C++ can be utilized in Unity through the use of native plugins, enhancing performance for compute-intensive tasks or integrating existing C++ libraries.
// Example: Simple C++ function to add two numbers
extern "C" {
__declspec(dllexport) int Add(int a, int b) {
return a + b;
}
}
Can You Use C++ in Unity?
Understanding Unity's Primary Language
Unity primarily uses C# as its scripting language. C# is designed to be easy to learn and allows for rapid game development, which is why it has become the language of choice for most Unity developers. Unity's extensive API and built-in tools are tailored for C#, simplifying the development process significantly.
The Role of C++
While C# is dominant, integrating C++ into Unity can be advantageous in specific scenarios. C++ is a powerful language known for its performance capabilities and low-level memory management, which can be crucial for resource-intensive applications such as complex physics calculations or gameplay mechanics where performance bottlenecks may occur.
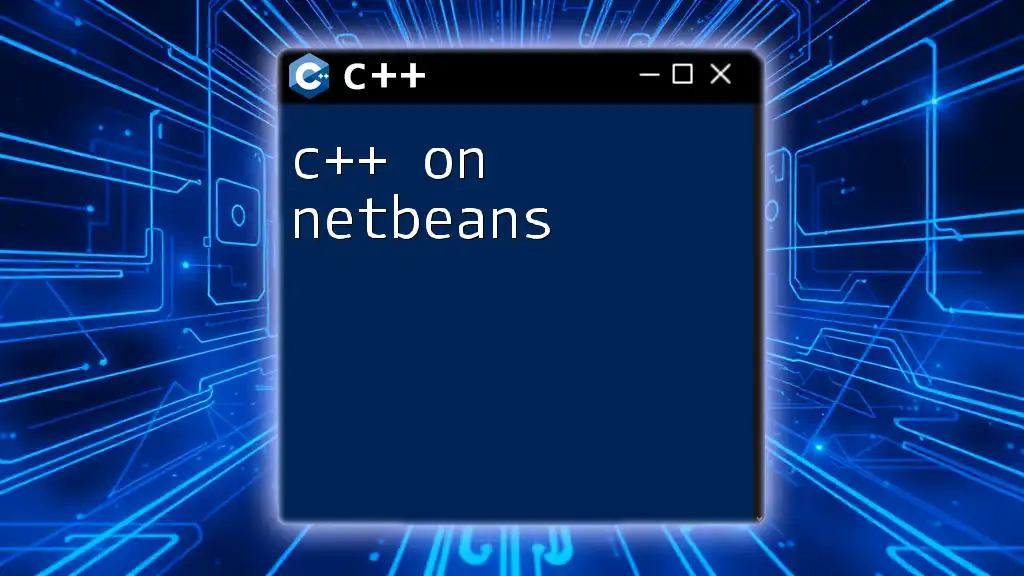
C++ Unity: How to Set It Up
Prerequisites
Before getting started, ensure you have the following software installed:
- Unity Hub and Unity Editor
- Visual Studio (with C++ development tools)
- Basic understanding of both C++ and C#.
Setting Up Your Project
- Create a New Unity Project: Open Unity Hub, create a new project, and select your desired settings.
- Integrate C++ into Your Unity project: The integration is mainly achieved through native plugins. This means you'll be creating a shared library that Unity can call from C# scripts.
Creating a Native Plugin
What is a Native Plugin?
A native plugin is a shared library written in C++ that can be used by Unity. This allows developers to harness native code’s performance while taking full advantage of the Unity ecosystem.
Step-by-Step Guide to Creating a Simple C++ Plugin
-
Set Up a C++ Project in Visual Studio:
- Open Visual Studio and create a new project.
- Select "Class Library" under C++ to create a shared library.
-
Write a Simple C++ Function: Create a function to perform a simple task, such as adding two integers.
extern "C"
{
__declspec(dllexport) int Add(int a, int b)
{
return a + b;
}
}
The `extern "C"` linkage is essential for C++ functions when exporting them to ensure they use C-style name mangling, making them callable from C#.
- Compile the Project: Build the project, which generates a `.dll` file that can be utilized in Unity.
Importing the Plugin into Unity
- Import the Compiled .dll: Drag and drop your generated `.dll` file into your Unity project's "Assets" folder.
- Set the Plugin for Different Platforms: In the Inspector window, on the `.dll` file, specify which platforms the plugin should target (e.g., Windows, macOS).
