C++ and Rust are both powerful programming languages that prioritize performance, but C++ focuses on object-oriented programming with manual memory management, while Rust emphasizes safety and concurrency with its ownership model.
Here's a simple comparison of C++ and Rust syntax for defining a variable and printing it to the console:
// C++ example
#include <iostream>
int main() {
int number = 42;
std::cout << "The number is: " << number << std::endl;
return 0;
}
// Rust example
fn main() {
let number = 42;
println!("The number is: {}", number);
}
Overview of C++
What is C++?
C++ is a powerful, high-performance programming language that has been widely adopted in systems programming, game development, and application development since its inception in the early 1980s. It is an extension of the C programming language, introducing features such as classes and object-oriented programming concepts. With its rich history, C++ has evolved into a language favored for its performance characteristics and extensive libraries.
Key Features of C++
- Object-Oriented Programming: C++ supports encapsulation, inheritance, and polymorphism, allowing developers to create modular and reusable code.
- Memory Management: C++ provides direct control over system resources, allowing for manual memory management through pointers and dynamic memory allocation.
- Performance and Speed: It is renowned for close-to-hardware performance, making it an ideal choice for applications requiring high efficiency.
- Large Standard Library: The Standard Template Library (STL) offers a collection of useful classes and functions to facilitate complex operations with less coding overhead.
When to Use C++
C++ excels in scenarios requiring extensive use of system resources or when performance is critical. Common use cases include:
- Game engines (e.g., Unreal Engine)
- Real-time systems (e.g., simulations)
- Operating systems (e.g., Microsoft Windows)
- High-frequency trading platforms
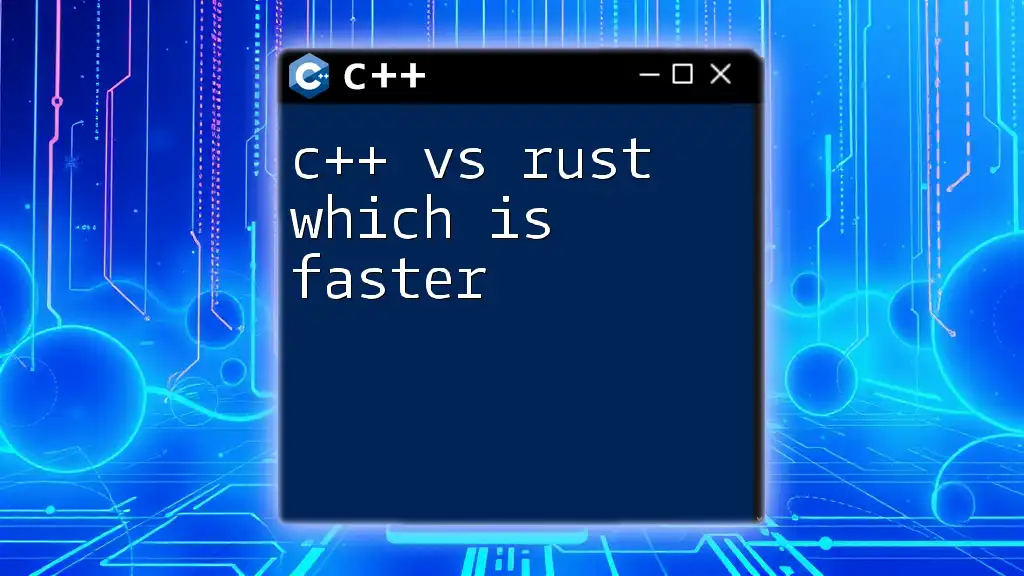
Overview of Rust
What is Rust?
Rust is a systems programming language designed with a keen focus on safety and concurrency. Introduced by Mozilla in 2010, Rust aims to eliminate common programming errors, such as null pointer dereferencing and buffer overflows, through its unique ownership system.
Key Features of Rust
- Memory Safety without Garbage Collection: Rust enforces strict ownership rules at compile time, ensuring that there are no data races and memory-related bugs.
