The `C++ _countof` macro is used to determine the number of elements in a statically allocated array, providing a simple and effective way to calculate its size.
#include <iostream>
#define _countof(array) (sizeof(array) / sizeof(array[0]))
int main() {
int arr[] = {1, 2, 3, 4, 5};
std::cout << "Number of elements in arr: " << _countof(arr) << std::endl; // Outputs: 5
return 0;
}
What is _countof?
Definition
The `c++ _countof` is a macro designed to determine the number of elements in a static array. It operates by utilizing the `sizeof` operator to calculate the total size of the array and then dividing that by the size of an individual element. This calculation is crucial for ensuring that when we iterate over arrays, we don't exceed their bounds, which could lead to undefined behavior or runtime errors.
The key aspect distinguishing `_countof` from other methods, such as using a hard-coded size or manual counting, is its ability to dynamically assess the size during compilation. This provides a layer of safety and flexibility when working with arrays.
Historical Context
The `_countof` macro is rooted in C/C++ programming standards that emerged to streamline array management. Scholars and practitioners alike noted that managing array sizes frequently resulted in errors, leading to the creation of `_countof` as a convenient solution. It finds a place in many coding practices, aiding developers by abstracting the complexity of size calculations.
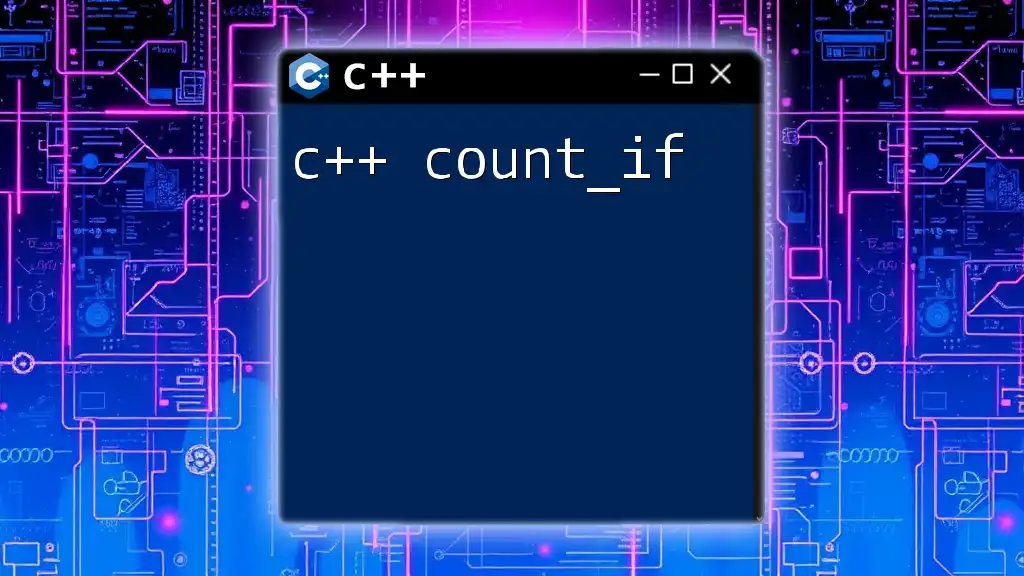
How to Use _countof
Syntax
The syntax for the `_countof` macro is straightforward. It is defined as follows:
#define _countof(arr) (sizeof(arr) / sizeof(arr[0]))
This definition breaks down to taking the total size of the array `arr` and dividing it by the size of an individual element `arr[0]`. The result is an integer representing the total number of elements.
Practical Examples
Counting Elements in an Array
Using the `_countof` macro is particularly useful when working with static arrays where sizes are known at compile-time. Below is a simple example demonstrating its usage:
#include <iostream>
#define _countof(arr) (sizeof(arr) / sizeof(arr[0]))
int main() {
int myArray[10];
std::cout << "Number of elements: " << _countof(myArray) << std::endl;
return 0;
}
In this example, `myArray` contains ten integers, and `_countof(myArray)` correctly evaluates to 10, allowing developers to avoid hardcoding the size.
Using with Multidimensional Arrays
The `_countof` macro can also be employed with multidimensional arrays, although the calculation becomes slightly more complex. Here's how it can be applied:
#include <iostream>
#define _countof(arr) (sizeof(arr) / sizeof(arr[0]))
int main() {
int my2DArray[3][4]; // 3 rows, 4 columns
std::cout << "Number of rows: " << _countof(my2DArray) << std::endl; // Outputs 3
return 0;
}
In this case, `_countof(my2DArray)` evaluates to the number of rows (3). However, note that `_countof` returns only the first dimension size, which is critical to remember when designing your array.
