Rust often outperforms C++ in speed for certain tasks due to its efficient memory management and zero-cost abstractions, making it a compelling choice for high-performance applications.
Here’s a simple example of a C++ program demonstrating basic performance measurement:
#include <iostream>
#include <chrono>
int main() {
auto start = std::chrono::high_resolution_clock::now();
// Example computation
for (int i = 0; i < 1000000; ++i) {
// Perform some computation
}
auto end = std::chrono::high_resolution_clock::now();
std::chrono::duration<double> elapsed = end - start;
std::cout << "Elapsed time: " << elapsed.count() << " seconds\n";
return 0;
}
Understanding Performance Metrics
What Does Performance Mean?
Performance in programming refers to how efficiently a language's code executes in terms of speed, memory usage, and responsiveness. The goal is to write code that runs quickly and consumes minimal resources. In this context, the metrics we consider when comparing Rust vs C++ speed include execution time, memory efficiency, and overall throughput of tasks.
Metrics for Measuring Speed
When discussing programming speed, we typically evaluate the following metrics:
- Execution Time: This is the time taken by a specific code segment to complete its task. It can be measured using different timing methods available in both languages.
- Memory Usage: The amount of memory a program consumes while running is crucial, as more efficient memory usage allows the system to manage multiple tasks effectively.
- Throughput: Refers to the number of operations or tasks completed in a given time frame. High throughput often correlates with high performance.
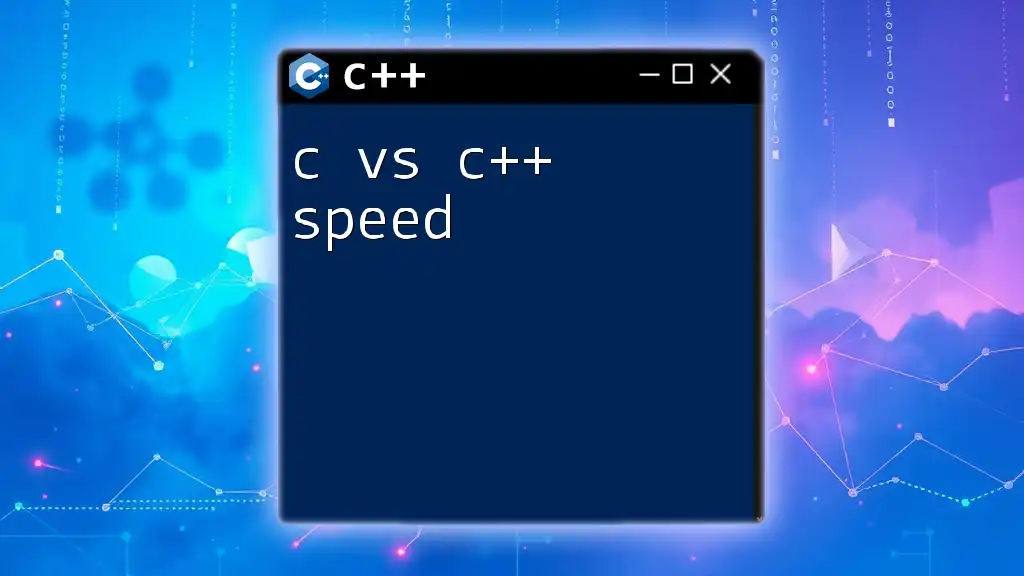
Overview of Rust and C++
Rust and C++ are two programming languages known for their powerful performance characteristics, though they serve slightly different purposes.
C++, developed in the early 1980s, is known for its versatility and speed, making it a go-to choice for system software, game development, and applications requiring real-time performance. Its robust feature set allows fine-grained control over system resources.
Rust, released in 2010, emphasizes safety and concurrency, prioritizing memory safety without sacrificing speed. Its unique ownership model helps prevent common bugs found in languages like C++, thereby enhancing overall reliability.
