The factory pattern in C++ is a creational design pattern that allows the creation of objects without exposing the instantiation logic to the client, promoting loose coupling and flexibility.
Here’s a simple code snippet demonstrating the factory pattern in action:
#include <iostream>
#include <memory>
// Product interface
class Product {
public:
virtual void use() = 0;
};
// Concrete Product A
class ConcreteProductA : public Product {
public:
void use() override {
std::cout << "Using Concrete Product A" << std::endl;
}
};
// Concrete Product B
class ConcreteProductB : public Product {
public:
void use() override {
std::cout << "Using Concrete Product B" << std::endl;
}
};
// Factory
class ProductFactory {
public:
static std::unique_ptr<Product> createProduct(char type) {
if (type == 'A') {
return std::make_unique<ConcreteProductA>();
} else if (type == 'B') {
return std::make_unique<ConcreteProductB>();
}
return nullptr;
}
};
int main() {
auto productA = ProductFactory::createProduct('A');
productA->use();
auto productB = ProductFactory::createProduct('B');
productB->use();
return 0;
}
What is the Factory Pattern?
The Factory Pattern is a creational design pattern that provides a way to create objects without specifying the exact class of the object that will be created. This pattern allows for greater flexibility and decouples the code that creates objects from the code that uses those objects. It's particularly useful in scenarios where the client doesn't know beforehand what class of object it will need.
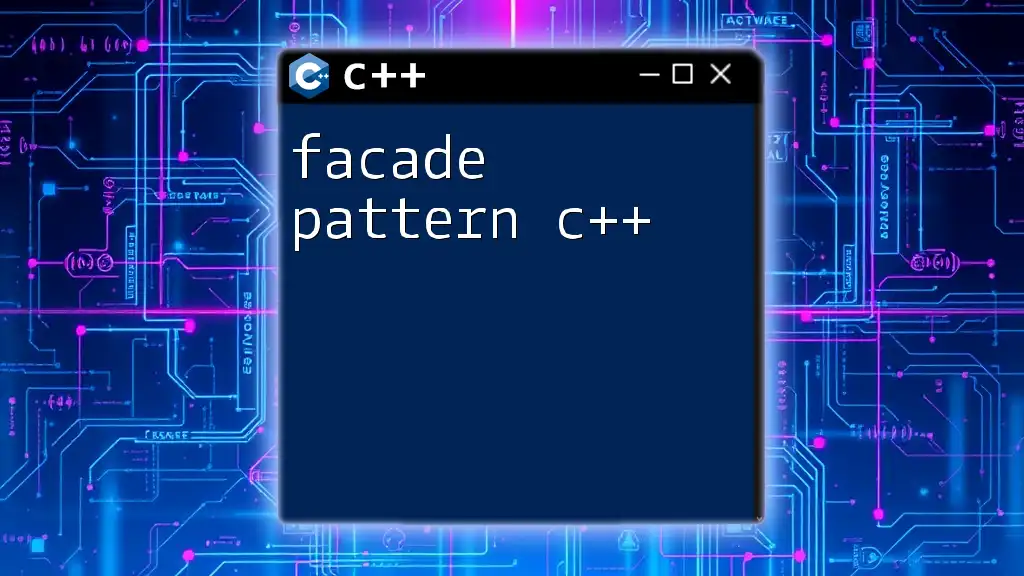
Importance of the Factory Pattern in C++
Using the factory pattern in C++ brings numerous advantages:
-
Encapsulation of Object Creation: It encapsulates the object creation process, leading to a cleaner and more organized code structure.
-
Decoupling: The client code is decoupled from the concrete classes, allowing for easier maintenance and modifications. When you want to add new product types, you can do so without modifying existing code.
-
Promoting Substitutability: The pattern promotes substitutability, allowing new types to be introduced at runtime without disturbing the existing code.
