An abstract class in C++ is a class that cannot be instantiated on its own and is meant to be subclassed, ensuring that derived classes implement certain methods defined as pure virtual functions.
Here's an example code snippet:
class AbstractClass {
public:
virtual void pureVirtualFunction() = 0; // Pure virtual function
};
What is an Abstract Class in C++?
An abstract class is a class that acts as a blueprint for other classes. It cannot be instantiated on its own and must be inherited by other classes. Abstract classes are an essential concept in C++, particularly in object-oriented programming (OOP), as they facilitate the design of systems that require a clear interface and promote code reuse.
Characteristics of Abstract Classes
-
Contains At Least One Pure Virtual Function: A pure virtual function is declared by assigning `0` to the function signature. This signals that the function must be overridden in derived classes.
-
Cannot Be Instantiated: You cannot create an object of an abstract class directly. Instead, it is intended to be subclassed for creating concrete objects.
Here’s a basic structure to illustrate an abstract class in C++:
class AbstractClass {
public:
virtual void pureVirtualFunction() = 0; // Pure virtual function
};
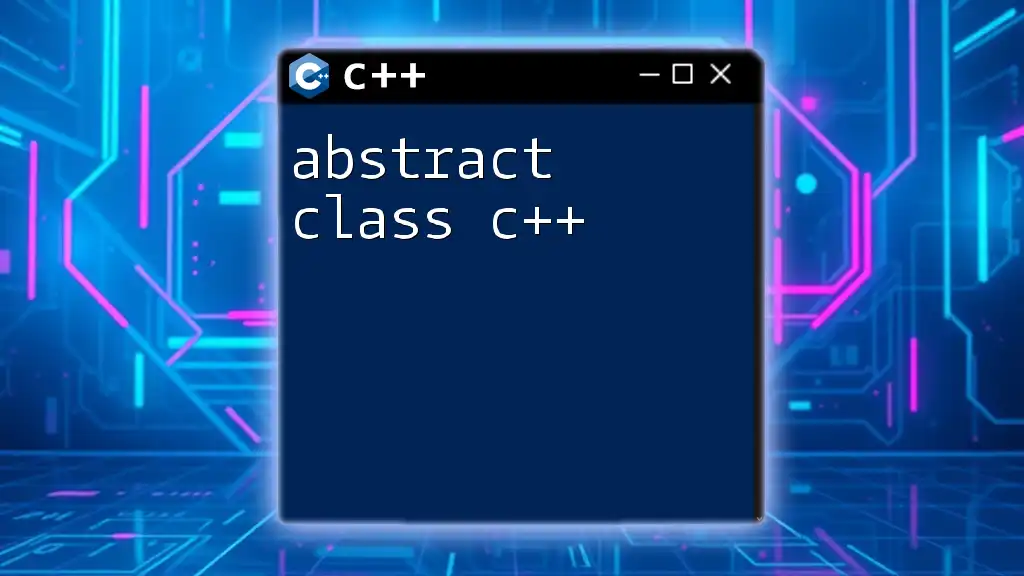
Understanding Pure Virtual Functions
What are Pure Virtual Functions?
A pure virtual function is a virtual function that has no definition in the class where it is declared. This means it serves as a placeholder that forces derived classes to provide specific implementations.
The syntax to declare a pure virtual function is as follows:
virtual void functionName() = 0; // Pure virtual function declaration
Difference Between Virtual and Pure Virtual Functions
While both virtual and pure virtual functions are designed to be overridden in derived classes, there are critical differences between them:
- Virtual Function: Has a definition in the class where it is declared, allowing derived classes the option to override it.
- Pure Virtual Function: Lacks a definition; the derived class must override it to remain a concrete class.
