In C++, a `struct` can be defined within a `class` to encapsulate related data, enabling organized and coherent data management.
class MyClass {
public:
struct MyStruct {
int id;
std::string name;
};
MyStruct data;
};
Overview of Structures in C++
What is a Struct?
In C++, a struct is a user-defined data type that allows you to group related variables of different types under a single name. Structs are mainly used to encapsulate data. The syntax for defining a struct is quite straightforward:
struct MyStruct {
int value;
std::string name;
};
One key characteristic of structs is that they have public access by default, meaning that their members can be accessed from outside the struct unless specified otherwise.
When to Use a Struct
Structs are typically preferred when the sole purpose is to hold data with minimal behavior. They are ideal for plain data structures without complex functionalities. Common use cases include representing simple data models, such as coordinates, points, or database records.
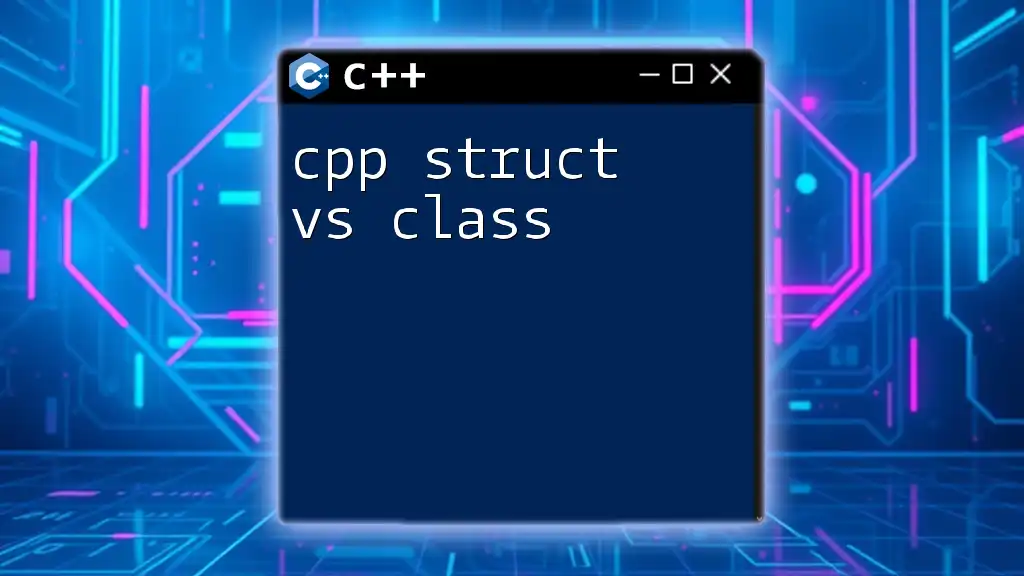
Creating Structs in a Class
Defining a Struct inside a Class
When you embed a struct within a class, you can extend the capabilities of that struct while still preserving its simplicity. Here's how you can define a struct inside a class:
class MyClass {
public:
struct MyStruct {
int value;
std::string name;
};
};
Accessing Struct Members
You can access and manipulate struct members from instances of the class. For example, to utilize the struct defined above, you would do the following:
MyClass::MyStruct myStruct;
myStruct.value = 10;
myStruct.name = "Example";
This illustrates how you can create an instance of the struct and set its values.