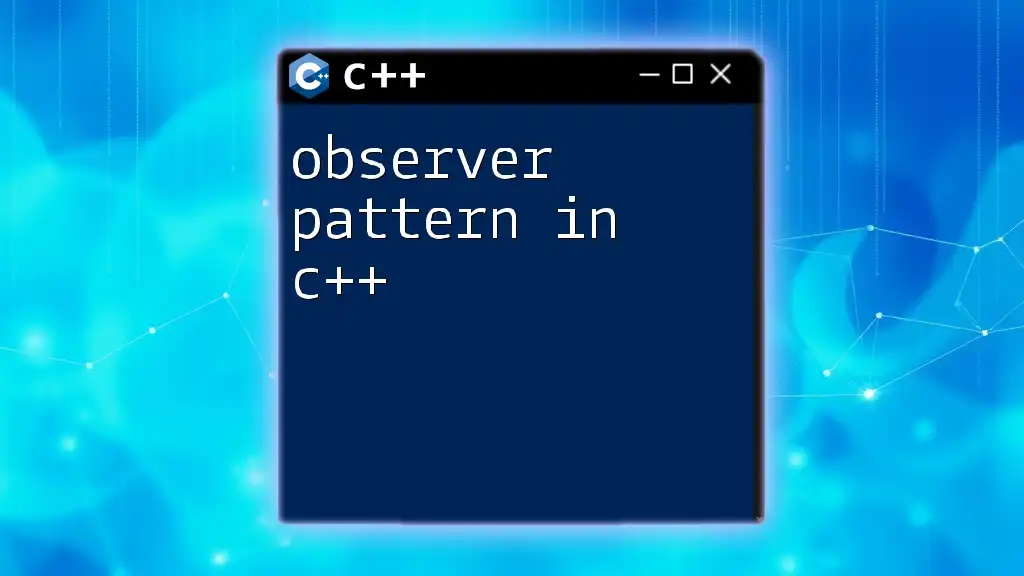
Understanding the Factory Method in C++
Defining the Factory Method
The Factory Method is an interface for creating objects in a superclass but allows subclasses to alter the type of objects that will be created. Unlike directly instantiating classes, the Factory Method delegates the instantiation process to subclasses.
How Factory Methods Work
Factory methods work by having a creator class that defines a method responsible for creating an object, often an object defined by an interface. Subclasses override this method to provide specific implementations. This design allows for flexibility in the types of objects created.
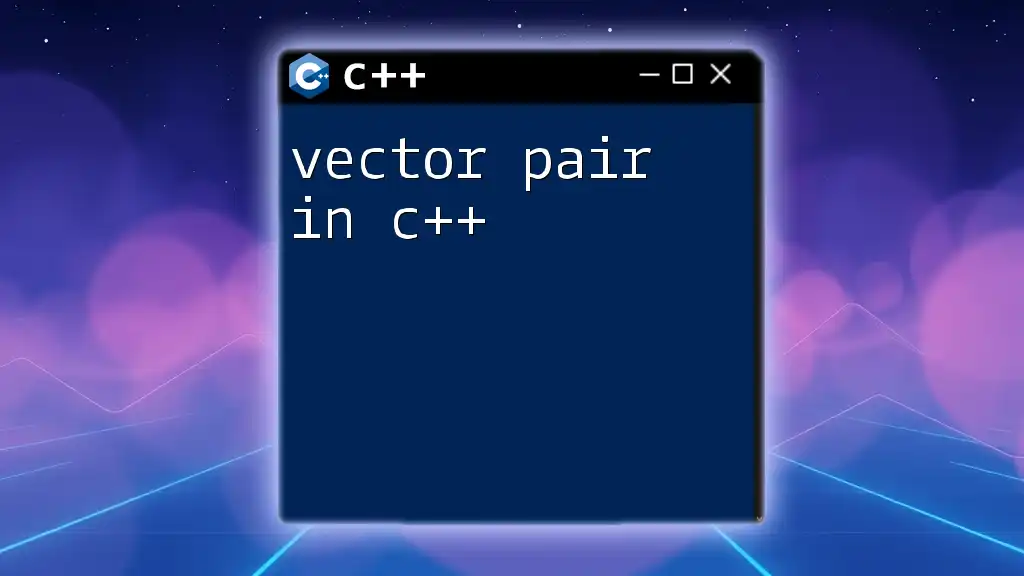
Key Components of the Factory Method Design Pattern in C++
The Product Interface
Central to the Factory Method Pattern is the Product interface. This interface defines the operations that can be performed on the products created by the factory.
class Product {
public:
virtual void use() = 0;
};
Concrete Products
Concrete classes implement the `Product` interface, providing specific functionality uniquely characteristic to each class.