- Concurrency without Data Races: Its ownership model enables fearless concurrency by allowing developers to write concurrent code without the typical pitfalls found in traditional languages.
- Strongly Typed Language: Rust enforces type checking at compile time, which reduces runtime errors and enhances code robustness.
- Performance Efficiency: Comparable to C++, Rust offers high performance combined with features that promote safe programming practices.
When to Use Rust
Rust is well-suited for:
- Web assembly (e.g., compiling code to run in web browsers)
- Embedded systems where memory safety is crucial
- Systems programming where performance and safety are paramount
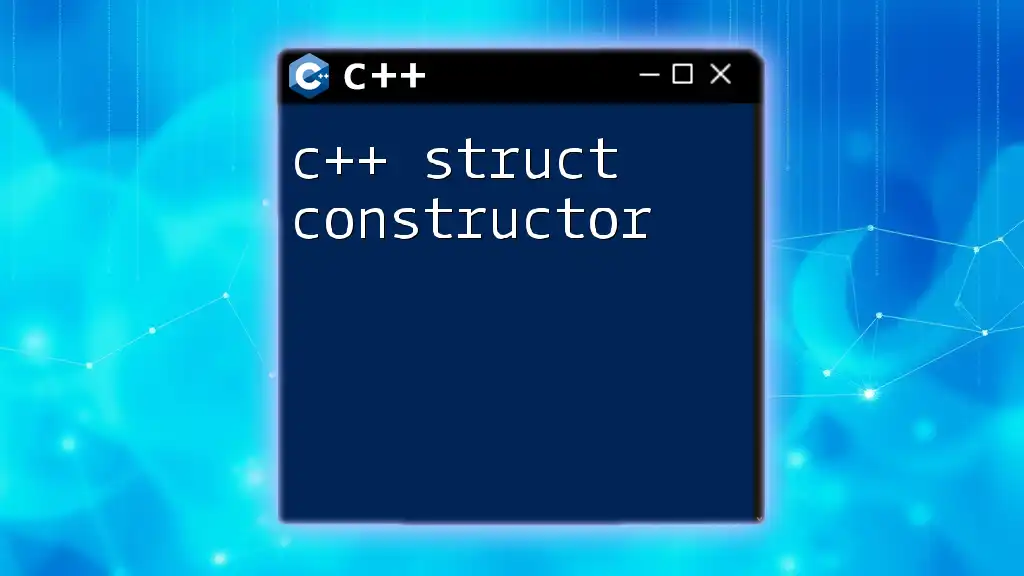
C++ vs Rust: Performance Comparison
Execution Speed
Both C++ and Rust deliver high execution speeds, but nuances exist. C++ is often considered slightly faster due to fewer abstractions and its ability to optimize code at a low level. However, Rust's advanced optimizations make it highly competitive. Benchmark tests typically show that both languages yield similar performance outcomes for CPU-bound tasks.
Memory Usage and Management
C++ gives developers granular control over memory through pointers. However, this can lead to leaks if not managed correctly. A simple example of manual memory management in C++ is:
int* ptr = new int;
*ptr = 42;
// Remember to delete it later
delete ptr;
Rust, on the other hand, uses an ownership model to automatically manage memory, effectively eliminating leaks. The code to create a similar effect in Rust would be:
let x = Box::new(42); // Memory is automatically released when `x` goes out of scope
Compilation Speed
C++ is known for relatively fast compile times, while Rust may have longer compilation times due to its advanced compile-time safety checks and optimizations. This difference can affect the development workflow, particularly in larger projects.