Common Pitfalls
Using with Pointers
It's vital to understand that `_countof` should not be used with pointers. This is because pointers do not carry size information about the array they are pointing to. Here's an example of a common mistake:
#include <iostream>
#define _countof(arr) (sizeof(arr) / sizeof(arr[0]))
int main() {
int* pArray = new int[10];
// std::cout << "Number of elements: " << _countof(pArray) << std::endl; // Incorrect
return 0;
}
In this code, `_countof(pArray)` would not yield the expected size; instead, it would generate compiler errors or unexpected behavior. It’s important to use `_countof` exclusively with static arrays.
Dynamic Arrays
The limitations of `_countof` extend to dynamically allocated arrays as well. For example:
#include <iostream>
#define _countof(arr) (sizeof(arr) / sizeof(arr[0]))
int main() {
int* dynamicArray = new int[10];
// std::cout << "Size: " << _countof(dynamicArray) << std::endl; // Incorrect
delete[] dynamicArray; // Good practice
return 0;
}
Given that `dynamicArray` does not retain size information after allocation, using `_countof` leads to confusion. Instead, always track the sizes of dynamically allocated arrays manually.
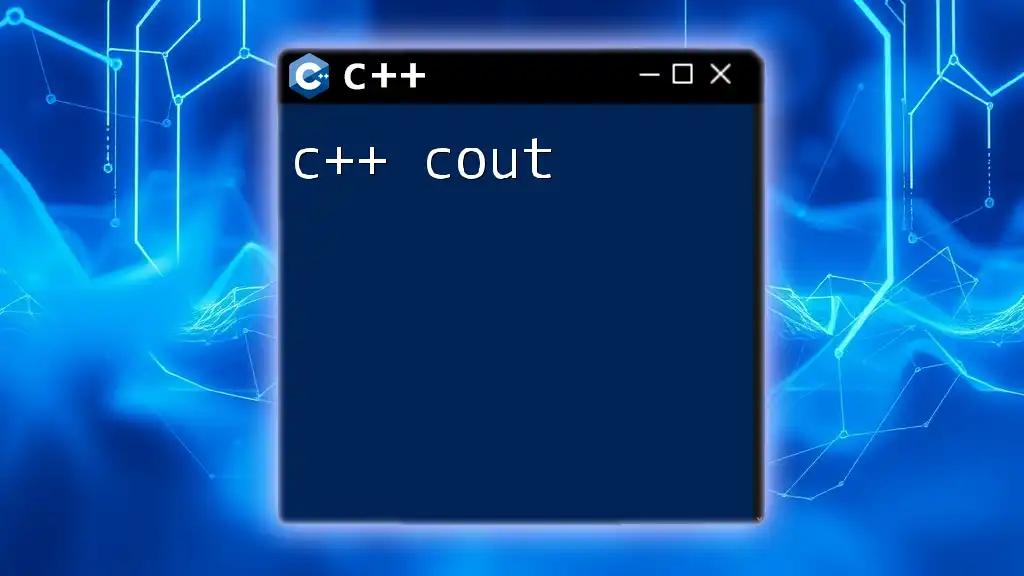
Alternatives to _countof
std::size
With the introduction of C++17, the standard library presents `std::size`, which provides a clearer and type-safe alternative to `_countof`. The usage of `std::size` is as follows:
#include <iostream>
#include <array>
int main() {
std::array<int, 10> arr = {0};
std::cout << "Number of elements using std::size: " << std::size(arr) << std::endl;
return 0;
}
Here, `std::size(arr)` provides the same functionality as `_countof`, but with improved safety and readability, thus minimizing the chances of errors.
Template Function for Array Size
Another alternative to `_countof` is utilizing a template function that guarantees the correct determination of the array size:
#include <iostream>
template <typename T, size_t N>
constexpr size_t array_size(T(&)[N]) noexcept {
return N;
}
int main() {
int myArray[10];
std::cout << "Number of elements: " << array_size(myArray) << std::endl;
return 0;
}
This approach not only calculates the size accurately but also works great with multidimensional arrays, retaining the advantages of type safety.