class ConcreteProductA : public Product {
public:
void use() override {
// Implementation for product A
std::cout << "Using Concrete Product A" << std::endl;
}
};
class ConcreteProductB : public Product {
public:
void use() override {
// Implementation for product B
std::cout << "Using Concrete Product B" << std::endl;
}
};
The Creator/Factory Class
The Creator class declares the factory method, which returns a `Product`. The creator may also provide a default implementation that returns a default product type.
class Creator {
public:
virtual Product* factoryMethod() = 0;
};
Concrete Creator Classes
Concrete creators implement the factory method to instantiate and return objects of specific types.
class ConcreteCreatorA : public Creator {
public:
Product* factoryMethod() override {
return new ConcreteProductA();
}
};
class ConcreteCreatorB : public Creator {
public:
Product* factoryMethod() override {
return new ConcreteProductB();
}
};
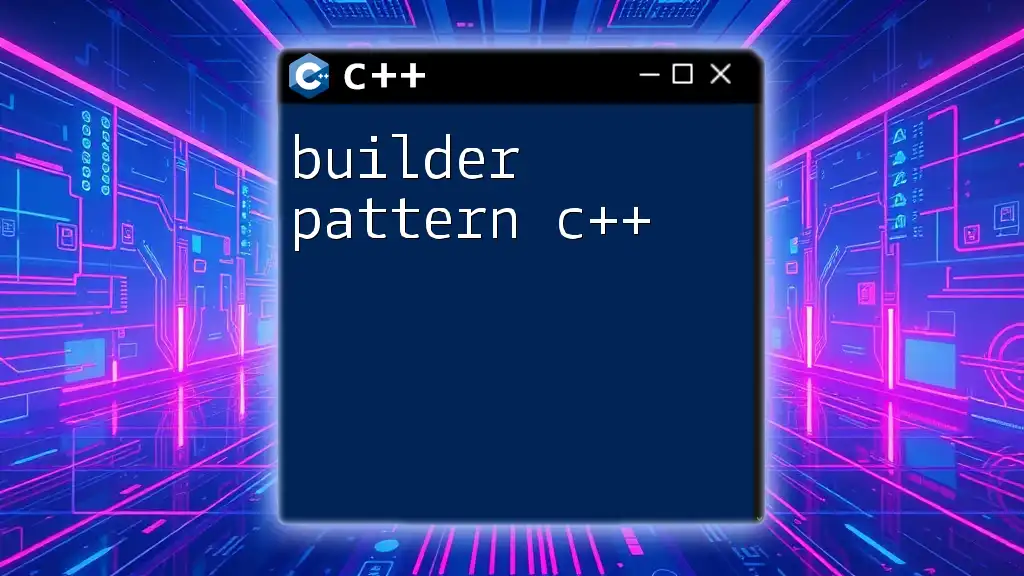
The Factory Design Pattern in Action
Creating Products Using Factory Method Design Pattern
The Factory Pattern can elevate your design by allowing for the creation of products without the client needing to know the specifics. Here’s how you would implement it.
void clientCode(Creator& creator) {
Product* product = creator.factoryMethod();
product->use(); // Use the created product
delete product; // Clean up
}
Decoupling Object Creation
One of the primary benefits of the Factory Method is its ability to decouple the creation of an object from its usage. This allows developers to change the product implementation without modifying the client code, which results in more maintainable systems.
