A C++ virtual file system (VFS) abstracts file and directory operations to provide a unified interface for accessing different types of file systems, making it easier to manage files across various platforms and environments.
Here’s a simple example of how you might implement a basic virtual file system interface in C++:
#include <iostream>
#include <string>
#include <unordered_map>
#include <memory>
class File {
public:
virtual void open() = 0;
};
class TextFile : public File {
public:
void open() override {
std::cout << "Opening text file." << std::endl;
}
};
class VirtualFileSystem {
private:
std::unordered_map<std::string, std::unique_ptr<File>> files;
public:
void addFile(const std::string& name, std::unique_ptr<File> file) {
files[name] = std::move(file);
}
void openFile(const std::string& name) {
if (files.find(name) != files.end()) {
files[name]->open();
} else {
std::cout << "File not found." << std::endl;
}
}
};
int main() {
VirtualFileSystem vfs;
vfs.addFile("example.txt", std::make_unique<TextFile>());
vfs.openFile("example.txt");
return 0;
}
Understanding Virtual File Systems
What is a Virtual File System?
A Virtual File System (VFS) is an abstraction layer that allows applications to interact with different types of file systems in a uniform manner. By creating this abstraction, a VFS enables developers to manage files and directories without needing to know the specifics of the underlying storage architecture. It allows applications to perform tasks such as file management, access control, and directory organization seamlessly, regardless of whether the files are stored on local disks, network drives, or cloud storage.
Benefits of Using a Virtual File System
Utilizing a C++ virtual file system offers numerous advantages:
- Abstraction of Underlying File Systems: A VFS hides the complexities of various file systems, offering a consistent API for operations.
- Portability Across Different Environments: Applications can easily adapt to different file systems or platforms without modifying the core logic.
- Easy File Management and Organization: Simplified operations on files and directories promote better organization and management.
- Performance Improvements: In some scenarios, VFS can improve performance by caching frequently accessed files or optimizing read/write operations.
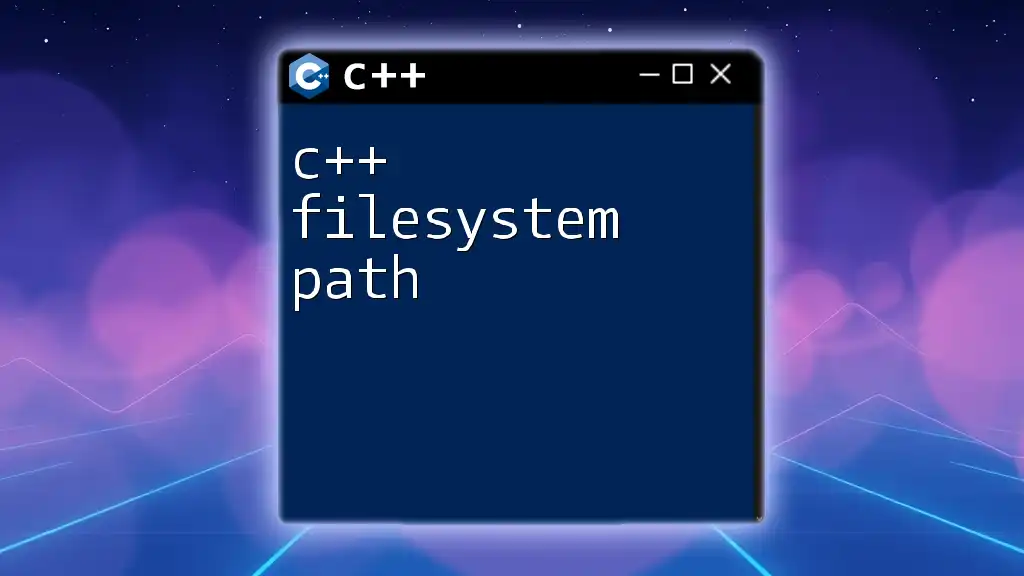
Core Concepts of C++ Virtual File System
Components of a C++ Virtual File System
To effectively implement a C++ virtual file system, several components must be understood:
-
Inodes and Files: In a file system, inodes store metadata about files, such as permissions and file size. A VFS logically represents files using these inodes, abstracting the details from the user.
-
File Handles: These are abstractions that represent an open file. They facilitate operations such as reading, writing, or seeking within a file.
-
Directory Structure: In a VFS, directories themselves can be treated as files that contain references to other files or directories (subdirectories). This hierarchical representation organizes data efficiently.
Key Operations in a Virtual File System
File Creation and Management
Creating and managing files in a C++ virtual file system is a fundamental operation. For example, one can implement file creation using the following code snippet:
VFS::createFile("virtualfile.txt");
In this operation, `createFile` typically checks if the file already exists and allocates an inode to the new file, initializing necessary metadata.
