C++ can be utilized to interact with databases through libraries like SQLite or MySQL, enabling developers to perform operations like querying and updating data efficiently.
#include <iostream>
#include <sqlite3.h>
int main() {
sqlite3 *db;
char *errorMessage = 0;
int exit = sqlite3_open("example.db", &db); // Open or create a database
if (exit) {
std::cerr << "Error opening database: " << sqlite3_errmsg(db) << std::endl;
} else {
std::cout << "Opened Database Successfully!" << std::endl;
}
// Closing database
sqlite3_close(db);
return 0;
}
Understanding Database Concepts
What is a Database?
A database is an organized collection of structured information, typically stored electronically in a computer system. Databases provide a way to manage data efficiently and support various applications where data retrieval and storage are crucial.
There are two primary types of databases:
- Relational databases: These databases store data in tables that relate to each other through keys. Examples include MySQL, PostgreSQL, and SQLite.
- Non-relational databases: Also known as NoSQL databases, these store data in formats other than tables, such as documents or key-value pairs. Examples include MongoDB and Redis.
Database Management Systems (DBMS)
A Database Management System (DBMS) is software that interacts with the database, allowing users to create, read, update, and delete data. Popular choices that integrate seamlessly with C++ include MySQL, PostgreSQL, and SQLite. Understanding these systems is vital for effectively using C++ to interact with databases.
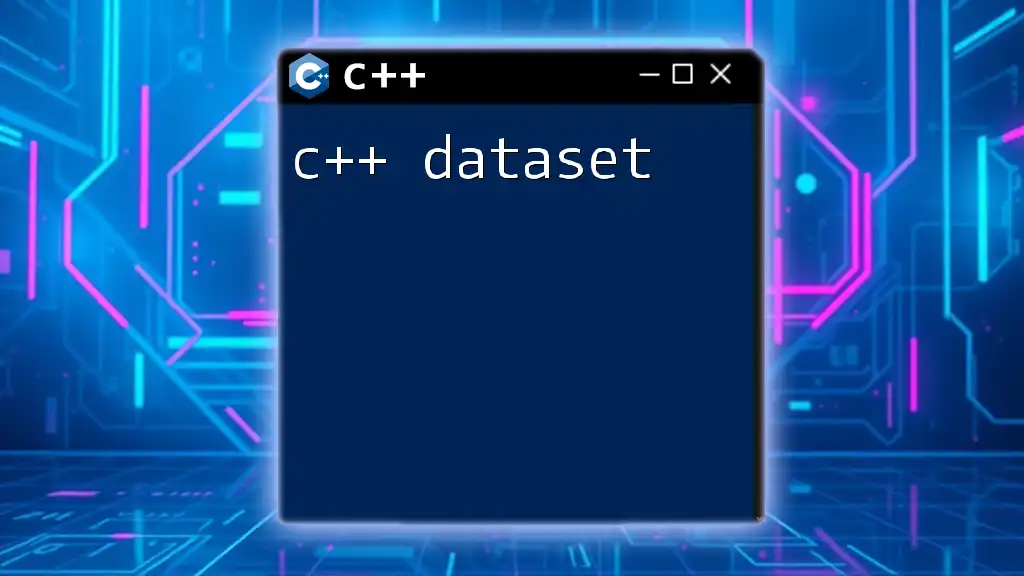
Working with Databases in C++
Choosing a Database for Your C++ Project
The choice of the database depends on various factors such as:
- Project requirements: Does your project need complex queries, many concurrent users, or specific data types?
- Performance: Assess the scalability and speed of the database solution.
- Ease of integration with C++: Some databases come with better-supported libraries than others.
Pros and cons of different databases should be thoroughly evaluated before committing to one.
Setting Up a Development Environment
Installing the Required Libraries
For working with databases in C++, it is essential to have the right libraries. Libraries such as MySQL Connector/C++ and SQLite3 provide robust support for database operations. Their installation typically involves downloading the library and linking it to your C++ project.
Creating a Sample C++ Project
Once the libraries are installed, you can start a new C++ project by setting up your development environment, creating a new source file, and ensuring your compiler can access the installed libraries.
