In C++, the error "does not name a type" typically occurs when the compiler encounters an identifier that it cannot recognize as a valid type in the current context, often due to missing declarations or incorrect syntax.
Here's a code snippet illustrating the error:
#include <iostream>
class MyClass {
public:
void display();
};
void MyClass::display() {
std::cout << "Hello World!" << std::endl;
}
int main() {
MyClass obj; // Correctly named type
obj.display();
// Uncomment the line below to observe the error "does not name a type"
// MyClass obj2;
// obj2.display; // Error: does not name a type
return 0;
}
In this snippet, if `obj2.display;` is uncommented, it will produce the error because it is missing parentheses to call the function.
Understanding the "Does Not Name a Type" Error
What Does It Mean?
The error message "does not name a type in C++" indicates that the compiler has encountered a reference to a type that is either not defined or not visible in the current scope. In C++, each variable, function, or object must be associated with a type, which the compiler must recognize to perform type checks and allocate memory appropriately. If the type is incomplete or missing, the compiler raises this particular error.
Common Causes
Incomplete Type Declarations
One of the most frequent reasons for receiving this error is when a type declaration is incomplete. When you declare a structure or class without providing a complete definition, and then attempt to instantiate or use it, the compiler will be unable to recognize it as a valid type.
Consider the following example:
struct Example;
Example e; // This will produce an error
In this case, the compiler sees the forward declaration of `struct Example` but lacks the full definition, leading to an error when trying to instantiate `Example e`. To fix this, you must provide the complete definition before creating instances.
Missing Header Files
Another common issue arises when the type you're trying to use is defined in another header file that hasn't been included in your source file. C++ relies on header files for declarations. If you attempt to use a type declared in a header file that has not been included, the compiler will raise the "does not name a type" error.
For instance:
class MyClass; // Forward declaration
MyClass obj; // Error if class definition is not included
In this example, without the actual class definition of `MyClass`, trying to declare an object of that type will result in an error. To resolve this, ensure that the header file containing `MyClass` is included at the top of your source file:
#include "MyClass.h"
Namespace Issues
Namespaces are crucial in avoiding name collisions, but they can also lead to the "does not name a type" error if not used properly. When a type is declared within a namespace, it must be fully qualified when referenced unless you are using a `using` directive.
Consider the code:
namespace Example {
class MyClass {};
}
Example::MyClass obj; // Works
MyClass obj2; // Error: does not name a type
In this scenario, the first line correctly refers to `MyClass` because it specifies the namespace. In contrast, `MyClass obj2;` produces an error since the compiler cannot find `MyClass` in the global scope. To avoid this error, always reference types with their full namespace or use a `using Example::MyClass;` directive.
Typedefs and Type Aliases
Using `typedef` and `using` to create type aliases can also lead to this error if not handled correctly. If a typedef is defined incorrectly or is missing when referenced, the compiler will throw an error.
Example:
typedef int Integer;
Integer var; // Correct
Typedef Integer; // Incorrect: does not name a type
In the second line, using `Typedef` instead of `typedef` will result in an error since the compiler does not recognize it. Always ensure the correct keyword is used when creating type aliases.
Less Common Causes
Macros and Preprocessor Directives
Macros can sometimes lead to confusion and compilation errors, including "does not name a type." If you define a type through a macro and later remove or modify that definition, you could inadvertently cause this error.
For instance:
#define MY_TYPE int
MY_TYPE var; // Correct
#define MY_TYPE // Removing type declaration
MY_TYPE var; // Error: does not name a type
Here, if the macro `MY_TYPE` is undefined or removed, any usage of it following the preprocessor directive will cause the compiler to fail to recognize it as a type. It’s advisable to use macros sparingly and maintain clarity in their definitions to avoid such errors.
Template Issues
Templates bring complexity into how types are declared and defined. If you declare a template without fully defining it, the compiler may throw the "does not name a type" error.
