In C++, a derived class constructor can explicitly call a base class constructor to initialize inherited member variables, as shown in the following example:
#include <iostream>
using namespace std;
class Base {
public:
Base(int x) {
cout << "Base constructor called with value: " << x << endl;
}
};
class Derived : public Base {
public:
Derived(int x) : Base(x) { // Calling base class constructor
cout << "Derived constructor called with value: " << x << endl;
}
};
int main() {
Derived obj(10);
return 0;
}
Understanding Constructors
What is a Constructor?
In C++, a constructor is a special member function that is automatically called when an object of a class is created. Its primary purpose is to initialize the object's data members. Unlike regular member functions, constructors have the same name as the class and do not have a return type, not even void.
Types of Constructors
-
Default Constructor: A constructor that takes no parameters is called a default constructor. It initializes the object with default values.
-
Parameterized Constructor: A constructor that accepts arguments is known as a parameterized constructor, allowing for more flexibility in object initialization.
-
Copy Constructor: This constructor initializes an object using another object of the same class, typically for creating a copy.
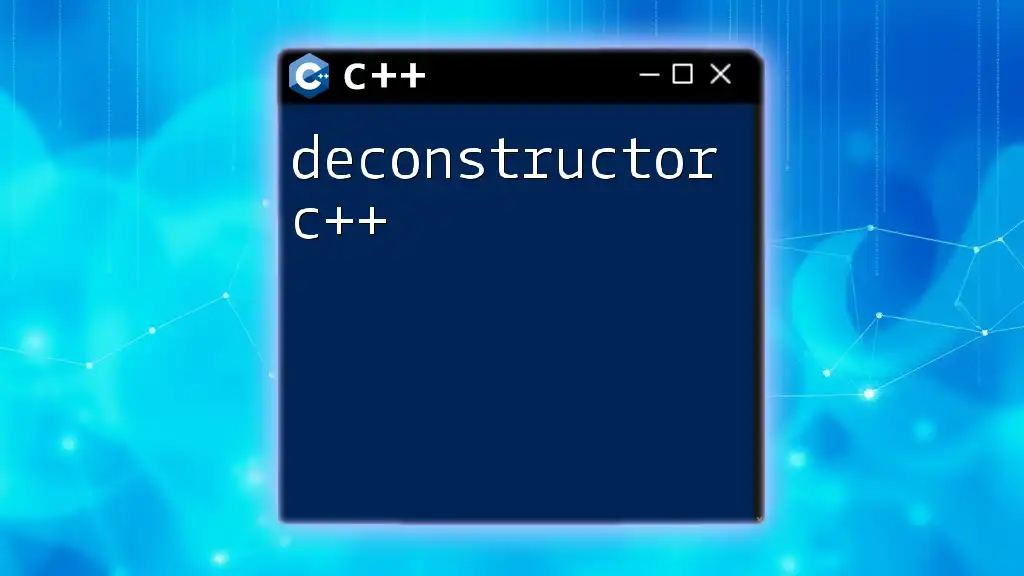
Derived Class Constructor in C++
What is a Derived Class?
A derived class in C++ is a class that inherits attributes and methods from another class, known as the base class. This allows for code reusability and structuring code with a hierarchy. Utilizing derived classes is a fundamental principle of inheritance in object-oriented programming.
The Role of Constructors in Inheritance
In a hierarchy of classes, constructor invocation behaves differently. When an object of a derived class is created, the constructor of its base class is called first, followed by the constructor of the derived class itself. This order is crucial because it ensures that the base portion of the derived class is initialized before the derived class's additional properties.
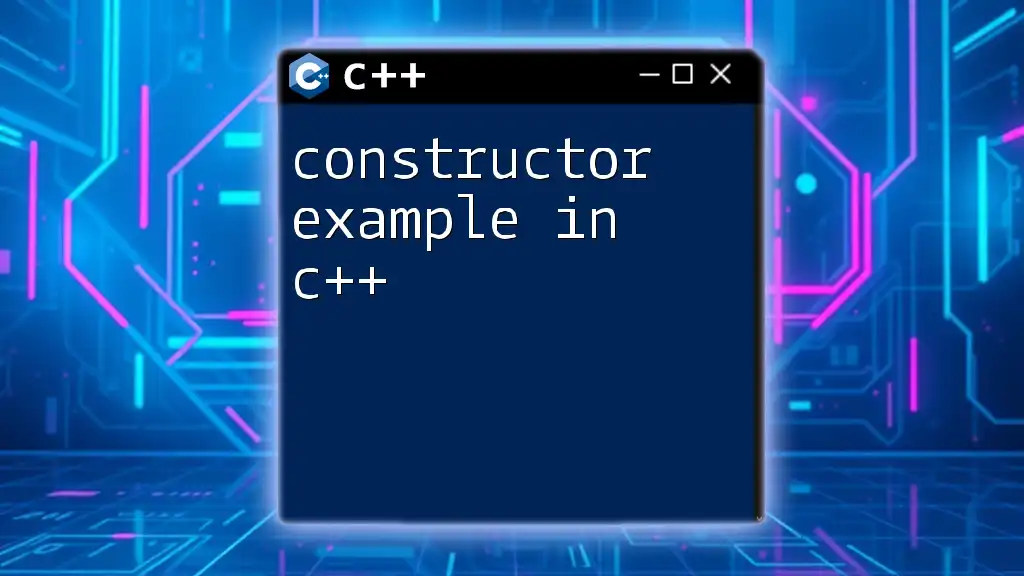
Constructing a Derived Class
Syntax of a Derived Class Constructor
When defining a derived class constructor, you specify the base class constructor that should be called. This is done using an initialization list, which follows the constructor's parentheses.
