In C++, the `std::this_thread::sleep_for` function can be used to pause program execution for a specified duration in milliseconds.
Here’s a code snippet demonstrating its use:
#include <iostream>
#include <thread>
#include <chrono>
int main() {
std::cout << "Pausing for 1000 milliseconds (1 second)..." << std::endl;
std::this_thread::sleep_for(std::chrono::milliseconds(1000));
std::cout << "Resumed execution!" << std::endl;
return 0;
}
Understanding Sleep Functionality
What is a Sleep Function?
A sleep function is a programming construct that allows a program to pause its execution for a specified amount of time. This functionality is crucial for various reasons, including synchronization, timing events, and managing resource usage.
Why Use Delays in C++?
Introducing delays in C++ programs can significantly enhance user experiences. For example, in graphical user interface (GUI) applications, delays can make transitions smoother, allowing users to perceive changes more naturally. Delays are also helpful in simulating processes or timed events in games or simulations, giving a more realistic feel to applications. Moreover, by preventing premature execution of certain code blocks, delays help in avoiding resource over-utilization, especially while waiting for resources to become available.
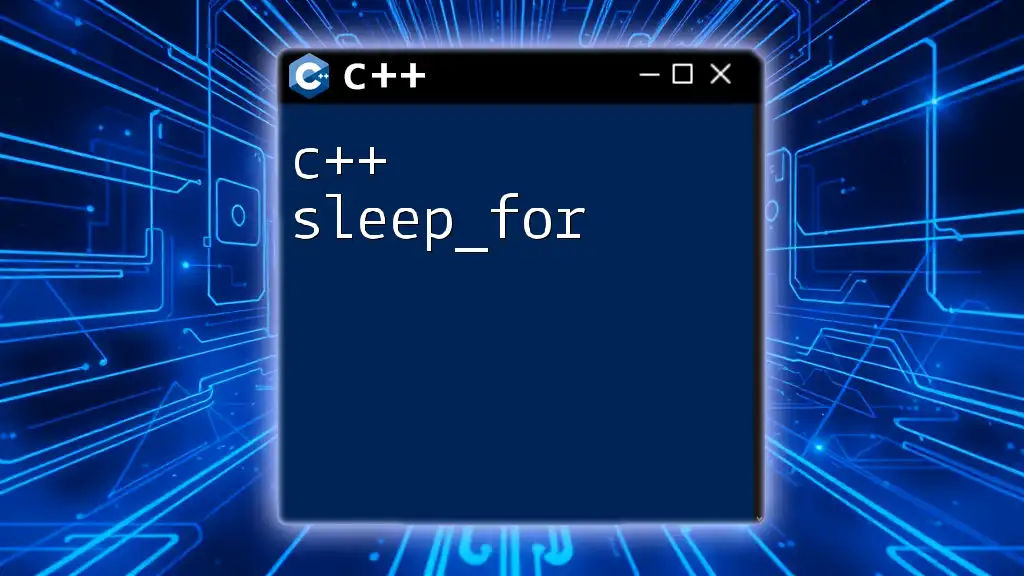
C++ Sleep Milliseconds: The Basics
What is Milliseconds?
A millisecond (ms) is one thousandth of a second, a critical measurement in programming when precise timing is necessary. Using milliseconds can ensure that events occur exactly when intended, which is vital for applications requiring synchronization or timed responses.
Overview of `sleep` in C++
In C++, delays can be managed using functions from the `<thread>` library. This library abstracts away platform-specific implementation details and provides a standardized way to handle delays across different operating systems.
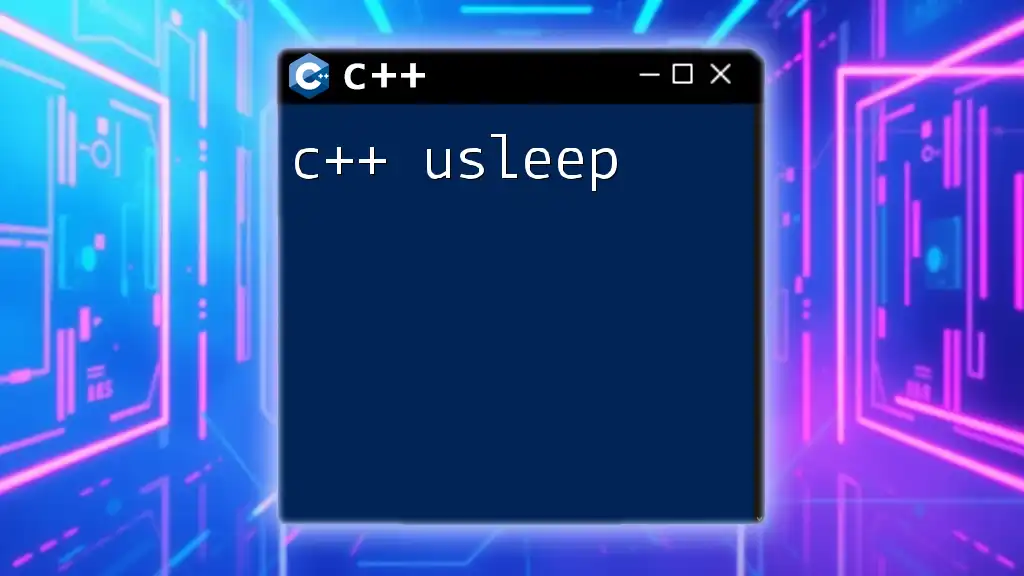
Using `std::this_thread::sleep_for`
How to Use `sleep_for` in C++
The `std::this_thread::sleep_for` function makes it straightforward to pause the execution of a thread for a specified duration. The syntax is clear and allows the programmer to specify the duration in various time units.
