A binary search tree (BST) in C++ is a data structure that stores items in a way that allows for efficient searching, insertion, and deletion operations based on the left-child-less-than-parent and right-child-greater-than-parent rule.
Here's a simple C++ code snippet demonstrating the structure of a binary search tree:
#include <iostream>
struct TreeNode {
int value;
TreeNode* left;
TreeNode* right;
TreeNode(int val) : value(val), left(nullptr), right(nullptr) {}
};
class BST {
public:
TreeNode* root;
BST() : root(nullptr) {}
void insert(int val) {
root = insertRec(root, val);
}
private:
TreeNode* insertRec(TreeNode* node, int val) {
if (node == nullptr) {
return new TreeNode(val);
}
if (val < node->value) {
node->left = insertRec(node->left, val);
} else {
node->right = insertRec(node->right, val);
}
return node;
}
};
Understanding the Basics of BSTs
Definition of Binary Search Tree
A binary search tree (BST) is a data structure that has the following characteristics:
- Every node has at most two children, referred to as the left and right children.
- For any given node, all values in the left subtree are less than the node's value, and all values in the right subtree are greater.
Properties of a Binary Search Tree
The organization of data within a binary search tree provides essential properties that support efficient operations. These properties include:
- Ordered Structure: The BST maintains an ordered structure, which allows for effective searching, inserting, and deleting of values.
- Search, Insert, and Delete Operations: Each of these operations can be performed with a time complexity of O(log n) in the average case, making BSTs a popular choice for dynamic data sets.
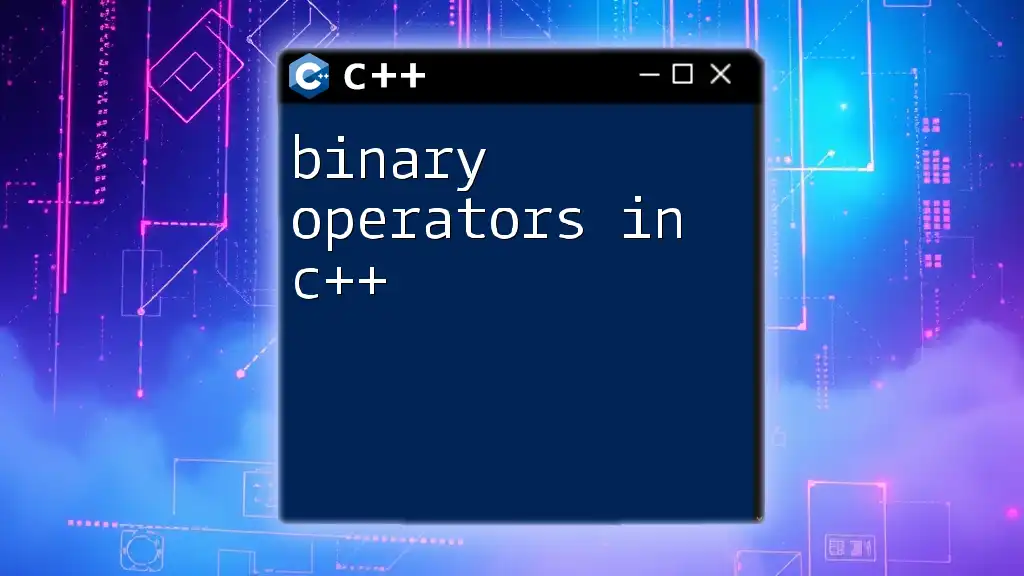
Advantages of Using a Binary Search Tree in C++
Time Complexity
One of the primary advantages of using binary search trees in C++ is their time complexity:
- Searching: On average, the search operation takes O(log n) time, as it eliminates half of the tree's nodes at each step.
- Insertion and Deletion: Both operations also have average-case complexities of O(log n). However, it’s important to note that these operations can degenerate to O(n) in the worst case if the tree becomes unbalanced.
