A C++ event system allows objects to communicate with each other by notifying and responding to events, enabling a flexible and decoupled design.
Here's a simple code snippet demonstrating a basic event system in C++:
#include <iostream>
#include <functional>
#include <vector>
class Event {
public:
void subscribe(const std::function<void()>& listener) {
listeners.push_back(listener);
}
void notify() {
for (auto& listener : listeners) {
listener();
}
}
private:
std::vector<std::function<void()>> listeners;
};
int main() {
Event onButtonClick;
onButtonClick.subscribe([]() {
std::cout << "Button clicked!" << std::endl;
});
onButtonClick.notify(); // Simulate button click
return 0;
}
Importance of Event Systems
Event systems play a crucial role in modern programming, particularly in C++. These systems allow applications to respond to various actions, enhancing user experience and ensuring smoother program operation. In interactive applications, such as GUI software or games, event systems are essential for capturing user inputs and system notifications. The responsiveness and interactivity of applications heavily depend on how effectively they handle events.
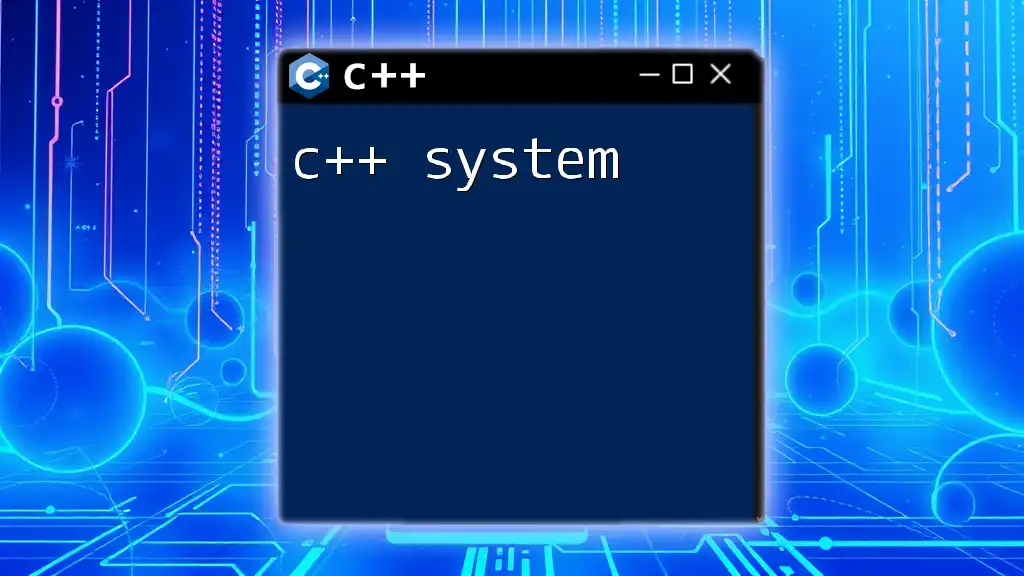
Overview of C++ Event Systems
At its core, a C++ event system is designed to manage events like keyboard strokes, mouse movements, and other actions that occur during program execution. By organizing how events are captured and handled, C++ event systems simplify complex interaction models and allow for modular code design.
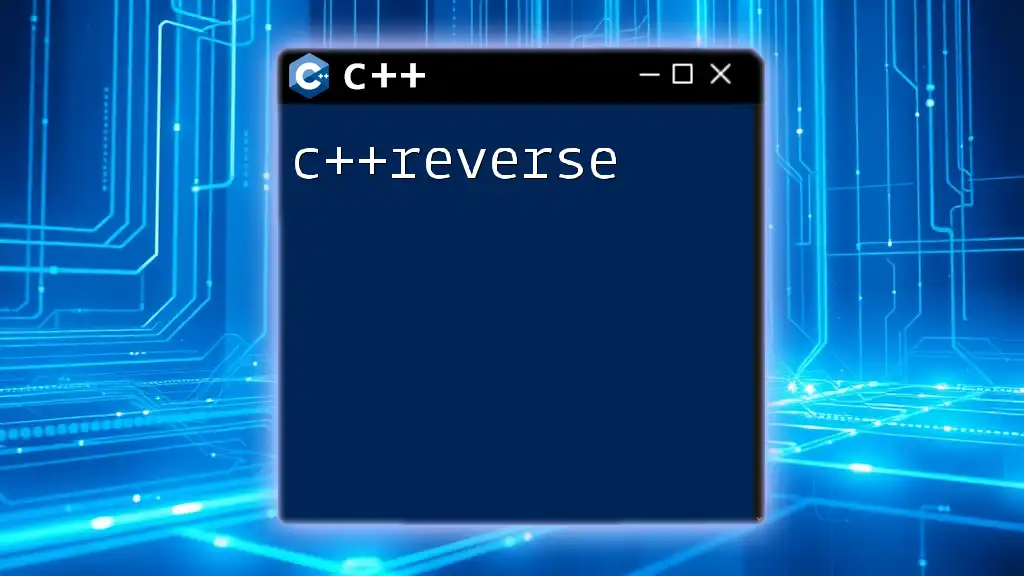
Understanding Events
What is an Event?