Reading and Writing Files
Reading from a VFS is remarkably straightforward. To retrieve the content of a file, one might use:
std::string content = VFS::readFile("virtualfile.txt");
Conversely, to write data to a virtual file, the following code could be employed:
VFS::writeFile("virtualfile.txt", "Hello, VFS!");
In both cases, the VFS handles the underlying file system's specifics, allowing developers to focus on application logic rather than file storage mechanics.
Navigating Directories
Directory manipulation is crucial for organizing files within a VFS. To list files in a virtual directory, the following command can be issued:
VFS::listDirectory("/myVirtualDirectory");
This feature enables users to explore contents just as if they were interacting with a traditional filesystem.
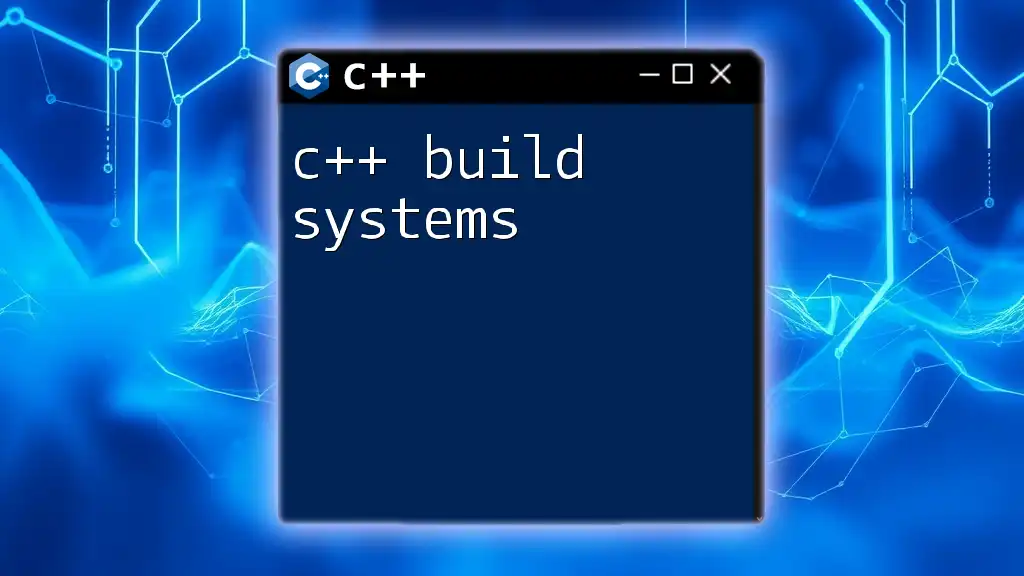
Implementing a Simple C++ Virtual File System
Setting Up the Environment
To start with a C++ virtual file system, appropriate libraries and dependencies must be identified. This setup may involve using STL for string and vector manipulations, ensuring compatibility in handling file names and data structures. Ensure that your project has a well-defined file structure to organize files and related code efficiently.
Writing the Code for VFS
Defining the VFS Class
To create a basic VFS, start by defining the `VFS` class. Here's an example:
class VFS {
public:
void createFile(const std::string& name) { /* Implementation */ }
std::string readFile(const std::string& name) { /* Implementation */ }
void writeFile(const std::string& name, const std::string& data) { /* Implementation */ }
std::vector<std::string> listDirectory(const std::string& path) { /* Implementation */ }
};
Each method requires careful implementation to correctly manage file operations, making sure that file attributes are handled appropriately and that errors are reported effectively.
Testing the Virtual File System
Sample Test Cases
Testing the functionalities of your VFS is critical to ensure reliability and performance:
-
Creating and managing files: Test the creation of multiple files and check that metadata updates correctly.
-
Reading and writing validation: After writing data to a file, attempt to read and check that the returned data matches the expected content.
-
Directory navigation test cases: Create and navigate through directories, ensuring files are listed and can be accessed as intended.
