In C++, a deleted function is a function that cannot be invoked, typically to prevent certain operations on objects of a class, such as copy or move operations, which can be explicitly deleted to enforce rules about object management.
Here's a code snippet demonstrating the use of a deleted function:
#include <iostream>
class MyClass {
public:
MyClass() = default;
MyClass(const MyClass&) = delete; // Delete copy constructor
MyClass& operator=(const MyClass&) = delete; // Delete copy assignment operator
};
int main() {
MyClass obj1;
// MyClass obj2 = obj1; // Error: use of deleted function
// MyClass obj3;
// obj3 = obj1; // Error: use of deleted function
return 0;
}
What is a Deleted Function?
A deleted function in C++ is a way to specify that a function cannot be used. By declaring a function as deleted, you instruct the compiler to prevent any attempts to invoke it, thereby allowing developers to control usage more rigorously. The basic syntax involves using the `= delete` specifier after the function declaration.
Purpose of Deleted Functions
Deleted functions serve multiple purposes in C++, including:
- Preventing unwanted function calls: By deleting certain functions, you ensure that they cannot be invoked, avoiding potential runtime errors.
- Controlling object behavior: They help enforce certain behaviors of classes, maintaining integrity and reliability.
- Ensuring safety and integrity of code: By disallowing dangerous operations, you make your code safer and more maintainable.
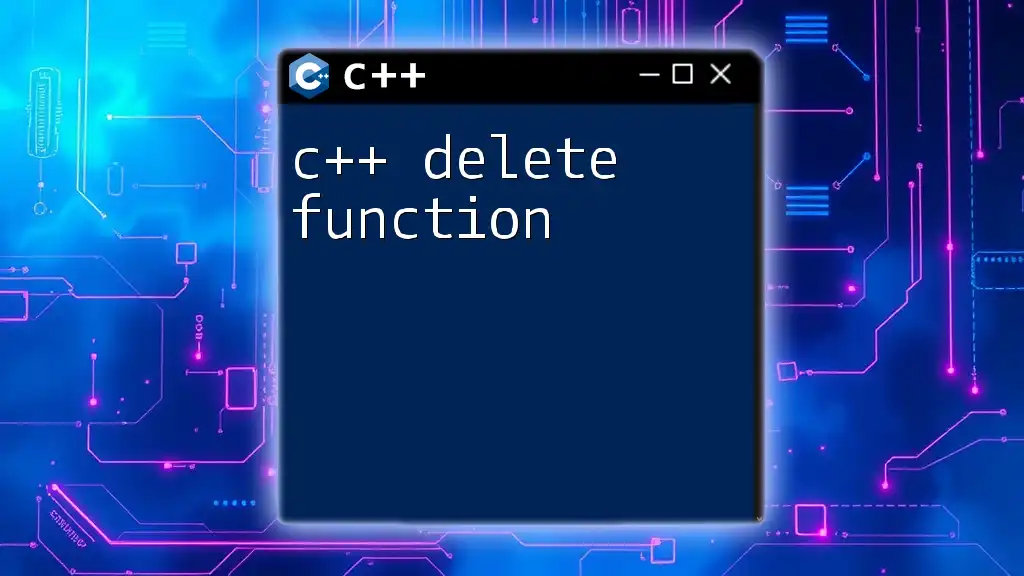
When to Use Deleted Functions
Disallowing Copying and Assignment
One of the most common scenarios for using deleted functions is to prevent copying and assignment of objects. When a class defines resources that are meant to be unique and not duplicated, copying can lead to resource management issues, such as double deletions or memory leaks. The solution is to delete both the copy constructor and the assignment operator.
Here's an example:
class NonCopyable {
public:
NonCopyable() = default;
// Disable copy constructor
NonCopyable(const NonCopyable&) = delete;
// Disable assignment operator
NonCopyable& operator=(const NonCopyable&) = delete;
};
With the above class, attempting to copy or assign a `NonCopyable` instance will result in a compile-time error, clearly indicating the intention of the implementation.
Preventing Default Initialization
In some cases, you may want to avoid the creation of an object without an explicitly defined initial state. By deleting the default constructor, you can enforce that users of your class must supply necessary parameters at construction.
For instance:
class NoDefault {
public:
NoDefault() = delete; // Prevent default construction
NoDefault(int value) : value(value) {}
private:
int value;
};
This class now cannot be created using a default constructor, forcing users to provide an integer value during instantiation.