An event is a signal indicating that a specific action has occurred within a program. Events can originate from various sources, such as user actions (pressing keys, clicking mouse buttons), system notifications (timer events), or internal triggers (completion of a task).
Event Sources and Event Listeners
-
Event Sources: Entities that generate events. For example, buttons in a GUI or objects in a game can be event sources.
-
Event Listeners: Specialized components that respond to events. They "listen" for specific events and execute corresponding actions when these events occur. In C++, event listeners are generally implemented as functions or classes that define how to respond to an event.
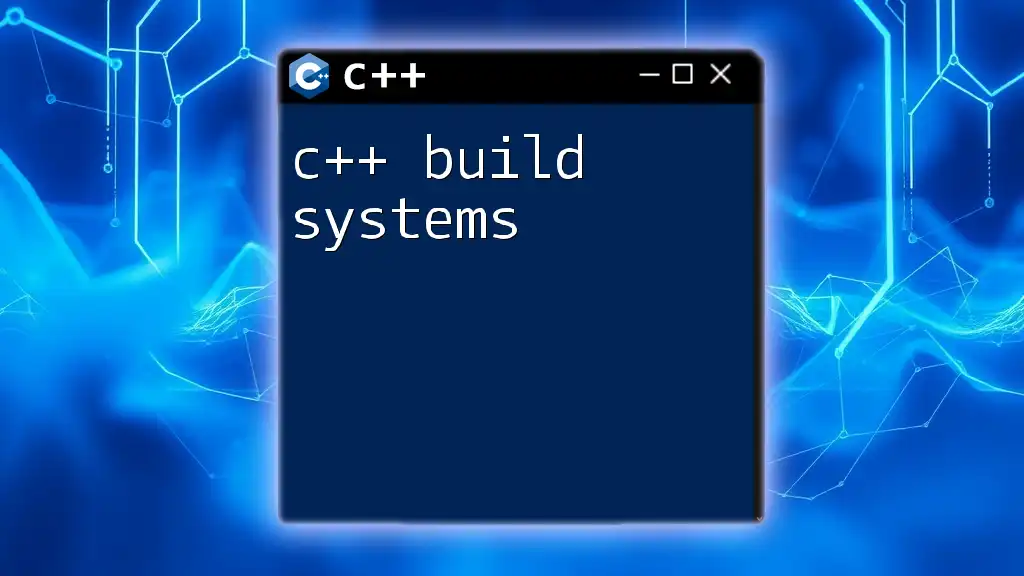
C++ Event Handling Basics
The Role of Callback Functions
In C++, callback functions are functions passed as parameters to other functions. These callbacks act as a bridge between the event source and the listener. When an event occurs, the event source triggers the callback function, allowing for dynamic and flexible event handling.
Creating a Basic Event System
To understand an event system, we'll create a simple example. In this project, we will develop a basic event system featuring an event source (a button click) and an event listener.
Step-by-step guide:
- Define an `Event` class to serve as the base for creating specific events.
- Create a `ButtonClickEvent` class that inherits from `Event`.
- Implement an interface for event listeners and develop a concrete listener that reacts to the button click.