Here is how you can implement it:
#include <iostream>
#include <thread>
#include <chrono>
int main() {
std::cout << "Sleep for 1000 milliseconds...\n";
std::this_thread::sleep_for(std::chrono::milliseconds(1000));
std::cout << "Done sleeping!\n";
return 0;
}
Explanation of the code:
- The `<iostream>`, `<thread>`, and `<chrono>` libraries are included to ensure access to the necessary functions and types.
- In the `main()` function, a message is printed to indicate the start of the sleep period.
- The `sleep_for` function is called with `std::chrono::milliseconds(1000)`, which pauses execution for 1000 milliseconds (or 1 second).
- After the sleep period, the program outputs a message indicating it has resumed execution.
When to Use `sleep_for`
The `sleep_for` function is particularly useful in scenarios where you want to delay execution without needing an exact wakeup time. For instance, during loops handling events, it can be used to moderate the processing speed, preventing the program from consuming too much CPU when waiting for user input.
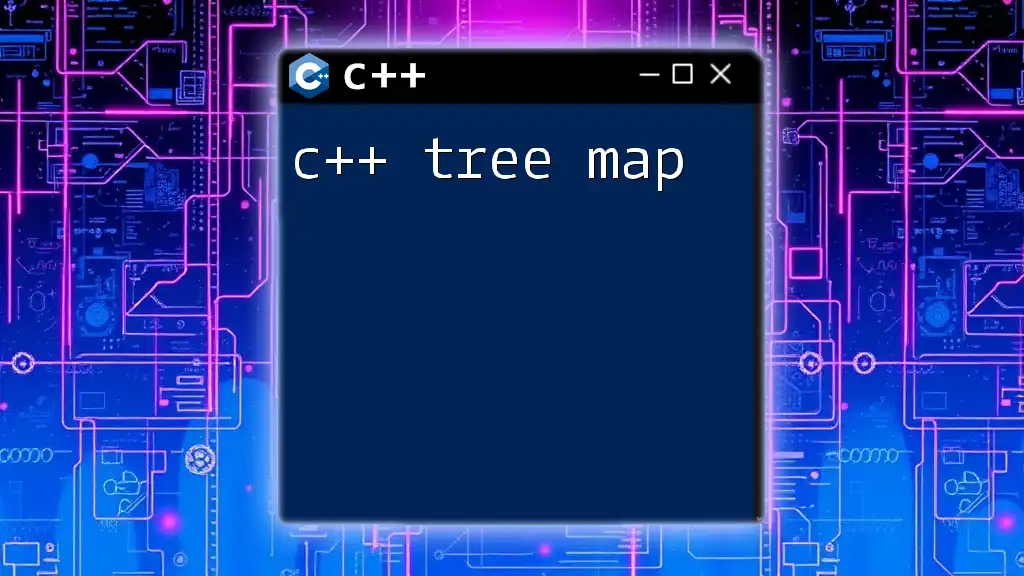
Using `std::this_thread::sleep_until`
Overview of `sleep_until`
The `std::this_thread::sleep_until` function allows you to pause the execution of a program until a certain time point is reached, rather than for a preset duration. This can be especially beneficial when you need to synchronize actions with an external event or a specific point in time.
Example Code Snippet:
#include <iostream>
#include <thread>
#include <chrono>
int main() {
auto wake_time = std::chrono::steady_clock::now() + std::chrono::milliseconds(500);
std::cout << "Sleeping until specified time...\n";
std::this_thread::sleep_until(wake_time);
std::cout << "Woke up!\n";
return 0;
}
Code breakdown:
- Just like the previous example, this snippet includes necessary libraries and defines a `main()` function.
- Here, a specific wake-up time is calculated by adding 500 milliseconds to the current time using `std::chrono::steady_clock::now()`.
- The `sleep_until` function is called with this calculated time, causing the program to pause until the specified moment.
- Finally, a message confirming that the program has "woken up" is printed.