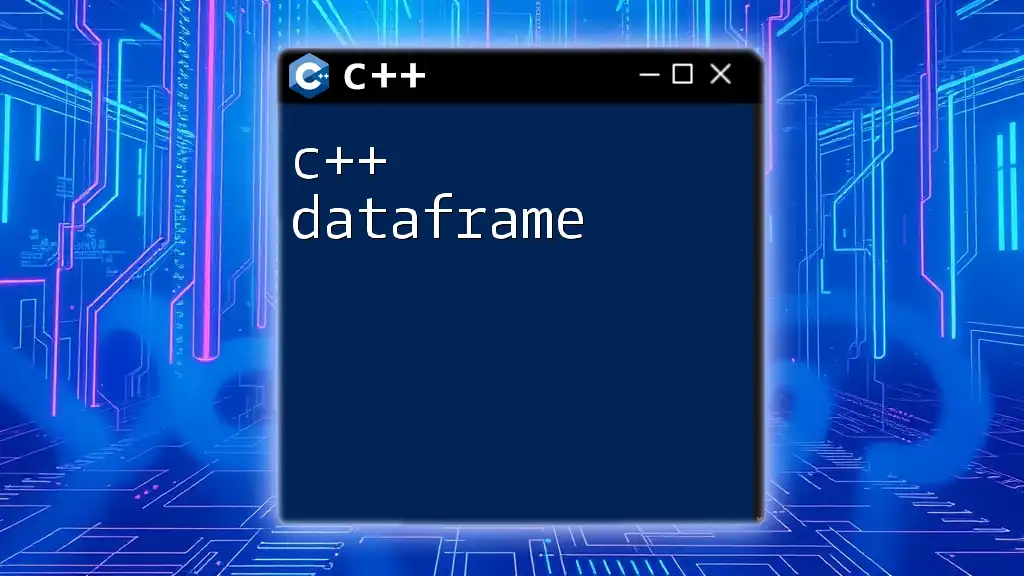
Establishing Database Connections
Connecting to a Database in C++
To interact with a database in C++, you first need to establish a connection:
#include <mysql/mysql.h>
MYSQL *conn;
conn = mysql_init(NULL);
if (conn == NULL) {
fprintf(stderr, "mysql_init() failed\n");
return EXIT_FAILURE;
}
if (mysql_real_connect(conn, "host", "user", "password", "database", 0, NULL, 0) == NULL) {
fprintf(stderr, "mysql_real_connect() failed\n");
mysql_close(conn);
return EXIT_FAILURE;
}
In the above code:
- Replace `"host"`, `"user"`, `"password"`, and `"database"` with your actual database connection details.
- Proper error handling is crucial; ensure you check for `NULL` in both `mysql_init` and `mysql_real_connect` to manage potential failures.
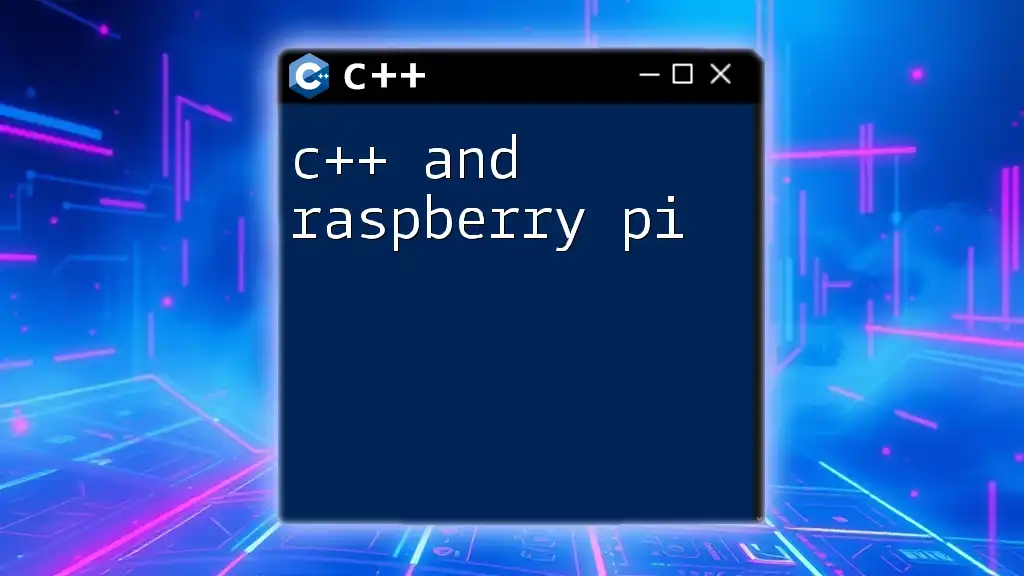
Executing SQL Commands
Basic SQL Commands Overview
SQL is the standard language for managing and manipulating databases. Basic SQL commands include:
- SELECT: Retrieve data from one or more tables.