Here’s the code for these steps:
class Event {
public:
virtual void trigger() = 0;
};
class ButtonClickEvent : public Event {
public:
void trigger() override {
// Code for button click event handling
std::cout << "Button clicked!" << std::endl;
}
};
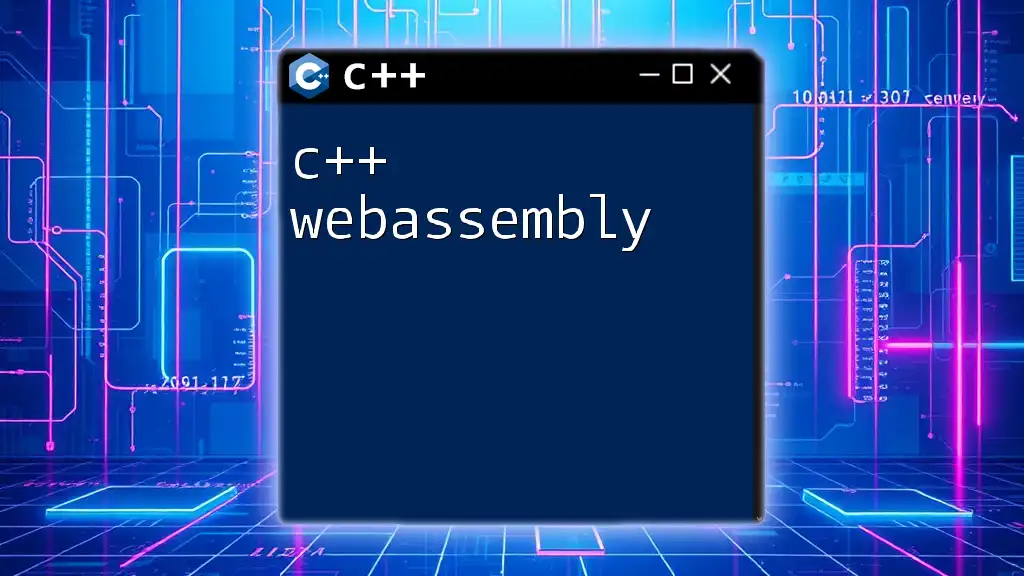
Implementing a C++ Event System
Setting Up Event Classes
To set up an event system, it is important to create event classes that encapsulate event details and behaviors. By implementing a listener interface, we can create event listeners that respond appropriately. Below is an example showcasing how to implement these concepts.
Example Code
class EventListener {
public:
virtual void onEvent(Event& e) = 0;
};
class ClickListener : public EventListener {
public:
void onEvent(Event& e) override {
e.trigger(); // Trigger the event behavior
}
};
Event Manager Implementation
An event manager orchestrates the relationship between events and listeners. It is responsible for registering listeners and notifying them when an event occurs. Here’s a simple implementation of an event manager:
class EventManager {
std::vector<EventListener*> listeners;
public:
void addListener(EventListener* listener) {
listeners.push_back(listener);
}
void notifyListeners(Event& e) {
for (auto listener : listeners) {
listener->onEvent(e);
}
}
};
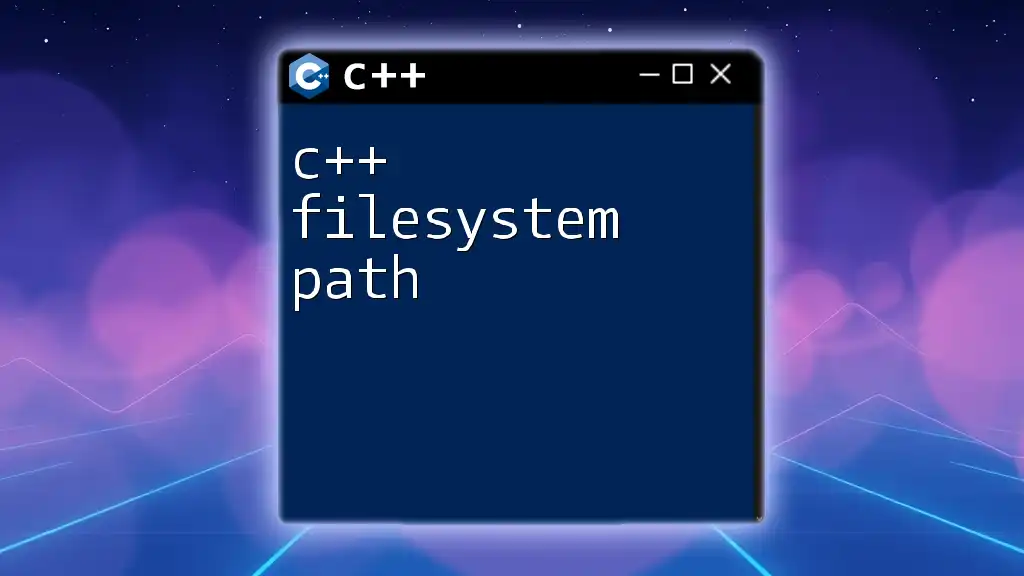
Advanced Event System Concepts
Asynchronous Event Handling
Asynchronous processing is a powerful feature of modern programming. By allowing event handlers to execute independently of the main thread, applications can remain responsive while handling multiple actions. In C++, implementing asynchronous event handling can be achieved using threads. Here’s an example demonstrating this concept:
#include <future>
void asyncCallback(Event& e) {
// Asynchronous event handling
e.trigger();
}
void triggerEventAsync(Event& e) {
std::async(std::launch::async, asyncCallback, e);
}
Event Queues
An event queue manages events in a first-in, first-out (FIFO) structure. This allows multiple events to be processed in an orderly fashion. Implementing an event queue can help in scenarios where events need to be queued for future handling:
class EventQueue {
std::queue<Event*> queue;
public:
void push(Event* event) {
queue.push(event);
}
Event* pop() {
if (queue.empty()) return nullptr;
Event* event = queue.front();
queue.pop();
return event;
}
};
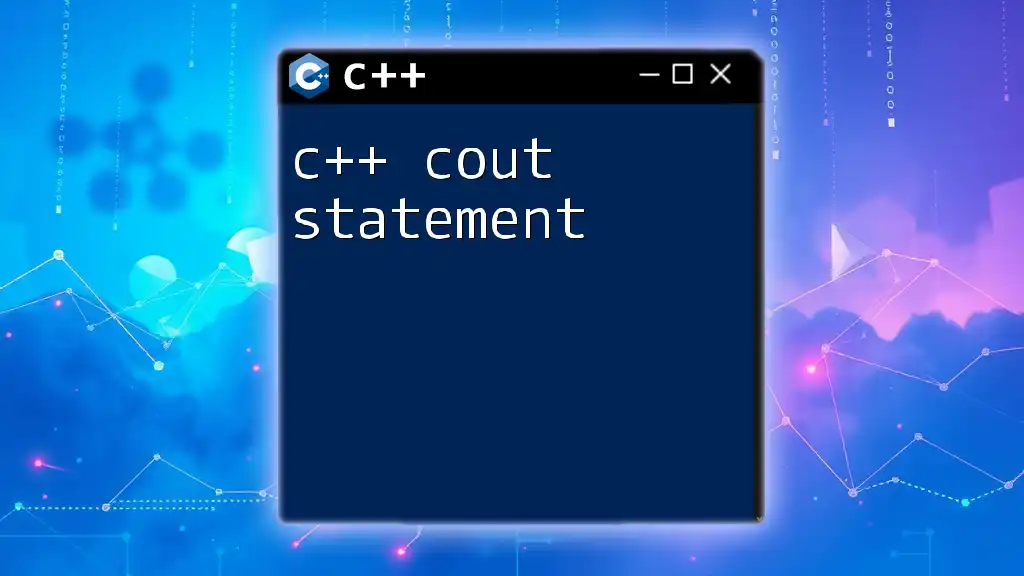
Best Practices for C++ Event Systems
Managing Memory in Events
Memory management is critical when dealing with dynamically allocated events. To avoid memory leaks, it's essential to ensure that events are properly deleted after they have been handled. Utilizing smart pointers (like `std::unique_ptr`) can help manage lifecycle automatically.
Performance Considerations
Performance is a key consideration in event systems. Minimizing the overhead caused by event handling is crucial, especially in applications that handle numerous events per second (e.g., games). Strategies for better performance include:
- Batch processing: Handle multiple events in a single iteration.
- Pooling: Reuse event objects to minimize memory allocation costs.