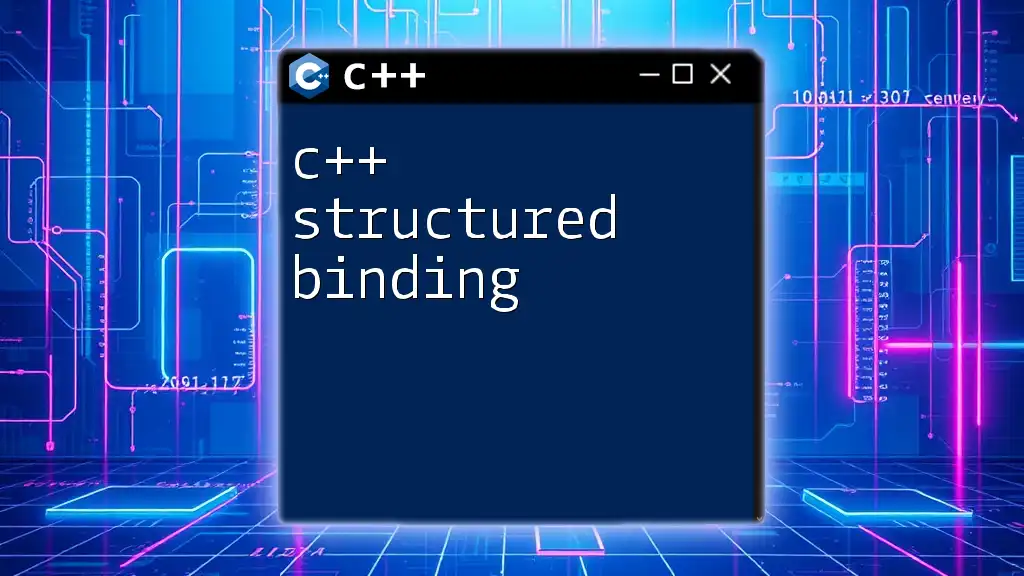
C++ vs Rust: Safety and Concurrency
Memory Safety
In C++, developers must manually manage memory with pointers, which can lead to undefined behavior if mishandled. For instance, accessing a dangling pointer can result in severe bugs. The following code snippet demonstrates this issue:
int* ptr = new int; // Allocate memory
delete ptr; // Free memory
// ptr is now a dangling pointer
std::cout << *ptr; // Undefined behavior
In contrast, Rust's ownership model enforces that no data can be accessed once its owner has been dropped, promoting safer code. When using Rust, such issues are caught at compile time.
let ptr = Box::new(42); // Memory is managed automatically
// No dangling pointer issues
Concurrency
C++ allows straightforward thread creation, but it does not guarantee data race prevention. It requires developers to be vigilant about synchronization. For example:
#include <thread>
#include <iostream>
void increment(int& counter) {
for (int i = 0; i < 1000; ++i) {
counter++; // Potential data race
}
}
int main() {
int counter = 0;
std::thread t1(increment, std::ref(counter));
std::thread t2(increment, std::ref(counter));
t1.join();
t2.join();
std::cout << "Counter: " << counter << std::endl; // Uncertain output
}
Rust’s model ensures safe concurrency by enforcing ownership and borrowing rules, preventing data races at compile-time. For example:
use std::sync::{Arc, Mutex};