Here's an example illustrating the differences:
class Base {
public:
virtual void virtualFunc() {
// Definition provided; can be overridden
}
virtual void pureVirtualFunc() = 0; // Must be overridden in derived classes
};
class Derived : public Base {
public:
void pureVirtualFunc() override {
// Required implementation
}
};
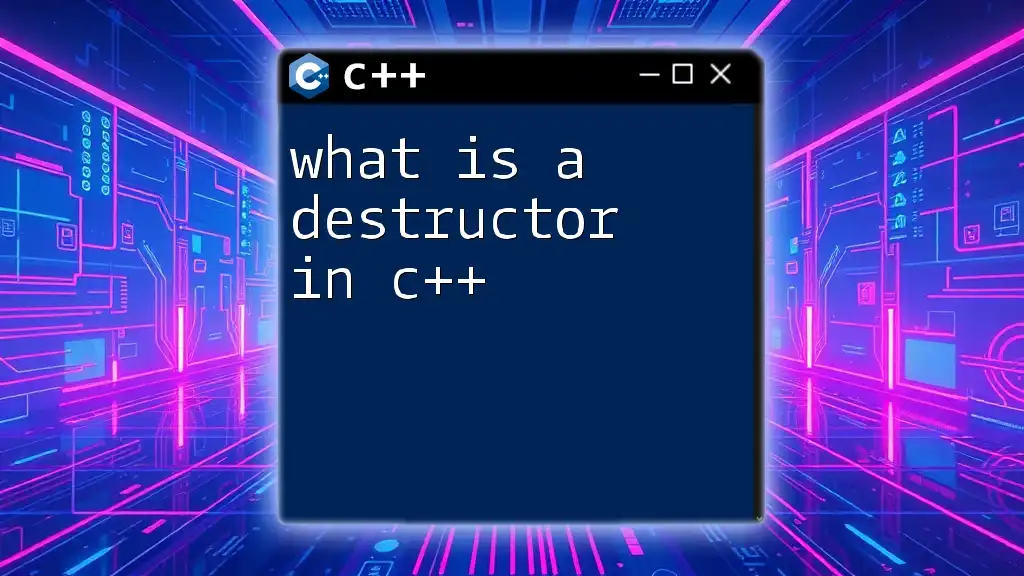
Creating an Abstract Class in C++
Steps to Define an Abstract Class
To create an abstract class, follow these steps:
- Declare the class.
- Define one or more pure virtual functions within the class.
- Derive concrete classes from the abstract class and implement the pure virtual functions.
Here's a code snippet demonstrating the creation of an abstract class:
class Animal {
public:
virtual void sound() = 0; // Pure virtual function
};
class Dog : public Animal {
public:
void sound() override {
std::cout << "Bark" << std::endl;
}
};
class Cat : public Animal {
public:
void sound() override {
std::cout << "Meow" << std::endl;
}
};
Practical Example of an Abstract Class
Let’s model a simple shape hierarchy using an abstract class called Shape, which will have derived classes like Circle and Rectangle.
class Shape {
public:
virtual double area() = 0; // Pure virtual function for area
};
class Circle : public Shape {
private:
double radius;
public:
Circle(double r) : radius(r) {}
double area() override {
return 3.14 * radius * radius; // Implementation specific to Circle
}
};
class Rectangle : public Shape {
private:
double width, height;
public:
Rectangle(double w, double h) : width(w), height(h) {}
double area() override {
return width * height; // Implementation specific to Rectangle
}
};
In the above example, both `Circle` and `Rectangle` provide their implementations for the `area()` function, effectively utilizing the abstract class Shape as a blueprint.