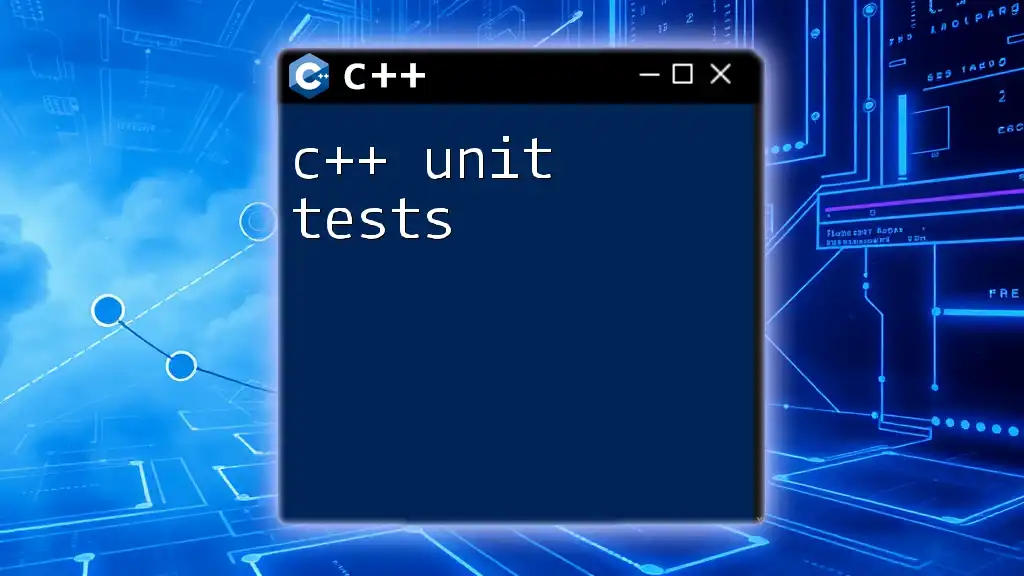
Does Unity Use C++ Behind the Scenes?
Unity's Architecture Overview
Although C# is the face of Unity scripting, the engine itself is built on a foundation of C++. Performance-critical components such as graphics rendering, physics simulation, and other intensive calculations are implemented in C++. This architectural choice allows Unity to manage resources efficiently and deliver high-performance gaming experiences.
Benefits of Using C++ in Unity
Utilizing C++ in Unity offers several distinct advantages:
- Performance Efficiency: C++ is known for its speed and efficiency, especially in computation-heavy tasks.
- Access to Low-Level System Resources: Developers can tap into hardware-level optimizations.
- Integration with Existing C++ Libraries: You can leverage third-party C++ libraries, giving you access to pre-existing functionalities for tasks like image processing or encryption.
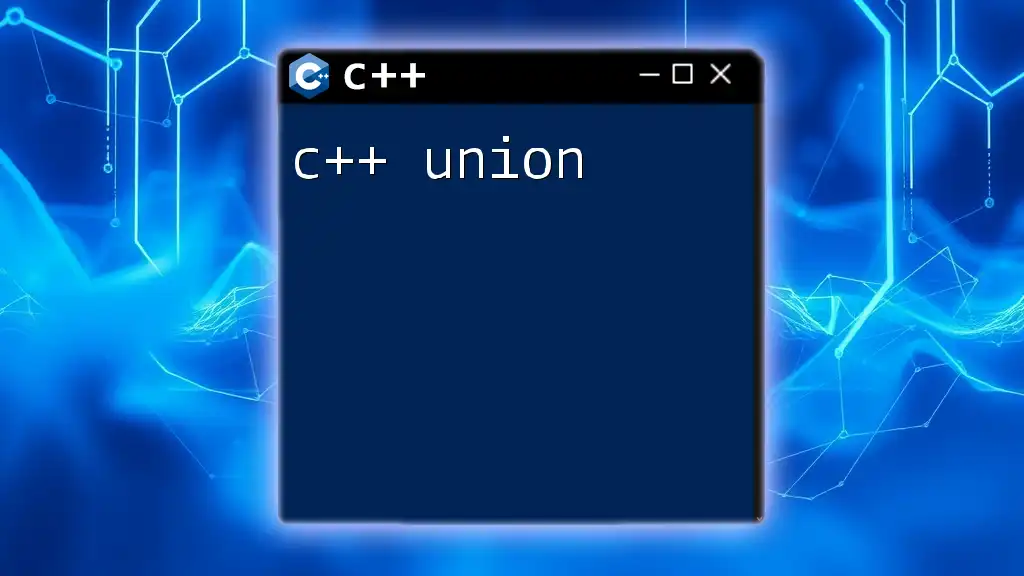
Unity C++: Code Examples and Use Cases
Example 1: Simple Math Operations
Once your C++ plugin is set up, you can call its functions from C# scripts. Here’s how to invoke the previously created `Add` function:
using System.Runtime.InteropServices;
using UnityEngine;
public class MathExample : MonoBehaviour
{
[DllImport("YourPluginName")]
private static extern int Add(int a, int b);
void Start()
{
int result = Add(3, 4);
Debug.Log("Result from C++: " + result);
}
}
This sample demonstrates how to use the `[DllImport]` attribute to call a C++ function directly from C#.