Memory Efficiency
Compared to other data structures such as arrays and linked lists, binary search trees are more memory efficient:
- Dynamic Size: BSTs allow users to insert and delete nodes dynamically, avoiding the need to allocate a fixed amount of memory in advance.
- No Wasted Space: Unlike arrays where space may go unused, BSTs only occupy memory for their allocated nodes.
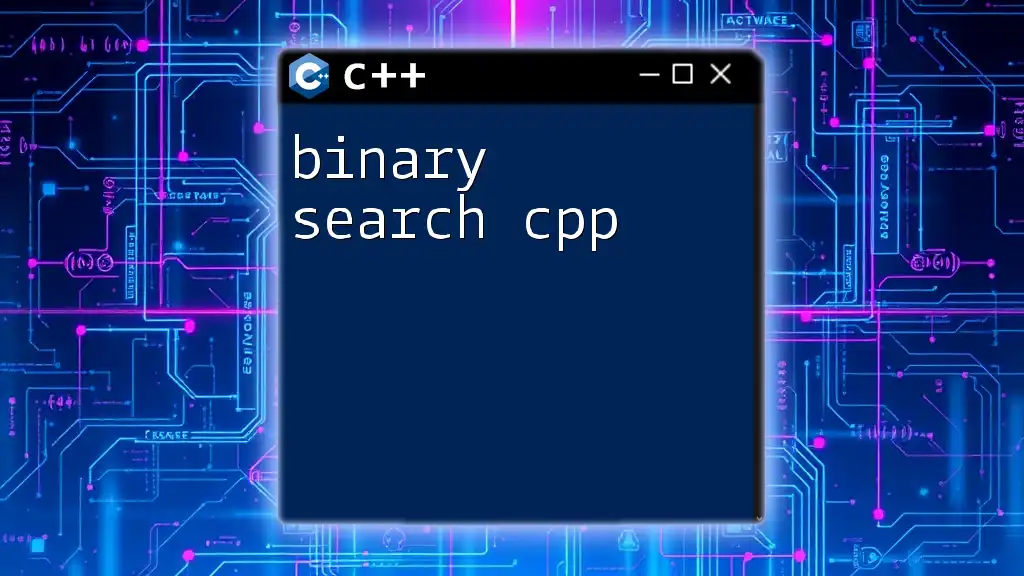
Implementing a Binary Search Tree in C++
Creating a Node Structure
To create a binary search tree, the first step is to define a node structure. This structure will represent each node of the tree.
struct Node {
int data;
Node* left;
Node* right;
};
In this structure:
- `data` stores the value of the node.
- `left` and `right` are pointers to the left and right child nodes, respectively.
Building the Binary Search Tree
The next step involves implementing the insertion function to build the binary search tree.
Node* insert(Node* root, int data) {
if (root == nullptr) {
root = new Node();
root->data = data;
root->left = root->right = nullptr;
} else if (data < root->data) {
root->left = insert(root->left, data);
} else {
root->right = insert(root->right, data);
}
return root;
}
In the code snippet above:
- If the current node is empty (i.e., `root` is `nullptr`), a new node is created.
- If the value to insert is less than the node's value, the function recursively calls itself on the left subtree; otherwise, it calls itself on the right subtree.