use std::thread;
let counter = Arc::new(Mutex::new(0));
let mut handles = vec![];
for _ in 0..2 {
let counter_clone = Arc::clone(&counter);
let handle = thread::spawn(move || {
let mut num = counter_clone.lock().unwrap();
for _ in 0..1000 {
*num += 1; // Safe increments
}
});
handles.push(handle);
}
for handle in handles {
handle.join().unwrap();
}
println!("Counter: {}", *counter.lock().unwrap()); // Guaranteed correct output
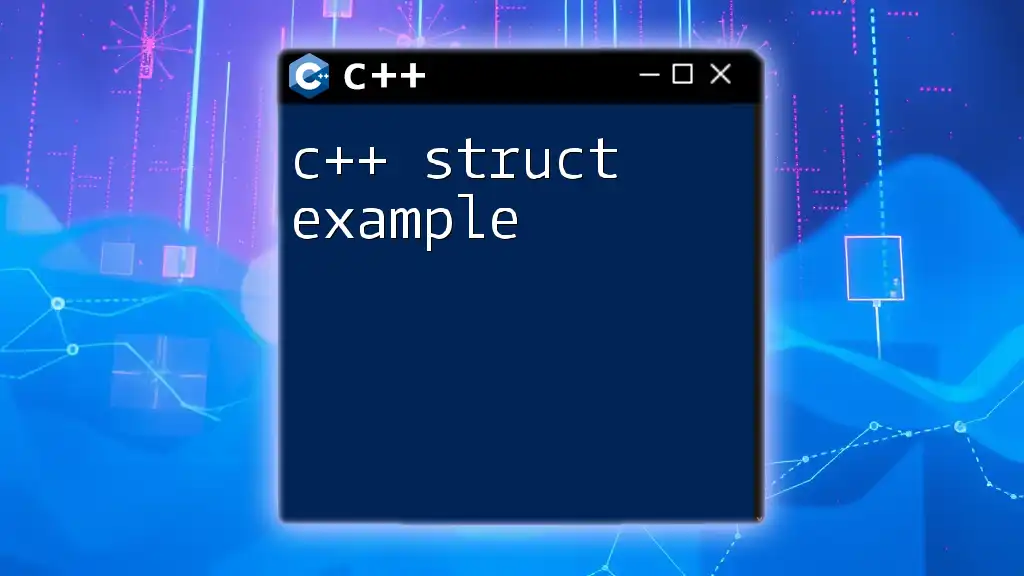
C++ vs Rust: Developer Experience
Ease of Learning
C++ has a steep learning curve due to its complexity, especially with manual memory management and intricate STL features. In contrast, many find Rust easier to grasp once they understand its ownership model, but it can be challenging for those accustomed to traditional C-style languages.
Community and Libraries
Both languages have strong communities and ecosystems. C++ has an older and broader range of libraries. In contrast, Rust’s ecosystem, though younger, is growing rapidly, supported by its excellent package manager, Cargo.
Tooling and IDE Support
C++ development can be enhanced by various IDEs like Visual Studio and CLion, which offer autocomplete, debugging, and static analysis tools. Rust enjoys robust tooling support from IDEs such as IntelliJ Rust and VS Code with Rust Analyzer, which promotes type safety and refactoring.
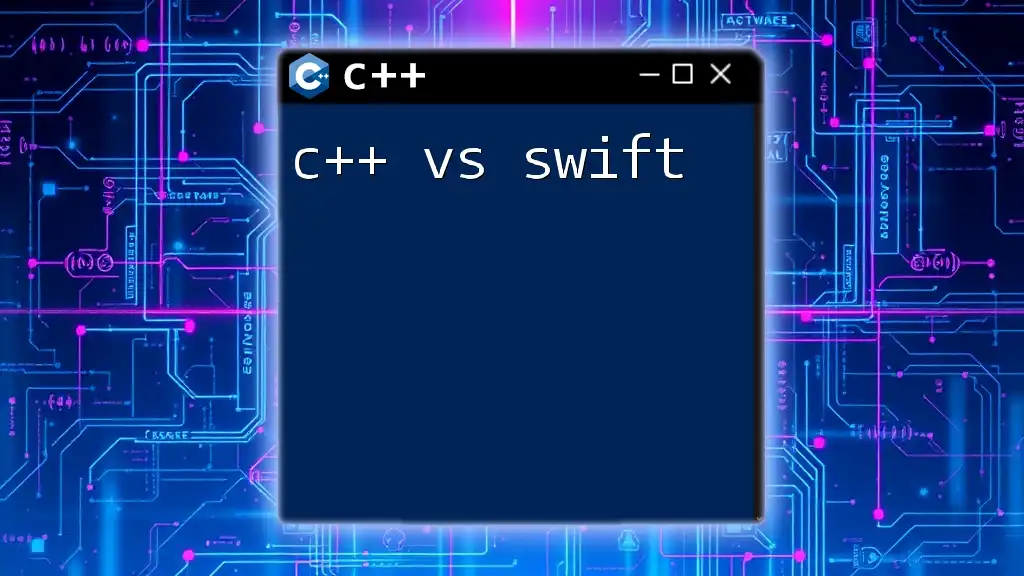
Is Rust Better than C++?
