C++ does not have a built-in garbage collector like some other languages, but it provides mechanisms for manual memory management using operators like `new` and `delete`.
Here's a simple example of manual memory management in C++:
#include <iostream>
int main() {
int* ptr = new int; // Allocating memory
*ptr = 42; // Assigning value
std::cout << *ptr << std::endl; // Outputting value
delete ptr; // Deallocating memory
return 0;
}
Understanding Memory Management in C++
What is Memory Management?
Memory management is a crucial aspect of programming that ensures efficient utilization of computer memory. It involves both allocating memory for storing data as a program runs and deallocating that memory once it is no longer needed. Understanding the difference between dynamic and static memory allocation is essential:
- Static Memory Allocation: This occurs at compile time, where fixed-size variables are allocated in memory, such as global and local variables within functions.
- Dynamic Memory Allocation: This happens at runtime, allowing more flexibility. Memory is allocated on the heap using pointers and can be resized as the program executes.
Manual Memory Management in C++
In C++, memory management is primarily manual, placing the responsibility on the programmer. This means developers must explicitly allocate and deallocate memory using pointers.
To illustrate, consider the following example:
int* array = new int[10]; // Allocate memory for an array of 10 integers
delete[] array; // Deallocate memory when done
While this gives developers precise control over memory usage, it comes with responsibilities. Improper handling can lead to memory leaks, where allocated memory is never freed, thus wasting resources.
Advantages and Disadvantages of Manual Memory Management
Advantages:
- High performance and efficiency due to direct control.
- Lower overhead compared to automated methods like garbage collection.
Disadvantages:
- Increased complexity in code.
- Higher risk of errors, including memory leaks and dangling pointers.
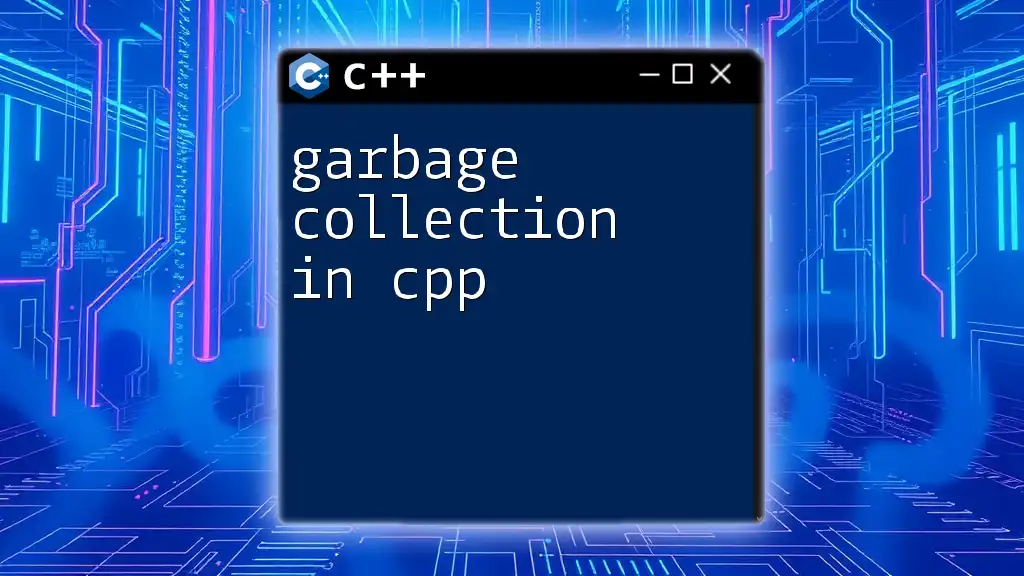
Garbage Collection: A Quick Overview
What is Garbage Collection?
Garbage collection is an automatic memory management feature that identifies and reclaims memory occupied by objects that are no longer in use. This process helps prevent memory leaks and makes programming easier, particularly in high-level languages.
