C is generally faster than C++ due to its simpler syntax and lower-level operations, which allow for more efficient memory management and execution.
Here's a simple code snippet illustrating a basic operation in both languages:
#include <iostream>
int main() {
int sum = 0;
for (int i = 0; i < 1000000; ++i) {
sum += i;
}
std::cout << "Sum: " << sum << std::endl;
return 0;
}
Understanding Performance in Programming Languages
What is Performance?
Performance in programming is defined by how efficiently code executes and how well it utilizes system resources. Two critical aspects of performance include execution time and memory usage. Execution time refers to how fast a program runs, while memory usage measures how much RAM it consumes during execution. Understanding these metrics is vital for developers, especially when choosing between C and C++ for specific tasks.
Importance of Performance in Software Development
In software development, performance is crucial as it directly impacts user experience. Applications that run smoothly and efficiently hold users' attention and create a positive impression. Conversely, slow applications can lead to frustration and abandonment. Hence, developers must consider how the choice between C vs C++ speed affects their work, especially in resource-constrained environments or real-time applications.
Metrics for Measuring Speed
When evaluating the speed of programming languages like C and C++, developers often rely on several performance metrics:
- Execution Time: The time it takes for a program to complete a task.
- Memory Usage: The amount of memory required by the code during its execution.
To measure these metrics accurately, developers often turn to benchmarking techniques:
- Microbenchmarks: Testing individual components of code.
- Macrobenchmarks: Assessing the performance of entire applications in real-world scenarios.
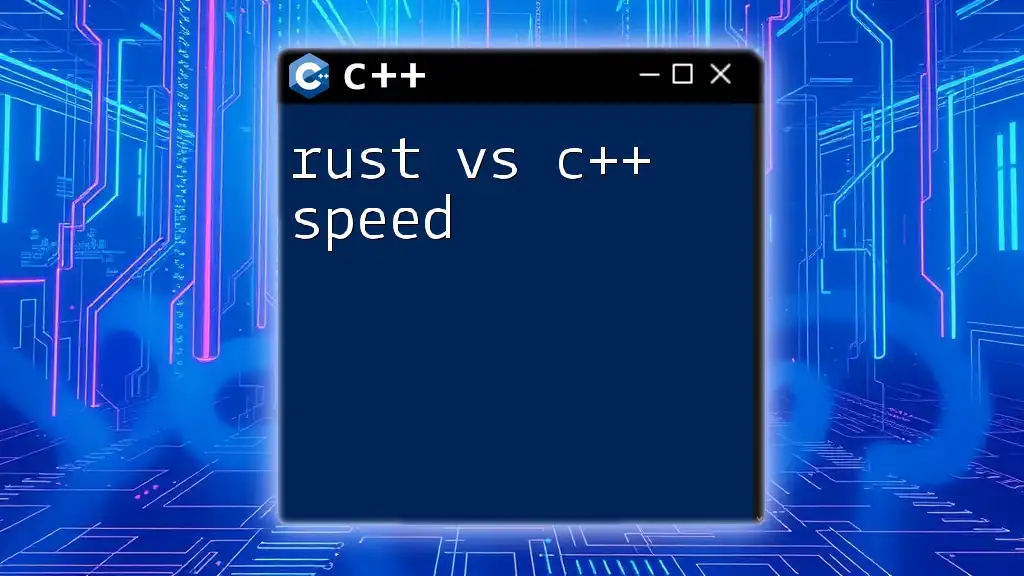
Core Differences Between C and C++
Language Paradigms
One significant difference between C and C++ is their programming paradigms.
- C is a procedural programming language, which means it focuses on the sequence of actions in a program.
- C++, on the other hand, incorporates object-oriented programming (OOP) principles, allowing developers to create objects that bundle data and functions.
Impact on Execution Speed
The choice of paradigm can significantly impact the execution speed of a program. C’s procedural approach can result in faster execution for straightforward tasks. In contrast, C++ may introduce some overhead due to its flexibility and additional features.
Consider the following code snippet, where both languages implement a simple loop operation:
C version:
#include <stdio.h>
int main() {
for (int i = 0; i < 1000000; i++) {
// Simple operation
}
return 0;
}
C++ version:
#include <iostream>
int main() {
for (int i = 0; i < 1000000; i++) {
// Simple operation
}
return 0;
}
Even though both snippets perform the same task, the underlying features and optimizations of the languages may yield different performance results.
Compiler Optimization
Another key factor in performance differences is how compilers optimize code for C and C++.
- C Compilers: C compilers often have simpler optimization models, focusing on procedural execution paths.
- C++ Compilers: C++ compilers leverage object-oriented features, which may lead to more nuanced optimizations but can also introduce overhead from features like virtual functions and inheritance.
