that of the base class. Deviation will lead to a compilation error, which is beneficial as it catches mistakes early in the development process.
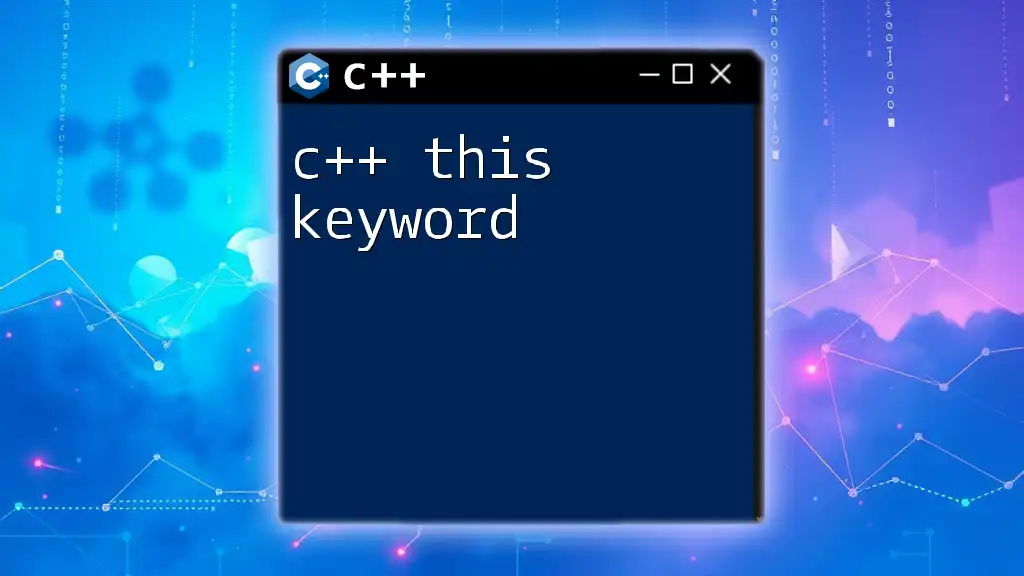
Rules and Validations for Using the Override Keyword
To successfully apply the c++ override keyword, certain rules and validations must be adhered to:
- The derived class function must match the signature (name, parameter types, const-ness) of the base class function.
- The base class function must be declared with the `virtual` keyword for overriding to be valid.
Understanding error scenarios can further illuminate the significance of these rules. For instance, if you accidentally change the parameter type while overriding a method, the compiler will raise an error:
class Base {
public:
virtual void greet() {
std::cout << "Hello from Base" << std::endl;
}
};
class Derived : public Base {
public:
// Incorrect: changing the signature by adding an int parameter
void greet(int) override; // Compiler Error
};
This enforces good practices while developing, preventing silent errors that could lead to runtime problems.
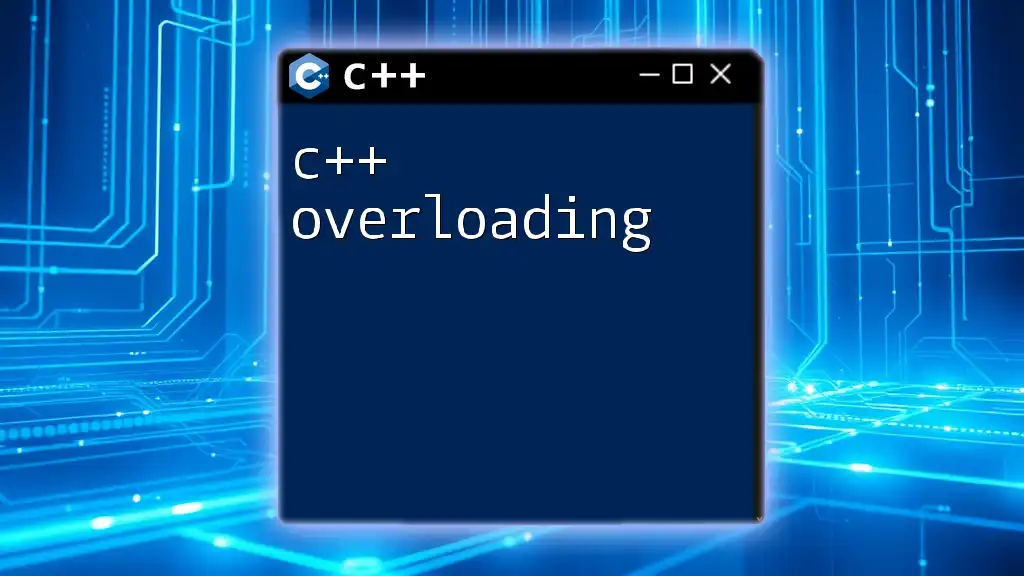
When to Use and When Not to Use Override
The c++ override keyword should be used when you are effectively overriding a function from a base class to ensure both clarity and correctness in your code. Here are some scenarios to consider:
- Use the `override` keyword when extending functionalities of base class methods while providing a specific implementation in derived classes.
- It's not necessary to use the `override` keyword in classes that do not intend to override any base class functionality. For example, standalone functions in a class hierarchy do not require it.