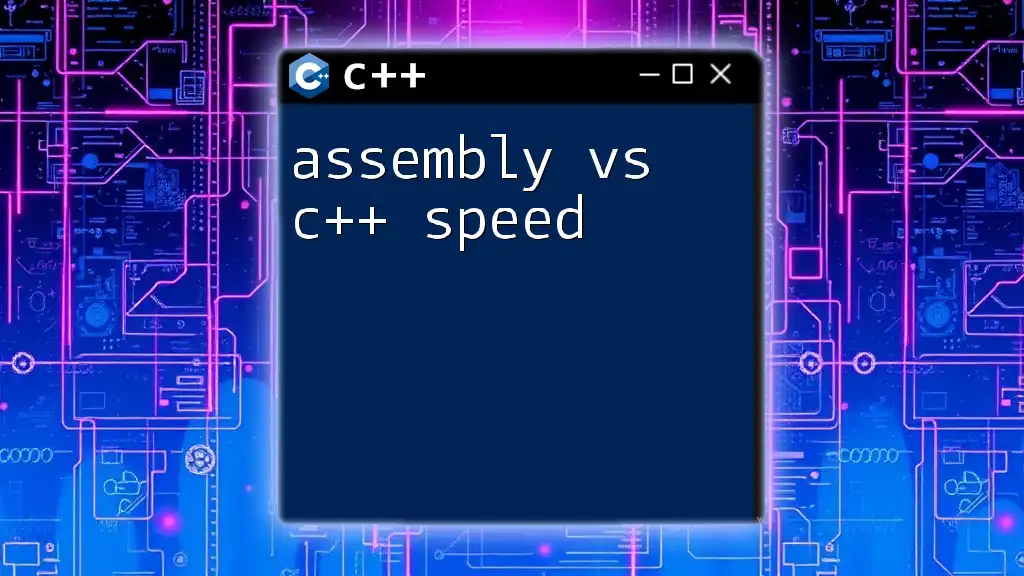
Speed Comparison: Rust vs C++
Compilation Speed
When it comes to compilation speed, C++ is generally faster due to its mature tooling and the absence of strict compile-time checks that Rust imposes, such as its borrow checker. While this features helps Rust ensure memory safety, it can lead to longer compilation times. Rust's focus on safety means that developers often face compile-time errors that require resolution, slowing down the development process but leading to potentially more reliable code.
Runtime Performance
Code Optimization
Both languages excel in optimization, but their approaches differ. Rust emphasizes zero-cost abstractions: you write high-level code, and the compiler generates efficient machine code without overhead.
C++, on the other hand, leverages templates and inlining, allowing significant optimizations at compile time. The built-in capabilities of both languages enable developers to write performance-critical applications.
Example: Simple Computation Task
Let’s analyze a simple computation task to compare performance. Both examples sum numbers from zero to one million.
C++ implementation:
#include <iostream>
#include <chrono>
int main() {
auto start = std::chrono::high_resolution_clock::now();
int sum = 0;
for (int i = 0; i < 1e6; ++i) {
sum += i;
}
auto end = std::chrono::high_resolution_clock::now();
std::cout << "Sum: " << sum << " Time: "
<< std::chrono::duration_cast<std::chrono::microseconds>(end - start).count()
<< " microseconds" << std::endl;
return 0;
}
Rust implementation:
fn main() {
let start = std::time::Instant::now();
let sum: i32 = (0..1_000_000).sum();
let duration = start.elapsed();
println!("Sum: {} Time: {:?}", sum, duration.as_micros());
}
While both snippets perform similarly, varying results may appear due to compiler optimizations, hardware differences, and specific cases. Benchmarks in real-world scenarios should always be conducted in the specific environment of interest.
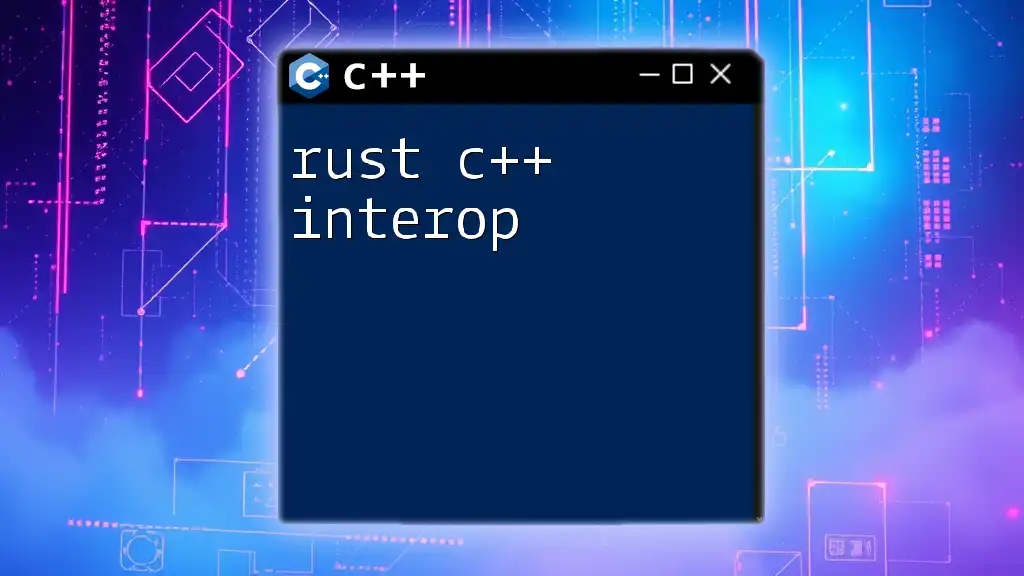
Factors Affecting Speed
Memory Management
Memory management has a profound impact on performance. C++ employs manual memory management, offering greater control over resource allocation. However, this can lead to errors like memory leaks or dangling pointers, potentially degrading speed.