By adjusting compiler flags, developers can influence performance in both languages. For example:
C:
gcc -O2 -o example_c example.c
C++:
g++ -O2 -o example_cpp example.cpp
Here, `-O2` tells the compiler to optimize the code for speed, but this may produce different results depending on the complexities of the respective codes.
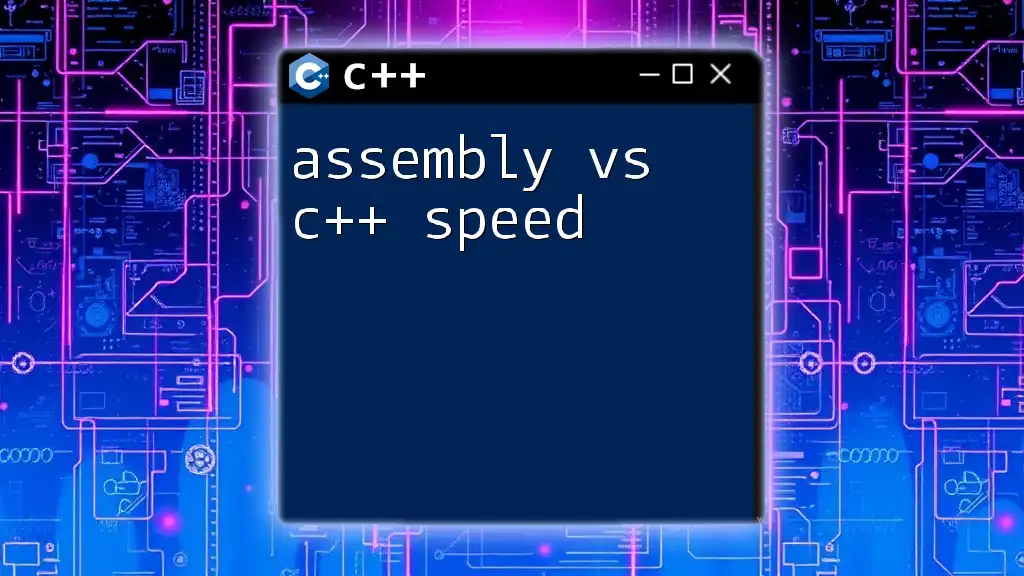
Is C Faster than C++?
Analyzing Speed Aspects
When consumers and developers alike ponder "C vs C++ speed," comparisons often arise. For direct execution speed tests, benchmarking can provide clarity. Here's a simplified benchmark example:
Running a computation in C:
#include <stdio.h>
#include <time.h>
int main() {
clock_t start = clock();
for (int i = 0; i < 1000000; i++) {
// Perform operation
}
clock_t end = clock();
printf("C execution time: %f seconds\n", (double)(end - start) / CLOCKS_PER_SEC);
return 0;
}
Now the same operation in C++:
#include <iostream>
#include <ctime>
int main() {
clock_t start = clock();
for (int i = 0; i < 1000000; i++) {
// Perform operation
}
clock_t end = clock();
std::cout << "C++ execution time: " << static_cast<double>(end - start) / CLOCKS_PER_SEC << " seconds" << std::endl;
return 0;
}
Even though both programs execute similar tasks, their execution times may reveal performance differences based on factors like compiler optimizations, language features, and system architecture.
Real-world Applications and Use Cases
In real-world applications, the choice between C vs C++ speed often comes down to the specific needs of the project.
- C is often favored in situations requiring maximum performance and minimal overhead, such as embedded systems or high-performance computing applications.
- C++ shines in scenarios where complex data structures and abstractions can significantly reduce development time, despite potentially slower execution due to additional features.
Interface and Overhead Considerations
C++ features such as inheritance, polymorphism, and templates can introduce performance overhead. While these features enhance code reusability and maintainability, they may slow down execution due to:
- Dynamic dispatch
- Increased memory usage for class metadata
For instance, a simple subclassing scenario in C++:
class Base {
public:
virtual void display() { std::cout << "Base Display"; }
};
class Derived : public Base {
public:
void display() override { std::cout << "Derived Display"; }
};
In this case, every call to `display()` incurs the overhead of dynamic polymorphism, which can marginally slow performance compared to a straightforward function call in C.