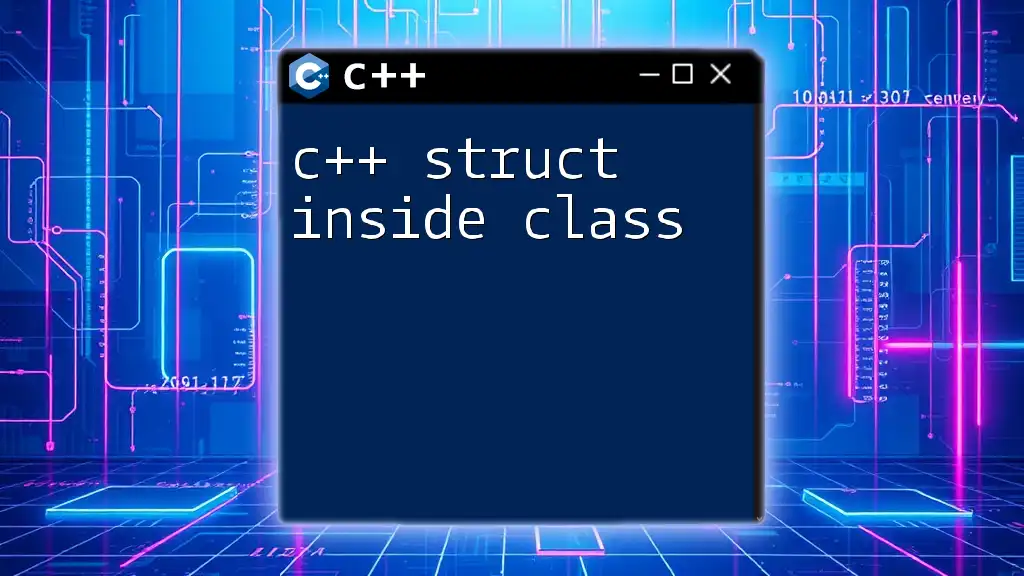
Practical Use Cases
Combining Structs and Classes
One of the greatest benefits of using structs within classes is the ability to create complex data structures. For instance, here’s how you can define a class with nested structs:
class Student {
public:
struct Address {
std::string street;
std::string city;
int zip;
};
Address studentAddress;
};
In this example, the `Student` class contains an `Address` struct, effectively grouping all address-related attributes in one place. This enhances code organization and readability.
Real-world Application: Database Record Representation
Structs can effectively represent entities in applications, such as database records. Below is an example of how to accomplish this:
class Database {
public:
struct Record {
int id;
std::string name;
float score;
};
void printRecord(const Record& r) {
std::cout << "ID: " << r.id << ", Name: " << r.name << ", Score: " << r.score << std::endl;
}
};
In this `Database` class, the `Record` struct encapsulates three attributes: `id`, `name`, and `score`. This compact representation makes it easy to handle data records as individual units.
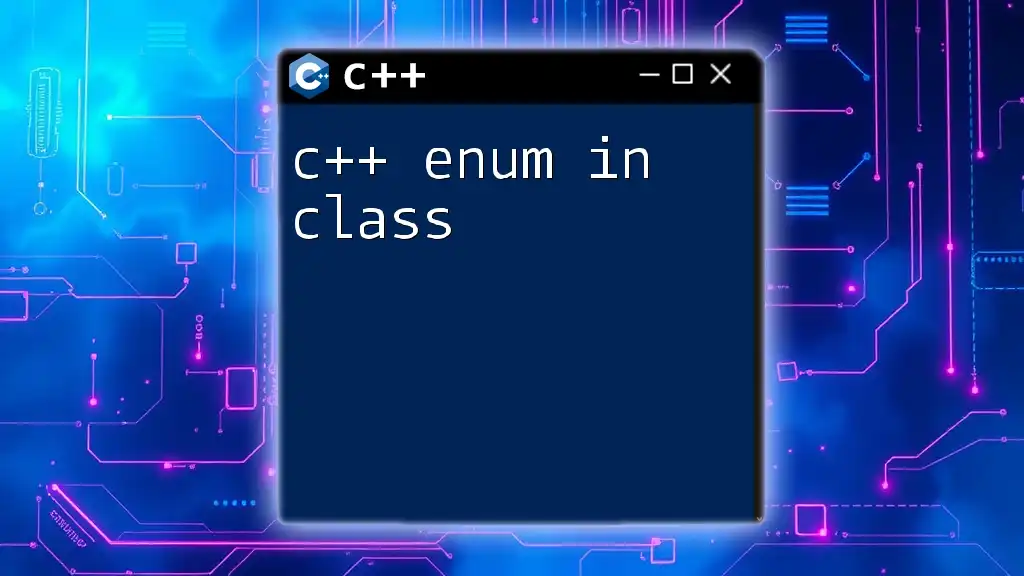
Member Functions with Nested Structs
Defining Member Functions that Operate on Structs
Classes can include member functions that utilize their inner structs, further enhancing their functionality. Here’s an example of defining a member function that operates on a struct:
class Team {
public:
struct Player {
std::string name;
int age;
};
void printPlayerDetails(const Player& player) {
std::cout << "Player Name: " << player.name << ", Age: " << player.age << std::endl;
}
};
In this `Team` class, the `printPlayerDetails()` function accepts a `Player` struct and prints its details, demonstrating the interaction between class methods and struct data.