Conversely, Rust implements an ownership model, ensuring that memory is automatically managed without garbage collection. This guarantees safety and often leads to better performance through lesser runtime checks.
Concurrency and Parallelism
Both languages provide robust concurrency features. Rust’s ownership system allows for safe concurrent programming, preventing data races at compile time. In contrast, C++ offers various libraries and language features for multi-threading but requires developers to handle concurrency risks manually.
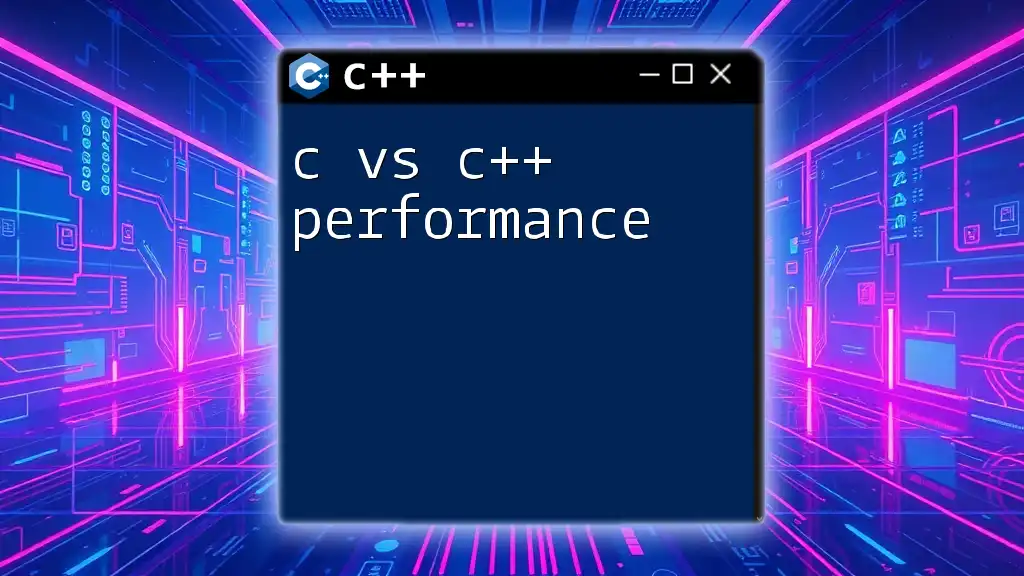
Real-World Applications and Benchmarks
Use Cases for Rust
Rust shines in domains requiring high performance and safety, such as:
- Web servers: Frameworks like Actix and Rocket leverage Rust’s speed.
- WebAssembly and systems programming: Rust is being utilized for applications that demand both efficiency and safety.
Benchmarks indicate that Rust can perform exceptionally well in high-load scenarios, rivaling the speed of C++ without sacrificing the integrity of the code.
Use Cases for C++
C++ is widely used in industries where performance is crucial. This includes:
- Game development: Popular engines like Unreal Engine rely on C++ for real-time performance.
- Graphics programming, such as OpenGL or rendering engines, takes advantage of C++’s efficiency.
Performance benchmarks often showcase C++ as a high-speed option for low-level system tasks, taking advantage of its fine control over hardware.