For instance:
template<typename T>
class MyClass;
MyClass<int> obj; // Error: does not name a type
In this case, the attempt to instantiate `MyClass<int>` fails because, while `MyClass` is declared as a template, it lacks a definition. To resolve this, you must provide a full template definition:
template<typename T>
class MyClass {
public:
T value;
};
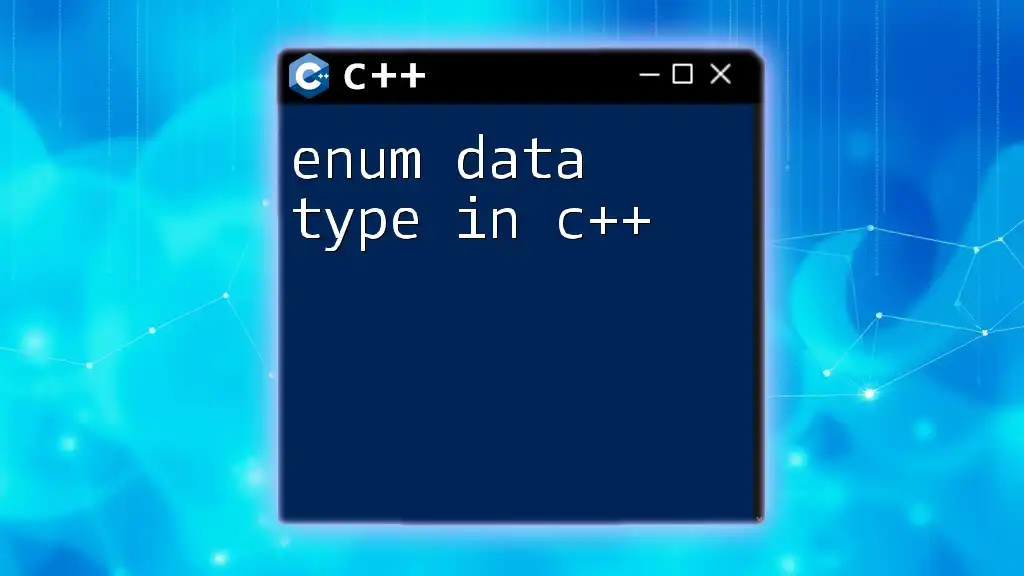
Diagnosing the Error
Reading Compiler Errors
The first step in fixing the "does not name a type" error is to carefully read the compiler's error message. Compiler messages typically provide information about where the error occurred and the nature of the issue. Pay attention to the line number and any additional descriptions that accompany the error, as they will guide you to the root cause.
Debugging Techniques
When troubleshooting "does not name a type" errors, it’s essential to isolate the offending line of code. Start by commenting out sections of the code to determine where the issue arises. If possible, build a small, self-contained example that reproduces the error. This will help clarify the problem and allow you to test potential fixes without the noise of unrelated code.
Using tools such as integrated development environments (IDEs) can also assist significantly. Many modern IDEs provide features like error highlighting and code suggestions, which can help pinpoint the exact cause of the issue.
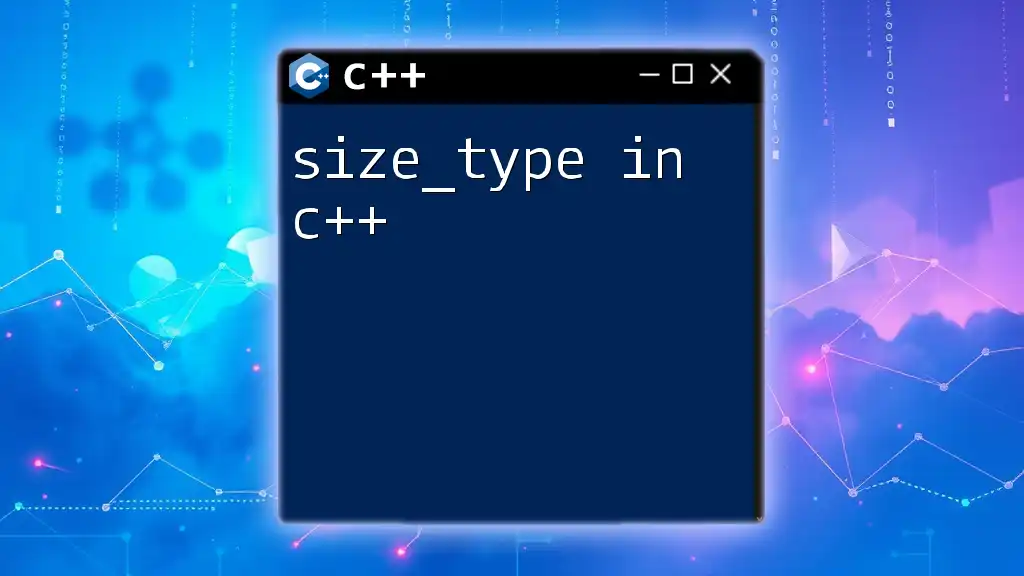
Best Practices to Avoid This Error
Declare Types Clearly
One of the best ways to prevent the "does not name a type" error is to be meticulous about type declarations and definitions. Always ensure that types are completely defined before they are used. This includes checking that all header files are properly included and that visibility rules are adhered to.
