C++ can be challenging to learn due to its complex syntax and concepts like pointers and memory management, but with the right resources and practice, anyone can master it.
Here’s a simple example demonstrating the basic structure of a C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++
What is C++?
C++ is a powerful, high-level programming language that has stood the test of time. Developed by Bjarne Stroustrup at Bell Labs in the early 1980s, C++ is an extension of the C programming language and supports object-oriented programming (OOP). This makes it a versatile tool for software developers, allowing them to create complex systems, applications, and games. With its blend of performance and abstraction, C++ remains particularly relevant in industries such as gaming, finance, and systems programming.
Key Features of C++
C++ is distinguished by several key features that contribute to its capabilities:
-
Object-Oriented Programming: The essence of C++ lies in its object-oriented nature, which encourages encapsulation, inheritance, and polymorphism. Understanding these concepts is crucial for effective C++ programming.
-
Low-Level Manipulation: C++ provides direct manipulation of hardware via pointers, which offers significant control over system resources. This can be both a powerful tool and a source of complexity for newcomers.
-
Standard Template Library (STL): The STL offers a collection of pre-built templates (data structures and algorithms) that greatly facilitate the coding process. Utilizing the STL can lead to more efficient and less error-prone code.
Here's a simple example of creating and using a class in C++:
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
int main() {
Dog myDog;
myDog.bark(); // Outputs: Woof!
return 0;
}
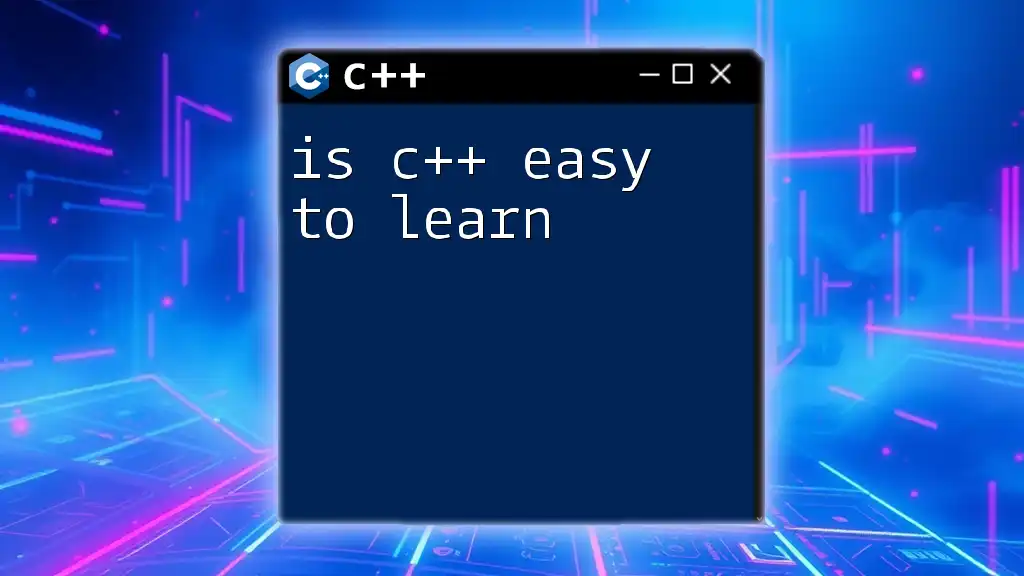
Factors Affecting Learning Difficulty
Prior Programming Experience
If you have prior experience in programming languages such as Python or Java, you may find it easier to grasp C++. Understanding programming fundamentals—variables, loops, and conditionals—can create a strong foundation. For instance, many of the concepts in C++, like conditional statements, are similar to those in other languages:
if (condition) {
std::cout << "Condition is met." << std::endl;
} else {
std::cout << "Condition is not met." << std::endl;
}
Complexity of Syntax
C++ syntax can initially appear daunting compared to higher-level languages. The rigorous syntax rules, such as the need for semicolons, proper brace matching, and case sensitivity, can be overwhelming for beginners. For instance, a simple "Hello, World!" program in C++ contrasts with Python's simplicity:
C++ version:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Python version:
print("Hello, World!")
