C++ is a powerful, high-performance programming language commonly used for system-level programming, while Swift is a modern language designed for simplicity and safety in iOS and macOS app development.
Here is a simple code snippet comparing how to define a function in both languages:
C++ Example
#include <iostream>
void greet() {
std::cout << "Hello, C++!" << std::endl;
}
Swift Example
func greet() {
print("Hello, Swift!")
}
Understanding C++
What is C++?
C++ is a high-performance, versatile programming language that was developed in the early 1980s by Bjarne Stroustrup. It is known for its powerful features which allow developers to write efficient and flexible code. One of its hallmark characteristics is its support for both procedural and object-oriented programming paradigms, enabling a wide range of applications from system software to video games.
Key Characteristics of C++
- Compiled Language: C++ is compiled, meaning that code is translated into machine language for better performance. This can lead to faster execution times compared to interpreted languages.
- Object-Oriented Programming (OOP): C++ provides robust support for OOP, making it easier to manage and structure larger codebases through concepts like inheritance, polymorphism, and encapsulation.
- Low-level Memory Manipulation: C++ allows developers to manipulate memory directly through pointers and dynamic allocation, giving them the flexibility to optimize performance and resource usage. For example:
#include <iostream>
int main() {
int* ptr = new int(5);
std::cout << *ptr; // Output: 5
delete ptr;
return 0;
}
This example shows how C++ allows direct access to memory to allocate and deallocate variables, a responsibility that gives developers both power and complexity.
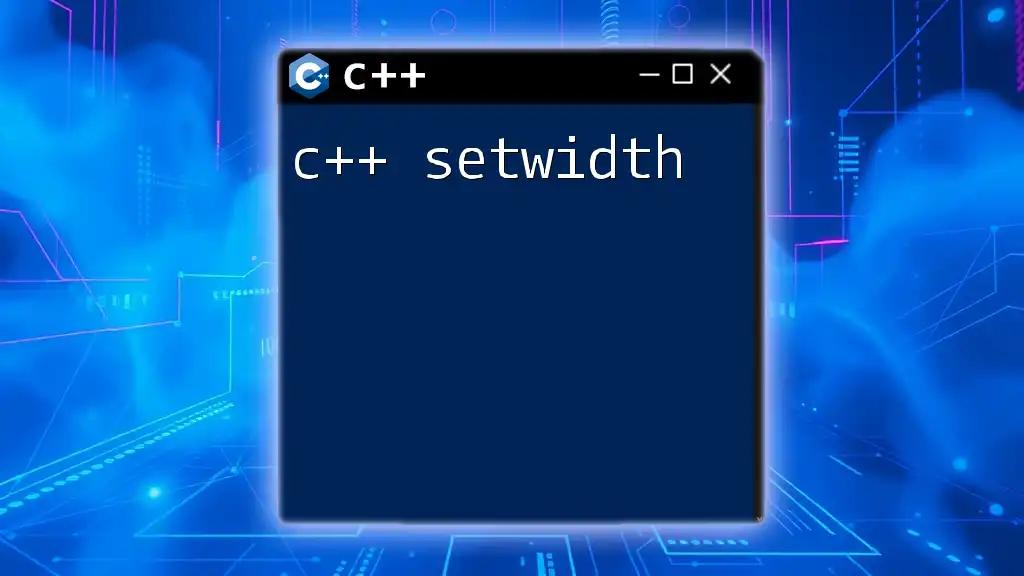
Understanding Swift
What is Swift?
Introduced by Apple in 2014, Swift is a modern programming language specifically designed for iOS and macOS development. It aims to provide a more intuitive and safer coding experience than its predecessor, Objective-C, while maintaining performance. Swift simplifies many of the challenges that programmers face, making it easier to write reliable code quickly.
Key Characteristics of Swift
- Interpreted Language: Unlike C++, Swift is mainly compiled and can also be interpreted, allowing faster feedback when coding due to features like playgrounds where developers can interactively run Swift code.
- Safety and Performance: Swift incorporates safety features like optionals and automatic memory management, reducing the chances of common programming errors such as null pointer dereferencing. This leads to more robust applications.
- Modern Syntax: Swift’s syntax is clean and easy to read, emphasizing coding efficiency. For instance:
let greeting = "Hello, World!"
print(greeting) // Output: Hello, World!
Such a sleek syntax not only makes Swift code approachable but also encourages best practices in programming.
