When comparing assembly and C++ speed, assembly typically offers faster execution due to its low-level nature and direct hardware manipulation, but C++ often provides sufficiently efficient performance with enhanced readability and maintainability.
// Example of a simple C++ function
#include <iostream>
int sum(int a, int b) {
return a + b;
}
int main() {
std::cout << sum(3, 4) << std::endl; // Outputs: 7
return 0;
}
What is Assembly Language?
Definition of Assembly Language
Assembly language is a low-level programming language that provides a way to write instructions that are specifically tailored for a computer's architecture. Unlike high-level programming languages that are more abstract and human-readable, Assembly language closely resembles machine code, which consists of binary instructions directly executed by the CPU. Assembly code uses mnemonics, which are symbolic representations of various machine instructions, making it slightly easier to write and understand compared to pure binary.
How Assembly Language Works
Assembly language serves as an intermediary between high-level programming languages and machine code. When the programmer writes Assembly code, it must go through an assembler, a tool that translates it into machine code using the appropriate syntax for the CPU architecture. The performance of Assembly language heavily depends on factors such as instruction set architecture, the efficiency of the assembler, and the skill of the programmer.
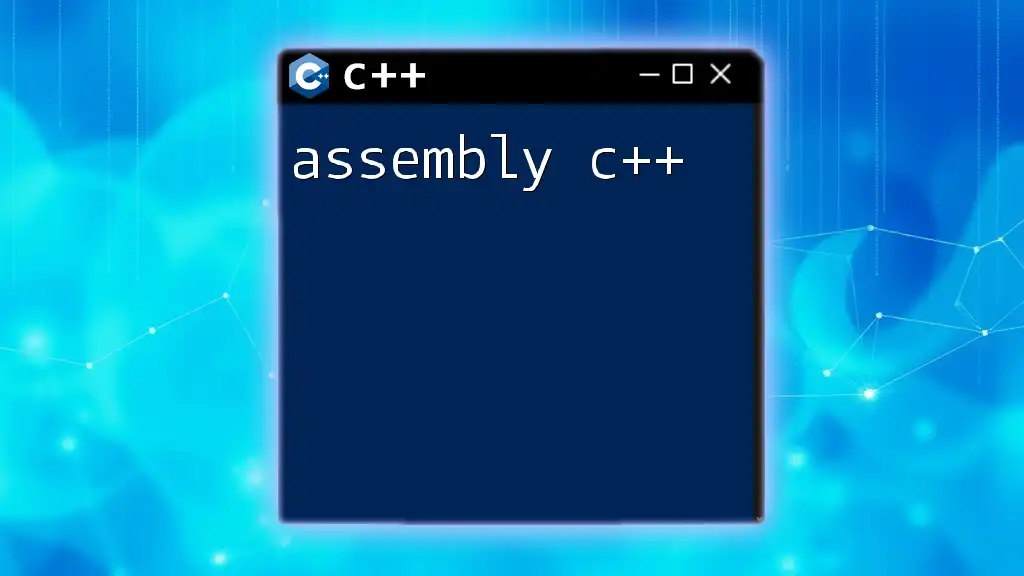
What is C++?
Definition of C++
C++ is a powerful high-level programming language that combines both high-level and low-level language features. It is renowned for its versatility and efficiency, particularly in object-oriented programming. C++ allows for a blend of low-level manipulation (similar to Assembly) with high-level constructs that facilitate complex software development. It incorporates features like classes, inheritance, and operator overloading, enabling developers to write robust and maintainable code.
How C++ is Compiled
The process of transforming C++ code into executable machine code involves several stages. Initially, the code undergoes preprocessing, where directives are handled. Next comes the compilation phase, during which the C++ code is converted into object code. Finally, linking combines the object code with libraries and other modules to create an executable. Modern C++ compilers are highly efficient and integrate optimization techniques that can significantly enhance the speed of the final program. Optimization levels, such as `-O2` or `-O3`, allow developers to adjust compiler behavior to balance speed and code size.