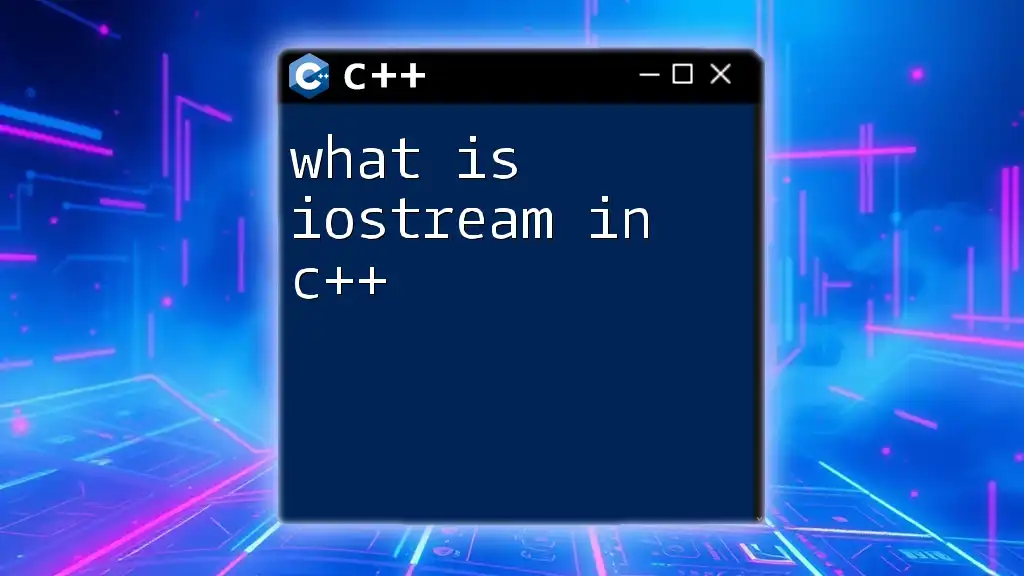
Advantages of Using Abstract Classes in C++
Using abstract classes brings several advantages:
-
Promotes Code Reusability: By enforcing a common interface, different classes can share the same base functionalities without duplication.
-
Facilitates a Cleaner Design: Abstract classes help to separate interface from implementation, leading to cleaner and more maintainable code.
-
Supports Polymorphism: They enable a form of polymorphism, where the base class type can refer to derived class objects, allowing for flexible and dynamic code.
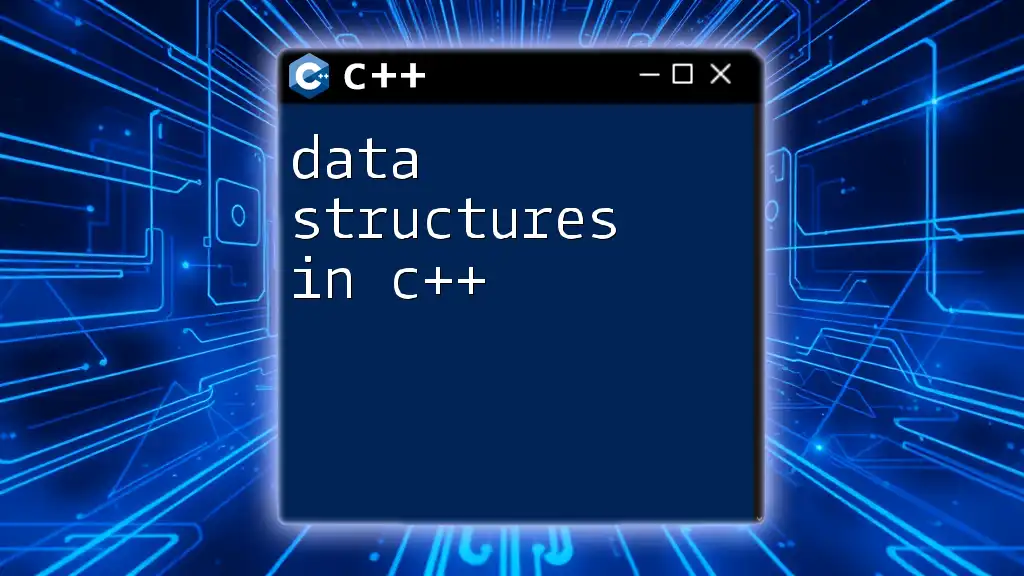
Abstract Classes vs. Interfaces in C++
While abstract classes and interfaces are often discussed together, they serve different purposes:
-
Abstract Classes: Can have member variables and methods with implementations. They may contain both pure virtual and regular functions.
-
Interfaces: In C++, interfaces are typically pure abstract classes; they only contain pure virtual functions without any implementation or member variables.
