"Assembly to C++ involves translating low-level assembly instructions into higher-level C++ code to improve readability and maintainability while leveraging the power of C++’s abstractions."
Here's a code snippet showcasing a simple example of translating an assembly operation to C++:
// Assembly: mov eax, 5
int main() {
int a = 5; // Equivalent C++ code
return 0;
}
Understanding Assembly Language
What is Assembly Language?
Assembly language is a low-level programming language that provides a symbolic representation of a processor's machine code. Unlike high-level programming languages, which use natural language and abstract away the hardware, assembly language is closely tied to the architecture of the computer, allowing programmers to write instructions that the CPU can execute directly.
How Assembly Differs from High-Level Languages
One key difference between assembly and high-level languages like C++ is abstraction. High-level languages provide constructs that allow developers to work with complex data structures without needing to understand the underlying hardware. Assembly, on the other hand, operates at a very granular level, allowing programmers to control hardware directly but requiring them to manage very low-level details, such as memory addresses and register contents.
The primary reason for using assembly code lies in performance. In cases where every cycle counts, assembly can be more efficient than high-level languages, as it allows fine-tuned optimization that higher-level languages may not permit.
Key Terminology in Assembly Language
Understanding a few fundamental terms related to assembly language is crucial for translating assembly to C++. Here are some of the most important:
- Registers: Small storage locations within the CPU where data is kept for quick access.
- Instructions: The commands that the CPU executes.
- Opcode: The part of an instruction that specifies the operation to be performed.
- Operands: The values or locations used in the operation defined by the opcode.
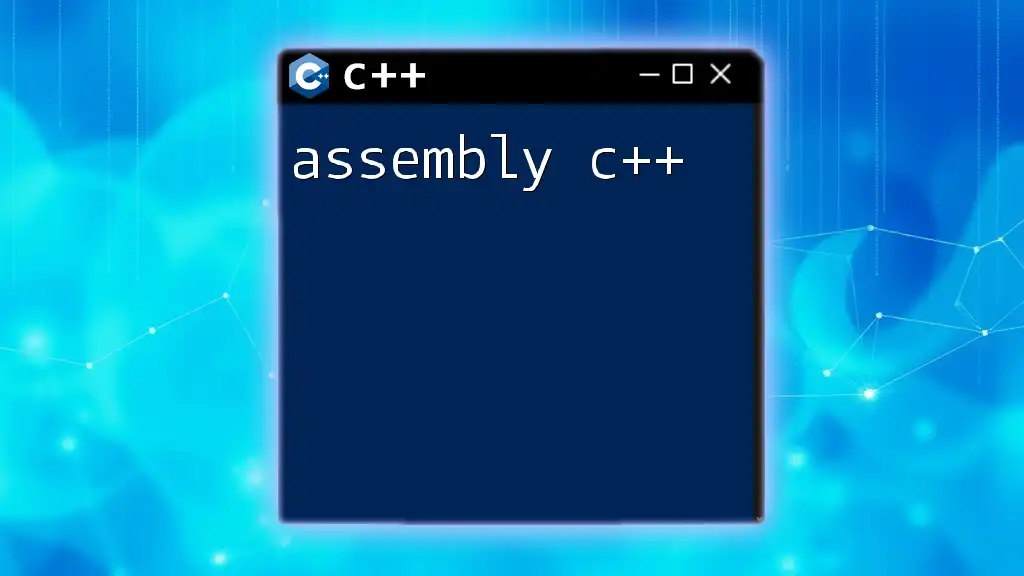
Why Use Assembly in C++?
Performance Optimization
When you need extreme performance, understanding how to integrate assembly with C++ can be invaluable. For instance, algorithms that demand maximum efficiency—such as those in graphics processing or real-time systems—often benefit from custom assembly routines. In such contexts, assembly can handle specific tasks, such as bit manipulation or mathematical operations, faster than standard C++ code.