Conceptual Load
C++ introduces several advanced concepts, such as pointers and memory management, that can pose challenges for beginners. Pointers, in particular, introduce a level of complexity that requires a deep understanding of how data is stored in memory. Here’s an example highlighting the difference between a pointer and a reference in C++:
void updateValue(int* p) {
*p = 10; // Changing the value at the address pointed by p
}
int main() {
int x = 5;
updateValue(&x); // Passing the address of x
// Now x is 10
return 0;
}
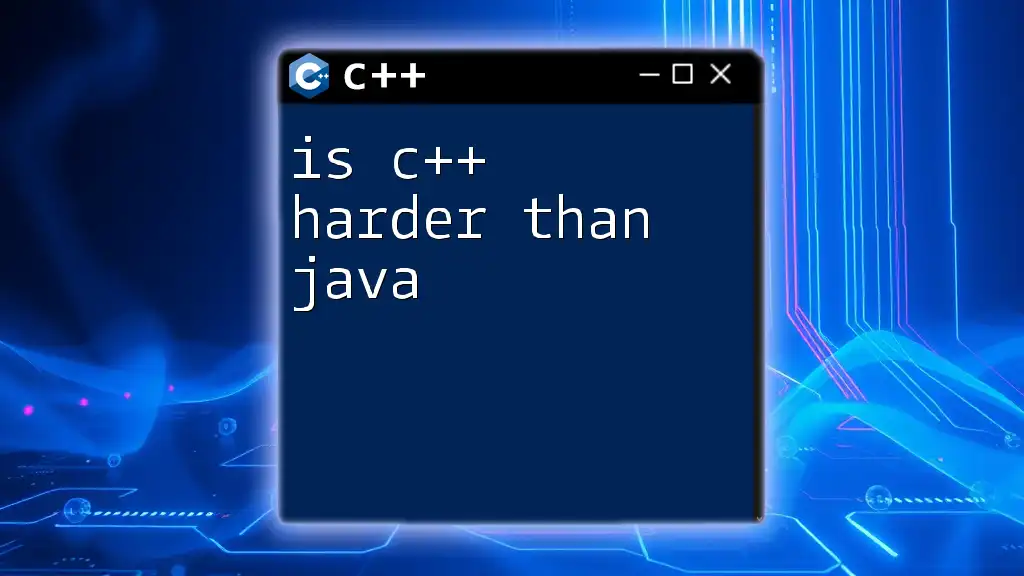
The Learning Curve
Getting Started: Initial Steps
Starting your C++ journey requires a solid foundation. First, choose your learning resources wisely. Recommended options include:
- Books: Titles like "C++ Primer" and "Effective C++" are excellent starting points.
- Online Courses: Websites such as Coursera, Udemy, and Codecademy offer structured courses.
- Video Tutorials: YouTube channels provide engaging, visual explanations of concepts.
Moreover, setting up a development environment is crucial. Install a reliable IDE (such as Visual Studio, Code::Blocks, or CLion) and a compiler (like GCC). Familiarize yourself with these tools, as they will become your workspace.
Building Foundational Skills
Focusing on core principles is vital for gaining confidence in C++. Practice makes perfect—regular coding exercises and projects help solidify your understanding. Start with basics, such as:
- Variables and data types
- Control structures: loops (for, while) and conditionals (if-else)
- Functions: how to declare and define them
Transitioning to Advanced Topics
Once you are comfortable with foundational concepts, gradually delve into advanced topics. This includes understanding pointers, templates, and the STL. Here are some strategies to consider:
- Break down complex concepts into smaller, digestible parts.
- Practice coding challenges related to pointers, such as implementing dynamic arrays or linked lists.
- Engage with resources that focus on these advanced topics specifically.