- INSERT: Add new data into a table.
- UPDATE: Modify existing records.
- DELETE: Remove records from a table.
Executing Queries with C++
To execute an SQL query, use the following code snippet:
const char *query = "SELECT * FROM table";
if (mysql_query(conn, query)) {
fprintf(stderr, "SELECT * failed. Error: %s\n", mysql_error(conn));
return EXIT_FAILURE;
}
This code snippet demonstrates how to send a query to the database and includes error handling that displays an error message if the query fails.
Fetching Data from the Database
Reading Query Results
After executing a SELECT statement, you need to process the returned data. You can use the following code to read query results:
MYSQL_RES *res;
res = mysql_store_result(conn);
MYSQL_ROW row;
while ((row = mysql_fetch_row(res)) != NULL) {
printf("%s\n", row[0]); // Print first column of each row
}
mysql_free_result(res);
This retrieves the results using `mysql_store_result` and then iterates through each row with `mysql_fetch_row`, allowing you to access individual records by indexing.
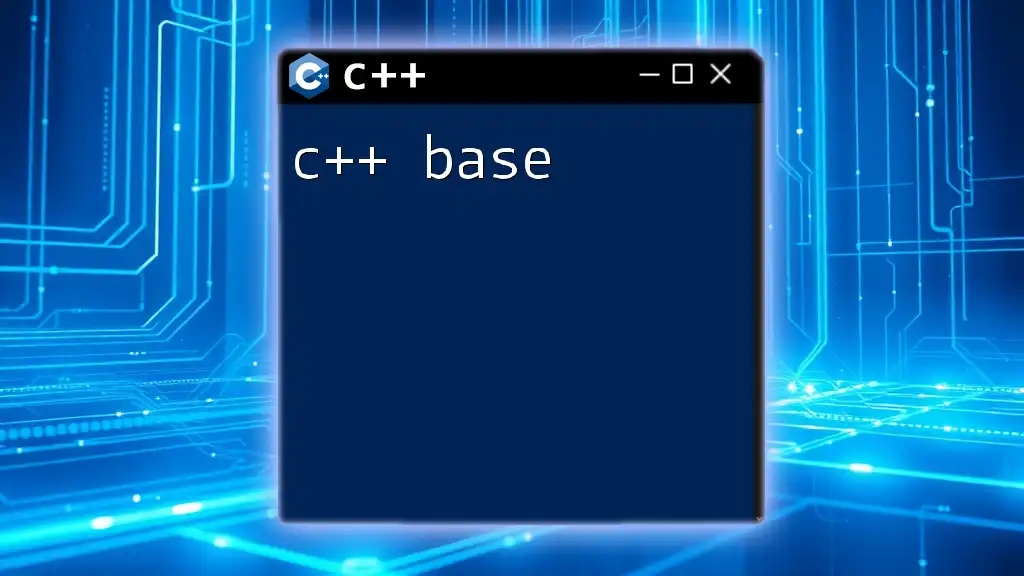
Modifying Data in the Database
Inserting Data into a Database
To insert data into a table, utilize the following example:
const char *query = "INSERT INTO table (column1, column2) VALUES ('value1', 'value2')";
mysql_query(conn, query);
In this snippet, ensure that `table`, `column1`, and `column2` match your database schema. Error checking can be included to ensure the INSERT command executed successfully.