Example 2: Using C++ for Complex Gameplay Mechanics
Consider a scenario where you need to compute physics calculations that involve significant computational overhead. In such cases, using C++ can shift the burden from C# and enhance performance, allowing the main game loop to run smoother.
Example 3: Interfacing with External C++ Libraries
Integrating third-party C++ libraries into Unity can be beneficial, especially for specialized tasks. For example, if you're utilizing OpenCV for computer vision applications, you can create a C++ plugin that wraps the library, enabling your Unity game to call CV functions seamlessly.
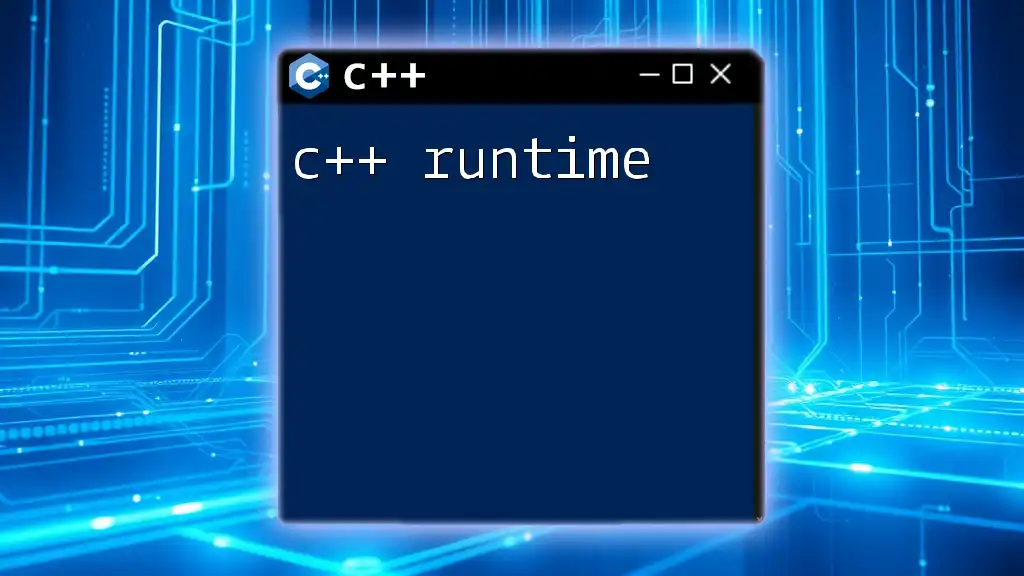
Best Practices for Using C++ in Unity
When to Choose C++ Over C#
Knowing when to use C++ versus C# is key to optimizing your Unity projects. Typically, you should consider C++ for:
- Performance-intensive tasks.
- Reusing existing C++ libraries.
- Implementing custom plugins for specialized functionality.
Debugging C++ Code in Unity
Debugging C++ code can be more complex than debugging C#. Utilize Visual Studio’s debugging tools to step through your C++ code, set breakpoints, and check variable values. Make sure your Visual Studio project is configured for debugging to catch any issues effectively.
Common Pitfalls and How to Avoid Them
Be mindful of the data types you use and ensure you are managing memory correctly to prevent leaks. Additionally, test your C++ code thoroughly to catch any issues before integrating it into your main project.