When to use abstract classes versus interfaces often depends on your design needs. Use abstract classes when you want to share code among subclasses and interfaces when you want to enforce a contract without any shared implementation.
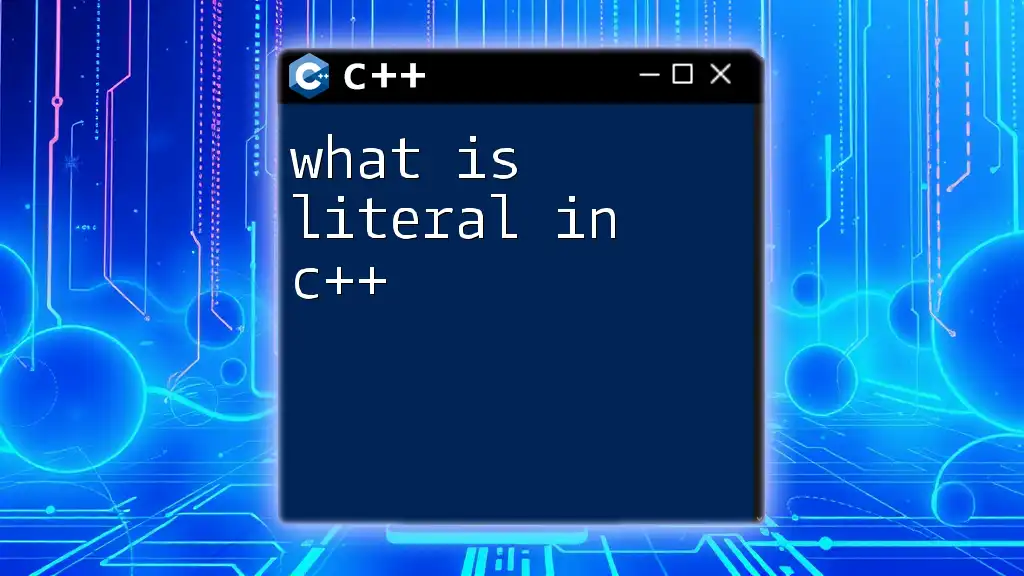
Common Mistakes to Avoid With Abstract Classes
While working with abstract classes, programmers may encounter some common pitfalls:
-
Failing to Implement Pure Virtual Functions in Derived Classes: If a derived class does not implement all pure virtual functions, it remains abstract and cannot be instantiated.
-
Confusing Abstract Classes with Concrete Classes: Understand that abstract classes serve as templates rather than concrete entities.
-
Instantiating Abstract Classes: Attempting to create an instance of an abstract class will lead to compilation errors.
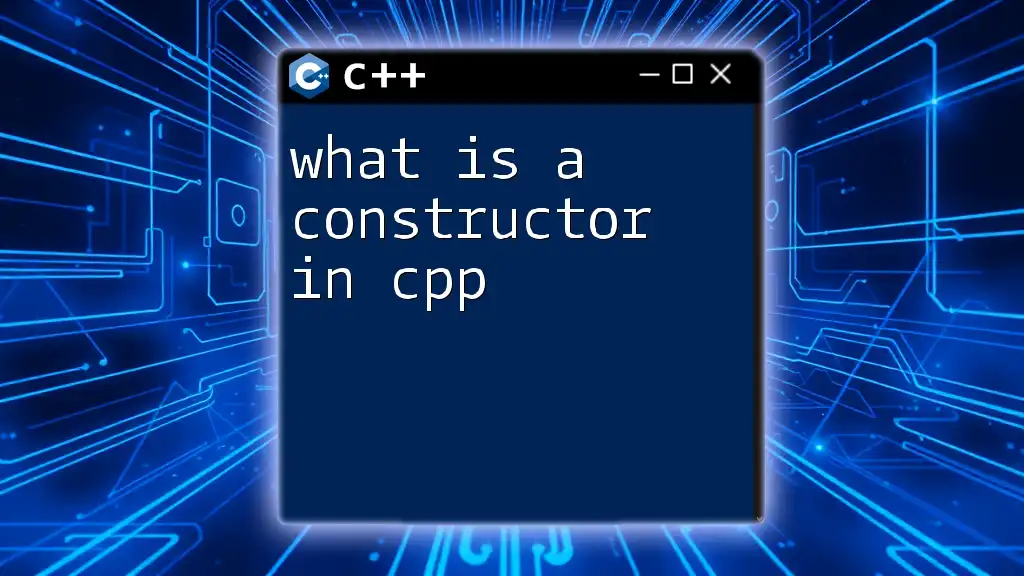
Best Practices for Using Abstract Classes in C++
-
Define Abstract Classes When Necessary: Use them when it makes sense in your design, particularly when several classes will share a similar interface.