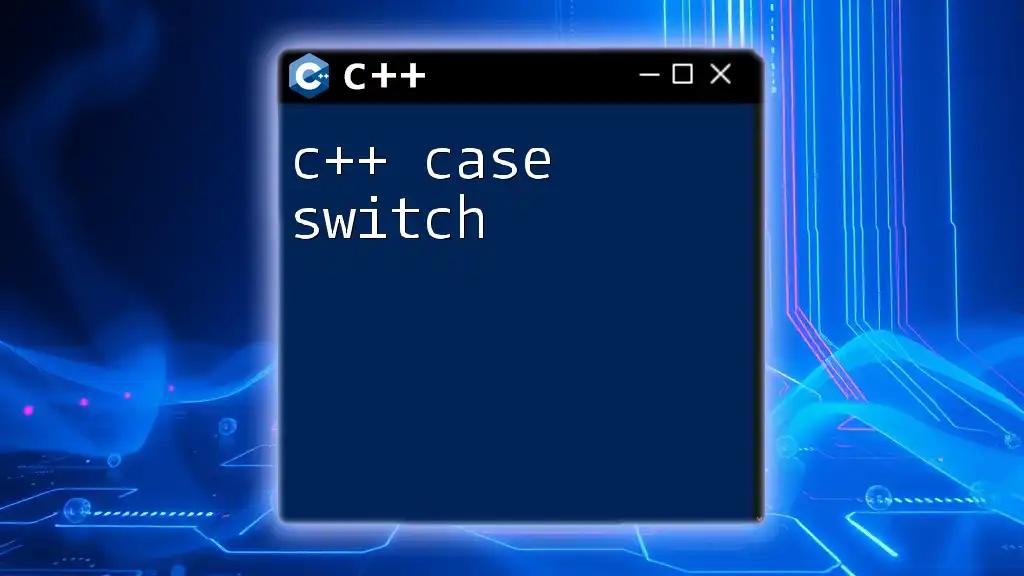
C++ vs Swift: A Detailed Comparison
Syntax Comparison
One of the most striking differences between C++ vs Swift lies in their syntax. C++ syntax can be verbose, particularly when dealing with loops and conditionals, while Swift offers a more concise and readable approach.
For instance, consider how a simple for-loop is defined:
// C++ Example
for(int i = 0; i < 5; i++) {
std::cout << i << " ";
}
// Swift Example
for i in 0..<5 {
print(i, terminator: " ")
}
The Swift code demonstrates the use of more natural language-like constructs, which can make for easier understanding, especially for beginners.
Performance Comparison
Performance is a crucial aspect when evaluating C++ vs Swift. Historically, C++ has been preferred for performance-critical applications due to its ability to compile code directly to machine language. Comparisons have shown that well-optimized C++ code executes operations significantly faster than interpreted or even compiled Swift code.
However, Swift has made substantial gains in performance, especially when optimized for Apple’s hardware, and can be quite competitive in specific scenarios, particularly in app development where rapid iterations are necessary.
Error Handling
Error handling is another key area where the two languages differ significantly. C++ employs exception handling that requires a well-defined structure for try-catch blocks. Here’s an example:
// C++ Example
try {
// code that may throw an exception
} catch (std::exception& e) {
std::cout << e.what();
}
On the other hand, Swift utilizes a more modern approach with try-catch and Rust-like optionals, which can help prevent runtime errors. This feature emphasizes safety, allowing developers to handle potential errors gracefully and succinctly:
// Swift Example
do {
try riskyFunction()
} catch {
print("Error: \(error)")
}
Community and Library Support
In the realm of support, both languages boast robust communities, but they focus on different domains. C++ has a longer history and, therefore, a larger ecosystem of libraries and frameworks for various applications such as game engines and operating systems. Existing libraries like Boost and STL are invaluable resources for C++ developers.
Meanwhile, Swift’s community is dynamic, driven primarily by its focus on mobile application development. Although its ecosystem is smaller than C++, Swift's package manager has been growing rapidly, making a wide variety of third-party libraries easily accessible.

Use Cases: When to Choose C++ vs Swift
Ideal Scenarios for Using C++
C++ is well-suited to scenarios where performance is critical, such as:
- Systems Programming: Operating systems and drivers that require efficient resource management.
- Game Development: Engaging in high-performance graphics and game engines where speed and memory control are paramount.
- High-Performance Applications: Such as scientific computing, where the utmost efficiency is crucial.
Ideal Scenarios for Using Swift
On the other hand, Swift shines in:
- iOS App Development: Its syntax and libraries are tailored specifically for Apple’s frameworks, making it the go-to language for mobile developers.
- Server-Side Development: Swift is increasingly being adopted for server-side applications, offering a modern option for backend programming.