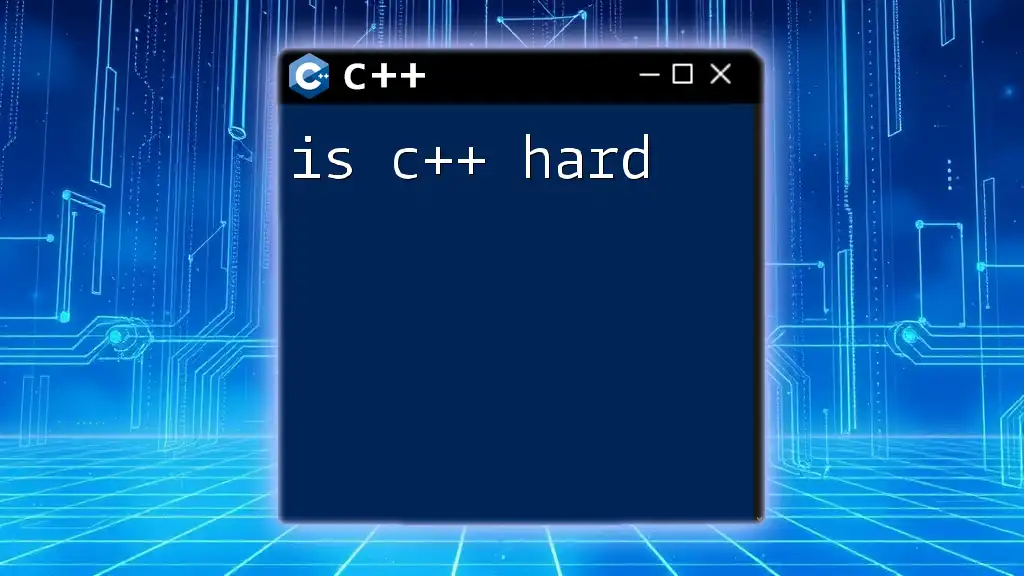
Common Misconceptions
“C++ is Just for Advanced Programmers”
Many believe C++ is accessible only to those with extensive programming experience. This is a misconception. With the right resources and guidance, beginners can learn C++. Taking it one step at a time, a motivated individual can start writing basic C++ programs and progressively tackle more advanced topics.
"C++ is Too Complex for Modern Development"
Some argue that C++ is no longer relevant in the modern programming landscape. However, C++ excels in performance-intensive applications where speed and memory management are crucial, such as game development and systems programming. Recognizing C++'s strengths and weaknesses in context can help beginners appreciate its importance.
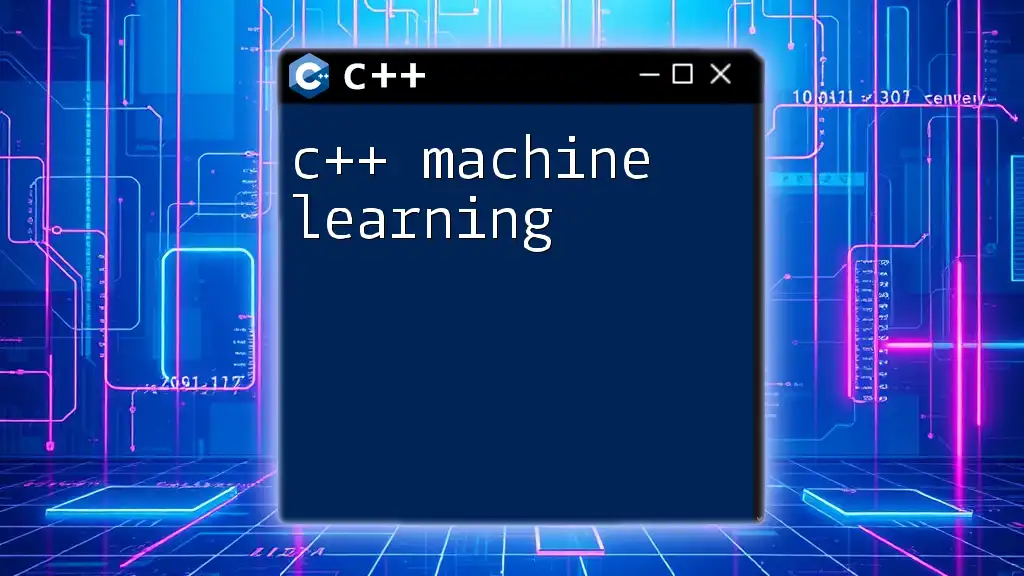
Tips for Learning C++
Practice Makes Perfect
Consistency is key. Engage in regular coding challenges on platforms like LeetCode or HackerRank. These challenges will not only reinforce your skills but also expose you to diverse problems encountered in real-world programming.