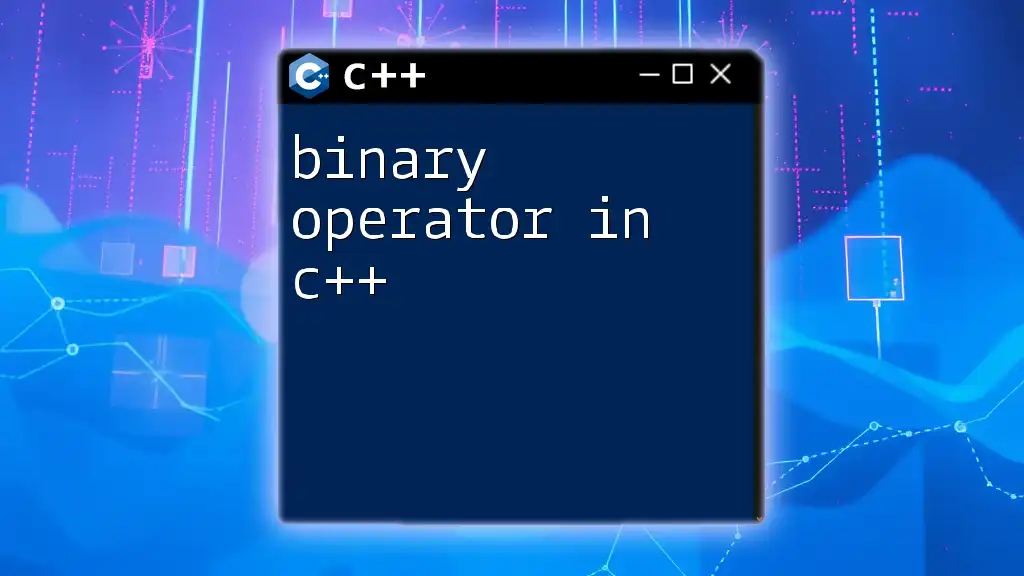
Searching in a Binary Search Tree
Searching for a value in a binary search tree is straightforward due to its organized structure.
Node* search(Node* root, int data) {
if (root == nullptr || root->data == data)
return root;
if (data < root->data)
return search(root->left, data);
return search(root->right, data);
}
In this search function:
- If the current node is null or matches the search value, it returns that node.
- If the search value is less than the current node's value, it moves to the left child; otherwise, it proceeds to the right child.
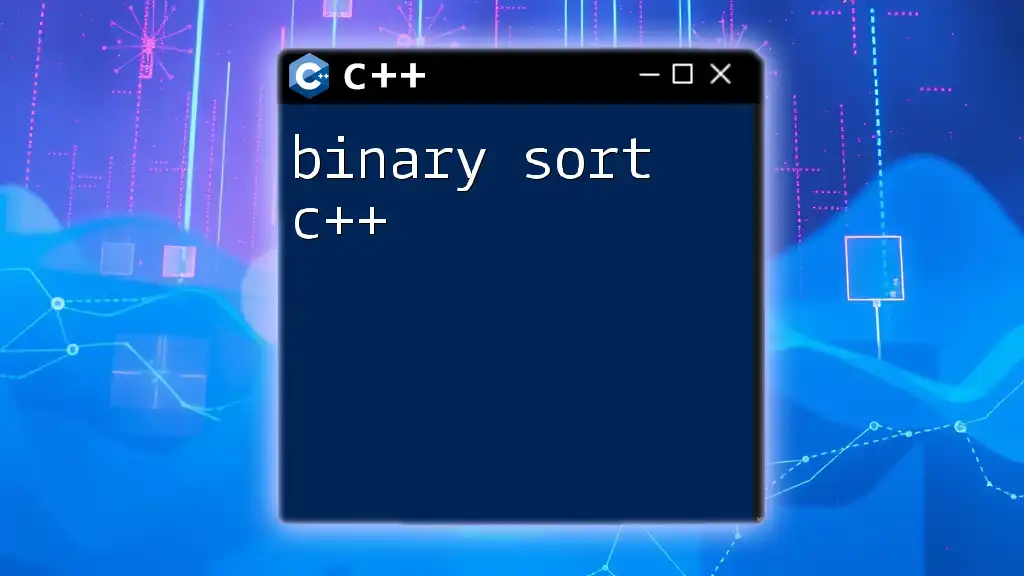
Deleting from a Binary Search Tree
Delete operations in a binary search tree can be more complex than insertion, as it involves three distinct scenarios:
- Leaf Node: If the node to be deleted is a leaf, simply remove it.
- One Child: If the node has one child, replace the node with its child.
- Two Children: If the node has two children, find the inorder successor (the smallest node in the right subtree), copy its value to the deleting node, and remove the successor.