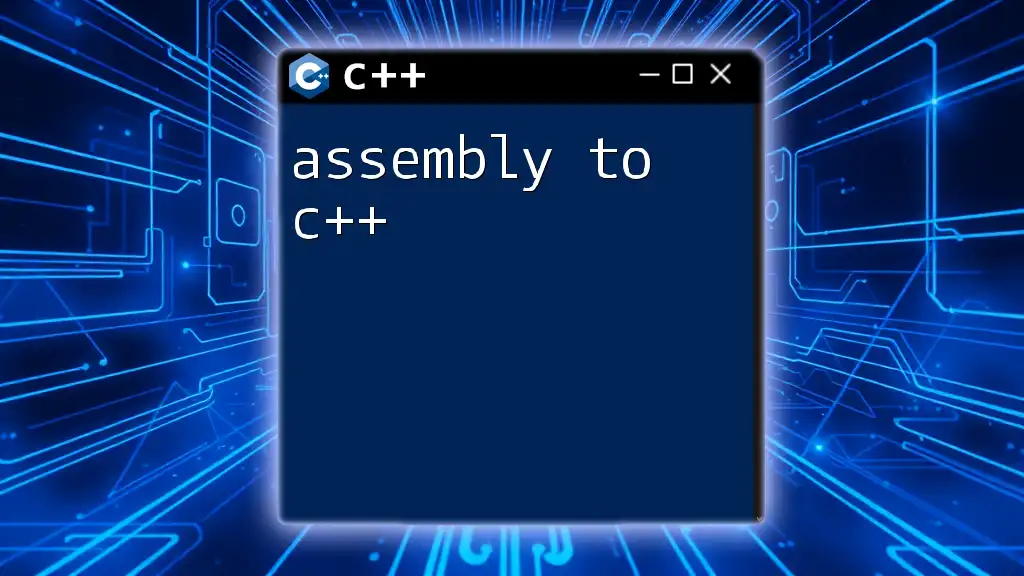
Speed Comparison: Assembly vs C++
Performance Factors
Compilation vs Assembly
Understanding the difference in execution speed between Assembly and C++ starts with recognizing the compilation process. Assembly language is already close to machine code and requires no compilation overhead. In contrast, C++ must go through a compiling and linking phase, which introduces some delays, although modern compilers manage to optimize this well.
Overhead in C++
Overhead refers to extra time and resources consumed by various features of a programming language. In C++, things like function calls and memory management can introduce overhead. For instance, the use of virtual functions incurs additional costs due to dynamic dispatch. While these features enhance flexibility and maintainability, they can also lead to slower execution times, especially in performance-critical applications.
Benchmarks and Case Studies
Real-World Benchmarks
To illustrate the differences between Assembly vs C++ speed, consider benchmarks conducted on similar tasks, such as numerical computations or graphics rendering. In scenarios that demand meticulous control over the CPU, Assembly may shine, often achieving execution times significantly lower than C++. Conversely, for broader applications, C++ can perform exceptionally well, especially when optimized for particular tasks.
Case Study: Algorithm Implementation
An example algorithm, such as the Fibonacci sequence, demonstrates the disparity between C++ and Assembly. Below are two implementations, illustrating how performance can differ:
Assembly Implementation (x86 syntax):
section .text
global _start
_start:
mov ecx, 10 ; Compute the 10th Fibonacci number
call fib
; Exit program
mov eax, 1
int 0x80
fib:
cmp ecx, 1
jle .done
push ecx
dec ecx
call fib
mov ebx, eax
pop ecx
dec ecx
call fib
add eax, ebx
.done:
ret
C++ Implementation:
#include <iostream>
int fibonacci(int n) {
if (n <= 1) return n;
return fibonacci(n - 1) + fibonacci(n - 2);
}
int main() {
int result = fibonacci(10);
std::cout << result << std::endl;
return 0;
}
While the Assembly version minimizes overhead directly on the CPU, the C++ version benefits from readability and expressiveness, making it easier to implement and maintain.