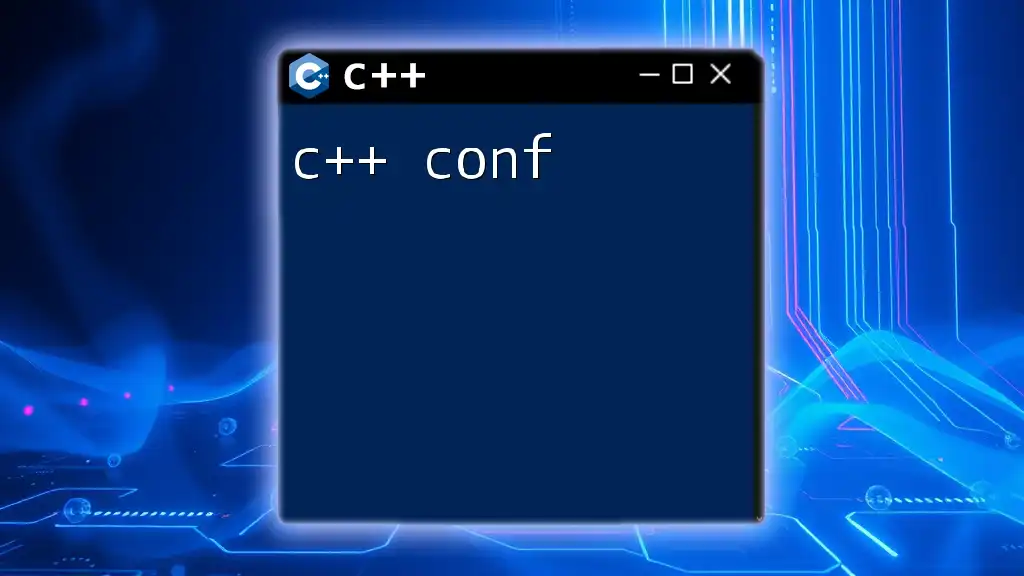
Best Practices
When to Use _countof
Using `c++ _countof` is appropriate in scenarios where you are working with fixed-size arrays and need a quick way to prevent overruns during iterations. It adds clarity to your code by emphasizing that the end of the array will be respected.
Avoiding Misuse
To avoid common pitfalls, always ensure that `_countof` is applied strictly to static arrays. Avoid using it with pointers or dynamically allocated arrays, as mentioned previously. Additionally, maintain clear documentation and comments within your code, noting the safety and limitations of `_countof`, especially for those who may read your code in the future.
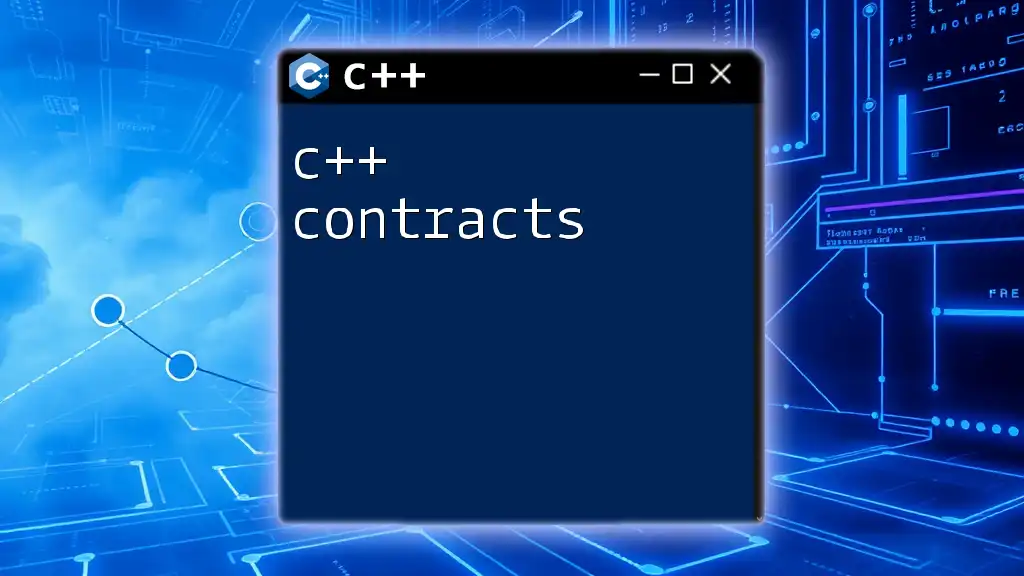
Conclusion
In summary, the `c++ _countof` macro serves as a valuable tool for developers working with static arrays in C++. By leveraging this mechanism, you can enhance both the safety and clarity of your code, ensuring that array boundaries are respected. While there are modern alternatives available, such as `std::size`, knowing how to use `_countof` remains a useful skill.
As you continue to explore C++, remember to experiment with the macro in various coding scenarios. Understanding its strengths and limitations will serve you well in crafting robust applications.