Engage with the Community
Join C++ forums, discussion groups, or local meetups to connect with fellow learners and experienced developers. Engaging in pair programming can provide valuable insights and support as you navigate your learning journey.
Continuous Learning
C++ is constantly evolving, with new standards being introduced (e.g., C++11, C++14, C++17). To stay ahead, familiarize yourself with the new features added in each iteration. Embrace a mindset of continuous learning by following blogs, attending webinars, and enrolling in advanced courses after mastering the basics.
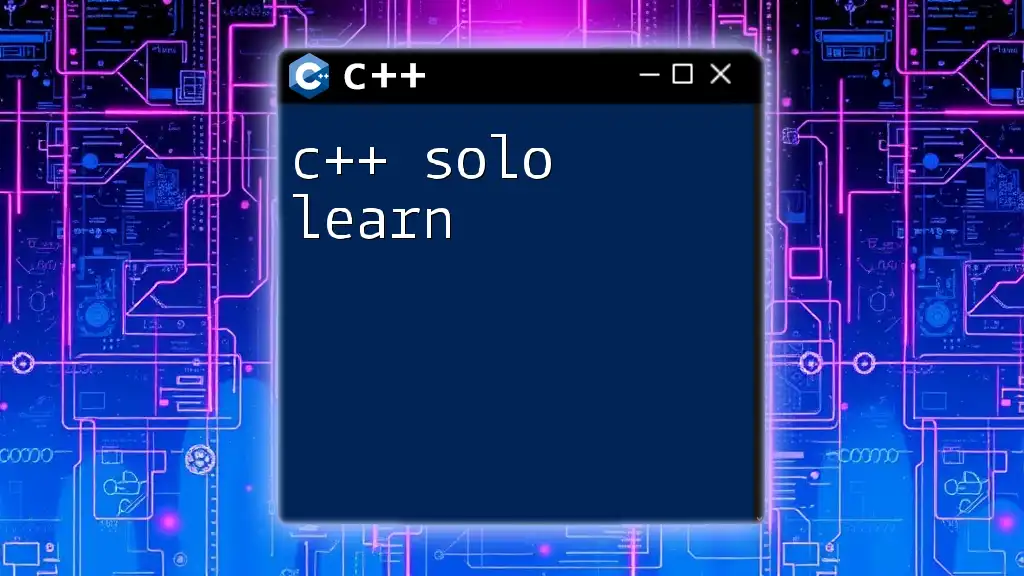
Conclusion
In summary, is C++ hard to learn? The answer depends on various factors, including your prior experience, your approach to learning, and your willingness to engage with the complexities of the language. While C++ can present challenges, it is a highly learnable language for dedicated individuals. Remember, every expert was once a beginner. Embrace the journey of learning C++, and soon enough, you will find yourself applying your knowledge in practical situations.