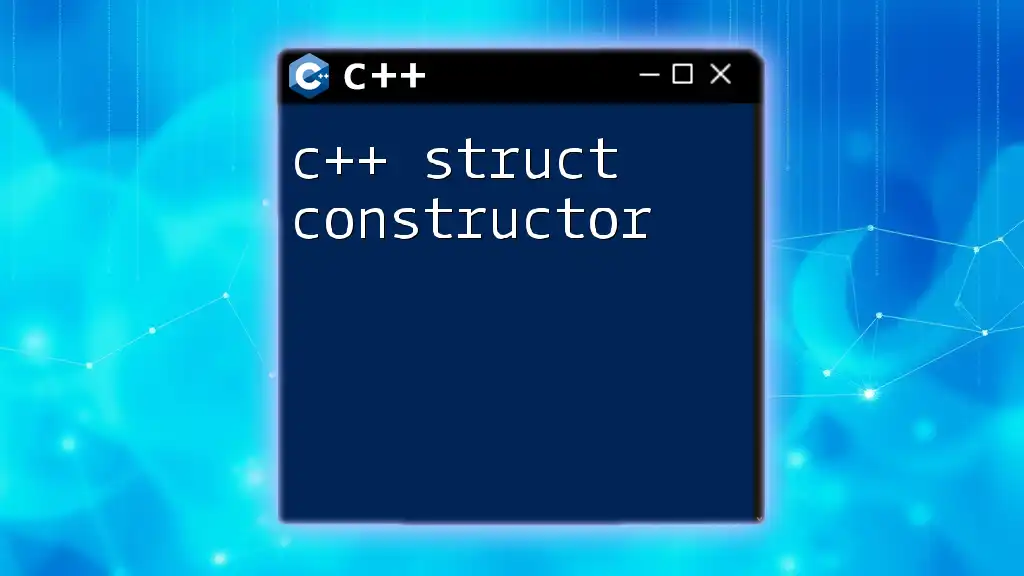
Best Practices
Choosing Between Struct and Class
When deciding between a struct and a class, consider the following guidelines:
- Use structs for simple data structures that predominantly hold public data without much accompanying functionality.
- Opt for classes when you need encapsulation (e.g., private members), inheritance, or more complex behaviors.
Using Access Specifiers Wisely
Even though structs are public by default, it’s essential to consider access specifiers carefully to control the visibility of struct members. For example:
class Example {
public:
struct InnerStruct {
int data; // Public by default
};
private:
struct PrivateStruct {
float hiddenValue; // Private
};
};
In this example, `InnerStruct` is accessible from anywhere, while `PrivateStruct` can only be accessed by the `Example` class, allowing for better data protection.