Learning Opportunity
Learning how to translate assembly to C++ can significantly enhance your programming skills. It enables you to appreciate what occurs under the hood when you write high-level code, making you more proficient in optimizing C++ programs. Moreover, grasping assembly language fundamentals equips you with the tools to troubleshoot performance bottlenecks in applications.
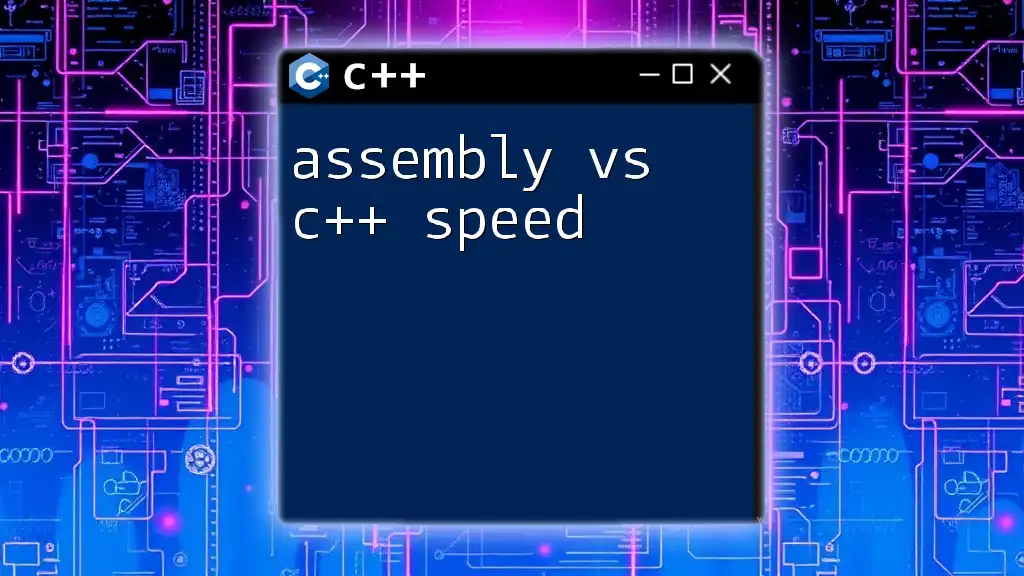
Translating Assembly to C++
Basic Structure of Assembly Code
Every assembly language program consists of several instructions that interact with the CPU. Here's a simple example:
mov eax, 5
add eax, 10
In this snippet, `mov` is an instruction that moves the value `5` into the register `eax`, and the `add` instruction then adds `10` to the current value in `eax`.
Simple Assembly to C++ Conversion
Translating the assembly example above into C++ is relatively straightforward. Here’s how it looks:
int main() {
int eax = 5;
eax += 10;
return 0;
}
In this C++ code, we declare an integer called `eax`, assign it the value of `5`, and then increment it by `10`. Each step corresponds closely to the operations performed in the assembly code. Understanding such conversions can facilitate more effective debugging and optimization.
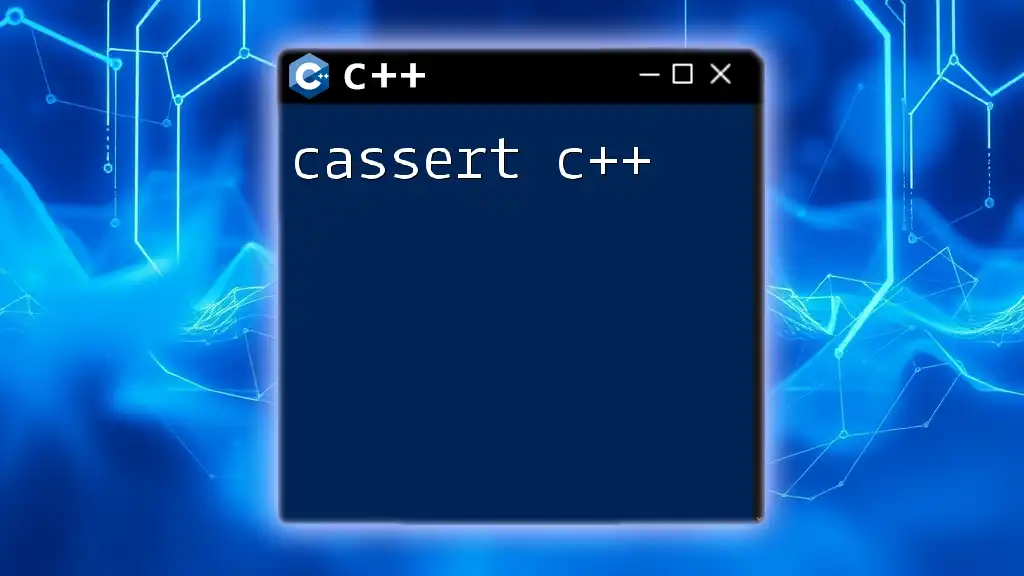
Common Assembly Instructions and Their C++ Equivalents
Data Movement Instructions
Data movement instructions are crucial for transferring data between registers and memory locations.