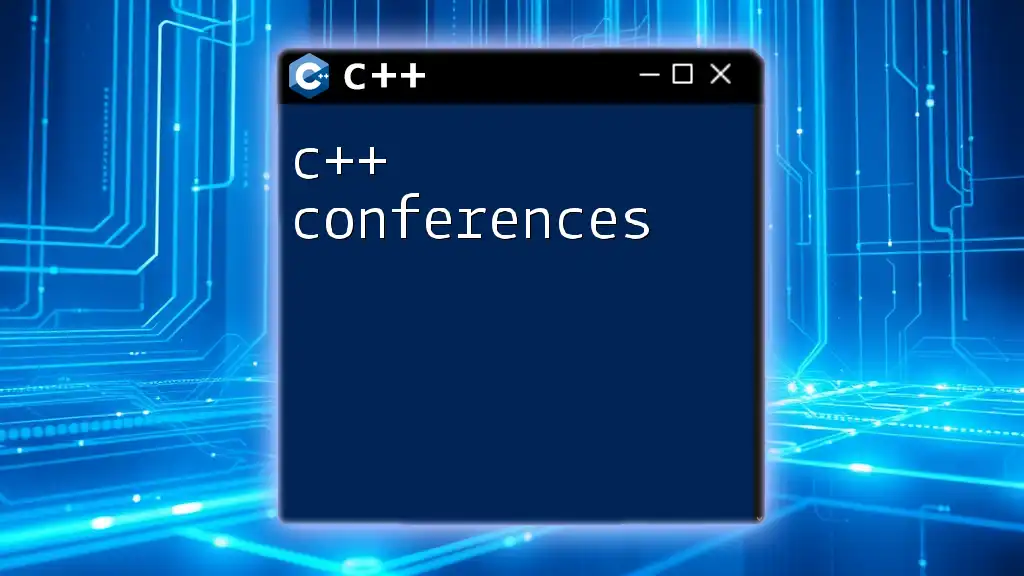
Additional Resources
- For more in-depth knowledge, refer to the official C++ documentation which outlines array operations and standards.
- Consider books like "The C++ Programming Language" by Bjarne Stroustrup for comprehensive learning.
- Engage with coding forums such as Stack Overflow for community support and additional guidance.
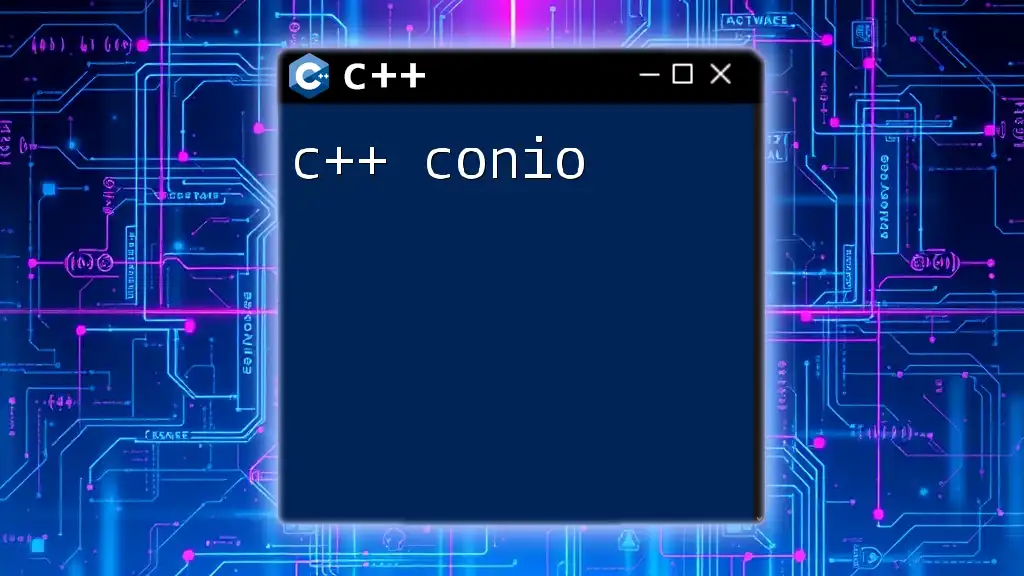
FAQs
What are the advantages of using _countof?
Using `c++ _countof` can enhance performance by providing compile-time calculations and can significantly reduce errors associated with manual size tracking.
Can I use _countof in my project?
You can use `_countof` in projects that heavily rely on static arrays but be careful not to mix it with pointers or dynamically allocated arrays.
Are there any replacements for _countof in modern C++?
Yes, alternatives like `std::size` and custom template functions provide improved safety and are more aligned with current C++ best practices.