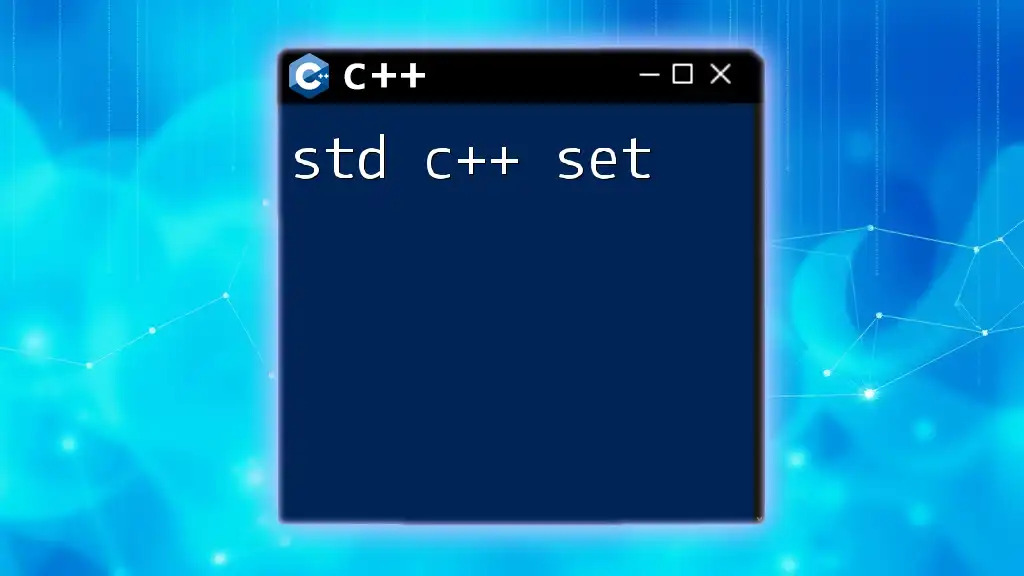
Community and Ecosystem Impact on Performance
Libraries and Tools
Both languages boast extensive ecosystems. Rust's toolchain, including Cargo and Clippy, assists in maintaining performance while simplifying dependency management. C++ benefits from robust libraries like Boost, enabling various optimizations that yield substantial performance improvements.
Ongoing Trends and Future Developments
As technology evolves, so does the performance landscape. Both Rust and C++ are receiving regular updates, incorporating features to enhance speed and safety. With Rust gaining traction in multi-threaded applications and C++ seeing improvements in language features like ranges and concepts, the future of both languages looks promising.
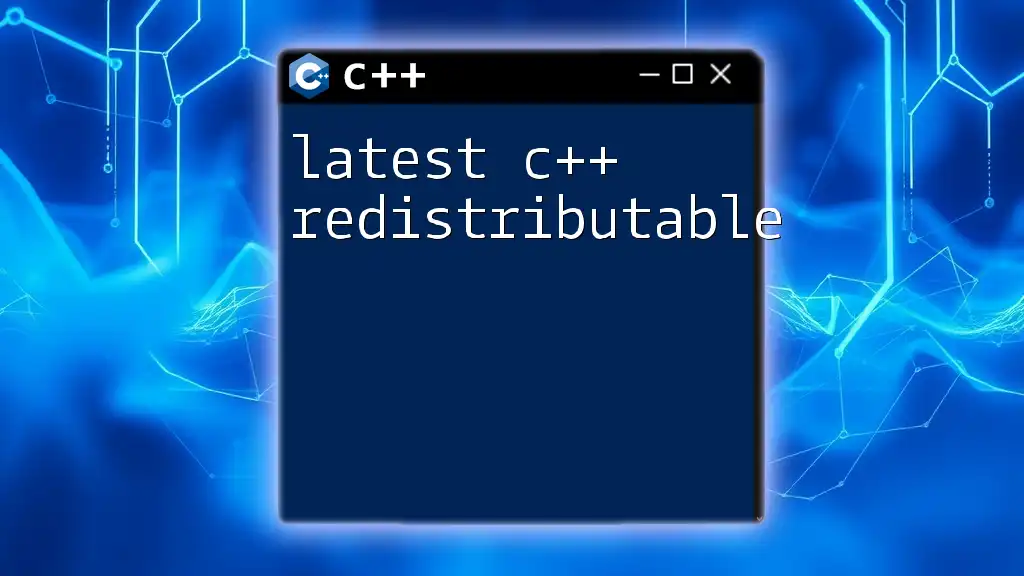
Conclusion
In the debate of Rust vs C++ speed, the conclusion is nuanced. C++ offers speed and efficiency with greater control, while Rust promotes safe programming practices, often leading to reliable performance in demanding scenarios. Ultimately, the choice between Rust and C++ hinges on the specific requirements of the project, the experience of the development team, and performance goals.
Understanding the characteristics that influence Rust vs C++ performance is key. Developers must consider compile times, runtime performance, memory management, and community support when making their decisions.