Example: MOV in Assembly
Consider the following assembly instruction:
mov eax, 10
Its equivalent in C++ is:
int eax = 10;
This straightforward translation represents the assignment of the value `10` to a variable `eax`.
Arithmetic Instructions
Arithmetic instructions perform basic mathematical operations.
Example: ADD in Assembly
This assembly instruction:
add eax, 5
Corresponds to the following C++ code:
eax += 5;
The translation is direct, as both instructions increase the value in `eax` by `5`.
Control Flow Instructions
Control flow instructions direct the execution flow of the program based on conditions.
Example: Jump Instructions
In assembly, you might find:
cmp eax, 10
je label
The equivalent C++ code would resemble:
if (eax == 10) {
// execute label code
}
In this context, the `cmp` instruction compares the value in `eax` with `10`, and if they are equal, control of execution jumps to a certain section of the code.
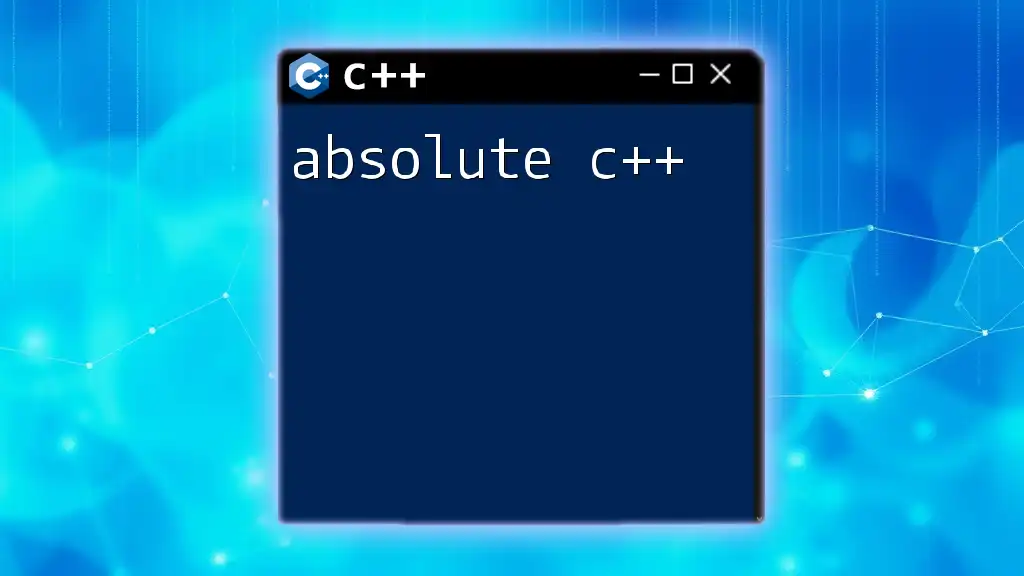
Using Inline Assembly in C++
What is Inline Assembly?
