C++ is a powerful programming language that combines high-level abstraction with low-level memory manipulation, allowing for efficient system and application development.
#include <iostream>
int main() {
std::cout << "Hello, C++ Awesome!" << std::endl;
return 0;
}
Understanding the Basics of C++
To fully appreciate the awesomeness of C++, it is essential to grasp its fundamental syntax and structure. C++ is a statically typed language that builds on the syntactical foundation of the C programming language, and its core components allow developers to create efficient and powerful applications.
Essential C++ Syntax
The basic syntax of C++ consists of the following key elements:
- Include Directives: Used to include libraries.
- Namespace: Contains identifiers to avoid naming conflicts.
- Main Function: The entry point of every C++ program.
Here is a simple example that encapsulates these elements, offering a glimpse of a complete C++ program:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, C++ World!" << endl;
return 0;
}
In this example, we are including the iostream library, using the standard namespace, and defining the `main` function, which prints a greeting to the console.
Core Concepts of C++
Understanding core concepts contributes significantly to your ability to write effective C++ code. The essentials include variables, data types, and control structures.
Variables and Data Types
C++ supports several data types, including int, double, char, and string. Variables are declared to store values of these types.
int age = 30; // Integer type
double salary = 50000.50; // Double type
string name = "John"; // String type
Here, we declared variables of different types and assigned them values. Understanding how to manipulate these types is crucial to mastering C++.
Control Structures
Control structures allow your program to perform conditional and repetitive tasks. The most common structures include `if`, `else`, `switch`, and loops (`for`, `while`, `do-while`).
A simple loop that prints numbers can be seen below:
for(int i = 0; i < 5; i++) {
cout << "Number: " << i << endl;
}
This loop iterates through numbers 0 to 4, demonstrating a foundational loop mechanism in C++.
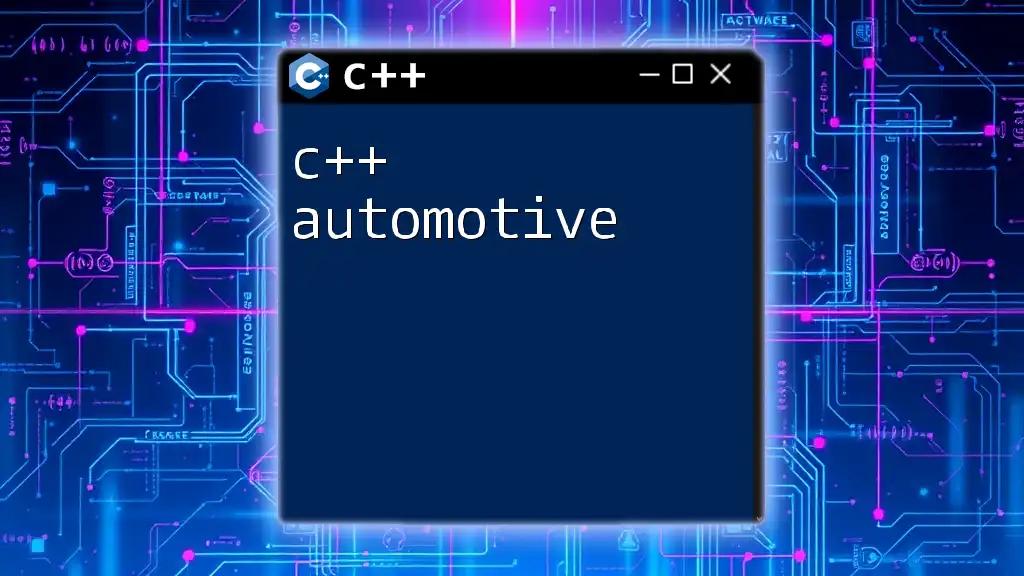
Diving Deeper into Awesome C++
Once you're comfortable with the basics, it’s time to dive deeper into more awesome features of C++.
Object-Oriented Programming (OOP) in C++
C++ supports Object-Oriented Programming, which is based on four main principles:
- Encapsulation: Bundling data and the methods that operate on that data within a single unit (or class).
- Inheritance: Creating new classes from existing ones, inheriting attributes and behaviors.
- Polymorphism: Using the same interface for different underlying forms (data types).
Here’s a simple class demonstration:
class Car {
public:
string brand;
string model;
int year;
void honk() {
cout << "Honk! Honk!" << endl;
}
};
This `Car` class encapsulates attributes and methods, showcasing the essence of OOP in C++.
Templates and the Power of Generics
Templates are one of the awesome features of C++. They allow you to define functions and classes with placeholders for data types, which promotes code reusability.
template <typename T>
T add(T a, T b) {
return a + b;
}
In this example, the `add` function can accept any datatype, making it incredibly versatile.