Calling Base Class Constructors
Default Base Class Constructor
When you create a derived class object that does not require any parameters for the base class, the default constructor of the base class is implicitly invoked. Here's how it works:
class Base {
public:
Base() {
cout << "Base class default constructor called." << endl;
}
};
class Derived : public Base {
public:
Derived() {
cout << "Derived class constructor called." << endl;
}
};
When you create an object of `Derived`, the output will be:
Base class default constructor called.
Derived class constructor called.
This shows the sequence in which constructors are called, highlighting the initialization flow from base to derived.
Parameterized Base Class Constructor
In cases where the base class requires parameters for its constructor, the derived class must explicitly call it using an initialization list. For example:
class Base {
public:
Base(int x) {
cout << "Base class parameterized constructor called with value: " << x << endl;
}
};
class Derived : public Base {
public:
Derived(int y) : Base(y) {
cout << "Derived class constructor called." << endl;
}
};
In this code, when you create an object of the `Derived` class with an integer argument, it will output:
Base class parameterized constructor called with value: [value]
Derived class constructor called.
This illustrates how the derived class constructor initializes the base class with the specified values, ensuring the correct setup of the inheritance framework.
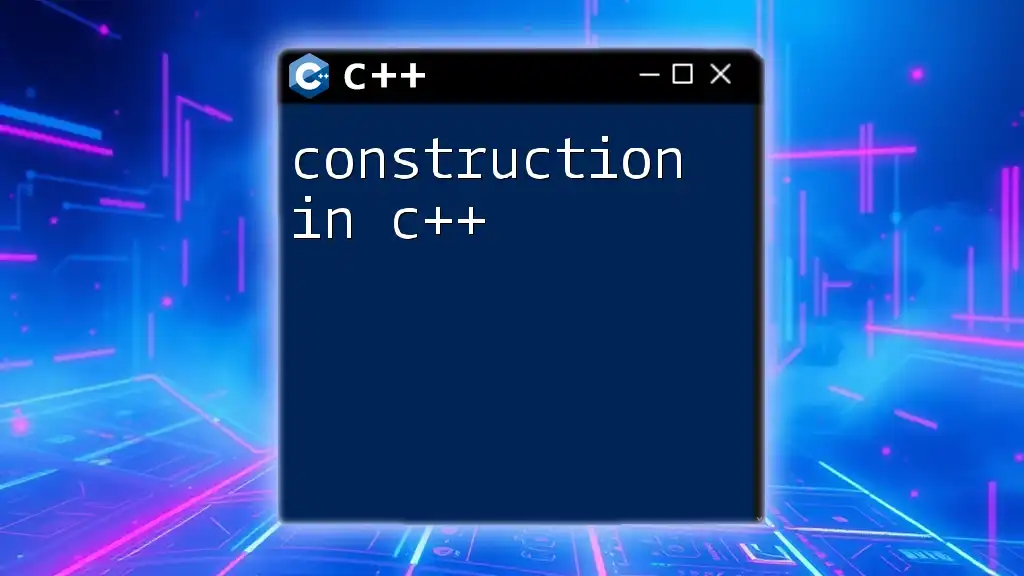
Example: Custom Constructors in a Derived Class
Creating a Base Class
Let's consider a practical example. We'll create a base class representing an animal, which will hold an animal's name.
class Animal {
public:
Animal(string name) {
cout << name << " created." << endl;
}
};
Extending with a Derived Class
Now, we'll extend the `Animal` class with a `Dog` class that adds dog-specific features:
class Dog : public Animal {
public:
Dog(string name) : Animal(name) {
cout << "Dog instantiated." << endl;
}
};
Putting It All Together
By integrating both classes, we can observe the full behavior of constructors upon object instantiation:
int main() {
Dog myDog("Rover");
return 0;
}
When you run this program, it outputs:
Rover created.
Dog instantiated.
This demonstrates the complete sequence of constructor calls—from the base class to the derived class—showing how constructors work together during object initialization.