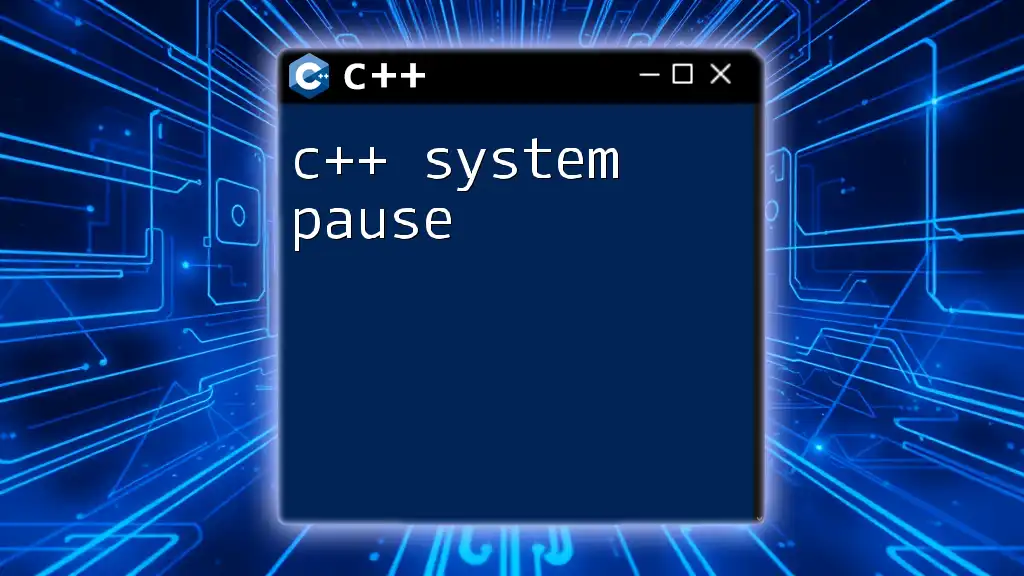
Real-World Use Cases of C++ Event Systems
GUI Applications
In graphical user interfaces, event systems capture user actions such as clicks, drags, and keyboard inputs. C++ libraries like Qt or wxWidgets provide built-in event systems that facilitate event handling, enabling developers to focus on the application logic rather than the complexities of interaction management.
Game Development
Event systems are fundamental in game development, where player inputs trigger various in-game actions. For instance, when a player presses a key, an event is generated that controls character movement or initiates other game features. Efficiently managing these events can significantly enhance game performance and player experience.
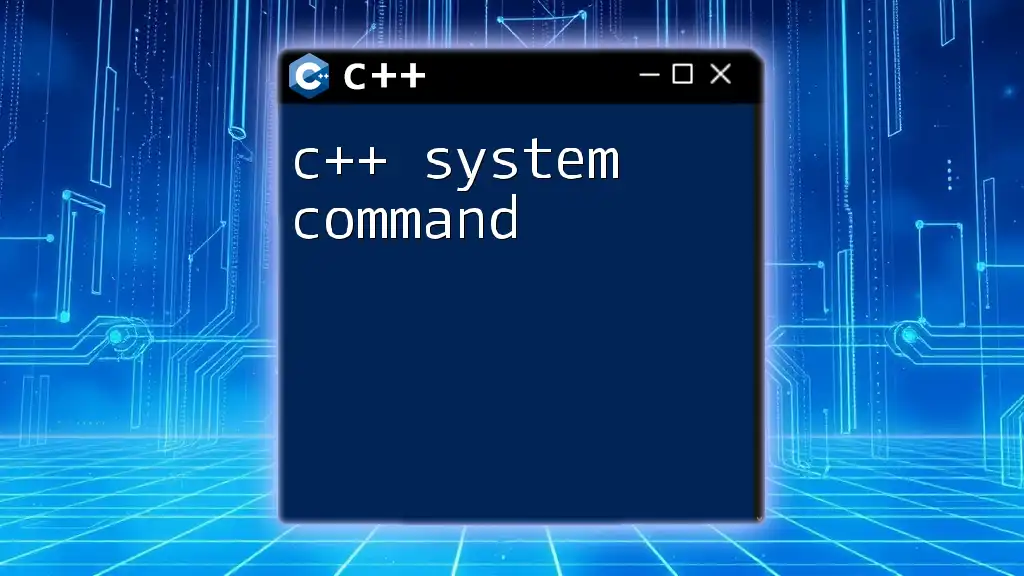
Conclusion
In summary, the C++ event system is a vital component in application development that enhances responsiveness and user interaction. By understanding the fundamental concepts of events, listeners, and event managers, developers can create powerful and efficient applications that seamlessly handle user actions and system notifications. As you build your own event systems, consider implementing advanced techniques and best practices to optimize performance and usability. Happy coding!