Updating Existing Records
Updating records in a database can be done as follows:
const char *query = "UPDATE table SET column1 = 'newValue' WHERE condition";
mysql_query(conn, query);
Deleting Records
If you need to delete records, do so with this command:
const char *query = "DELETE FROM table WHERE condition";
mysql_query(conn, query);
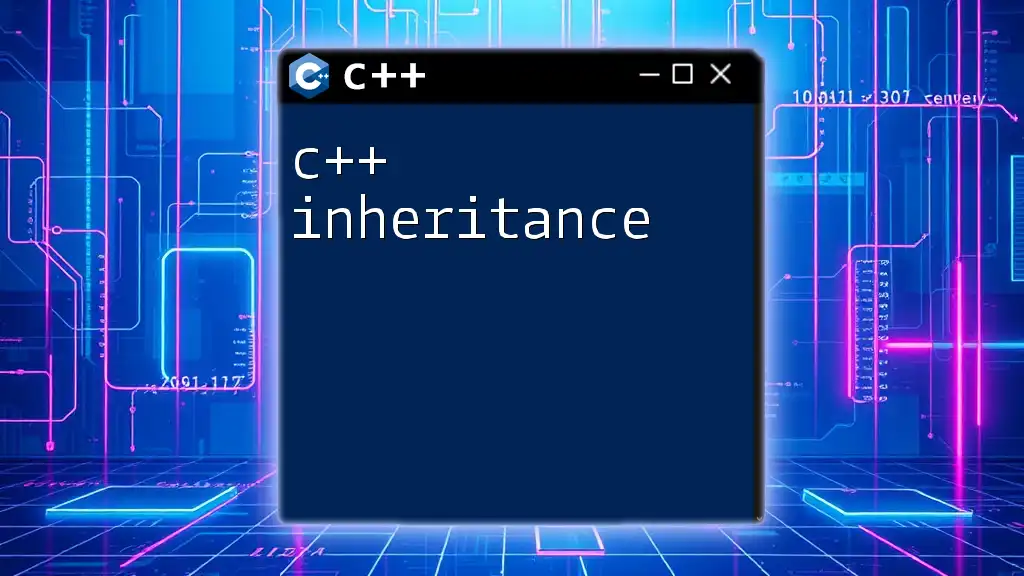
Best Practices for Database Management in C++
Security Measures
When working with databases in C++, always validate inputs and utilize parameterized queries to prevent SQL injection attacks. For instance, use prepared statements to help secure your queries against malicious input.
Efficiency Tips
To enhance efficiency:
- Connection pooling: Instead of creating and destroying connections repeatedly, maintain a pool of connections to reuse.
- Prepared statements: They minimize parsing time for repeatedly executed queries.
- Transaction handling: Use transactions to ensure data integrity when performing multiple operations.
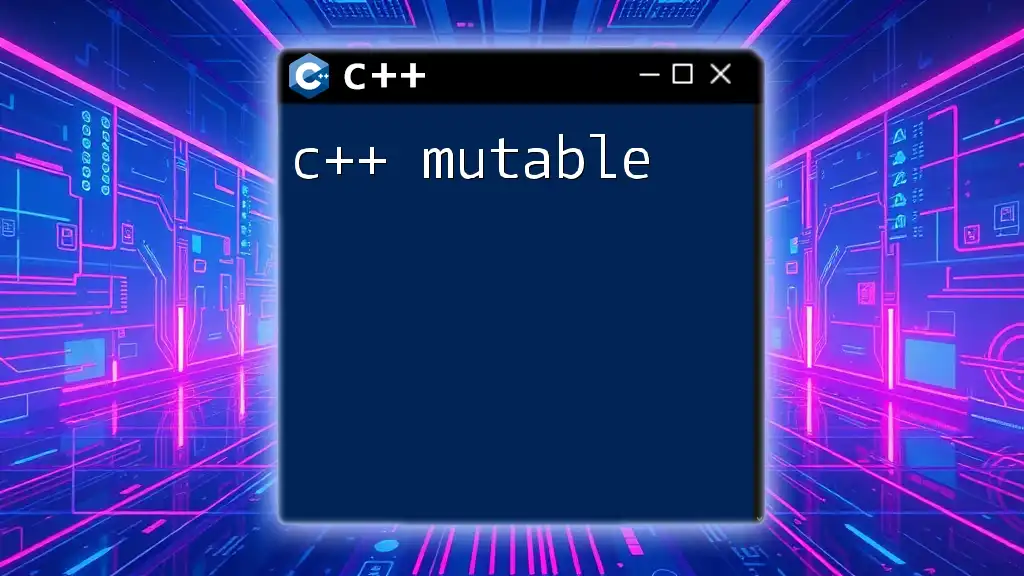
Error Handling and Troubleshooting
Common Errors in C++ Database Applications
When developing C++ applications that interact with databases, you may encounter various errors, such as connection failures, syntax issues in SQL commands, or runtime exceptions. Being aware of potential issues helps you proactively manage them.
Error messages returned by the database (using `mysql_error(conn)`) can guide you in debugging your application. Always log these messages for better visibility when issues arise.
Debugging Techniques
Implement logging mechanisms to capture errors. Utilize debugging tools built into your IDE to step through the code and directly observe what happens during database interactions. Validating input data before it reaches the database will further aid in debugging.