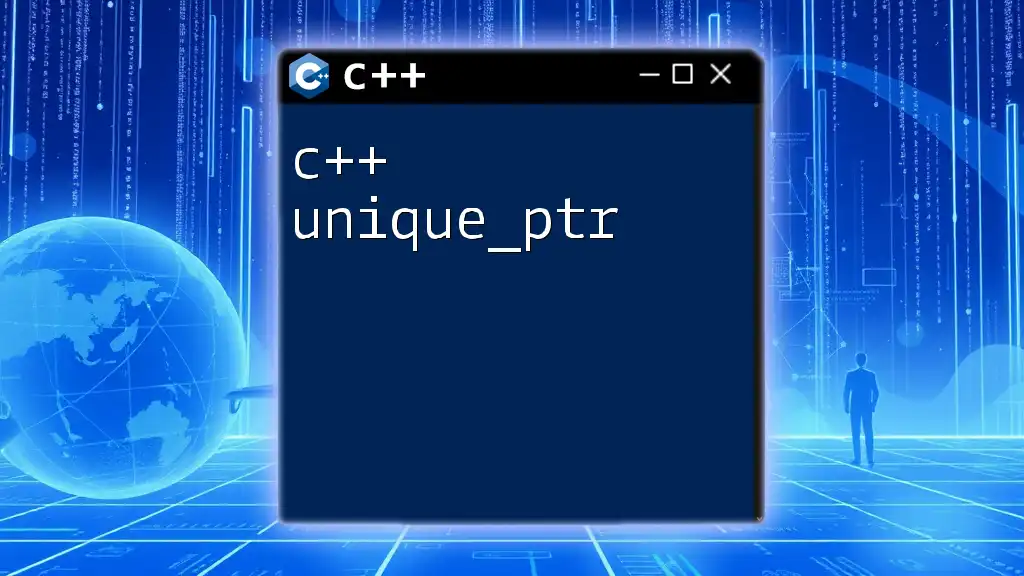
Conclusion
Incorporating C++ on Unity opens up a realm of possibilities that blend high-level ease of C# with the performance prowess of C++. By creating native plugins, leveraging low-level system resources, and optimizing critical game components, developers can enhance their Unity projects significantly. As you embark on the journey of integrating C++, remember to weigh the advantages carefully and apply best practices to unlock the full potential of your game development efforts.
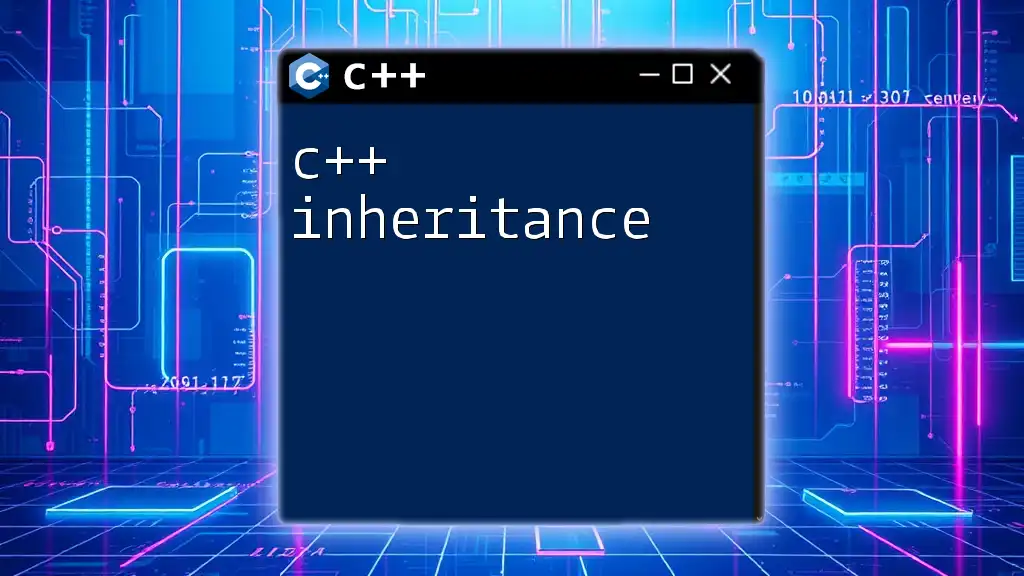
Additional Resources
For further learning and mastery, check the official Unity documentation, join community forums, and explore tutorials that delve deeper into the integration of C++ with Unity. Consider examining performance optimization techniques and more advanced use cases to elevate your skills in leveraging both languages effectively.