Making Certain Functionality Private
Another important use of deleted functions is to restrict access to specific functionalities while keeping others available. This is part of the encapsulation principle, promoting cleaner and more manageable code.
class PrivateMethod {
public:
void allowedFunction() {
// some logic
}
void disallowedFunction() = delete; // Prevent this function from being called
};
In this example, `allowedFunction` remains public and can be accessed, while `disallowedFunction` cannot be invoked, enforcing better control over class usage.
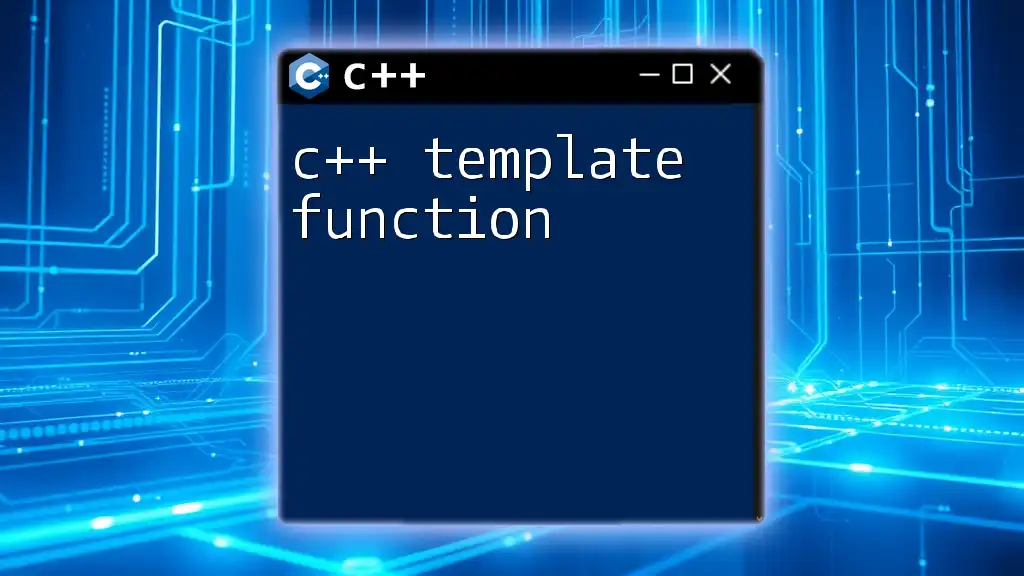
How to Delete Functions in C++
Syntax Overview
The syntax for deleting functions is straightforward. You declare a function as you normally would, appending `= delete` to the function signature. This clear indication signals to the compiler and other developers about the intended restrictions.
Implicitly Deleted Functions
The compiler also implicitly deletes certain functions under specific circumstances. For example, if a class contains a member that's not copyable or non-movable, the compiler automatically deletes the copy constructor, copy assignment operator, and in certain cases, even the move constructor and move assignment operator.
Understanding these implicit deletions can help clarify why certain constructors and operators are unavailable, leading to more effective debugging.
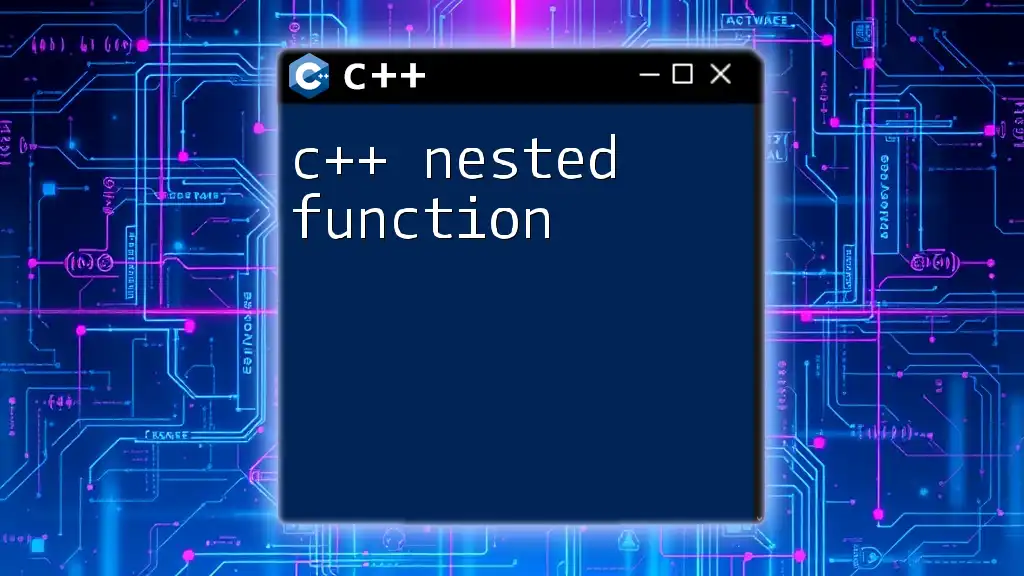
Error Handling with Deleted Functions
Compiler Errors
When a deleted function is called, the compiler will generate an error indicating that the function cannot be used. This error serves as a helpful reminder of the intended restrictions and can guide you towards correcting misuse.