The Standard Template Library (STL)
The Standard Template Library provides a set of useful classes and functions, making C++ even more powerful. It includes components such as vectors, lists, maps, and sets.
Here’s how to declare a vector:
#include <vector>
using namespace std;
vector<int> numbers = {1, 2, 3, 4, 5};
Vectors are dynamic arrays, allowing more flexibility than standard arrays. Understanding STL is crucial for efficient C++ programming.
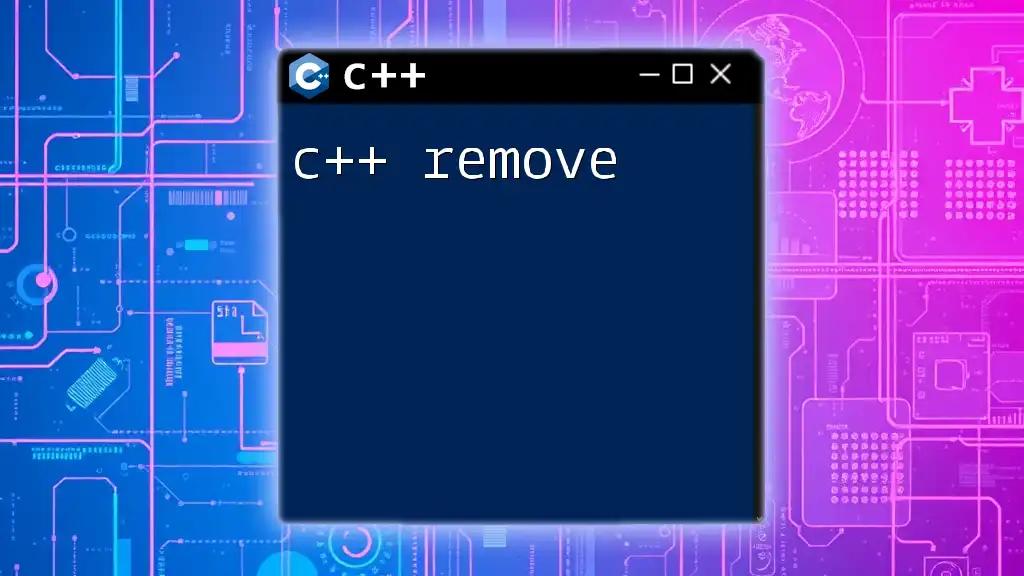
Advanced C++ Features
Introduction to advanced features will further showcase the awesomeness of C++ and broaden your development toolkit.
Smart Pointers and Memory Management
Managing memory is a critical part of C++ programming. Smart pointers are introduced to help with memory management automatically.
- `unique_ptr`: A smart pointer that owns a dynamically allocated object exclusively.
- `shared_ptr`: A smart pointer that can share ownership of an object.
Here’s how you can use `unique_ptr`:
#include <memory>
using namespace std;
unique_ptr<Car> myCar = make_unique<Car>();
This eliminates the need for manual memory management, minimizing memory leaks and ensuring data integrity.
Lambda Expressions
Lambda expressions introduce a concise way to express function objects in C++. They are particularly useful in modern C++ for inline function definitions.
Here’s a simple example:
auto sum = [](int a, int b) { return a + b; };
cout << sum(5, 7); // Outputs: 12
The above example declares a lambda that takes two integers and returns their sum, demonstrating how C++ can enhance code brevity and clarity.
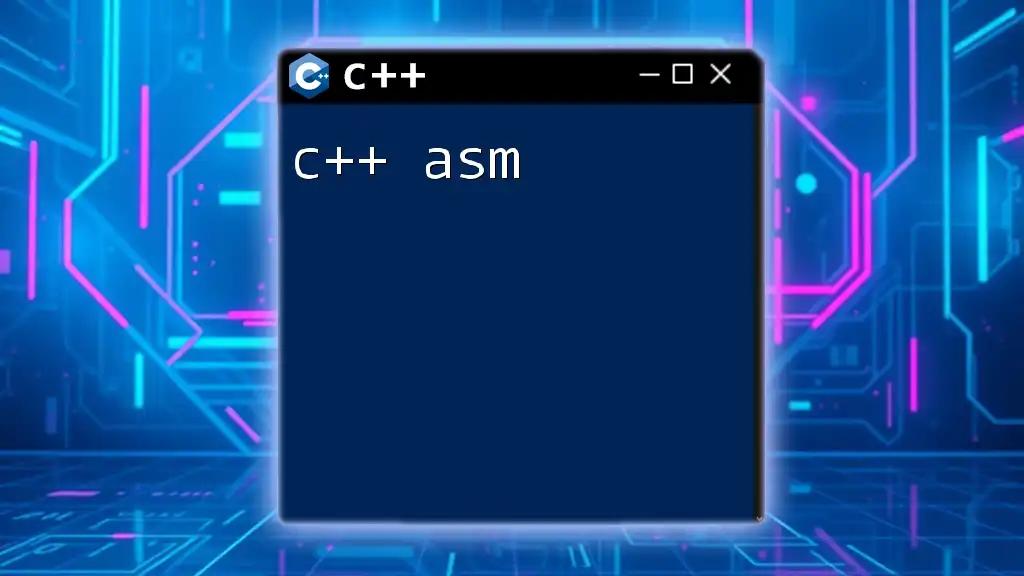
Practical Applications of Awesome C++
Building a Simple C++ Application
To solidify your understanding, let's consider building a simple console-based calculator. It can perform basic operations like addition, subtraction, multiplication, and division.