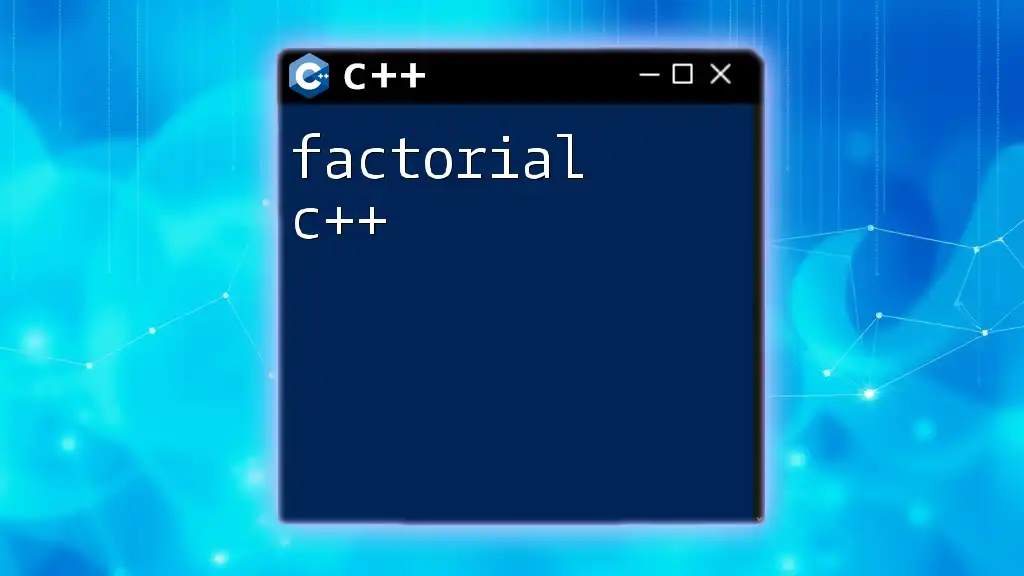
Benefits of Using the C++ Factory Pattern
Enhanced Code Maintainability
The Factory Pattern ensures that variations of products do not need to be scattered throughout the codebase. When adding a new product type, only the relevant creator class needs updates, leaving client code untouched. For instance, if a new `ConcreteProductC` is added, you just need a new creator that implements the factory method for it.
Improved Flexibility and Scalability
The pattern supports adding new products without altering existing code, leading to better scalability. For instance, if a client requests different product types, you can easily branch out by adding new factories and corresponding products without disturbing existing functionality.
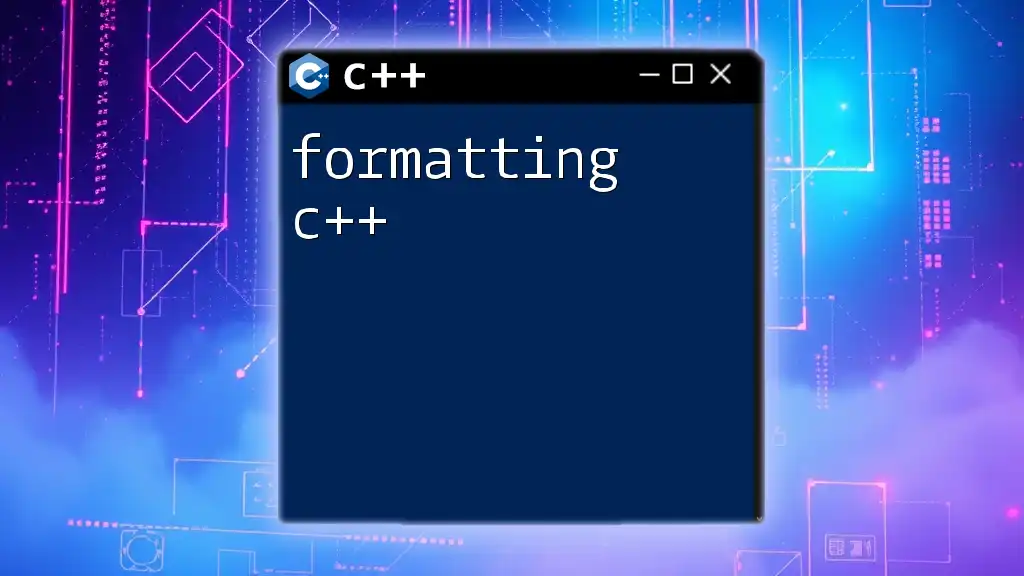
Challenges and Considerations
Understanding Complexity
While the Factory Pattern can simplify object creation, it can also introduce unnecessary complexity, especially if an application contains many products. Striking a balance between flexibility and complexity is essential.
Choosing the Right Pattern
Understanding when to use the factory pattern is crucial. If the product instantiation logic is simple, the factory method may be overkill. Conversely, if you foresee needing multiple product variations, adopting the Factory Pattern early on can save significant time and effort in the long run. Alternatives like the Builder Pattern might be preferred for more complex object creation processes.