Languages with Built-In Garbage Collection
Many modern programming languages, such as Java and Python, come equipped with integrated garbage collectors. These languages allow programmers to focus more on the logic of their applications instead of the nitty-gritty details of memory management.
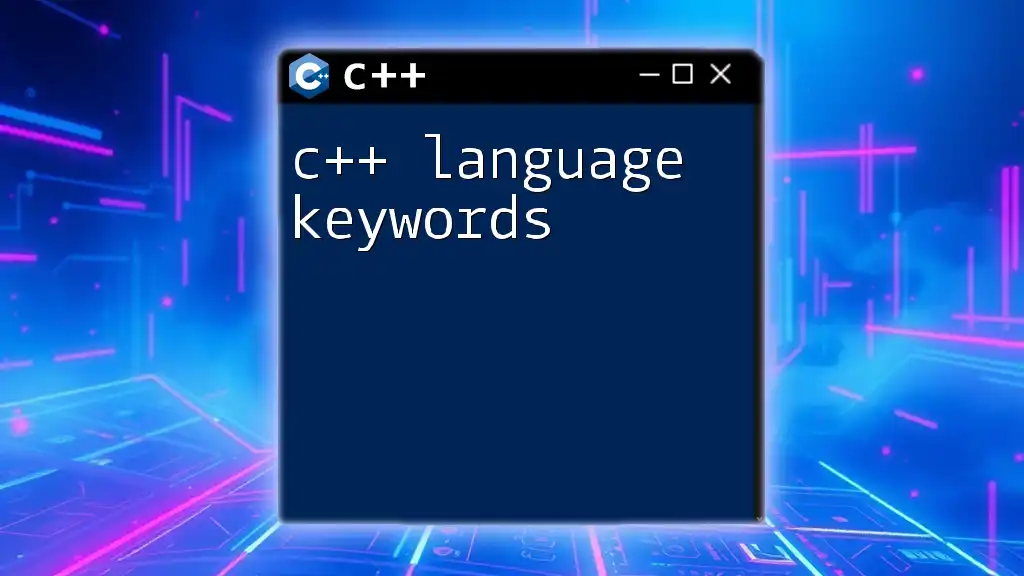
Does C++ Have Garbage Collection?
Core Philosophy of C++
The design philosophy of C++ emphasizes performance and control. C++ was intended to be a systems programming language that is efficient and close to hardware. Consequently, it offers no built-in garbage collector.
Native Garbage Collection in C++
To answer the question: Does C++ have garbage collection? The straightforward answer is no. Unlike languages with built-in garbage collectors, C++ relies on developers to manage their memory effectively and manually.
Why C++ Lacks Built-In Garbage Collection
The primary reason for the absence of garbage collection in C++ is the language's focus on performance. Automatic garbage collection introduces overhead that can slow down programs. C++ permits developers to fine-tune their applications, allowing for high performance suitable for resource-intensive applications, drivers, and systems programming.