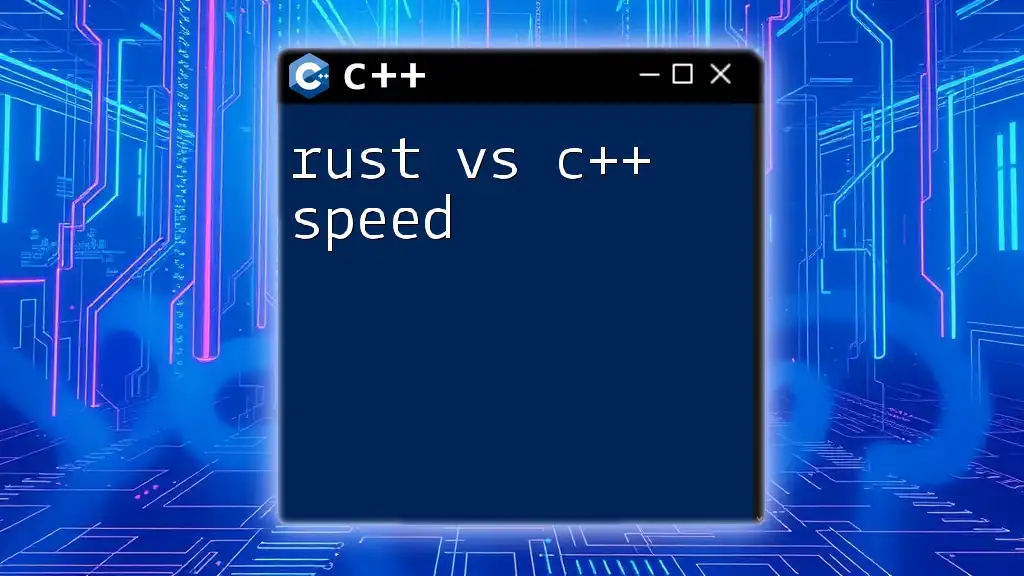
When to Choose Assembly Over C++
Situations Favoring Assembly
Assembly is ideal under certain conditions:
- Real-time systems: Applications that demand immediate response, such as embedded systems or firmware, may require the precision and speed of Assembly to meet specific timing constraints.
- Hardware-accelerated programming: When working closely with hardware, such as device drivers, Assembly language may be necessary for direct manipulation of the hardware resources.
Situations Favoring C++
C++ is often sufficient in many situations due to its expansive capabilities:
- Complex applications: Most software development uses C++ where the focus is on speed of development and maintenance rather than the absolute peak performance.
- Cross-platform development: C++ shines in scenarios where applications need to be portable across multiple operating systems, as its standardized libraries mitigate platform-specific concerns.
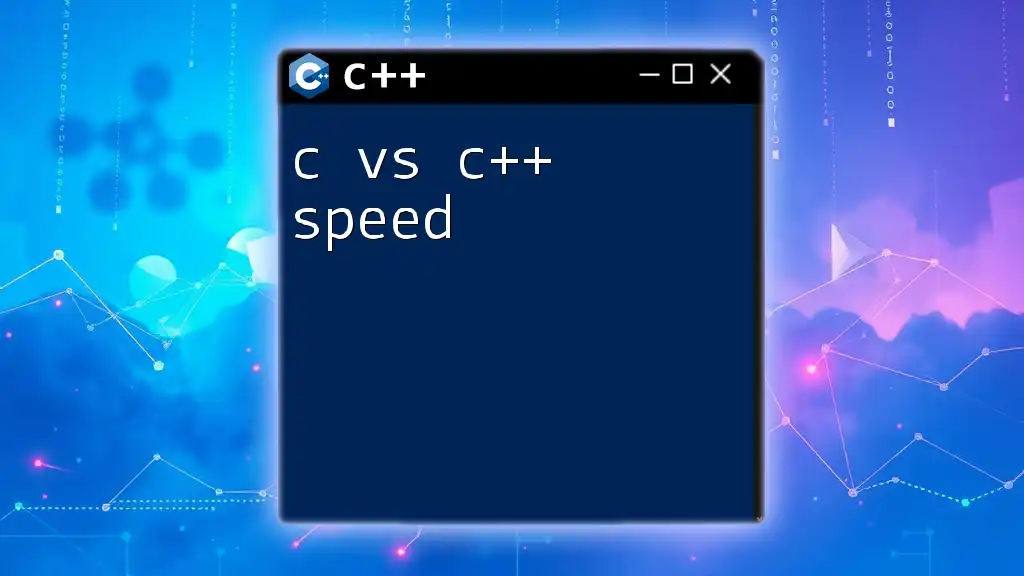
Advantages of Each Language
Advantages of Assembly Language
- Direct hardware control: Assembly permits explicit access and manipulation of hardware resources, making it indispensable in low-level programming.
- Efficiency: Assembly instructions directly correspond to machine instructions, leading to extremely efficient execution times when optimized.
Advantages of C++
- High-level constructs: C++ offers features like classes and templates, allowing developers to create modular and reusable code, which significantly increases productivity.
- Portability: C++ code can be compiled across different platforms with minimal modification, while Assembly is often bound to specific architectures.