Additionally, understanding its interaction with templates and inheritance can guide its effective application. While templates introduce complexity in function resolution, the `override` keyword remains crucial in adhering to standard inheritance protocols.
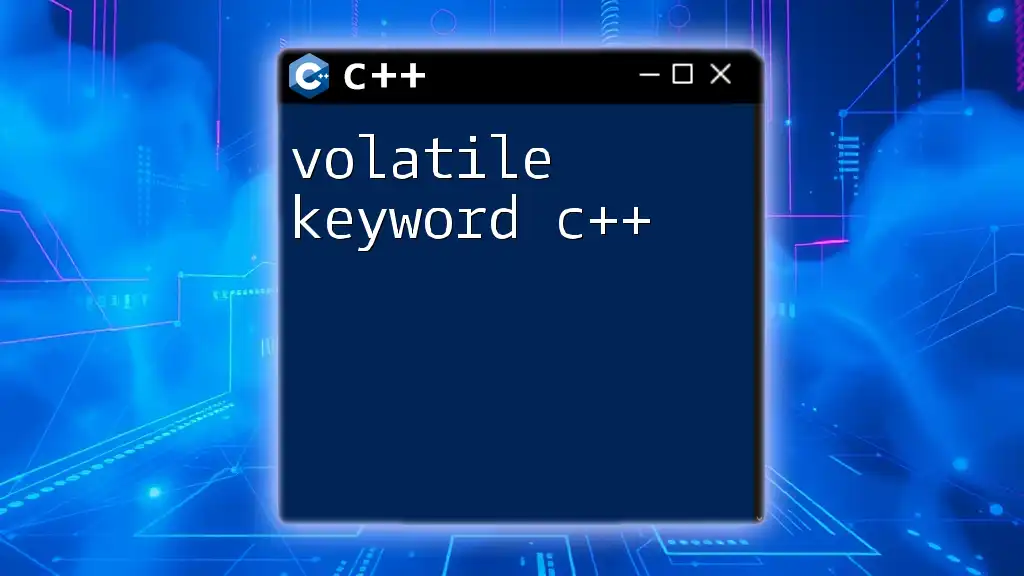
Common Mistakes with the Override Keyword
Sometimes developers make avoidable errors when using the c++ override keyword. It's essential to be aware of these common mistakes:
- Forgetting to include the `virtual` keyword in the base class function can lead to a scenario where the derived class function does not override the base class function.
- Misunderstanding function signatures can lead to compile-time errors that can disrupt the application flow.
Here’s an example of a common mistake:
class Animal {
public:
virtual void makeSound() {
std::cout << "Animal sound" << std::endl;
}
};
class Dog : public Animal {
public:
void makeSound(int volume) override; // Incorrect: parameter mismatch
};
In this case, the `Dog` class tries to override `makeSound`, but it introduces an additional parameter, leading to a compile-time error.
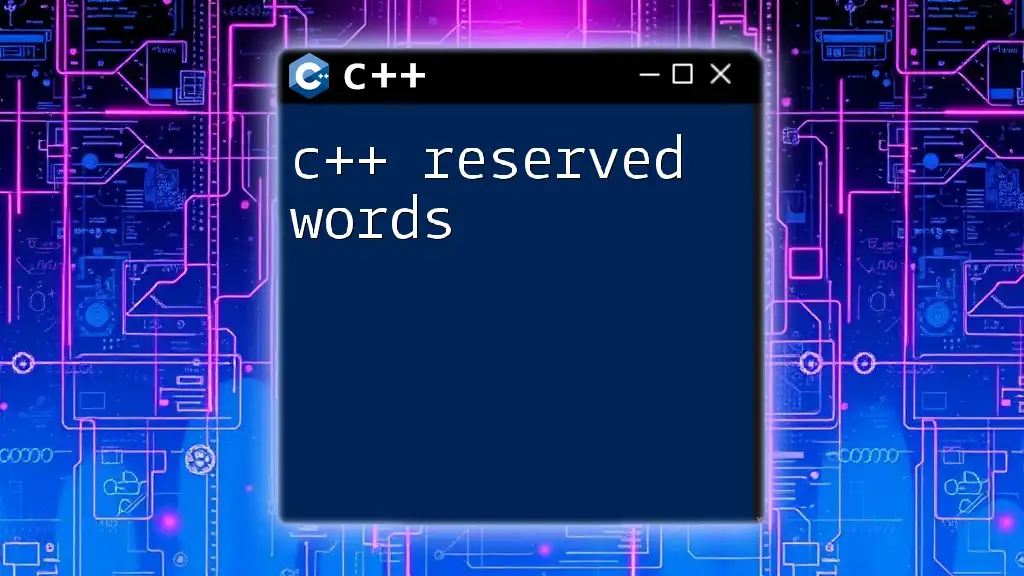
Best Practices for Using the Override Keyword
When implementing the c++ override keyword, consider these best practices:
- Always use `override` when a derived function implements a virtual function. This serves as documentation for other developers and aids in code maintenance.
- Maintain consistent naming conventions across base and derived classes to minimize confusion.