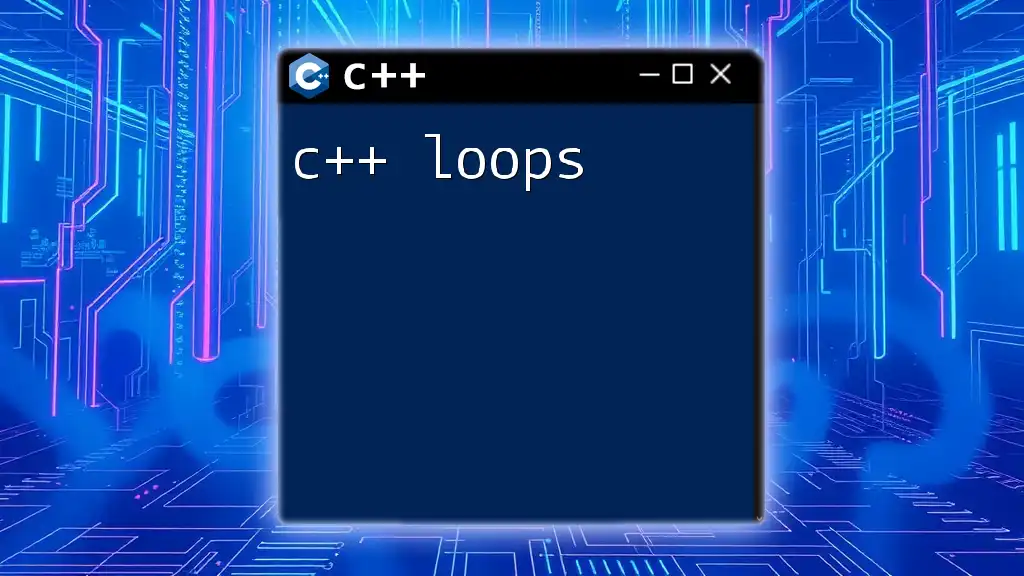
Best Practices for Using Sleep in C++
Avoiding Overuse of Sleep
While using sleep functions can offer benefits, excessive use can lead to performance bottlenecks and user frustration. It is essential to minimize the number of calls to `sleep` in your code and only use it when necessary, maintaining an efficient flow throughout the application.
Timing Accuracy Considerations
Timing accuracy is critical in many applications, and several factors can impact its effectiveness. Operating system scheduling delays, hardware differences, and the specifics of the implementation can introduce variability in timing. To ensure accurate delays, benchmark and monitor performance metrics in your application, making adjustments as necessary.
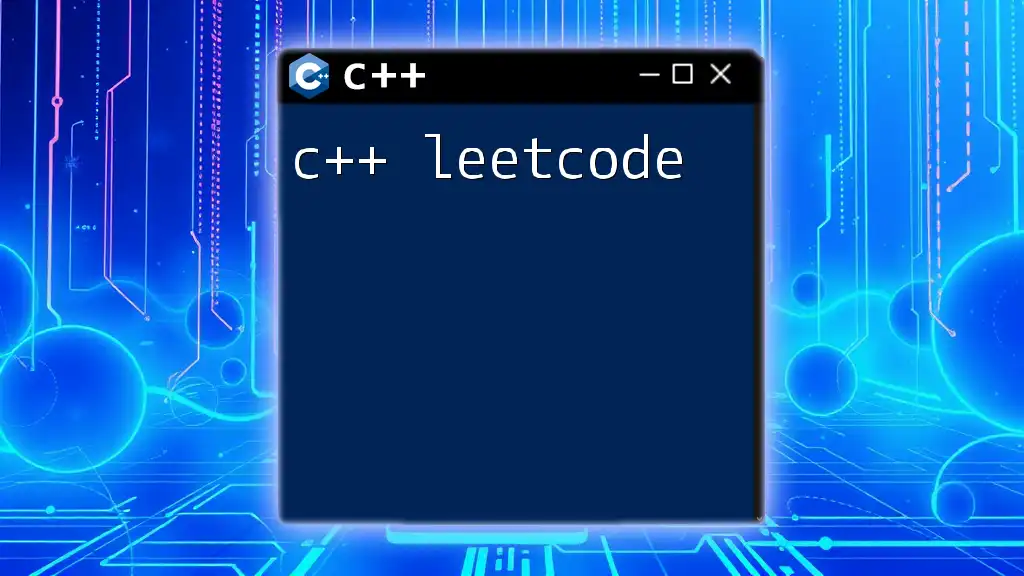
C++ Sleep in Real-world Applications
Examples of Applications that Use Sleep
- Games and Simulations: In game development, sleep functions can be used to create realistic gameplay experiences, pausing for animations or simulating time between events.
- Network Programming: In server applications, sleep functions can help manage request rates or ensure that retries occur at regulated intervals.