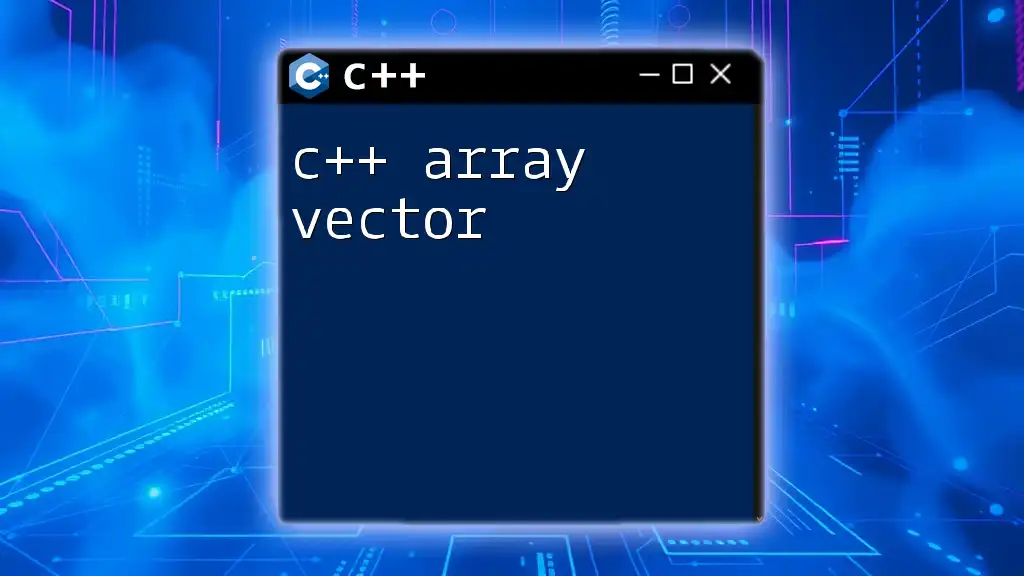
Alternatives to Garbage Collection in C++
Smart Pointers in C++
C++ offers smart pointers that provide a way to handle dynamic memory more safely and conveniently than raw pointers. There are several types of smart pointers available in C++:
- `std::unique_ptr`: Represents exclusive ownership of a resource, automatically deleting the resource when the owning pointer goes out of scope.
- `std::shared_ptr`: Allows shared ownership of resources. The memory will be freed once all `shared_ptr` instances owning it are out of scope.
- `std::weak_ptr`: Provides a non-owning reference to a resource managed by a `shared_ptr`, which is helpful in breaking circular references.
Consider the following example of using `std::unique_ptr`:
#include <memory>
void createUnique() {
std::unique_ptr<int> p1(new int(5)); // Owns the memory, automatically deleted
// No explicit delete call needed
}
Smart pointers significantly reduce the risk of memory leaks and dangling pointers while improving code safety and readability.
RAII (Resource Acquisition Is Initialization)
RAII is a programming idiom whereby resources are tied to the lifetime of objects. In C++, when objects go out of scope, their destructors are called, automatically releasing resources.
This principle helps manage memory safely. For example:
class Resource {
public:
Resource() { /* Acquire resource */ }
~Resource() { /* Release resource */ }
};
In this case, when an object of `Resource` is destroyed, its destructor handles memory cleanup automatically.
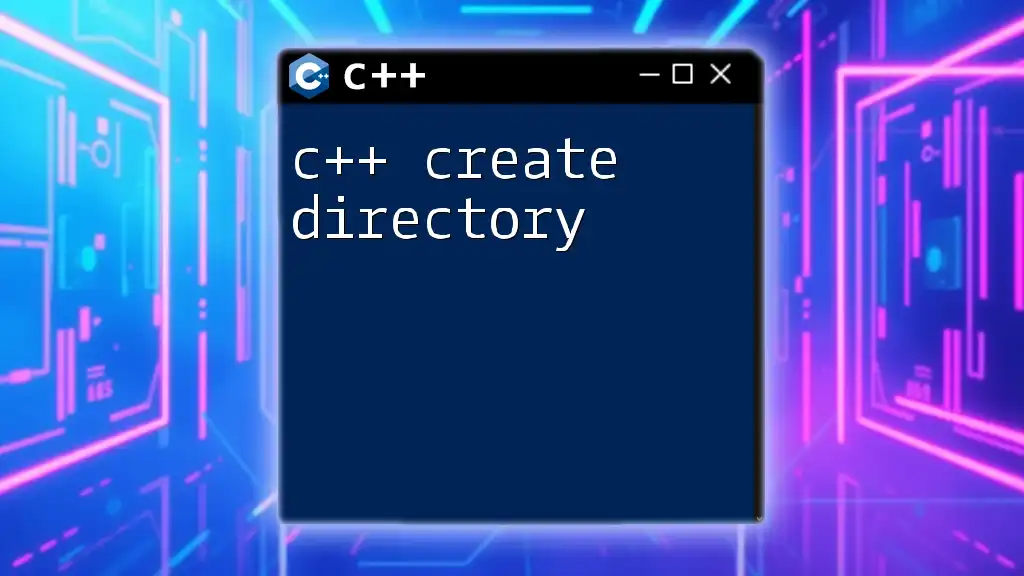
Best Practices for Memory Management in C++
Avoiding Memory Leaks
Memory leaks can severely affect applications, particularly in long-running processes. Common causes of memory leaks include:
- Allocating memory without subsequent deallocation.