- Use the `final` keyword in conjunction with `override` when you want to restrict further inheritance of the derived class.
Here's an example demonstrating how to combine overriding with the `final` keyword:
class Base {
public:
virtual void process() {
// Implementation
}
};
class Derived final : public Base { // 'final' restricts further inheritance
public:
void process() override {
// Implementation
}
};
In this scenario, `Derived` cannot be inherited further, ensuring that the overridden method's implementation remains intact and secure from changes in derived classes.
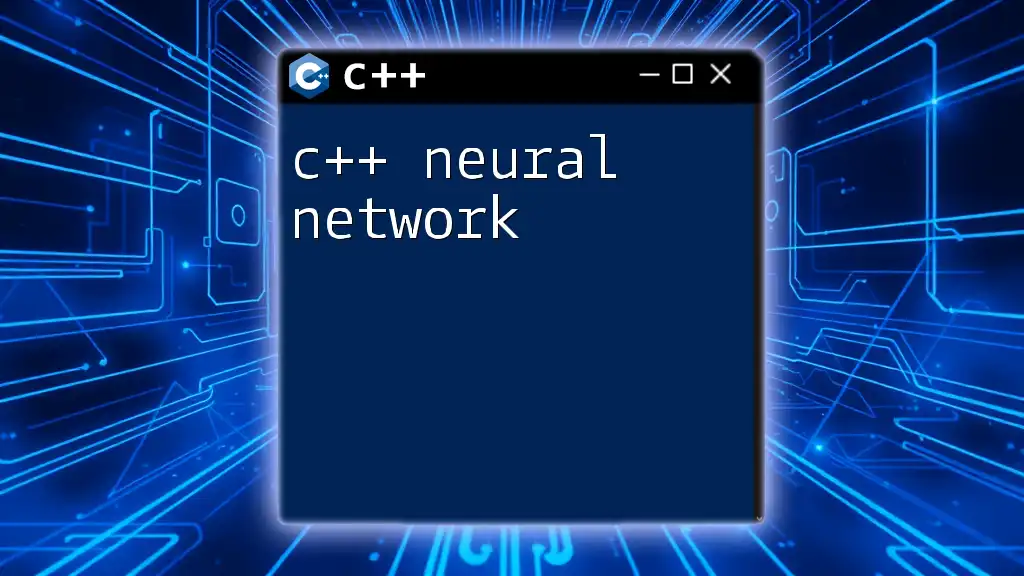
Conclusion
In summary, the c++ override keyword is an indispensable tool for developers working with inheritance and polymorphism in C++. It provides a clear way to indicate method overriding, ensuring that the intended method behavior is maintained while improving code clarity and safety. As you continue to implement OOP principles in your C++ applications, embracing the use of the `override` keyword will greatly enhance both the reliability and maintainability of your code. Remember to review your implementation frequently and leverage resources available to deepen your understanding. Happy coding!
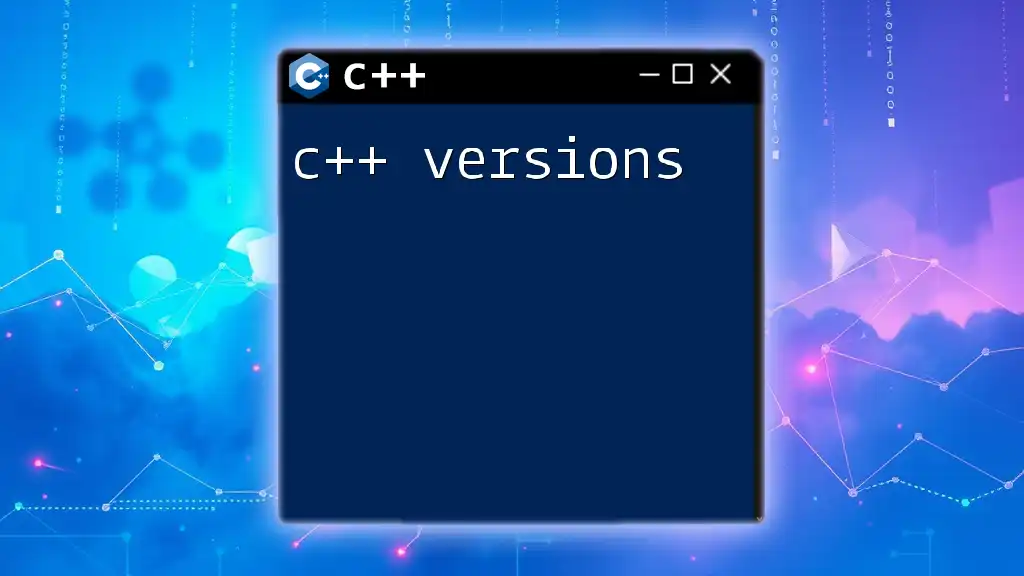
Further Reading
For those eager to expand their knowledge, several resources can assist in your journey with C++ and OOP:
- The official C++ documentation
- Books on advanced C++ programming and design patterns
- Online forums and communities dedicated to C++ development
Engaging with these resources will help you hone your skills and keep up with best practices in the industry.