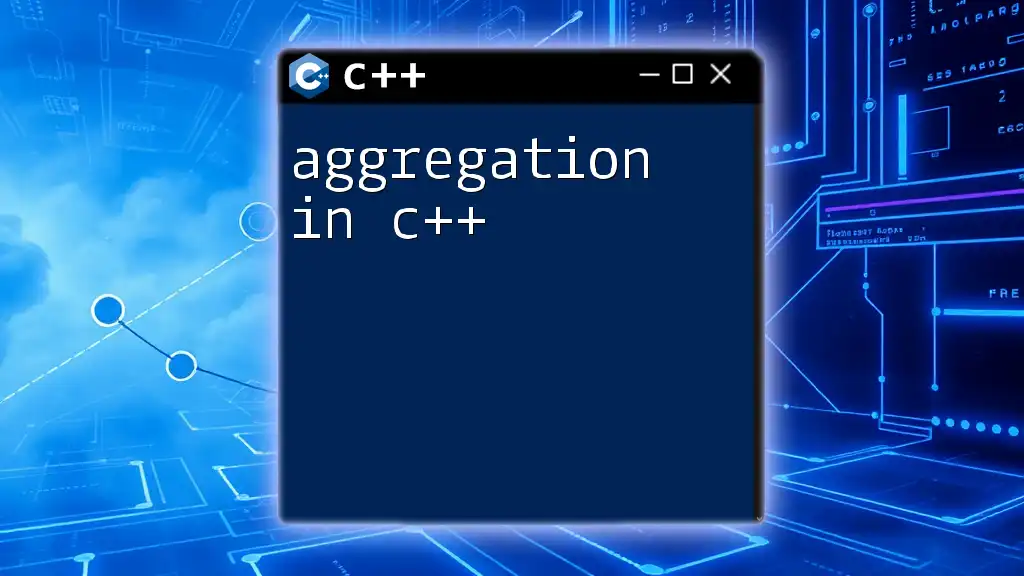
Conclusion
In summary, the factory pattern in C++ is a robust design solution that encapsulates the object creation process and provides a flexible framework for managing product types effectively. By understanding its components and implementation, developers can create scalable, maintainable, and decoupled code structures that stand the test of time in software development.
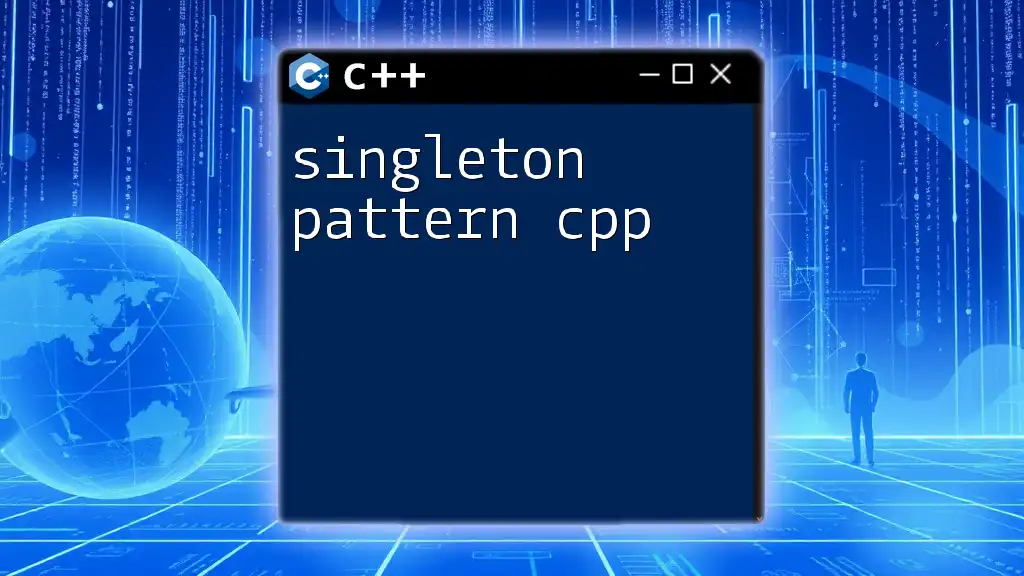
Additional Resources
Books and Tutorials for Further Learning
For deeper insights into design patterns, consider classic literature like "Design Patterns: Elements of Reusable Object-Oriented Software" by Erich Gamma et al. Online platforms like Coursera and Udemy offer courses focused on design patterns in C++, which can enhance your understanding.
Practical Examples and Code Repositories
You can find numerous examples and practical implementations of the Factory Pattern in C++ by exploring GitHub repositories. These examples illustrate various ways to apply the Factory Pattern across diverse scenarios, helping bridge theory and practice.