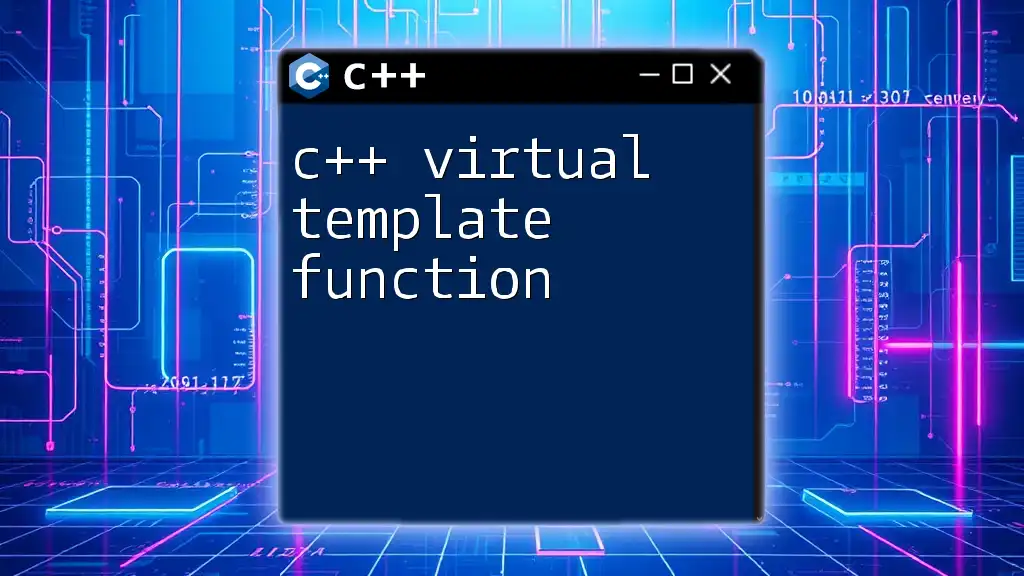
Advanced Features of C++ Virtual File Systems
Supporting Different Backends
One of the most powerful features of a VFS is its ability to support various storage backends. For instance, you can implement a backend system that facilitates both local file access and cloud storage operations. This enables users to interact with files without needing to change the API.
Error Handling in VFS
Since file systems can encounter a variety of issues—from missing files to permission errors—effective error handling is crucial. Developers should implement robust error-handling mechanisms, catching exceptions and logging errors to provide feedback to users:
try {
VFS::readFile("nonexistent.txt");
} catch(const std::exception& e) {
std::cerr << e.what() << '\n';
}
By explicitly managing these situations, the application becomes more user-friendly and resilient.
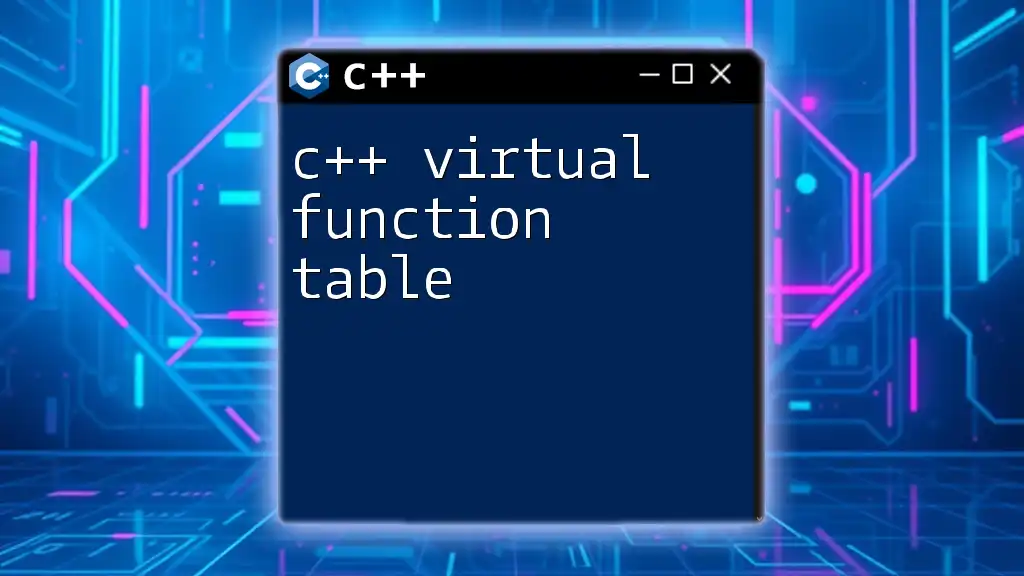
Use Cases for C++ Virtual File System
Real-world Applications
A C++ virtual file system can find utility in various domains:
-
Game Development: Frequently used for asset management, where developers can load textures, models, and sounds from different resources.
-
Cloud Services: Essential for data abstraction; VFS helps manage and access files stored across multiple cloud providers seamlessly.
-
Operating Systems: Many modern operating systems utilize a VFS to provide uniform access to different types of file systems.
Case Study: Implementing VFS in a Project
Consider a project that required integrating various file sources. By implementing a VFS, the development team could swiftly switch between local storage and a cloud service without altering the application’s core code. The outcome was a more agile development process and improved performance as file accesses were optimized.
