An STL map in C++ is an associative container that stores elements in key-value pairs, allowing for efficient retrieval based on the unique keys.
Here's a simple code snippet demonstrating how to use an STL map:
#include <iostream>
#include <map>
int main() {
std::map<std::string, int> age;
age["Alice"] = 30;
age["Bob"] = 25;
for (const auto& entry : age) {
std::cout << entry.first << ": " << entry.second << std::endl;
}
return 0;
}
What is a Map in C++ STL?
A map in C++ STL is a key-value pair associative container that stores unique keys, each mapped to a specific value. It is an efficient way to manage associations between two elements, allowing for quick retrieval and manipulation of data. The most significant characteristics of a C++ STL map include:
-
Unique Keys: Each key in a map must be unique. If you attempt to insert a second entry with the same key, the existing value associated with that key will be overwritten.
-
Ordered: Maps are sorted by keys. The elements in a map are always arranged in a specific order, typically according to the key's value. This makes searching for keys more efficient.
-
Value Access by Key: The primary method of accessing the values in a map is through their associated keys, making lookups quick and simple.
Maps are distinct from other associative containers in STL, such as `unordered_map`. While an `unordered_map` does not guarantee any specific order and is generally faster for key lookups due to its hashed nature, a `map` provides a sorted state.
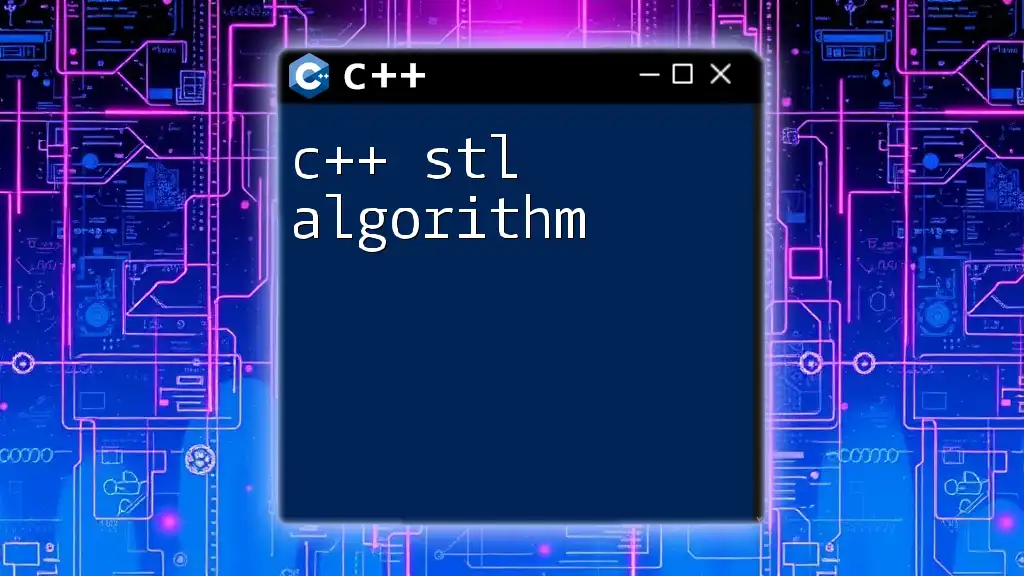
How to Include the Map Header
To use the C++ STL map in your programs, you need to include the appropriate header file. The necessary header file is:
#include <map>
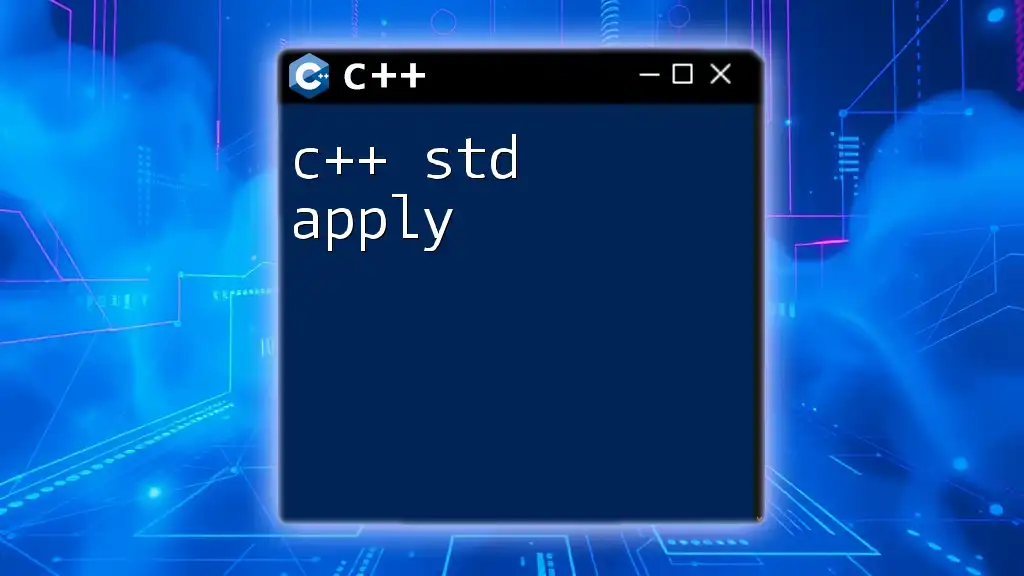
Declaring a Map
Syntax for Map Declaration
To declare a map, follow the basic syntax:
std::map<key_type, value_type> mapName;
Examples of Map Declaration
For example, if you want to create a map with integer keys and string values, you can declare it like this:
std::map<int, std::string> myMap;
If you wish to use custom class types as values, the declaration remains similar, just replacing `value_type` with your custom class.
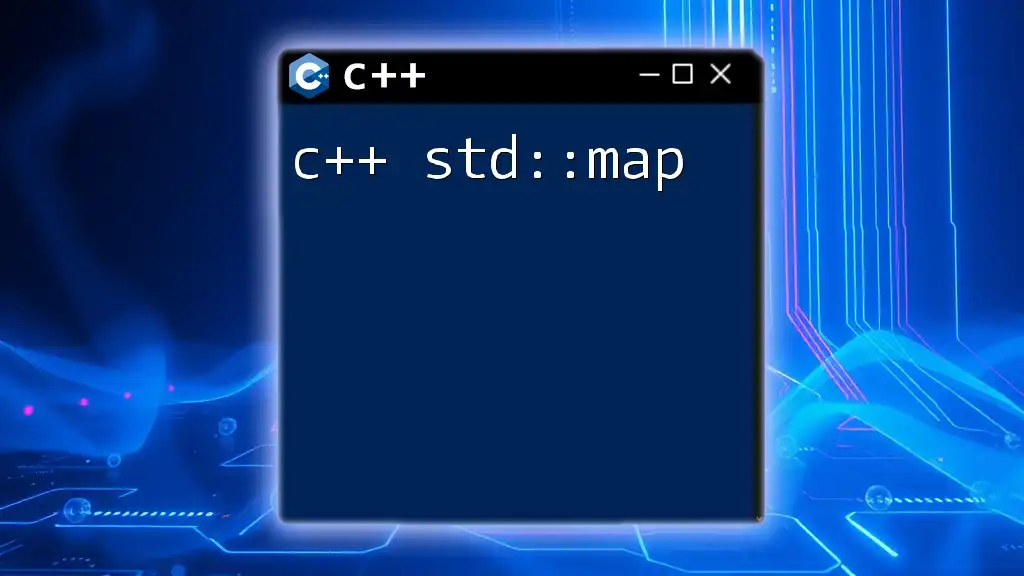
Inserting Elements into a Map
Using Insert Function
You can insert elements into a map using the `insert` function, which takes a `pair`. Here's an example:
myMap.insert(std::make_pair(1, "Apple"));
Using the Subscript Operator
An alternative and commonly used method for inserting elements is the subscript operator. This allows you to directly assign a value to a key:
myMap[2] = "Banana";
If the key does not exist, it will be created; if it does, its value will be updated.