Inline assembly allows you to insert assembly code directly into your C++ programs, granting you the ability to optimize specific sections for performance. While not all compilers support inline assembly, those that do enable developers to blend the efficiency of assembly language with the functionality of C++.
Syntax and Structure for Inline Assembly
Inline assembly can be structured as follows in C++:
__asm {
mov eax, 1
add eax, 2
}
This block of code demonstrates how you can write assembly instructions right within your C++ code. The advantages of this approach include enhanced performance in certain system-critical functions while maintaining your overall codebase in C++.
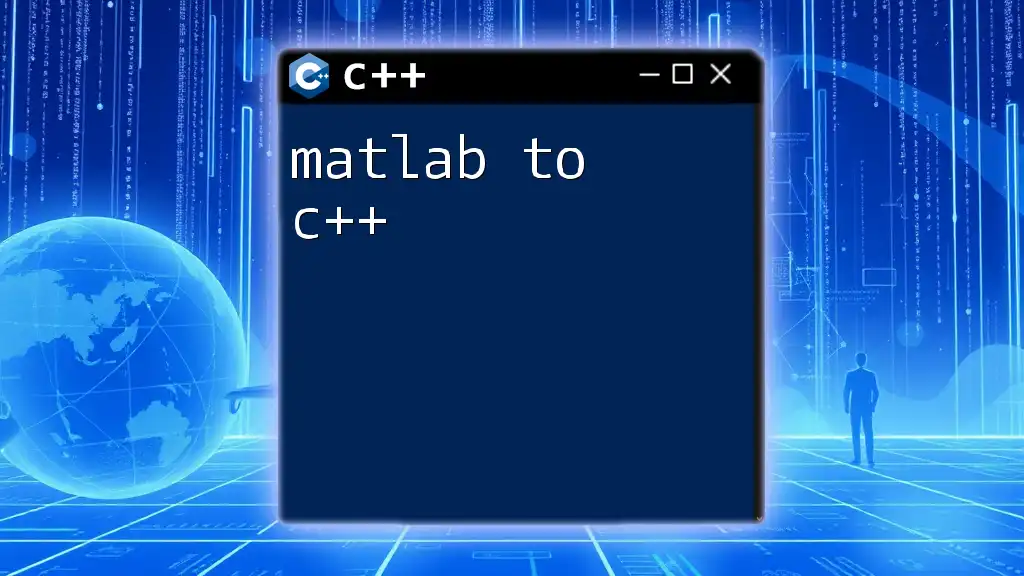
Best Practices for Mixing Assembly with C++
Maintain Readability
Maintaining readability in your code is essential, especially when mixing assembly with C++. While inline assembly can provide performance boosts, it can also lead to confusion if not documented well. Always comment your code generously to explain the purpose of any assembly instructions and how they relate to the surrounding C++. Structuring code correctly and keeping inline snippets brief can significantly enhance maintainability.
Debugging Considerations
Debugging a mix of assembly and C++ can be challenging. To aid the process, utilizing tools such as debuggers that support both formats can be beneficial. Pay special attention to the registers and memory addresses being manipulated, as mistakes in these areas can lead to unexpected behavior. Common pitfalls include improperly managing the state of registers between high-level calls and inline assembly, which can cause issues with program logic.