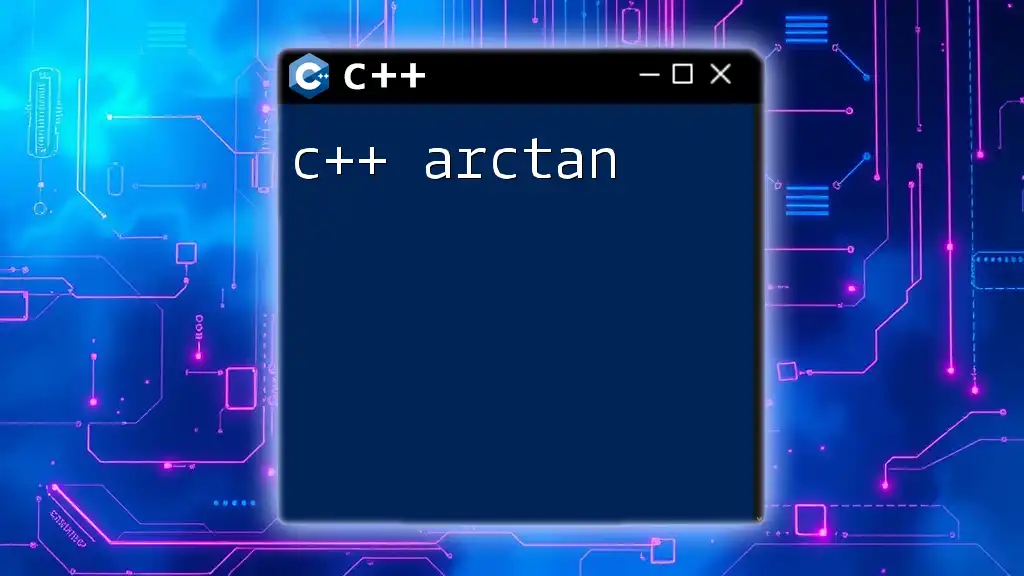
Call to Action
Are you ready to take your programming skills to the next level? Sign up for our C++ coaching sessions today to receive personalized guidance and resources tailored to help you succeed in learning C++. Check out our additional resources and blog posts for more in-depth insights into C++.
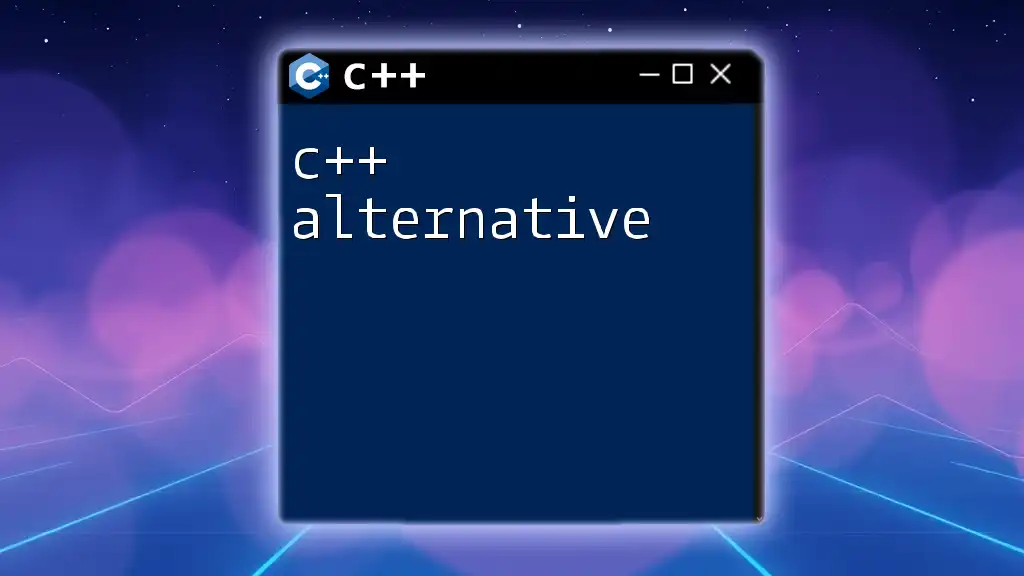
FAQs About C++
Is C++ worth learning in 2023?
Yes, learning C++ is highly beneficial due to its widespread use in high-performance applications and game development.
How long does it take to learn C++?
The time required can vary; however, with consistent effort, beginners can build a solid foundation in a few months.
What jobs can I get by learning C++?
Jobs in software development, game programming, and systems analysis are just a few areas where C++ skills are highly sought after.