- User Interfaces: In UI applications, delays can be utilized while loading data, keeping the user informed with progress indicators.
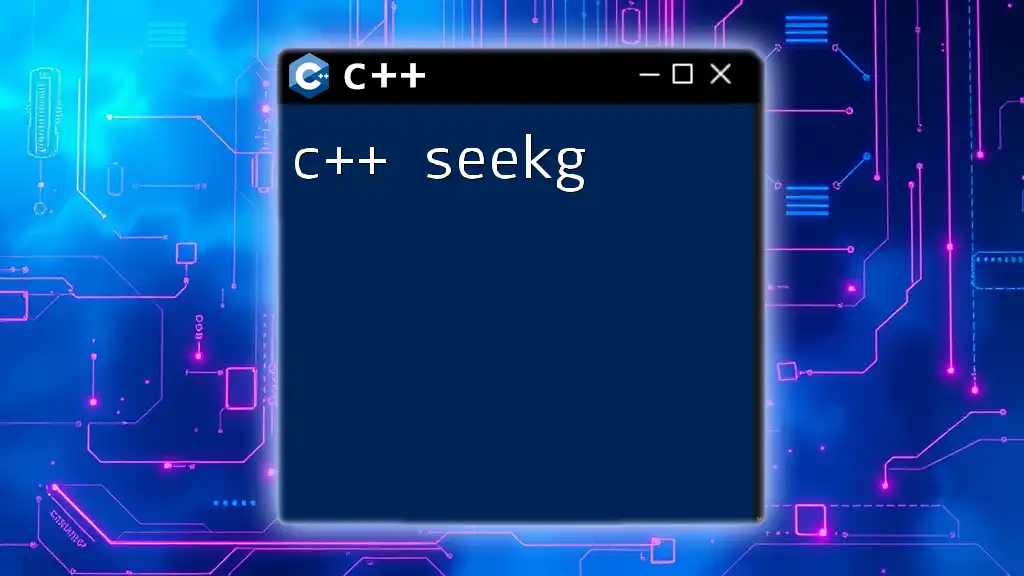
Troubleshooting Common Issues
Common Problems with Sleep Functions
One of the most common issues when using sleep functions is the challenge of timing consistency. If your application relies on precise timing (for instance, in game loops), the behavior of the `sleep` function can be unpredictable. Additionally, if multiple threads use `sleep`, careful consideration must be given to synchronization.
Performance Considerations
Using sleep effectively often involves a balance between code readability and system performance. Consider alternatives to sleep when possible, such as asynchronous programming approaches, which can provide more efficient handling of timing or delays without blocking execution threads.
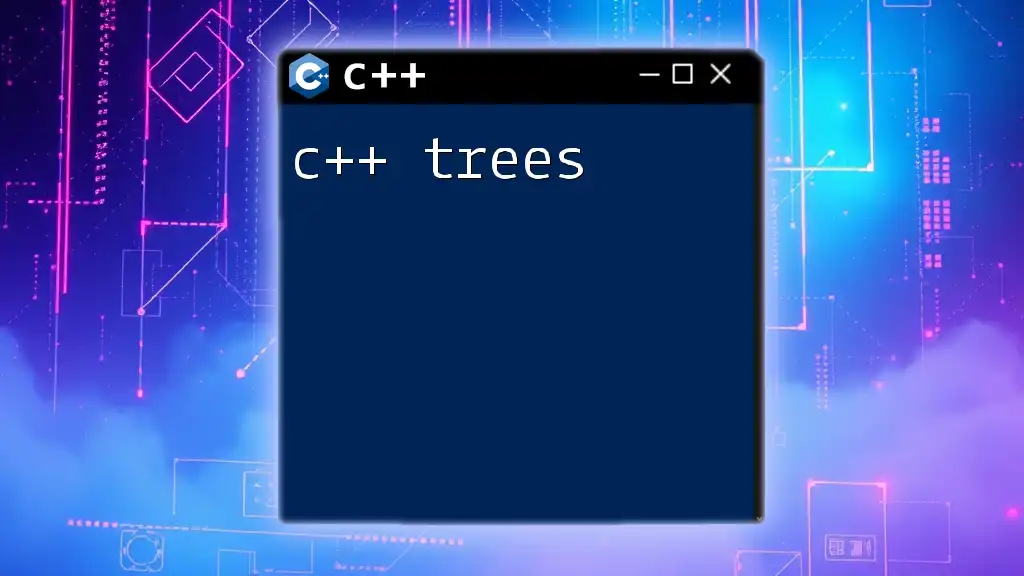
Conclusion
The C++ sleep ms functionalities provide a straightforward means to handle timing in applications, offering developers the ability to create more timed, user-friendly experiences. Understanding the nuances of `sleep_for` and `sleep_until`, and applying the best practices outlined above, will help in implementing effective delay mechanisms in your C++ applications.