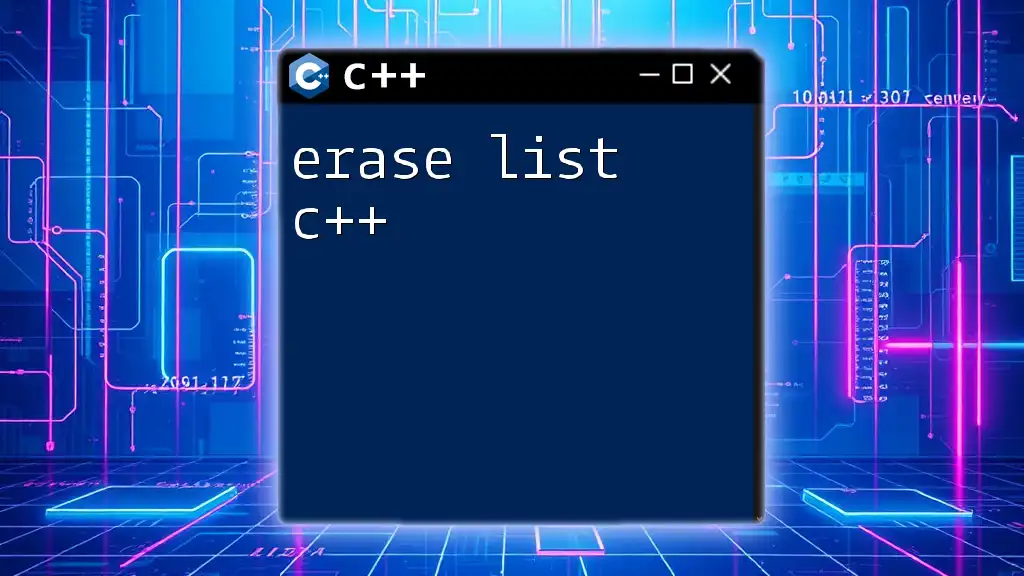
Conclusion
Summary of Key Points
Translating assembly to C++ showcases a fundamental skill for performance optimization and deeper understanding of how computers execute code. By learning assembly, you'll equip yourself with the knowledge needed to write more efficient programs.
Further Learning Resources
To extend your knowledge beyond this introductory guide, consider diving into specialized books focused on assembly language, C++ optimizations, and systems programming. Online courses and programming forums dedicated to assembly and C++ provide exceptional resources for further practice and engagement with the community.
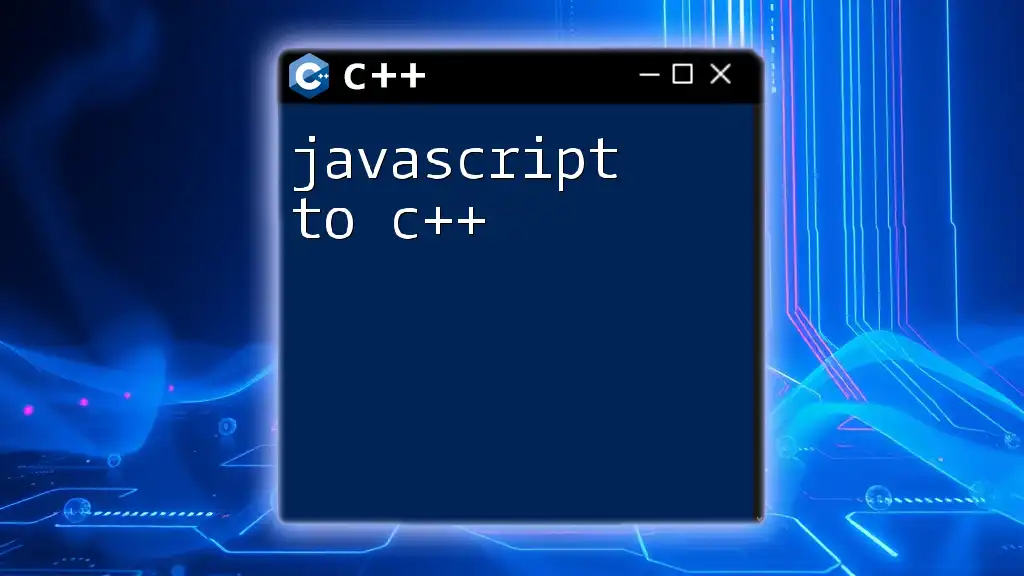
Call to Action
If you're interested in deepening your understanding of C++, assembly, and how they interact, connect with us for tailored classes and resources that will elevate your programming skills. Don't miss out on the opportunity to master performance optimization techniques! Whether you're a beginner or experienced programmer, our offerings will help you harness the full power of assembly to C++ translation.