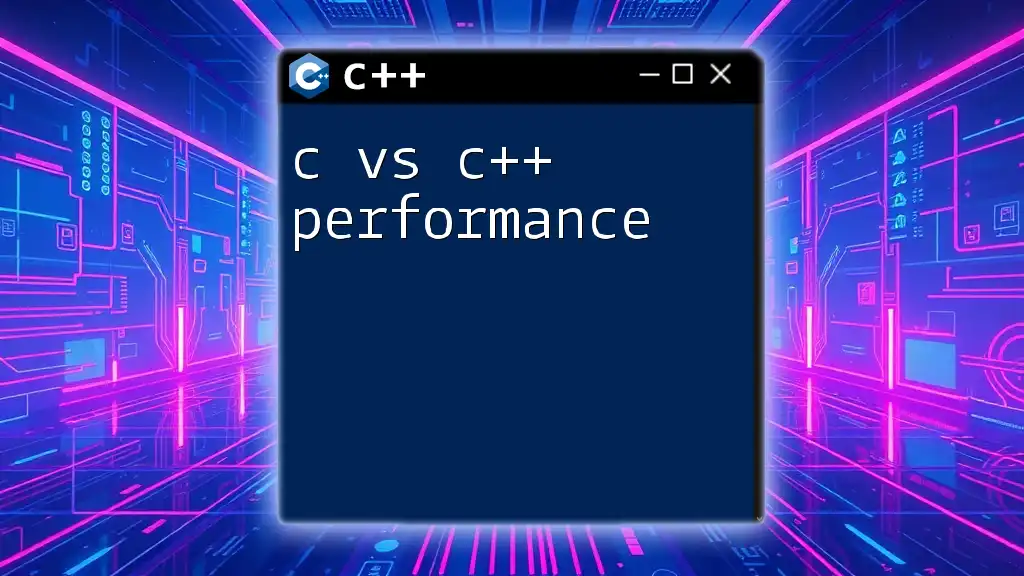
Trade-Offs Between Speed and Development Time
Speed of Development
One of the primary considerations when choosing between Assembly and C++ is the speed of development. C++ enables quicker iterations, allowing teams to build and refactor code much faster. If rapid development and a shorter timeline are priorities, C++ is the clear choice.
Maintainability vs. Performance
Over the long term, maintainable code often becomes more critical than optimized code. C++ supports clear abstractions and structured programming, which benefit collaborative teams and dynamic project requirements. Assembly, while efficient, can become complex and challenging to manage, making future enhancements difficult.
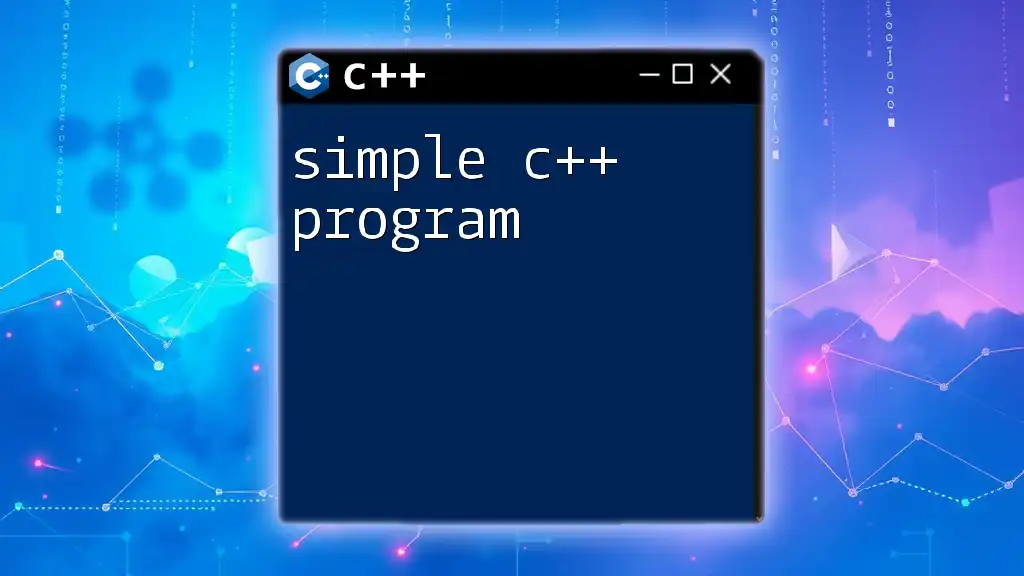
Conclusion
In the ongoing debate of assembly vs C++ speed, the choice is far from one-dimensional. Understanding the context of your project and its specific requirements will guide this decision. Assembly offers the ultimate control over hardware and performance, while C++ provides a balance of speed, flexibility, and maintainability. Ultimately, making an informed choice means weighing the specific benefits and drawbacks of both languages in terms of speed, efficiency, and development time.