Here's how to implement the delete function:
Node* deleteNode(Node* root, int data) {
if (root == nullptr) return root;
if (data < root->data)
root->left = deleteNode(root->left, data);
else if (data > root->data)
root->right = deleteNode(root->right, data);
else { // root is the node to be deleted
// Node has one child or no child
if (root->left == nullptr) return root->right;
else if (root->right == nullptr) return root->left;
// Node with two children: Get the inorder successor
Node* temp = minValueNode(root->right);
root->data = temp->data; // Copy the inorder successor's content
root->right = deleteNode(root->right, temp->data); // Delete the inorder successor
}
return root;
}
Understanding Delete Operations
This function efficiently handles each case, ensuring the tree's order remains intact after the deletion.
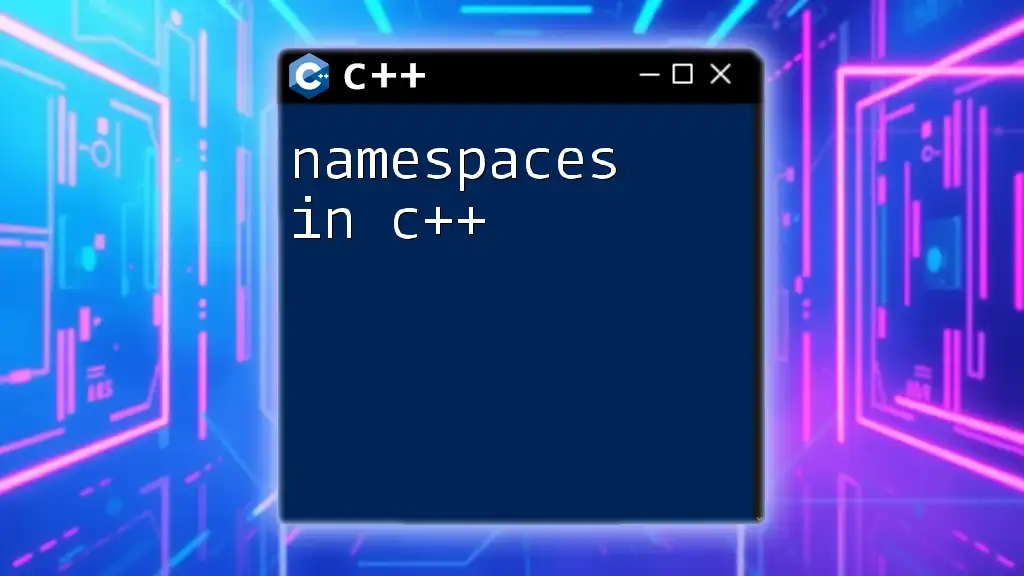
Traversing a Binary Search Tree
Traversal techniques allow users to visit all the nodes in a binary search tree effectively. The most common traversal methods include:
In-order Traversal
This traversal is critical as it outputs the BST's values in sorted order.
void inorder(Node* root) {
if (root) {
inorder(root->left);
cout << root->data << " ";
inorder(root->right);
}
}
In this implementation, the function goes recursively to the left child, prints the current node, and then visits the right child.
Pre-order and Post-order Traversals
- Pre-order Traversal: This traversal is useful for creating copies of the tree, invoking the current node before traversing its children.
- Post-order Traversal: This is primarily used for deleting nodes, as it ensures that children are deleted before their parent node.