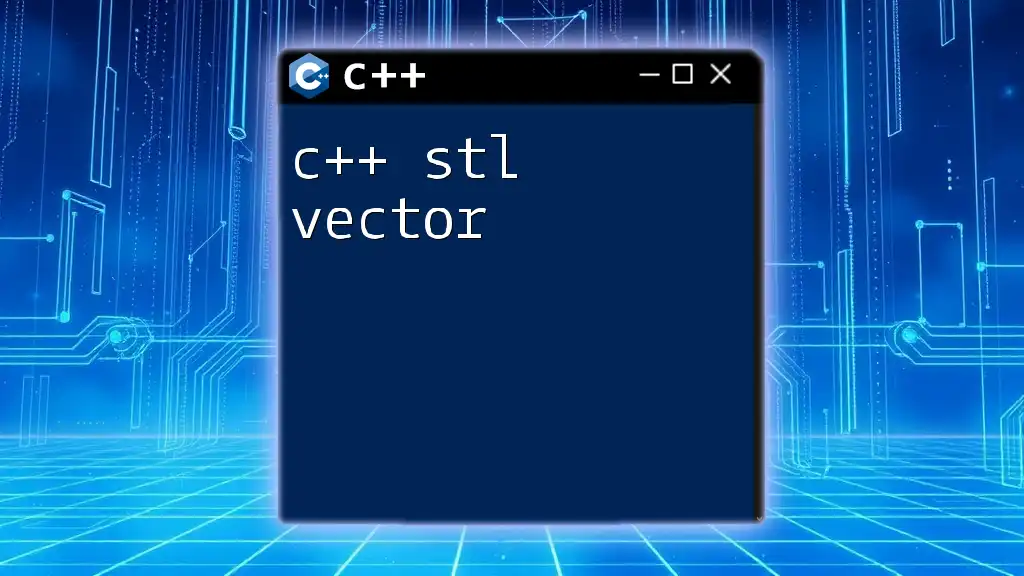
Accessing Elements in a Map
Finding Elements Using Keys
To access elements in the map, you can directly use the key. For instance:
std::cout << myMap[1]; // Outputs: Apple
Checking for Key Existence
Before accessing a key, it's a good practice to check whether it exists, which can be accomplished using the `find()` method:
if (myMap.find(3) != myMap.end()) {
std::cout << "Key found!";
}
If the key does not exist, `find()` will return `end()`, indicating that the lookup was unsuccessful.
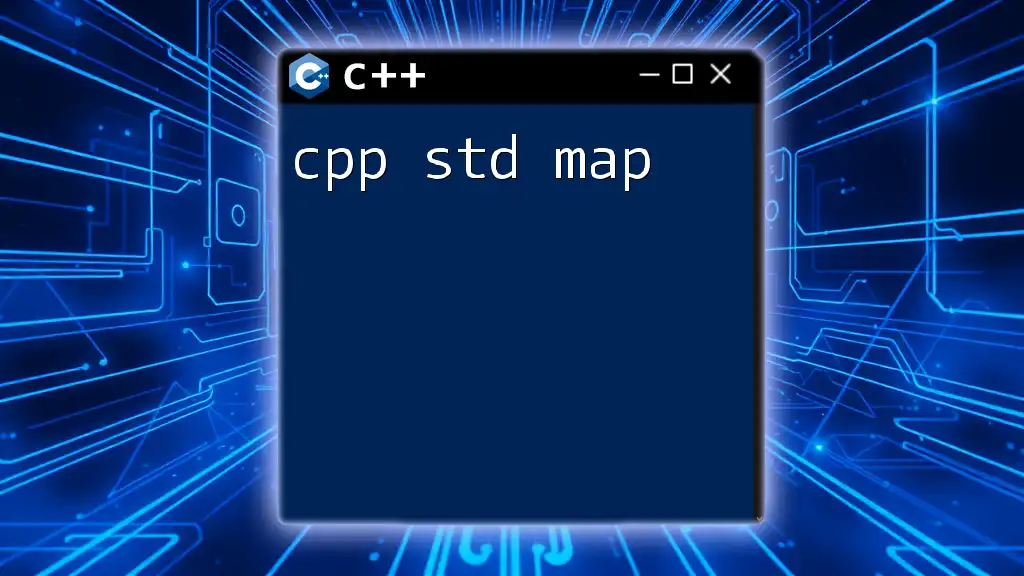
Iterating Through a Map
Using Range-Based For Loop
You can efficiently iterate over the entire map using a range-based for loop, which allows you to print out the key-value pairs easily:
for (const auto& pair : myMap) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
Using Iterators
Declaring Iterators
Alternatively, you can explicitly use iterators to navigate through the map:
std::map<int, std::string>::iterator it;
Example of Iterating with Iterators
Here’s how to iterate using iterators to print the key-value pairs:
for (it = myMap.begin(); it != myMap.end(); ++it) {
std::cout << it->first << ": " << it->second << std::endl;
}
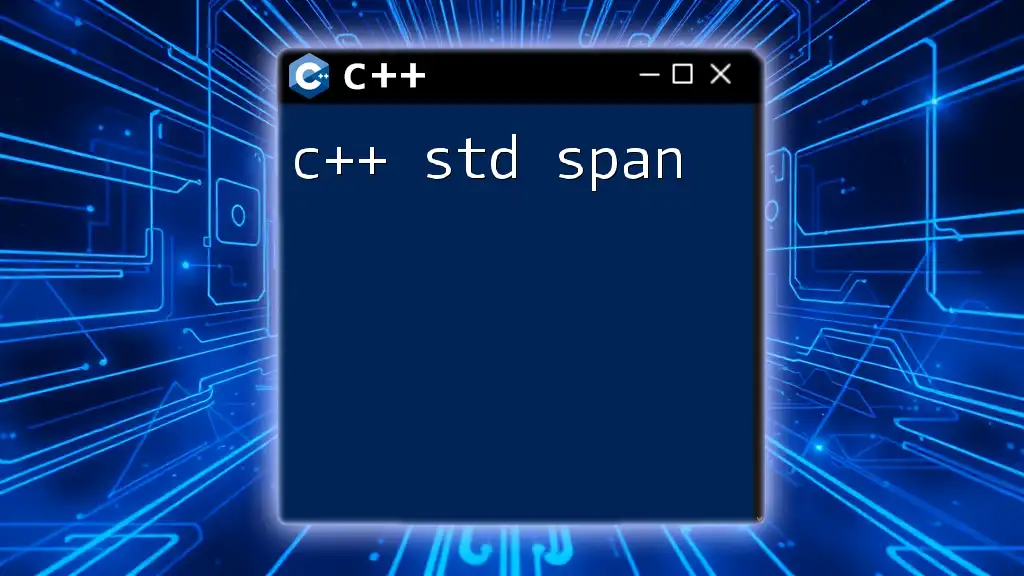
Modifying Map Elements
Updating values in a map is straightforward. You can modify the value associated with an existing key:
myMap[1] = "Grape"; // Updates the value associated with key 1
This approach makes map elements very flexible, allowing for easy adjustments as needed.
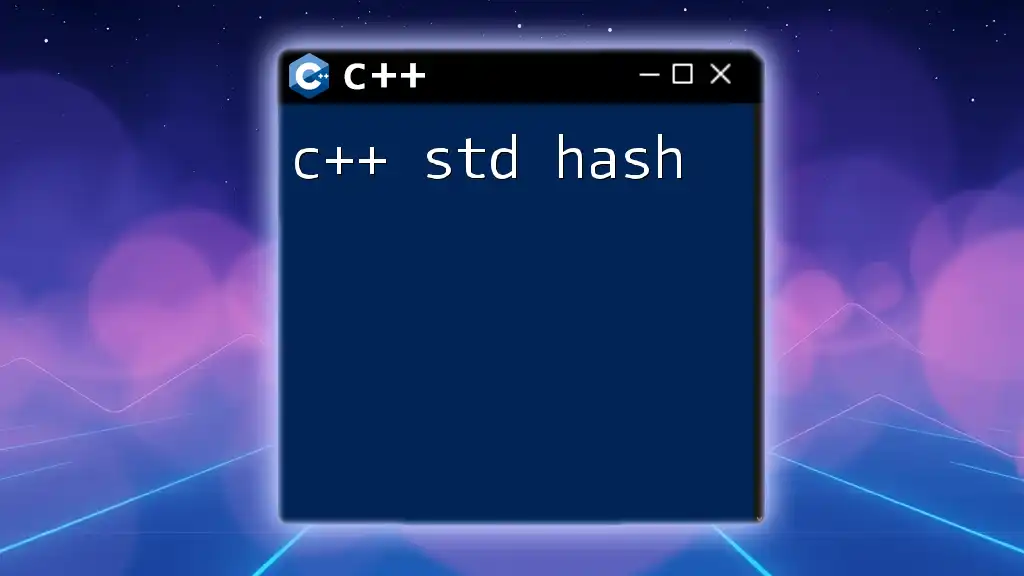
Deleting Elements from a Map
Removing Elements Using Erase Function
You can delete elements from a map using the `erase` method, which will remove the entry associated with a specific key:
myMap.erase(1); // Removes the element with key 1
Clearing the Map
If you want to remove all elements from the map, you can use the `clear` function. This will empty the map while keeping it available for further use:
myMap.clear();
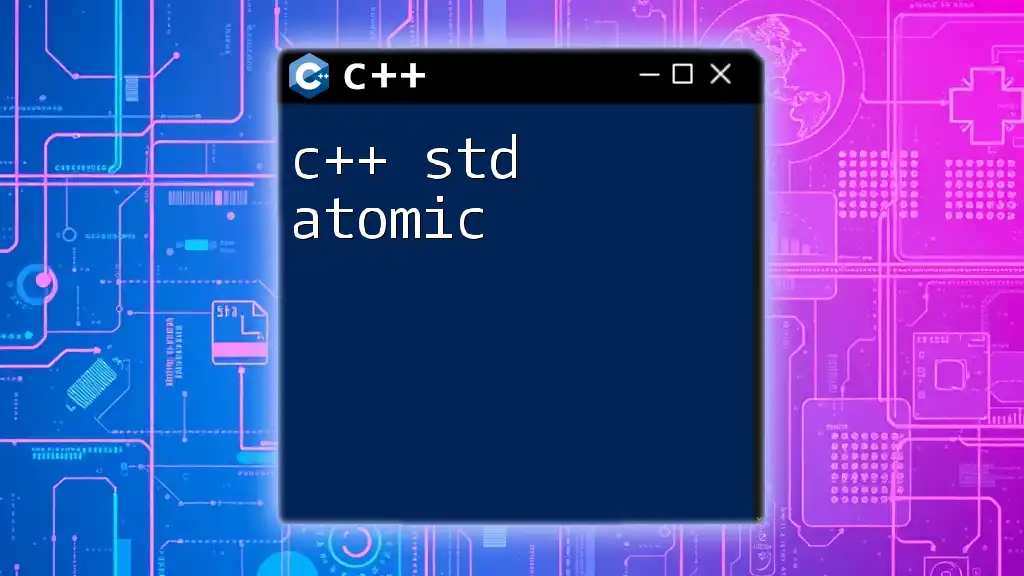
Map Functions and Member Functions
Size and Empty Functions
To check the size of the map, you can use the `size()` function. Additionally, to verify if the map is empty, you can use `empty()`:
if (myMap.empty()) {
std::cout << "The map is empty.";
}
Swap Function
A useful member function in C++ STL maps is `swap`, which exchanges the contents between two maps:
std::map<int, std::string> anotherMap;
myMap.swap(anotherMap);
This allows for efficient data handling without needing to copy large amounts of data.
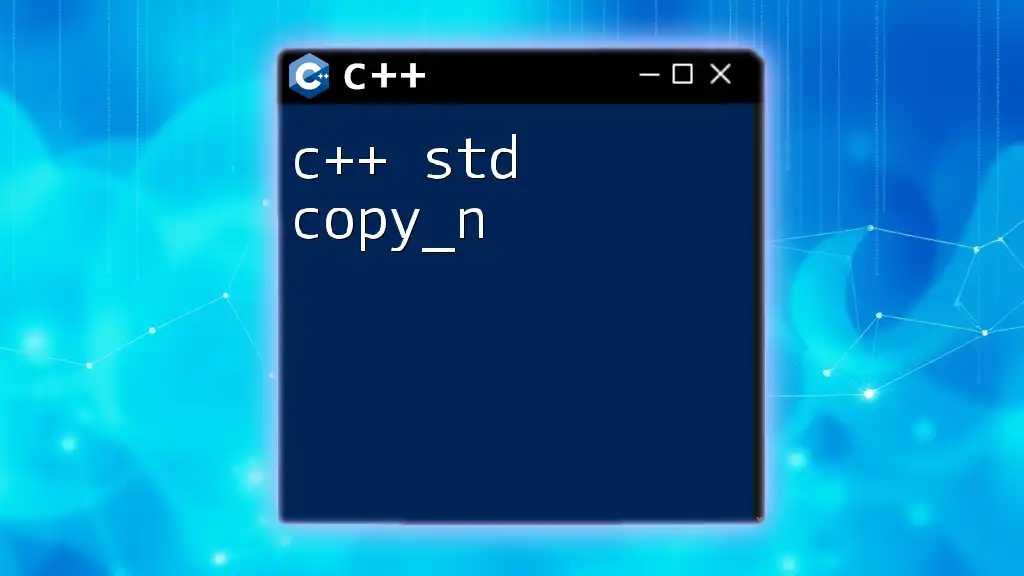
Use Cases for STL Map in C++
Use Case Scenarios
The C++ STL map is versatile and has various applications:
-
Counting Occurrences of Words: Maps can be used to efficiently store and count the frequencies of words in a text by treating the words as keys and their counts as values.