Pros of Using Rust Over C++
- Safer Code: Rust's safety features significantly reduce the potential for common programming errors, making it suitable for high-stakes applications.
- Stronger Concurrency Models: Rust’s ownership model makes it easier to write concurrent code without introducing data races, enhancing developer productivity and overall application safety.
- Simpler Syntax for Some Tasks: For many operations, Rust’s syntax is more straightforward than C++, particularly with pattern matching and iterators.
Cons of Rust Compared to C++
- Steeper Learning Curve: While Rust is generally praised for safety, its unique concepts can be initially challenging for new programmers.
- Compilation Speed: Rust's advanced features can lead to slower compile times, potentially hindering fast iteration in development.
- Newness of the Language in Legacy Systems: Established codebases in C++ are extensive, making it less feasible to switch to Rust for projects that heavily rely on C++ libraries.
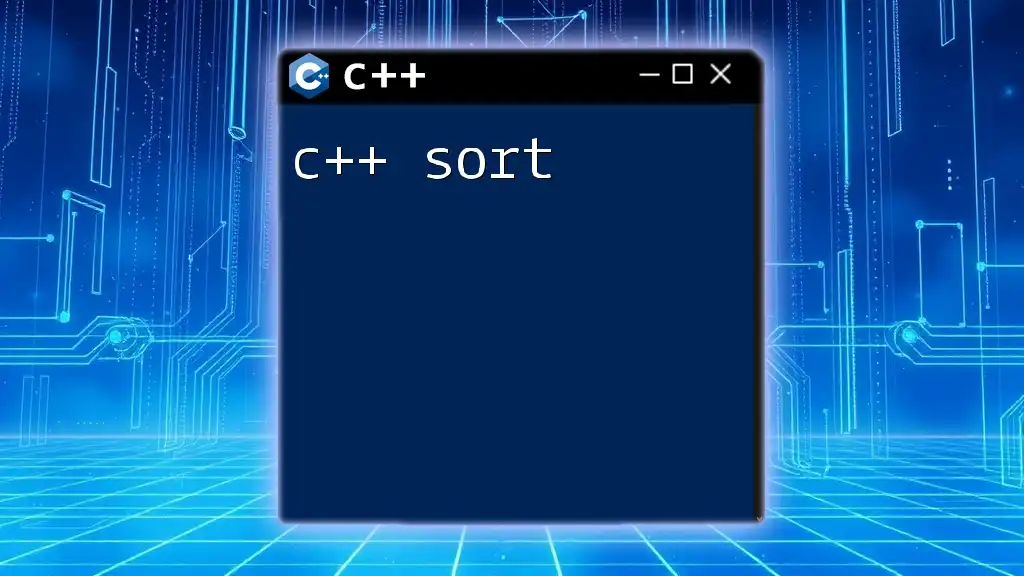
Rust vs C++: Use Cases
Best Use Cases for C++
C++ is irreplaceable in specific domains such as:
- Game Development: C++ dominates major game engines due to its performance and ability to interface with hardware.
- Real-Time Systems: Industries requiring real-time processing, such as telecommunications and automotive systems, often choose C++.
Best Use Cases for Rust
Rust shines in areas where safety and performance are critical, such as:
- System Programming: Rust’s memory safety features significantly reduce bugs in systems-level code.
- Web Assembly: Rust compiles into efficient web assembly, making it suitable for high-performance web applications.