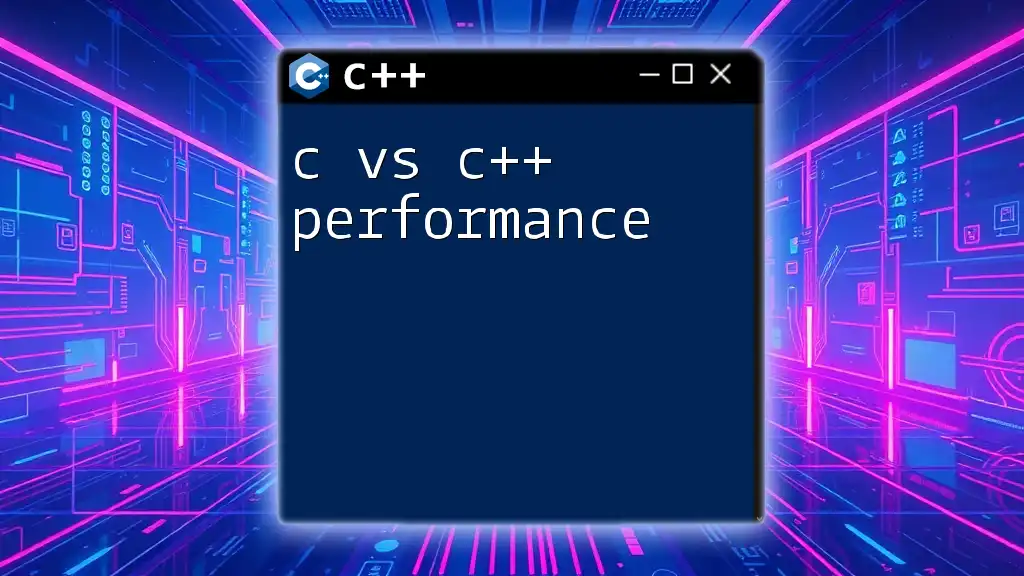
Trade-offs in Performance
Speed vs. Productivity
In many scenarios, the performance differences between C and C++ lead developers to consider a critical trade-off: speed vs. productivity.
- C may deliver superior raw performance, crucial for applications like game engines or high-frequency trading systems.
- C++, despite being potentially slower, allows developers to write less code and deliver faster implementations of complex systems due to its rich libraries and abstractions.
Memory Management
Finally, memory management plays a crucial role in the performance narrative.
- C employs manual memory management, granting developers ultimate control, but also placing the burden of allocation and deallocation on their shoulders.
- C++ incorporates constructs like smart pointers, which streamline memory management through automatic memory allocation and deallocation.
This allows C++ to manage memory more safely, albeit with possible performance costs due to the overhead of these abstractions. Consider the following C example for manual memory allocation:
#include <stdlib.h>
int main() {
int *array = (int *)malloc(sizeof(int) * 1000);
// Use array
free(array);
return 0;
}
In contrast, in C++, one might use:
#include <memory>
int main() {
std::unique_ptr<int[]> array(new int[1000]);
// Use array
return 0; // Automatically deallocated
}
This illustrates how C++ can automate memory handling, which is helpful for preventing memory leaks. Yet, this automation can come with its own performance trade-offs.
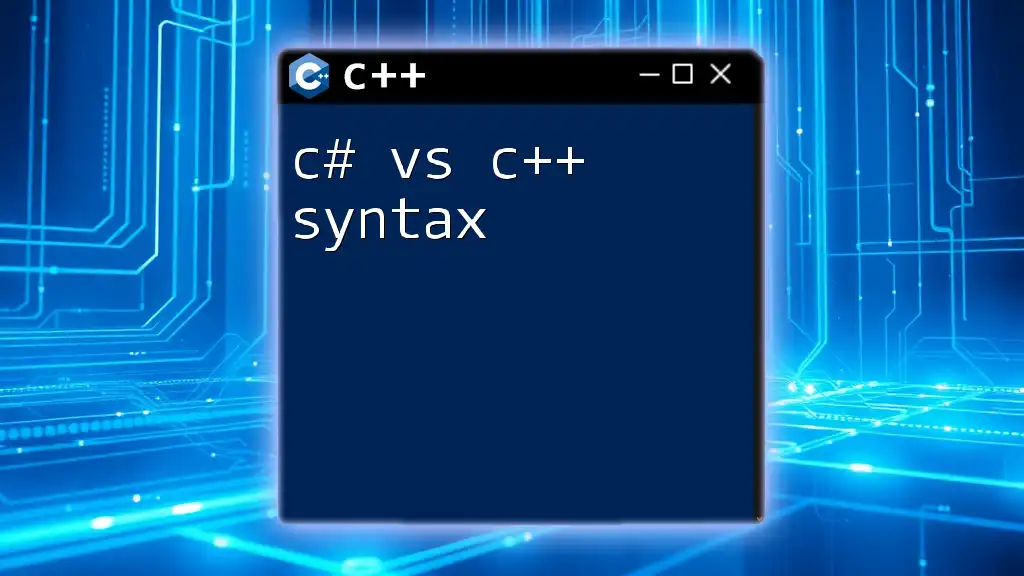
Conclusion
The comparison of C vs C++ speed reveals nuanced differences that depend on the context of use. While C often excels in raw execution speed, especially in simpler tasks, C++ provides more powerful abstractions that can enhance productivity and maintainability. Developers must weigh their priorities—whether raw performance or development efficiency—when choosing between these two languages.
As with any programming decision, understanding the specific requirements of a project will guide the choice, enabling developers to leverage the strengths of each language effectively.