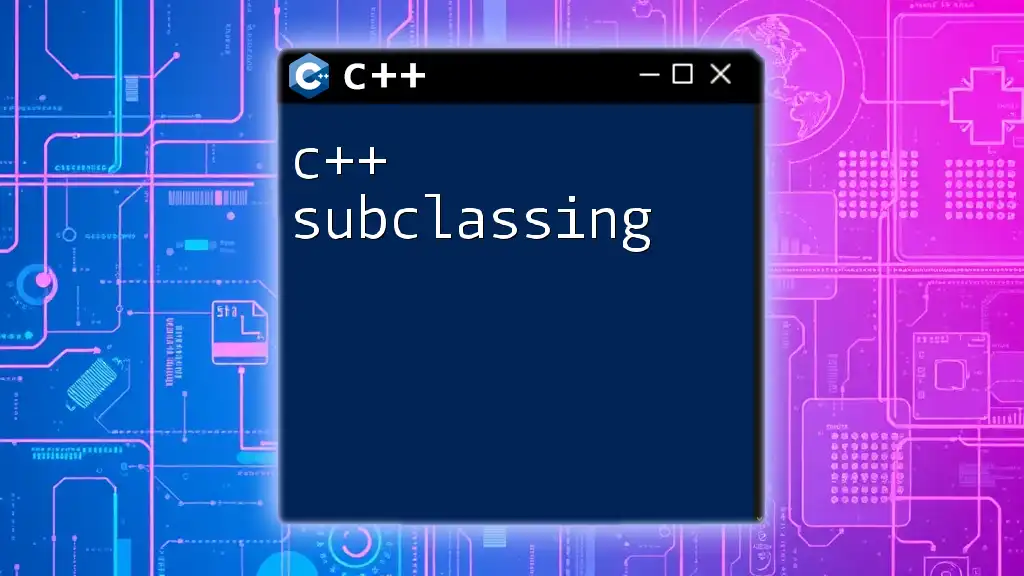
Conclusion
Understanding c++ struct in class is crucial for any serious software developer working with C++. Structs can help keep your data organized while maintaining ease of access and use. By combining the capabilities of structs and classes, you can design robust, clear, and maintainable code.
In your journey to mastering C++, don’t hesitate to experiment with implementing structs in your classes. Their versatility and straightforwardness can greatly enhance the clarity and structure of your code.
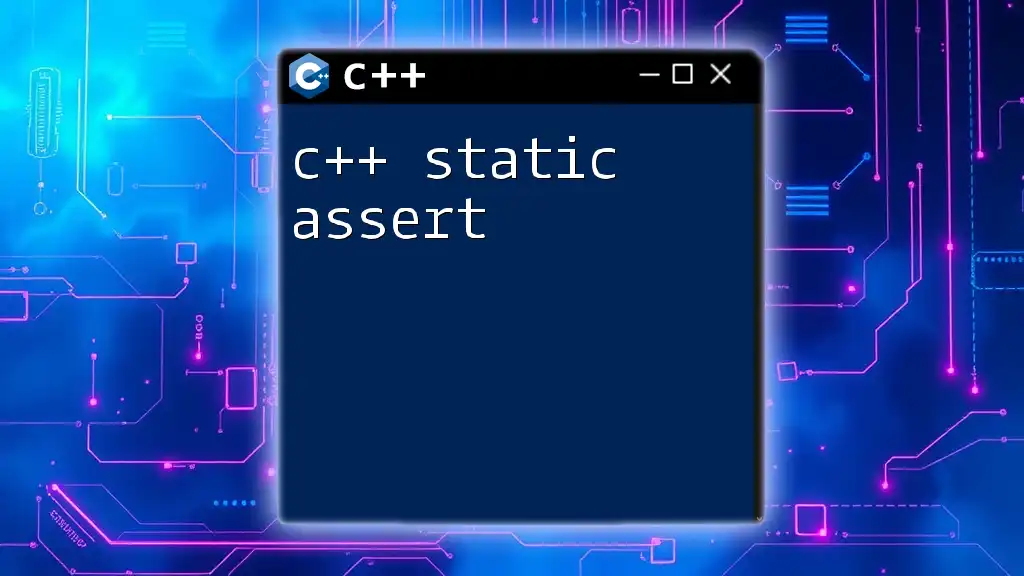
Additional Resources
To further delve into this topic, consider exploring:
- Books on C++ programming that cover data structures in detail.
- Online tutorials and courses that provide hands-on practice.
- Coding platforms that allow you to apply C++ coding techniques interactively.