Using `static_assert` for Safety Checks
To further enhance safety, you can use `static_assert` to ensure that certain conditions hold true at compile time, preventing unwanted use of deleted functions. This technique is especially useful in template classes.
For example:
template<typename T>
class SmartPointer {
public:
SmartPointer(T* ptr) : ptr_(ptr) {}
SmartPointer(const SmartPointer&) = delete; // Prevent copying
private:
T* ptr_;
};
In this implementation of a smart pointer, copying is deleted to ensure that ownership semantics are strictly maintained, ultimately safeguarding resource management.
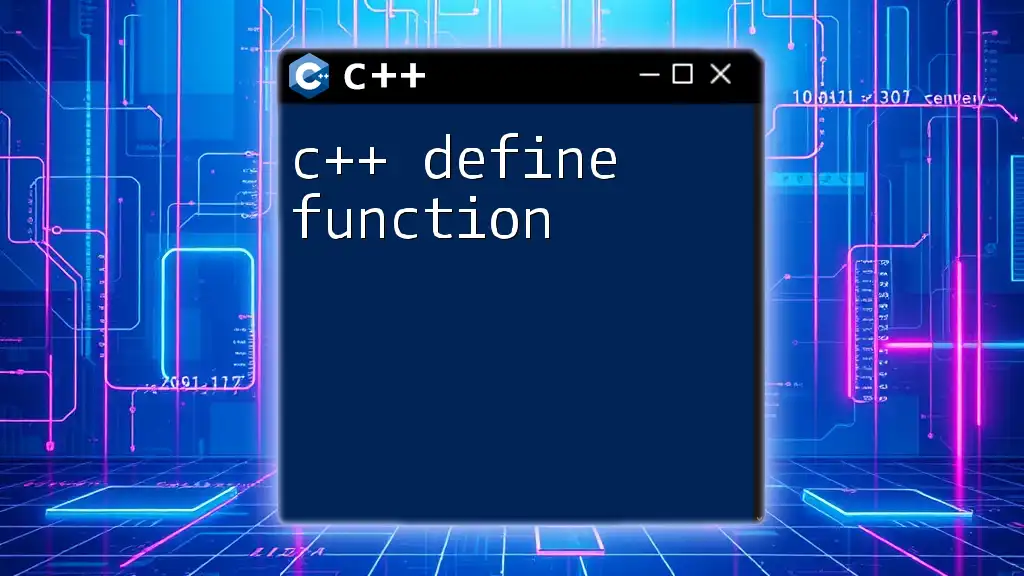
Pros and Cons of Using Deleted Functions
Advantages
- Enforcing strong type safety: By disallowing specific operations, you can design safer interfaces, ultimately preventing misuse by developers.
- Improving readability and maintainability: Clearly declared deletions provide context, making it easier for others to understand your intentions within the code.
Disadvantages
- Learning curve for beginners: New developers may find the concept of deleted functions challenging, leading to misunderstanding and incorrect usage.
- Potential for increased complexity in larger systems: Excessive use of deleted functions can contribute to confusion, especially if not well-documented.