Consistent Use of Namespaces
When working with namespaces, maintain a consistent and clear structure. Avoid declaring types in the global namespace while defining others in a specific namespace. Instead, use fully qualified names or `using` directives to make code more readable and to avoid ambiguity.
Keep Header Files Organized
Organizing header files effectively is crucial for reducing the risk of visibility issues that lead to compilation errors. Follow a conventional structure whereby types are declared in separate headers, and ensure that those headers are included whenever those types are required.
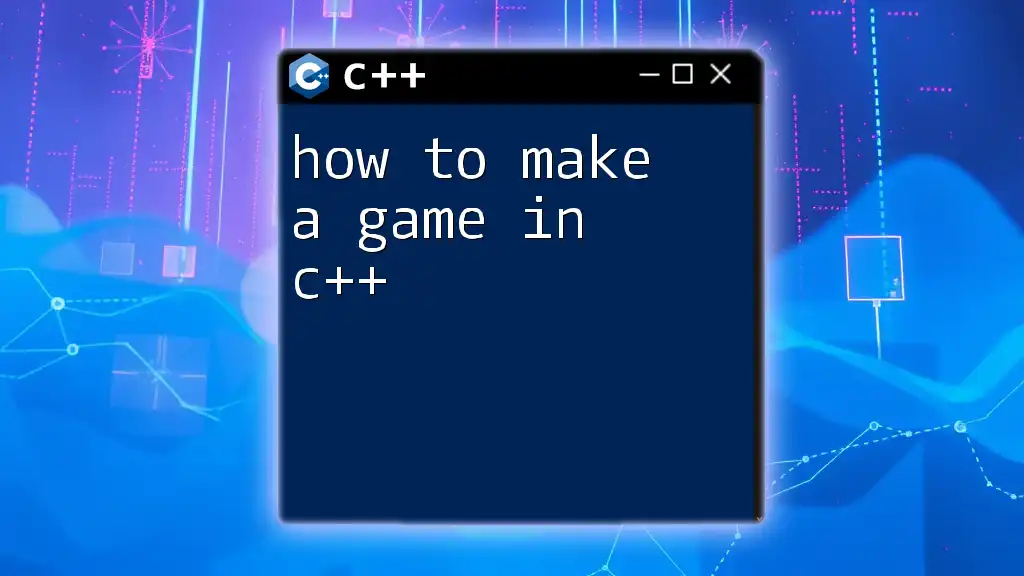
Conclusion
The error "does not name a type in C++" can be frustrating, especially for those new to the language. However, by understanding its causes—ranging from incomplete type definitions to namespace-related issues—you can effectively troubleshoot and resolve it. Remember to utilize thorough debugging techniques, maintain best practices in coding, and keep learning to improve your C++ skills. Each error encountered is an opportunity to deepen your understanding of this powerful language.