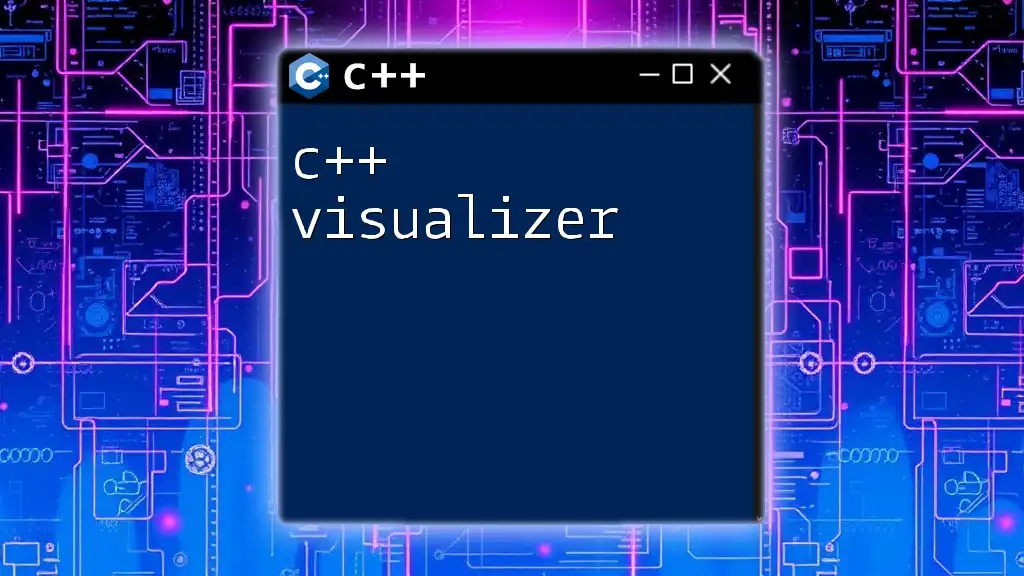
Conclusion
In summary, a C++ virtual file system enhances the flexibility and capability of applications by abstracting file management operations. Understanding its components and properly utilizing its features can lead to more robust and efficient software solutions. As you explore VFS concepts further, consider how these systems could benefit your own projects, improving both maintainability and user experience.
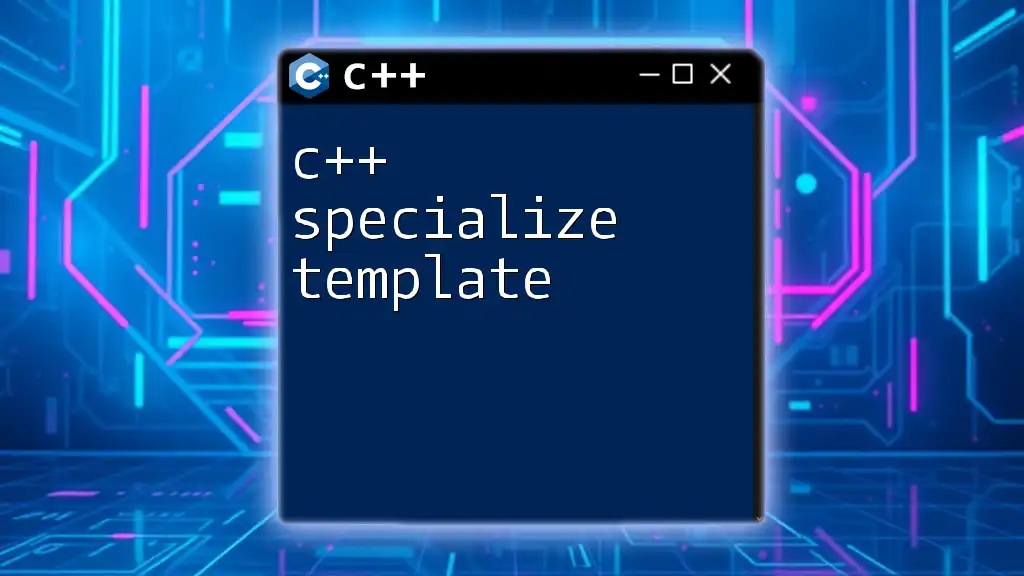
Additional Resources
Recommended Reading
For those looking to expand their knowledge on C++ virtual file systems, consider checking out specific books and online articles that delve deeply into both C++ programming and the theory behind virtual file systems.
Practical Tools and Libraries
Explore popular libraries that assist in VFS implementation, and find GitHub repositories that showcase working examples. Being part of an online community can also provide valuable insights and further enhance your learning journey.