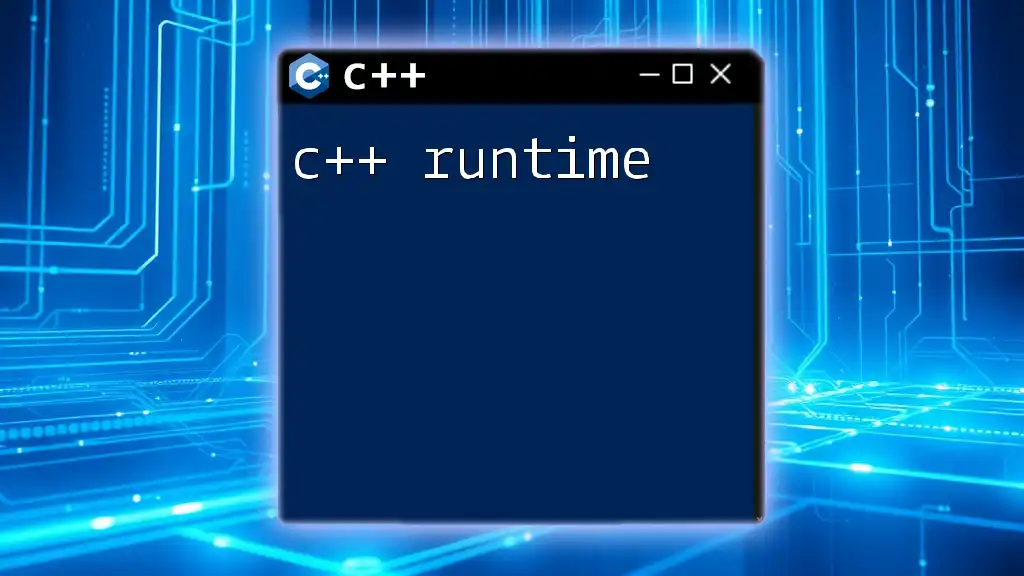
Conclusion
In the ongoing debate of C++ vs Rust, both languages present compelling advantages and disadvantages. The choice between them should depend on specific project needs, team expertise, and long-term project objectives. While C++ continues to be the backbone of many applications, Rust is quickly gaining traction among developers seeking safer, more modern solutions.
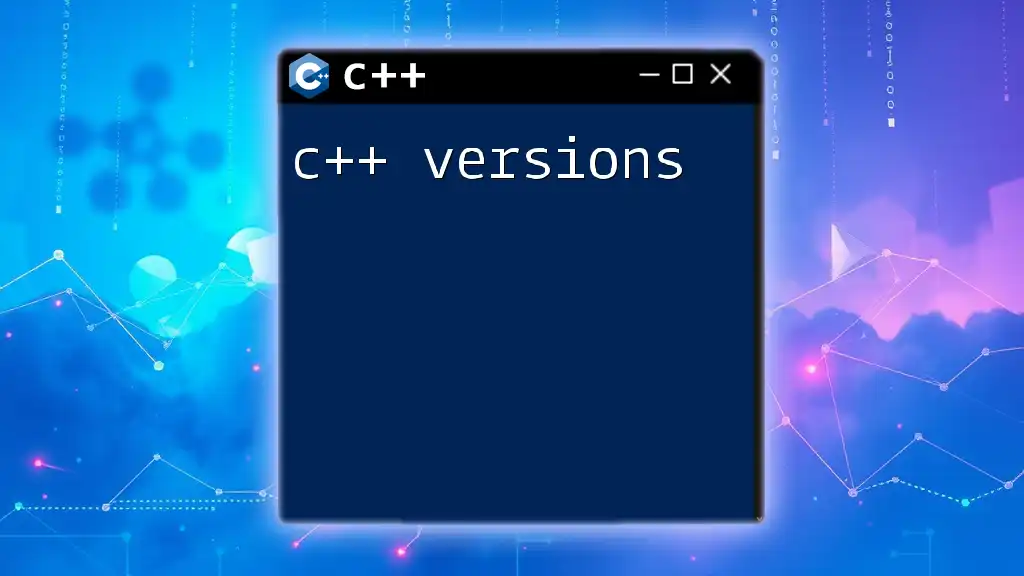
Additional Resources
For those looking to delve deeper, consider exploring online courses, documentation, and community forums surrounding both C++ and Rust to further enhance your knowledge and coding skills.