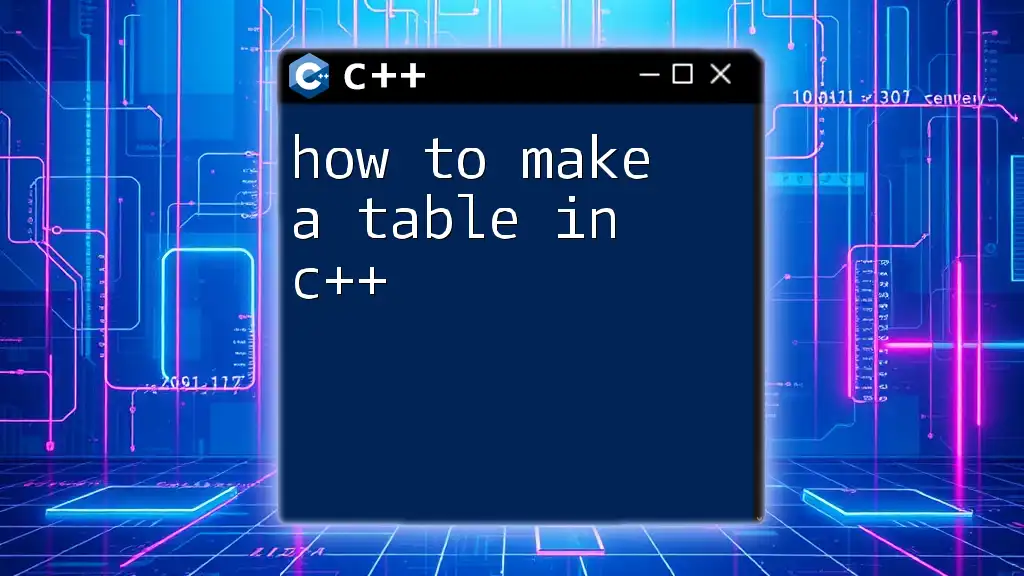
Frequently Asked Questions
What Should I Do If I Encounter This Error?
If you encounter the "does not name a type" error, begin by reviewing the error message closely to locate the specific line of code causing the issue. Check for any missing type definitions, included headers, or namespace discrepancies. If necessary, simplify your code to isolate the problem, and utilize IDE features to assist in identifying the root cause.
Can This Error Occur in Other Languages?
The challenge of type visibility and declaration is common in many programming languages, though the exact error message may differ. Languages like Java, C#, and others have their own specific type-related errors that arise from similar issues of incomplete declarations or missing imports. Understanding C++ can provide valuable insights into type systems in other languages.
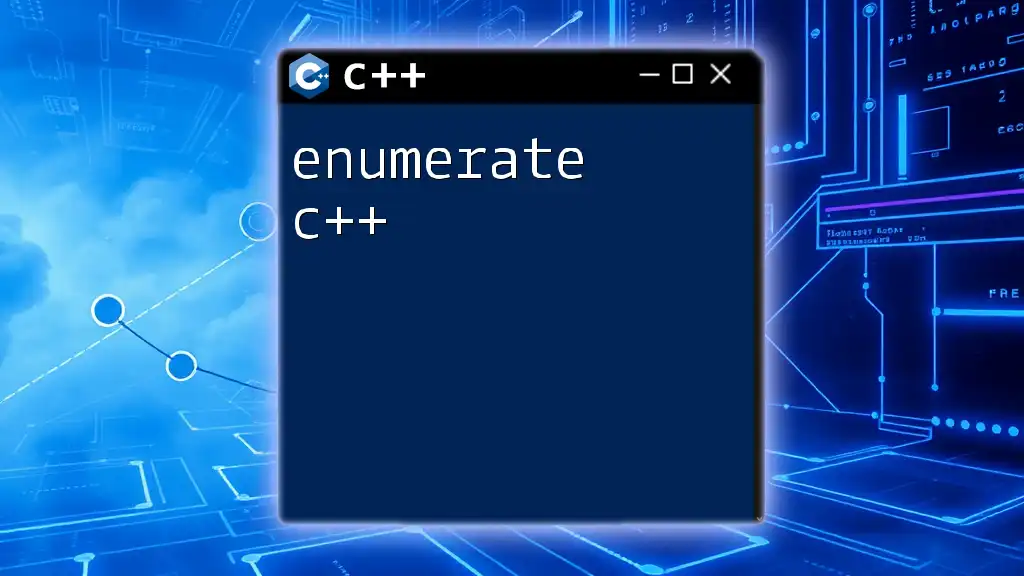
Additional Resources
For those wanting to deepen their knowledge of C++ types and compilation errors, various resources are available, such as official documentation, online tutorials, and community forums dedicated to programming challenges. Always take the time to explore these avenues for expanded learning and support.