-
Implementing a Phone Book: A map provides a simple interface to link names or identifiers with phone numbers.
-
Efficiently Managing Index-Keyed Information: When you need to manage data indexed by unique keys, such as user IDs or product codes, maps greatly simplify this task.
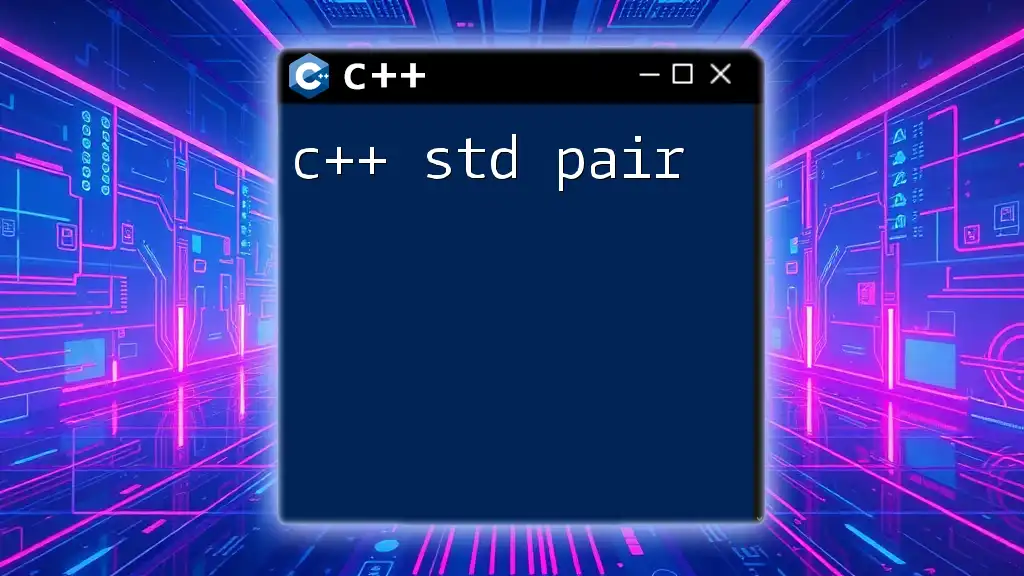
Performance Considerations
Time Complexity
While using a C++ STL map, it's essential to understand the efficiency of operations. The time complexities are as follows:
-
Insertion: Average O(log n), which can be slower than other data structures but ensures order.
-
Deletion: Average O(log n).
-
Finding: Average O(log n), making it efficient for lookups compared to other containers that might offer no order.
These performance characteristics make maps suitable for situations where key-value relationships and ordered iterations are essential.