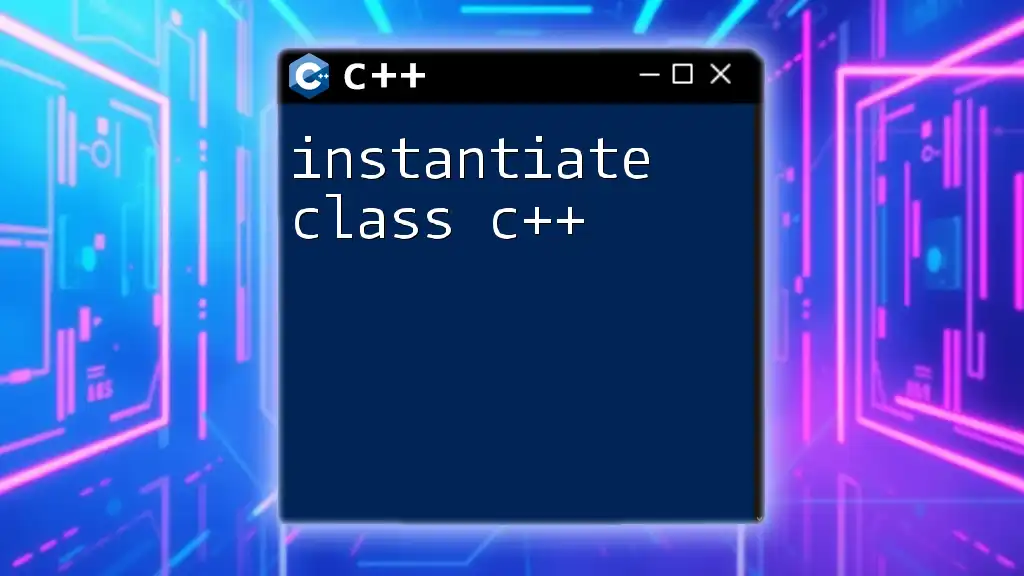
Important Considerations
Destructor in Derived Class
A derived class can have its own destructor, which is important for resource management. When a derived class object is destroyed, the destructor for the derived class is called first, followed by the base class destructor.
For instance:
class Base {
public:
Base() {}
~Base() {
cout << "Base class destructor called." << endl;
}
};
class Derived : public Base {
public:
Derived() {}
~Derived() {
cout << "Derived class destructor called." << endl;
}
};
Creating and deleting an object of the `Derived` class results in:
Derived class destructor called.
Base class destructor called.
This order is essential to ensure that derived class resources are released before base class resources.
Virtual Destructors
When dealing with inheritance, it's crucial to declare destructors as virtual in the base class. This ensures that the correct destructor is called for derived classes when an object is deleted through a base class pointer.
class Base {
public:
virtual ~Base() {
cout << "Base class destructor called." << endl;
}
};
class Derived : public Base {
public:
~Derived() {
cout << "Derived class destructor called." << endl;
}
};
This step guarantees that no resources are leaked and that the derived class's destructor is executed properly before the base class destructor.
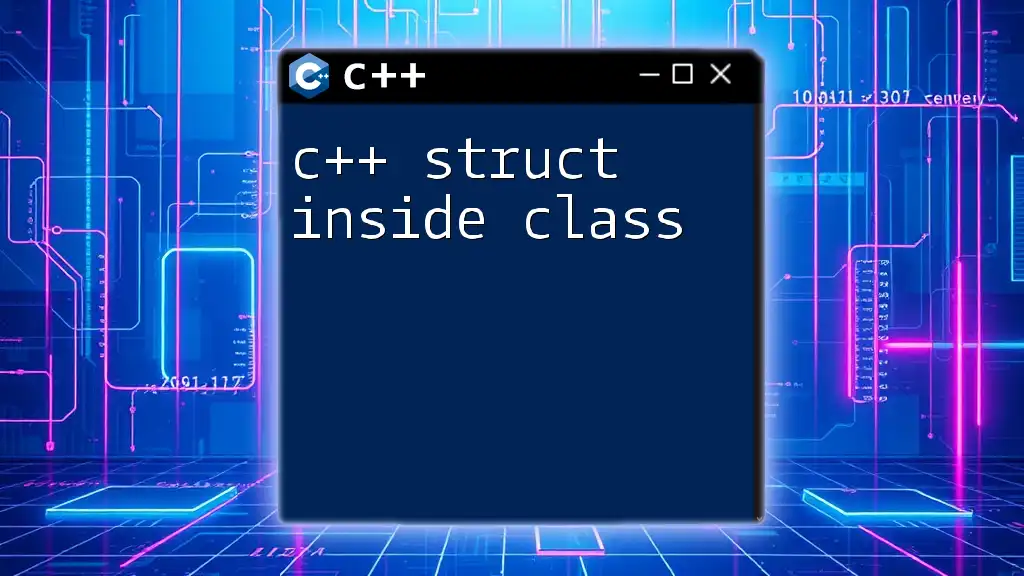
Conclusion
Understanding the use of constructors in derived class c++ is vital for effective memory management and object initialization in C++. By grasping how base class constructors are invoked and the significance of object life cycles—including destructors—you can better design robust, maintainable code.
Explore this further to deepen your knowledge, and consider joining our course for concise and effective learning in C++.