C++ can be considered harder than Java due to its complex syntax, manual memory management, and the need for a deeper understanding of low-level programming concepts.
Here’s a simple code snippet demonstrating a basic "Hello, World!" program in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ and Java
What is C++?
C++ is a general-purpose programming language developed by Bjarne Stroustrup starting in 1979 at Bell Labs. It is an extension of the C programming language and includes both high-level and low-level features, allowing for both efficient resource management and complex programming constructs. C++ supports several programming paradigms, primarily object-oriented programming (OOP), but also procedural and generic programming.
A simple "Hello, World!" program in C++ looks like this:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
What is Java?
Java, developed by Sun Microsystems and released in 1995, is a versatile, object-oriented programming language designed to be platform-independent, allowing developers to write code that runs on any device that supports the Java Virtual Machine (JVM). One of its most significant features is its robust garbage collection, which automatically manages memory, relieving developers from the need to handle memory manually.
Here’s a simple "Hello, World!" program in Java:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}

Comparing C++ and Java
Syntax Differences
The syntax differences between C++ and Java are quite stark, especially for newcomers. While both languages share C-like syntax, the way they express concepts can vary significantly.
When it comes to basic constructs such as variables, loops, and conditionals, one can see clear distinctions. For instance, here’s a loop written in both languages:
// C++ syntax for a loop
for(int i = 0; i < 10; i++) {
cout << i << endl;
}
// Java syntax for a loop
for(int i = 0; i < 10; i++) {
System.out.println(i);
}
Although the loop structures appear similar, Java's reliance on objects can complicate the syntax for more advanced requirements.
Memory Management
Automatic vs Manual Memory Management
Memory management is another area where C++ and Java differ fundamentally. Java employs automatic memory management through garbage collection, meaning that developers do not have to manually allocate and deallocate memory. This can significantly reduce the likelihood of memory leaks and errors.
For example, in the Java program, developers just create objects without worrying about deallocating them:
String greetings = new String("Hello");
In contrast, C++ requires manual memory management, giving developers greater control over resource allocation. While this can lead to improved performance, it also creates challenges, as improper handling can result in memory leaks. Here's how memory allocation works in C++:
int* ptr = new int(10); // Allocates memory for an integer
// Don't forget to deallocate
delete ptr; // Properly frees the allocated memory
Error Handling
Exceptions in Java
Java has a well-structured approach to error handling using exceptions, which allows developers to write cleaner code. By using `try`, `catch`, and `finally`, Java handles exceptions gracefully, allowing for recovery from errors without crashing the application:
try {
// Code that may throw an exception
} catch (ExceptionType e) {
// Handle exception
}
Error Handling in C++
C++ also supports error handling with exceptions, but its approach is not as robust as Java's. Developers can use `try` and `catch`, but the absence of built-in garbage collection means that memory management issues can lead to exceptions causing more significant problems:
try {
// Code that may throw an exception
} catch (const std::exception& e) {
// Handle exception
}

Performance Considerations
Execution Speed
When it comes to performance, C++ typically holds an edge over Java. C++ is a compiled language, translating code directly into machine code, which leads to faster execution times. Java, while faster than some interpreted languages, runs on the JVM, introducing overhead that can slow down execution speed.
Theoretical benchmarks illustrate that C++ outperforms Java in computation-heavy tasks, particularly those requiring low-level system access and resource optimization. The ability to use inline assembly in C++ can also provide performance enhancements that Java cannot achieve.
Resource Efficiency
C++ allows for fine-grained control over system resources, which can result in more efficient memory usage. However, this control comes at a cost; C++ developers must be vigilant about memory management practices to prevent issues.
In contrast, while Java consumes more resources due to its JVM and garbage collection overhead, the trade-off is often justified in terms of productivity and developer ease, particularly in large-scale applications.