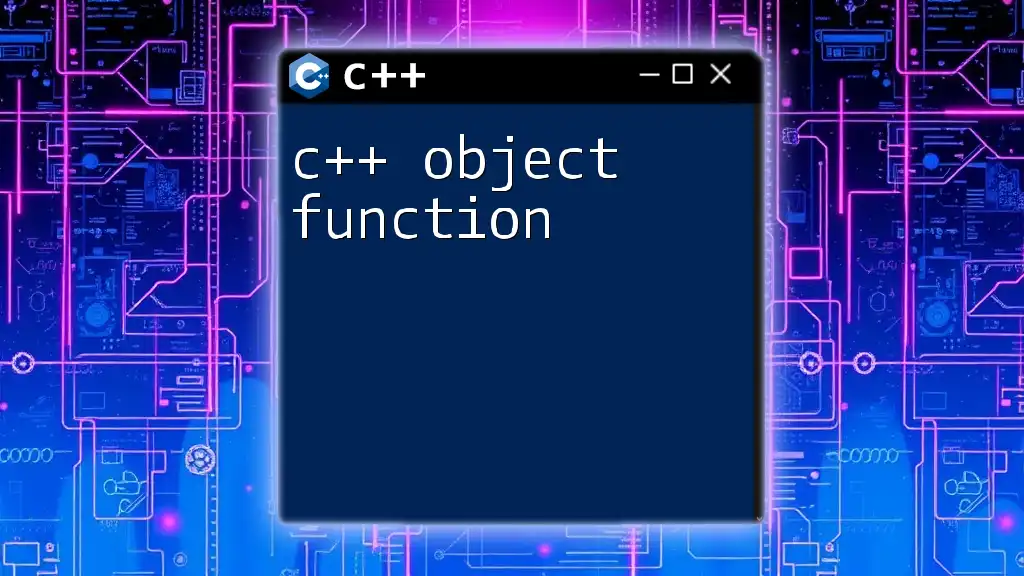
Best Practices for Using Deleted Functions
Clear Documentation
It's crucial to document why certain functions are deleted. Clear comments and documentation prevent misinterpretation and help other developers understand design choices.
Consistent Usage
Establishing guidelines for when and where to apply deleted functions can streamline coding practices, making codebases more uniform and easier to understand.
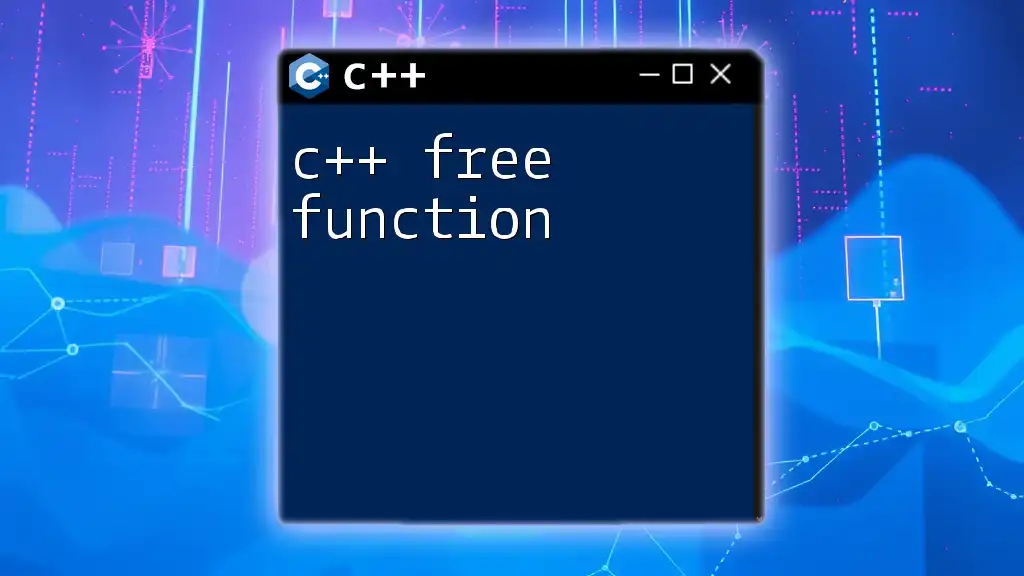
Real-World Applications
Examples in Standard Libraries
The C++ Standard Library provides multiple instances of deleted functions. For example, smart pointers like `std::unique_ptr` and `std::shared_ptr` use deleted functions to manage resource ownership safely and effectively.
Case Study: Custom Resource Management
Consider a custom resource manager that utilizes deleted functions to prevent copying and assignment. By imposing these restrictions, you ensure the uniqueness of resources being managed, leading to cleaner and safer resource handling.