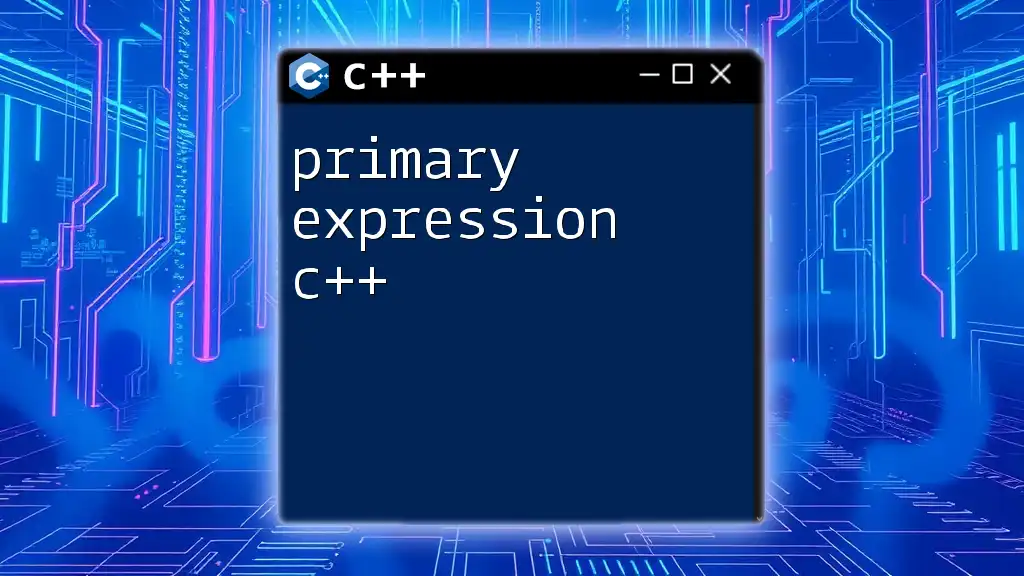
Balancing Binary Search Trees
Maintaining balance in a binary search tree is crucial for ensuring optimal time complexities. An unbalanced tree can lead to degenerated structures resembling linked lists, leading to O(n) time complexities for search and insertion operations.
Why Balance a BST?
To maintain quick access and manipulation, balanced trees such as AVL trees or Red-Black trees are often employed. These trees automatically perform rotations to maintain balance after each insertion or deletion.
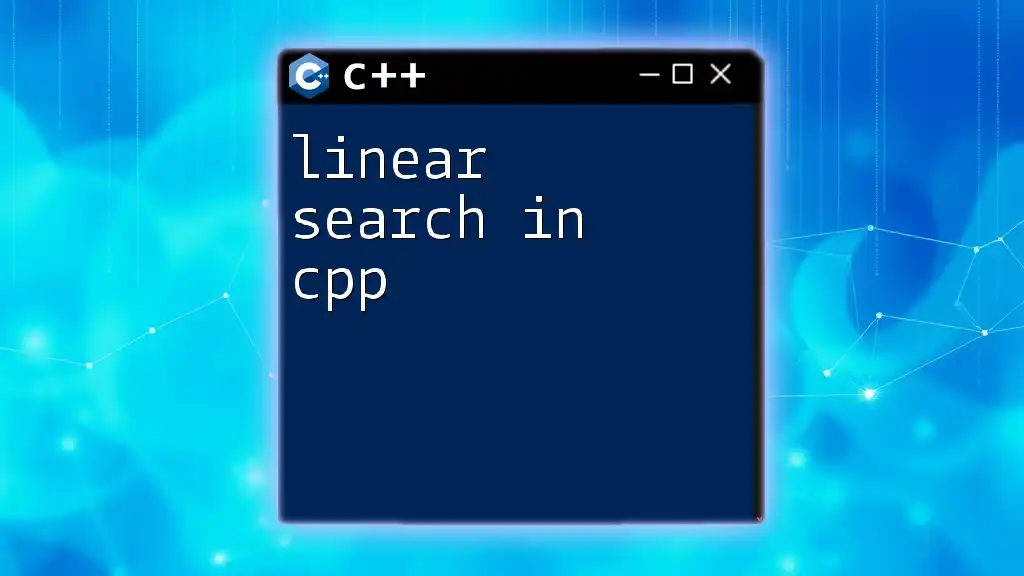
Conclusion
Understanding binary search trees in C++ is fundamental for efficient data organization and manipulation. The characteristics of BSTs, combined with their advantages over other structures, cement their place in programming. From searching and inserting data to deleting nodes and maintaining balance, mastering these concepts encourages more optimized coding practices.
As you dive deeper into mastering binary search trees, consider exploring related data structures and algorithms that can complement your knowledge and broaden your programming skill set.