-
Maintain a Clean Class Hierarchy: Avoid overly complicated hierarchies to keep the design comprehensible.
-
Document Your Classes and Methods: Clear documentation improves maintainability and helps other developers understand the intended use of your abstract classes.
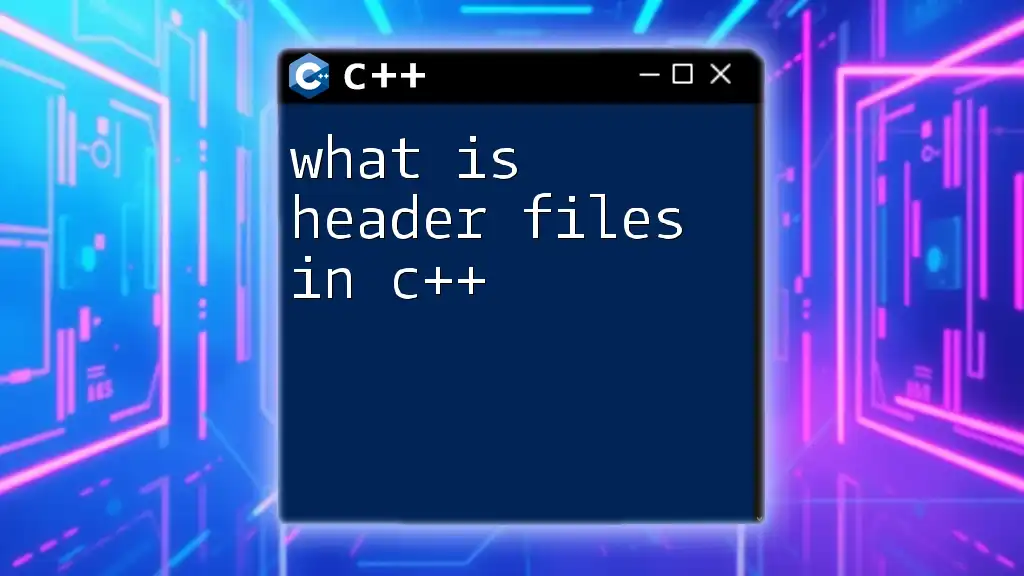
Conclusion
Understanding what is an abstract class in C++ is vital for anyone delving into object-oriented programming. Abstract classes provide a robust mechanism for defining a base interface that enhances code reuse and drives cleaner design. By mastering the concept of abstract classes and implementing them effectively, you can significantly elevate the quality of your C++ programming.