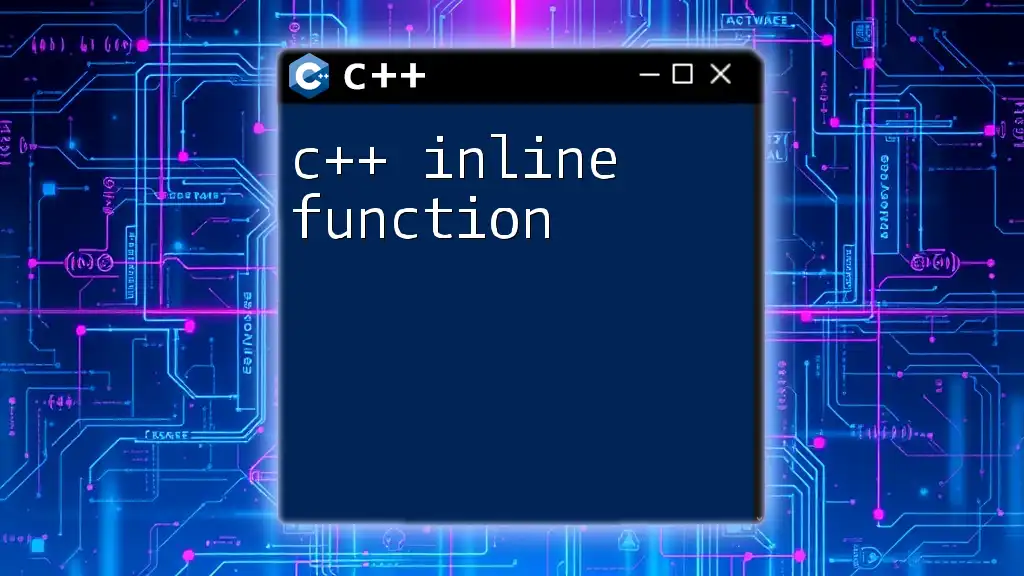
Conclusion
The C++ use of deleted functions provides developers with powerful tools to enforce usability constraints, enhance type safety, and manage resource integrity. By understanding their purpose and application, you can create more robust and maintainable code. As you integrate this knowledge into your C++ practices, take the time to document your design choices and maintain consistency throughout your codebase. Happy coding!
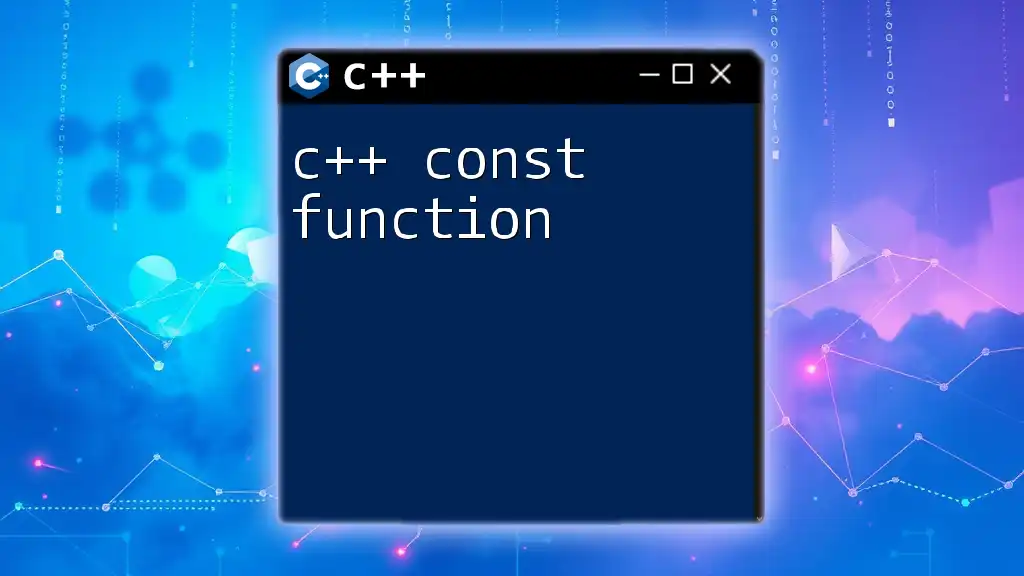
Additional Resources
For more information on C++ functions and best practices, explore further reading materials related to C++ standards, best coding practices, and advanced topics in object-oriented programming.