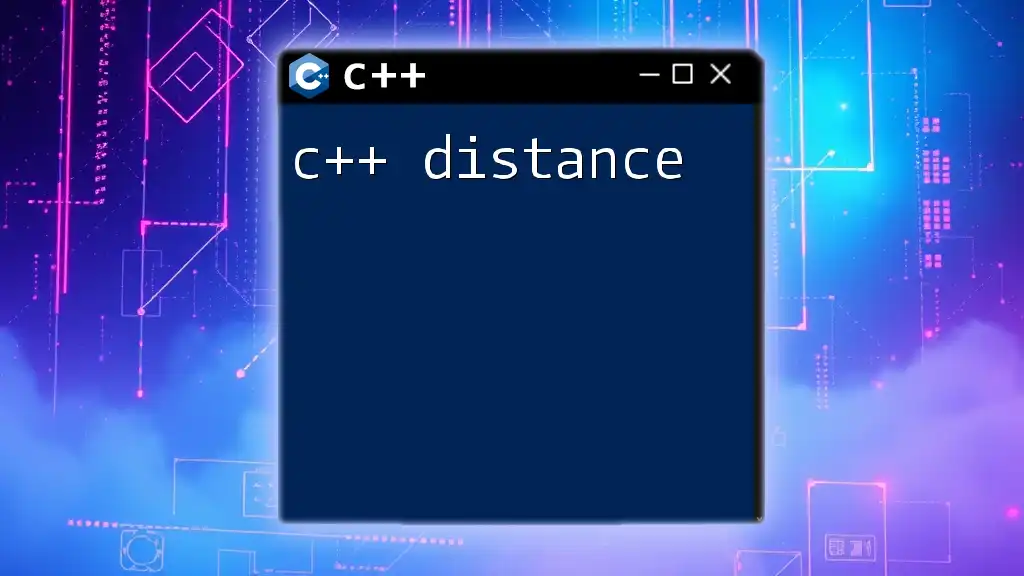
Conclusion
In summary, C++ and databases is a powerful combination that can lead to creating robust and efficient applications. By understanding the principles of databases, mastering the tools available for C++, and adhering to best practices, you can develop applications that leverage data effectively. Future advancements in C++ and database technologies will continue to simplify and enhance these interactions, making them even more accessible for developers.
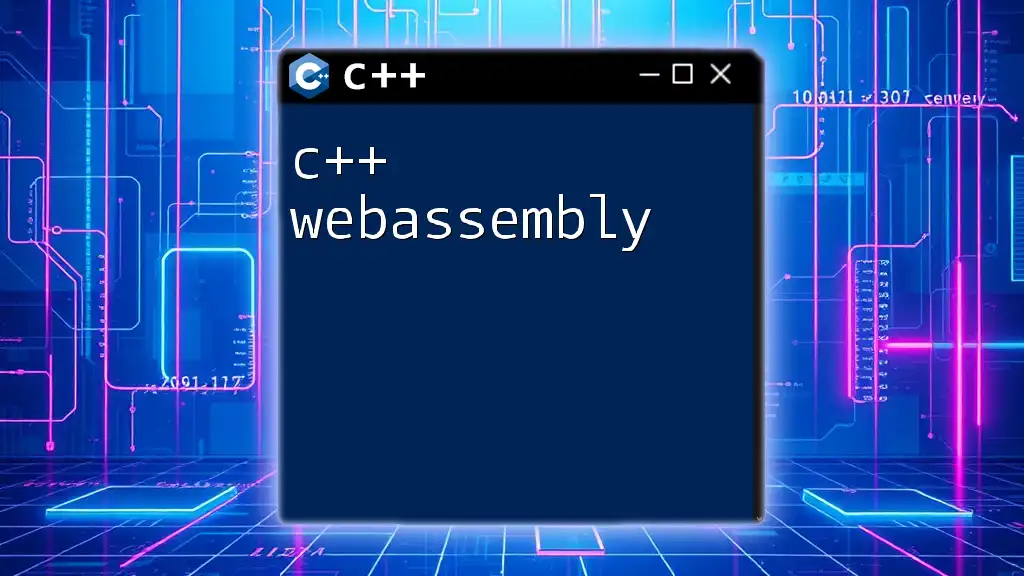
Additional Resources
For further exploration, consider checking official documentation for specific databases, community forums for practical advice, and current libraries suited for C++ database operations. These resources can provide additional insights as you delve deeper into C++ and databases.