Here’s a brief outline of how the main code structure could look:
#include <iostream>
using namespace std;
int main() {
float num1, num2;
char op;
cout << "Enter first number: ";
cin >> num1;
cout << "Enter operator (+, -, *, /): ";
cin >> op;
cout << "Enter second number: ";
cin >> num2;
switch(op) {
case '+':
cout << num1 + num2;
break;
case '-':
cout << num1 - num2;
break;
case '*':
cout << num1 * num2;
break;
case '/':
if (num2 != 0)
cout << num1 / num2;
else
cout << "Cannot divide by zero!";
break;
default:
cout << "Invalid operation";
break;
}
return 0;
}
This simple calculator serves as a practical application of the concepts covered, demonstrating user input, conditional logic, and arithmetic operations.
Common C++ Libraries and Frameworks
Numerous libraries and frameworks extend the functionality of C++. Popular libraries include:
- Boost: Offers a set of libraries for tasks like linear algebra, multithreading, and networking.
- Qt: A framework for developing GUI applications with extensive tools and libraries.
- SDL: Simple DirectMedia Layer, primarily used for game development.
Familiarizing yourself with these libraries will exponentially enhance your C++ capabilities.
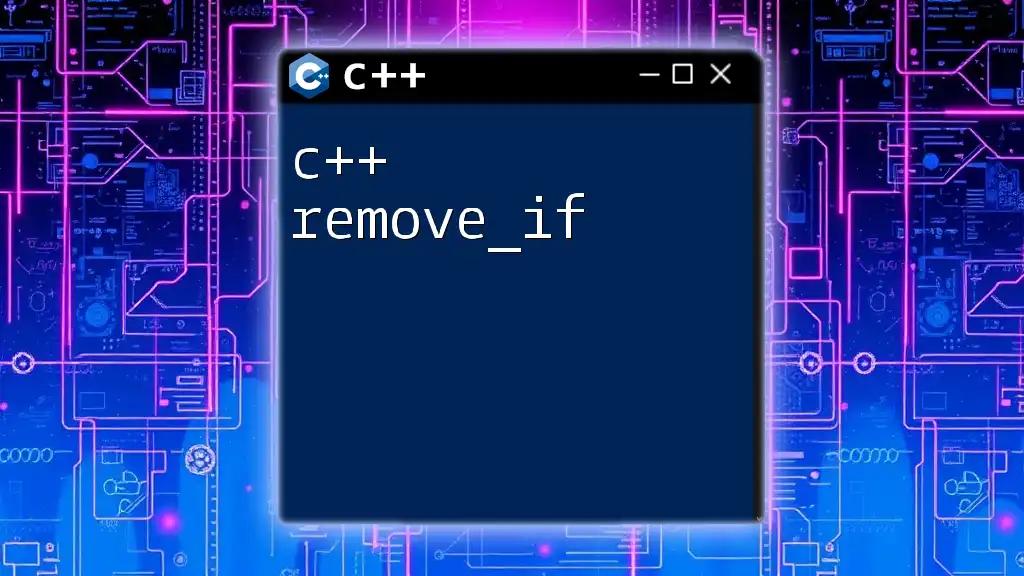
Tips and Tricks for Mastering C++
Best Practices in C++ Programming
To write clean and maintainable code, consider the following best practices:
- Follow Coding Standards: Adhere to established coding styles, making your code readable and maintainable.
- Comment Your Code: Use comments effectively to explain complex logic, making future code reviews easier.
- Utilize Version Control: Track changes and collaborate efficiently using tools like Git.
Resources for Further Learning
To continue your learning journey in C++, consider exploring:
- Online Courses: Platforms like Udemy, Coursera, or edX offer comprehensive C++ courses.