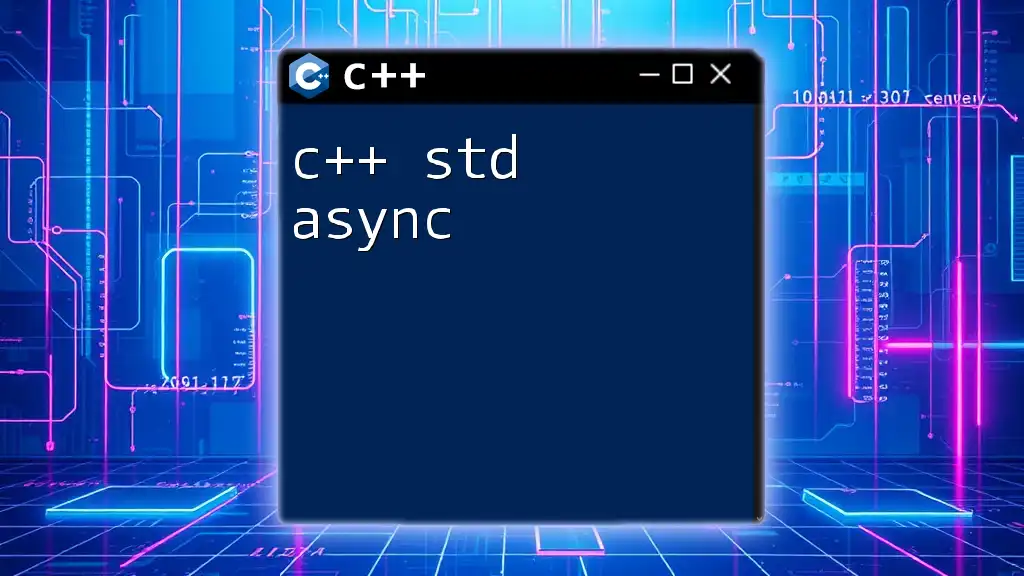
Practical Examples
Example: Creating a Simple Phone Book
Here's a straightforward implementation of a phone book using a C++ STL map:
std::map<std::string, std::string> phoneBook;
phoneBook["John"] = "12345";
phoneBook["Jane"] = "67890";
This allows for easy retrieval of phone numbers using names as keys.
Example: Counting Word Frequencies
A more complex application might be counting word frequencies in a paragraph:
std::map<std::string, int> wordCount;
std::string word;
// Sample reading words and counting occurrences
while (std::cin >> word) {
wordCount[word]++;
}
This shows how you can dynamically build a frequency map as you process input.
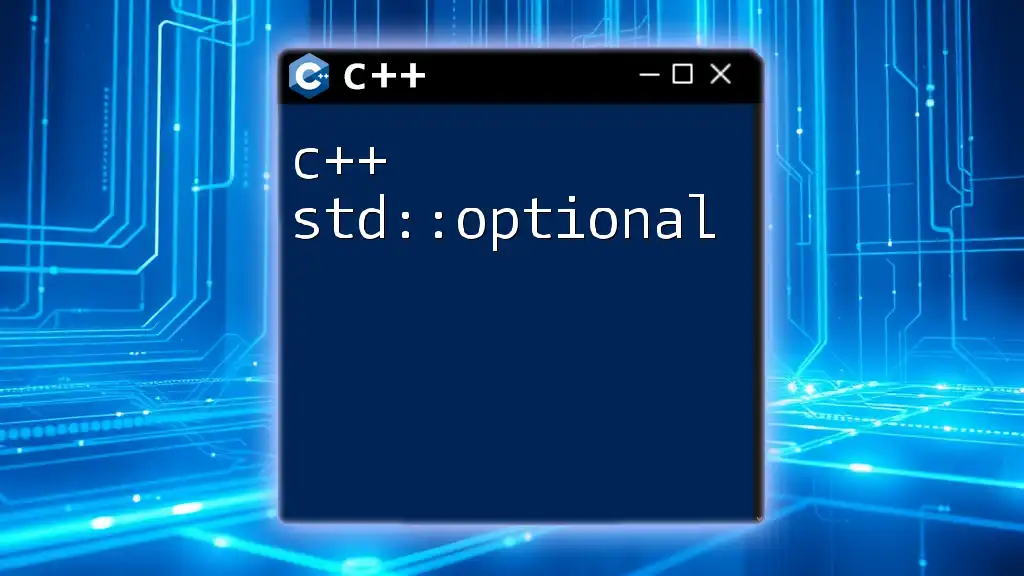
Common Mistakes to Avoid
When using C++ STL maps, it’s crucial to be aware of potential pitfalls:
-
Forgetting to Check for Key Existence: Accessing non-existing keys without checking can lead to unexpected behavior or errors.
-
Using a Map with Mutable Keys: Since keys must remain unique and consistent, avoid using mutable objects as keys; otherwise, you risk invalidating the integrity of the map.