- Losing track of pointers due to incorrect managing of object lifetimes.
To avoid these issues, consistently use smart pointers and ensure proper ownership semantics in your code.
When to Use Smart Pointers
The use of smart pointers should be guided by specific considerations:
- Use `std::unique_ptr` when a single ownership model suffices.
- Switch to `std::shared_ptr` when multiple owners of the same object are needed, but ensure to avoid circular references by incorporating `std::weak_ptr`.
Understanding and leveraging smart pointers can lead to more robust, efficient memory management techniques in C++.
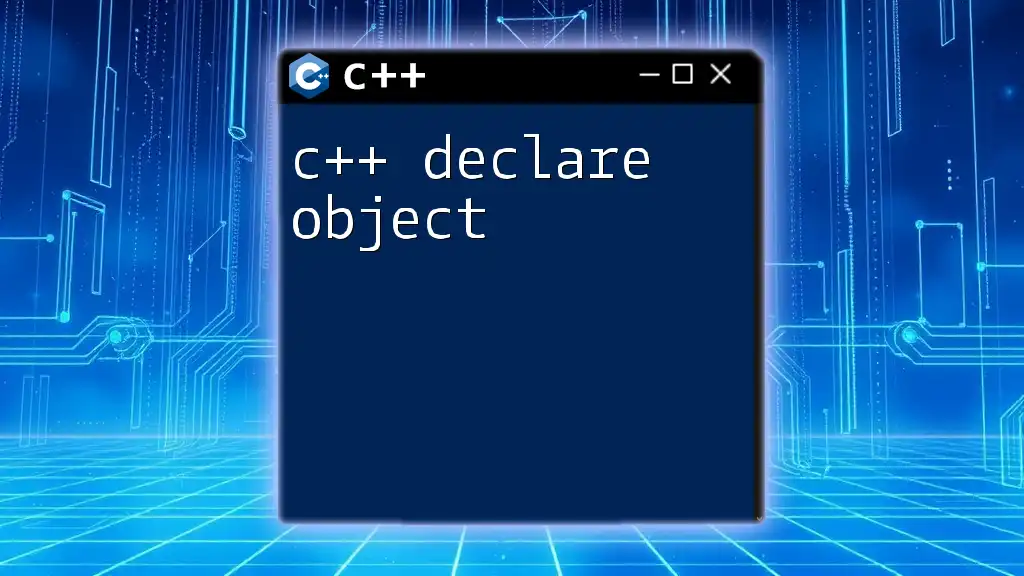
Conclusion
Recap of Key Points
In summary, C++ does not have a built-in garbage collector. Instead, it relies on manual memory management techniques that, while powerful, carry a higher risk of error. Smart pointers and RAII are valuable features in C++ that can help developers manage memory more safely without sacrificing performance.
Further Learning Resources
For those interested in deepening their understanding of C++ memory management, consider exploring recommended books and online resources dedicated to C++ programming. Topics may include advanced techniques and best practices for memory handling, making it easier for developers to navigate the complexities of C++ memory management confidently.
Call to Action
Engage in practical exercises with dynamic memory to sharpen your skills in C++. Share your experiences and questions related to memory management in C++ within your network or community. Enhancing your understanding of this critical topic can lead to significantly improved code quality and performance.