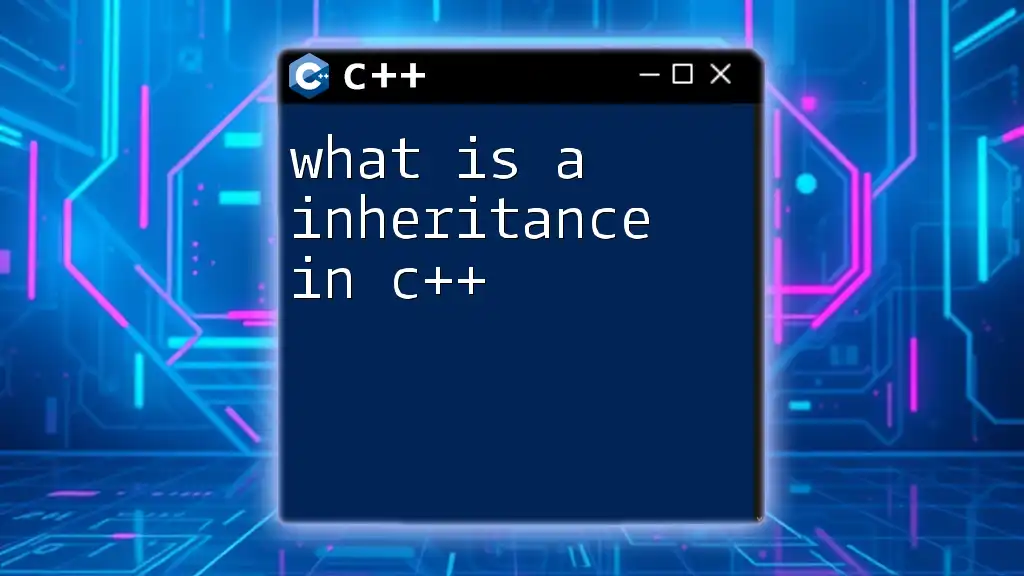
Example Code Snippet Section
Here, you'll find a collection of relevant code snippets that illustrate the concepts discussed in this article:
- Defining an Abstract Class:
class AbstractClass {
public:
virtual void method() = 0; // Pure virtual function
};
- Implementing Derived Classes:
class ConcreteClass : public AbstractClass {
public:
void method() override {
// Concrete implementation
}
};
- Using Polymorphism with Abstract Classes:
AbstractClass* obj = new ConcreteClass();
obj->method(); // Calls ConcreteClass's implementation
delete obj;
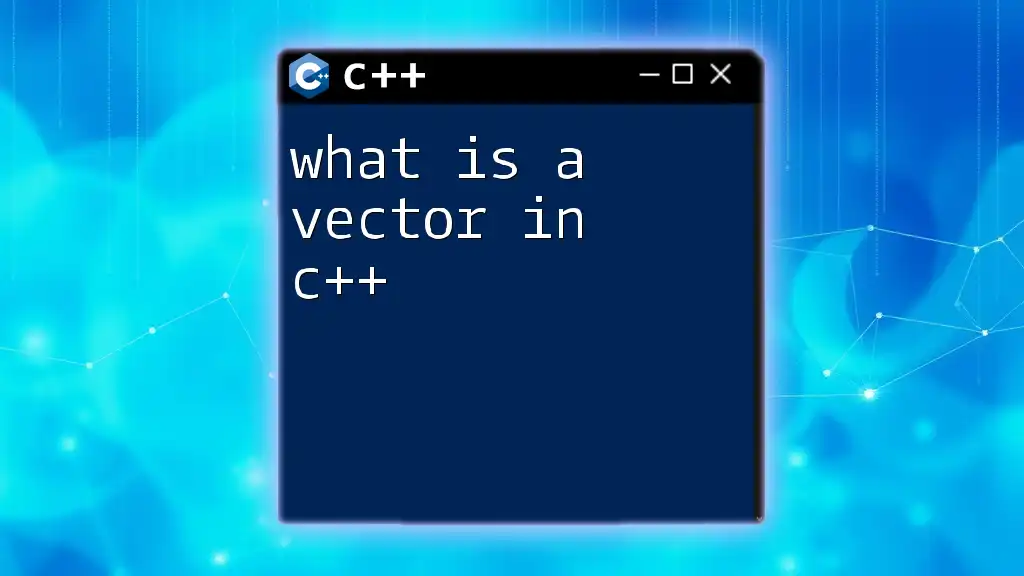
Frequently Asked Questions (FAQs)
-
What is the purpose of an abstract class in C++?
Abstract classes are used to define a common interface that must be followed by derived classes, promoting code reusability and ensuring a clean design. -
Can an abstract class have normal member functions?
Yes, an abstract class can have regular functions alongside pure virtual functions. -
How many pure virtual functions should be in an abstract class?
An abstract class should ideally have at least one pure virtual function, but there can be multiple depending on the requirements of your design.