Community and Ecosystem
Libraries and Frameworks
Both languages have rich ecosystems with extensive libraries and frameworks:
-
C++ boasts powerful libraries such as Boost and the Standard Template Library (STL), which provide reusable components for various applications, from networking to data structures.
-
Java's ecosystem includes robust frameworks like Spring and Hibernate, which simplify enterprise application development and data management.
Learning Resources
C++ Resources
There are countless resources available for aspiring C++ developers, including:
- Books like C++ Primer by Stanley B. Lippman.
- Online courses on platforms like Coursera and Udemy.
- Community forums such as Stack Overflow and CPlusPlus.com.
Java Resources
Similarly, Java learners have access to numerous resources:
- Books like Effective Java by Joshua Bloch.
- Online courses offered by Coursera, Udacity, and others.
- Java-specific forums and communities like JavaRanch and JavaWorld.
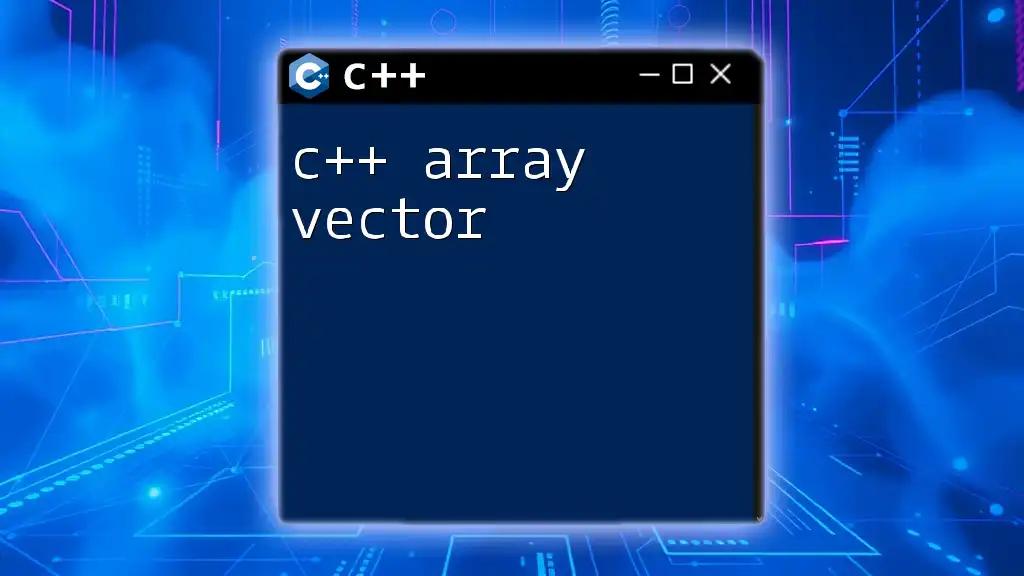
Learning Curve and Developer Experience
Difficulty Level for Beginners
For absolute beginners, Java is often perceived as an easier language to grasp due to its straightforward memory management and focus on object-oriented programming. Learning resources for Java are plentiful, often introducing concepts in a more digestible manner. In contrast, C++'s complexity in memory management and syntax may be daunting for newcomers.
Advanced Features
Templates and STL in C++
C++ offers advanced features such as templates and the Standard Template Library (STL), which allow for powerful generic programming. Here’s a simple template function that adds two numbers:
template <typename T>
T add(T a, T b) {
return a + b;
}
These features showcase C++'s flexibility but also introduce complexity, making mastery more challenging.
Java's Object-Oriented Features
Java excels in its object-oriented features, including inheritance, polymorphism, and interfaces. An example of inheritance in Java illustrates simplicity and clarity:
class Animal {}
class Dog extends Animal {}
While developer experience might be simplified through Java's strong emphasis on OOP, it can also lead to extensive boilerplate code compared to C++.

Conclusion
In addressing the question of "is C++ harder than Java?", it's clear that each language has its strengths and weaknesses. While C++ offers unparalleled control and performance, it also introduces complexity that can be intimidating, especially for beginners. Conversely, Java provides a more accessible learning curve through its clear syntax and automatic memory management, making it a favored choice among new developers wishing to learn object-oriented programming.
Ultimately, the choice between C++ and Java should depend on your specific goals, the type of applications you wish to develop, and your willingness to embrace the complexities of the language. Whether you choose to dive into the depths of C++ or venture into Java's ease of use, each path has its unique rewards.

FAQs
Is it worth learning C++ if I know Java?
Yes, learning C++ after Java can greatly enhance your understanding of memory management, system-level programming, and performance optimization, making you a more versatile developer.
Which language is in more demand in the job market?
Both languages have their niches, but demand can vary by industry. C++ is prevalent in system-level programming, game development, and high-performance applications, while Java is prominent in enterprise applications, web development, and Android app development.
Can I learn both simultaneously?
While learning both languages simultaneously is possible, it may be beneficial to focus on one language at a time to build a strong foundation before exploring another, especially given the differences in paradigms and complexities.