- Books: Classics like "C++ Primer" or "Effective C++" provide in-depth coverage of the language.
- Practice: Engage with coding communities on platforms like GitHub and Stack Overflow to learn from experienced developers.
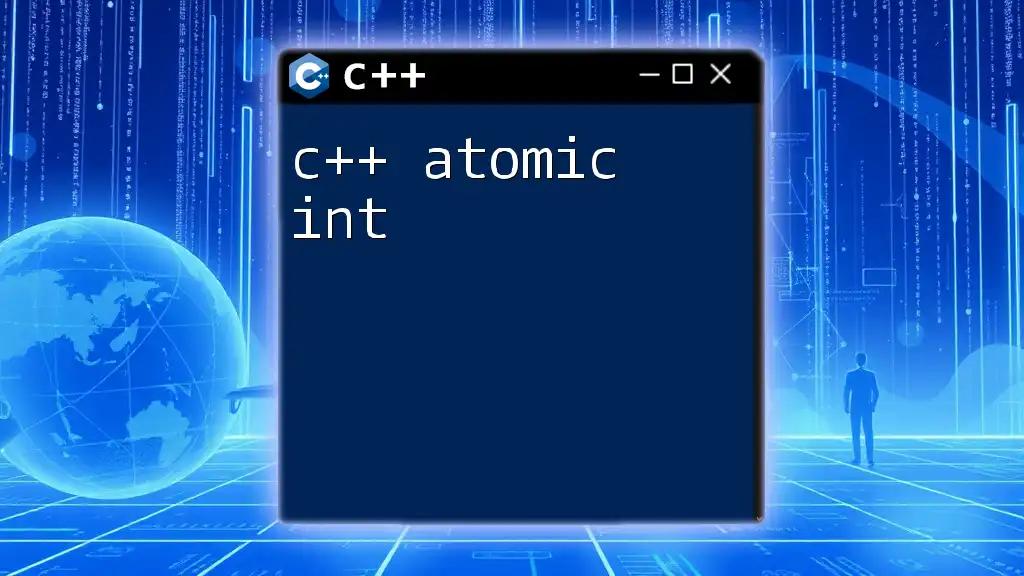
Conclusion
C++ remains an incredibly powerful and versatile programming language, offering an array of features that contribute to its awesomeness. From mastering the basics to exploring advanced concepts, your journey in C++ will enable you to develop robust applications efficiently.
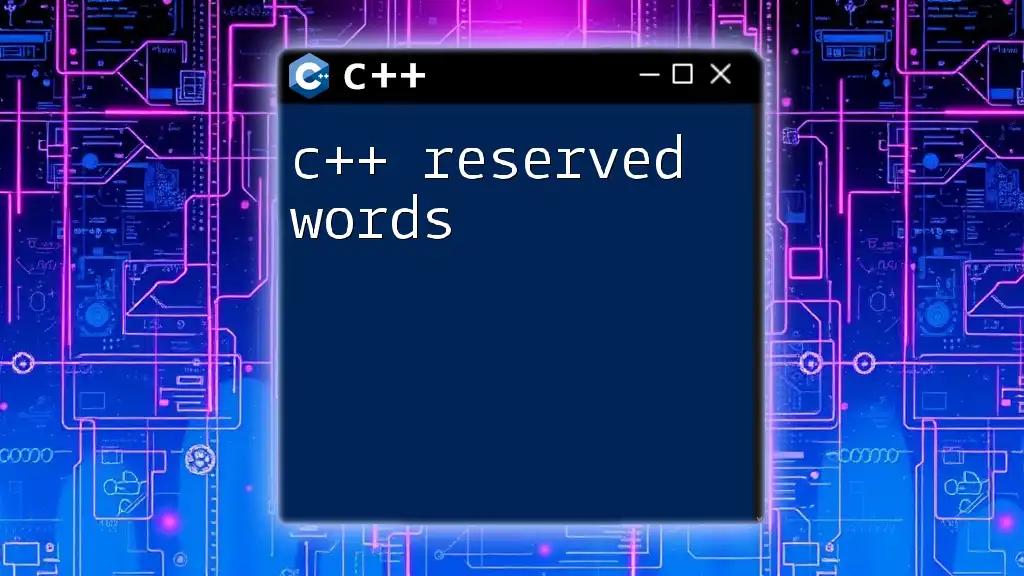
Call to Action
Are you ready to dive deeper into the world of C++? Join our classes to learn the intricacies of C++ programming in a concise and efficient manner. With hands-on experience and expert guidance, you'll be well on your way to mastering C++ awesome!