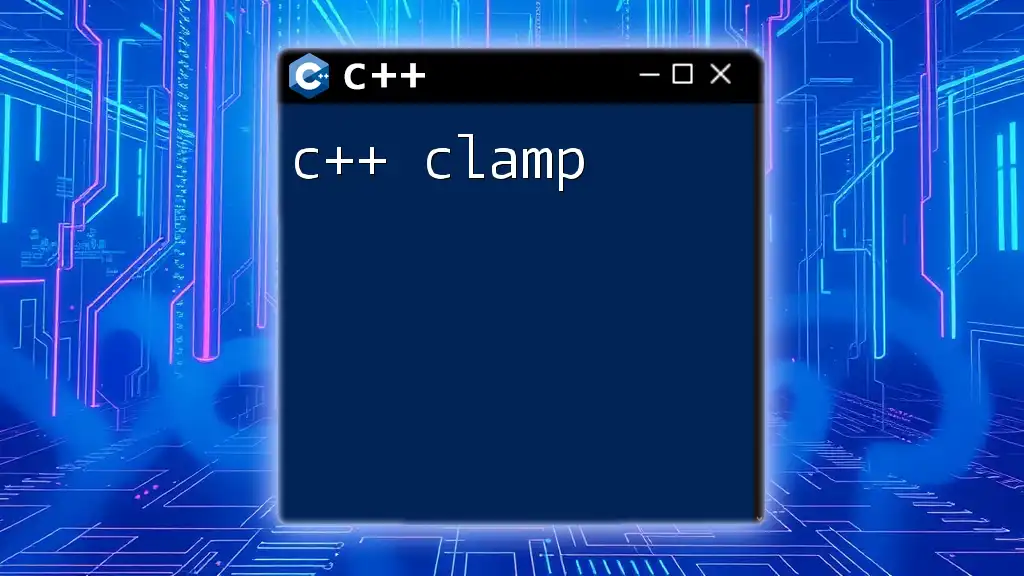
Conclusion
In summary, the C++ STL map is a powerful and versatile container used for managing key-value pairs in an ordered manner. Its distinct advantages, such as unique keys, automatic sorting, and efficient access times, make it a staple in C++ programming. Engaging with real-world use cases will enhance your understanding and skill in utilizing maps effectively.
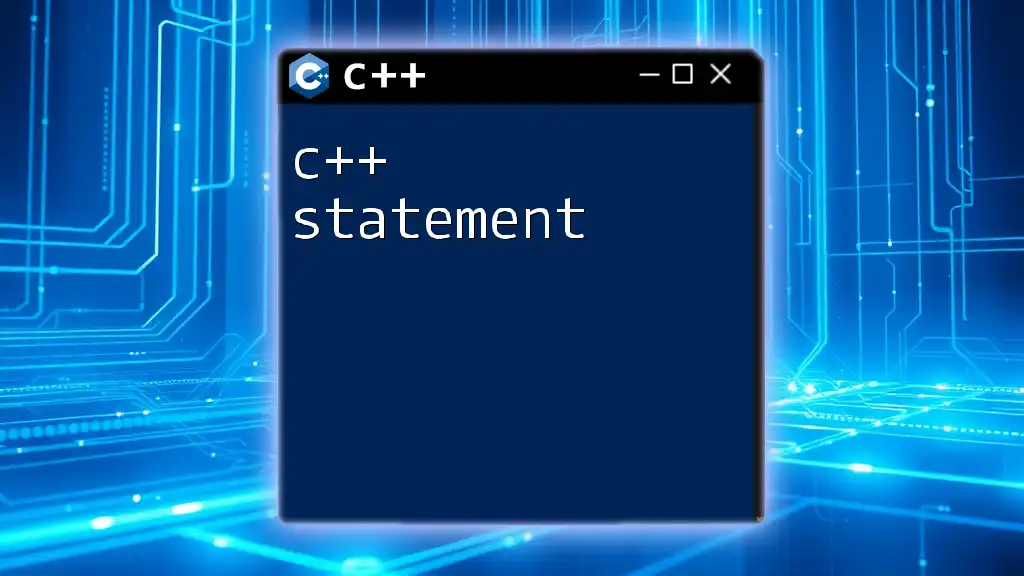
Call to Action
For further learning, consider exploring advanced C++ STL topics, including other associative containers, or practice with projects that incorporate maps in various data management scenarios. If you have questions or need clarification, feel free to reach out!