- Rapid Prototyping: The ease of writing Swift allows for quick iterations and testing of ideas, making it a favorite among startups and developers looking to innovate quickly.
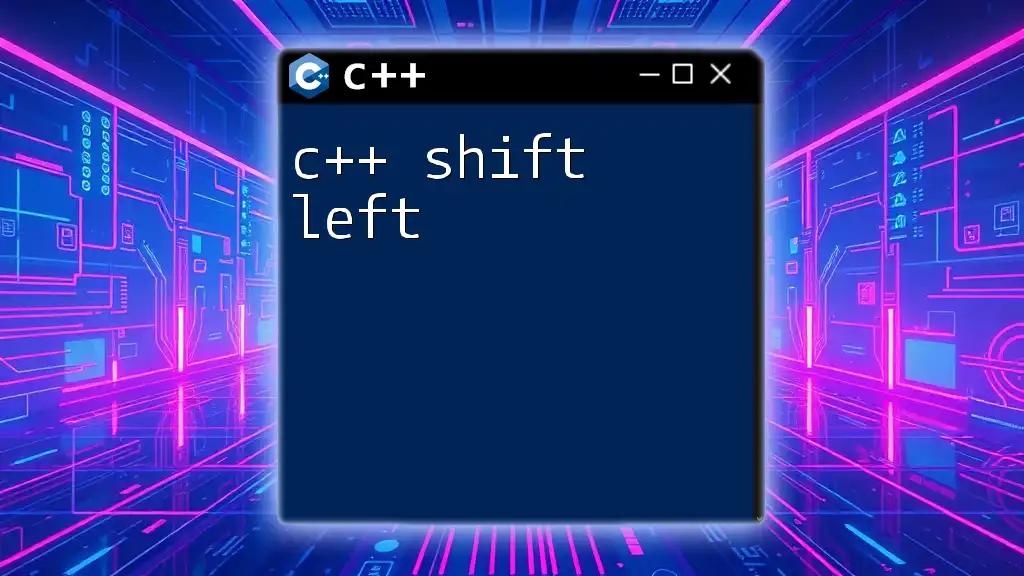
Conclusion
In summary, when comparing C++ vs Swift, it’s important to weigh the strengths and characteristics of each language against your project requirements. C++ stands out in performance and flexibility, while Swift excels in safety, readability, and rapid development for Apple ecosystems. Understanding these distinctions not only helps in choosing the right language for your projects but also enhances your overall programming skills.
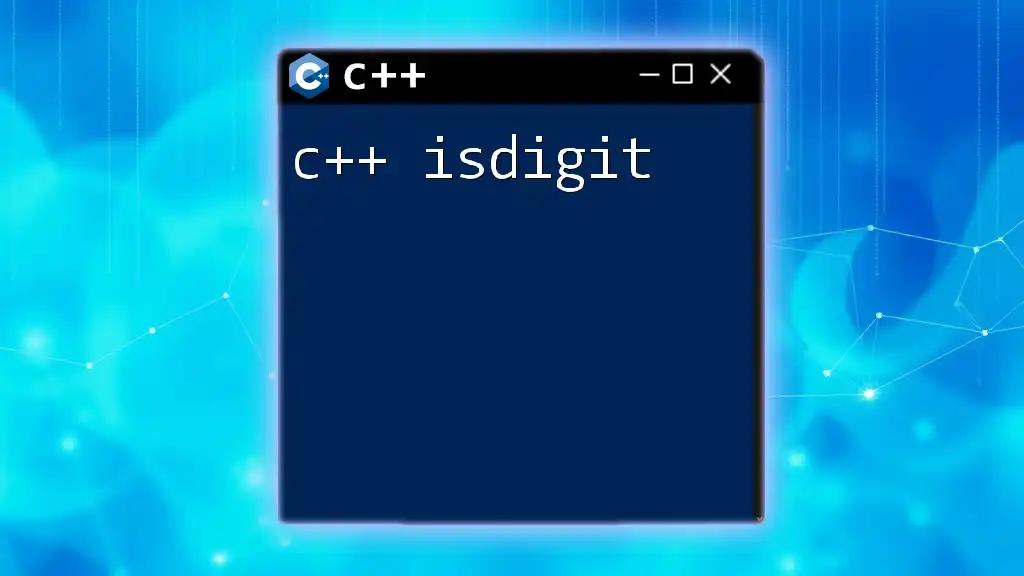
Call to Action
Embark on the journey to mastering both C++ and Swift through our comprehensive guides and resources. Share your experiences with these two powerful languages in the comments below, and let’s learn together!
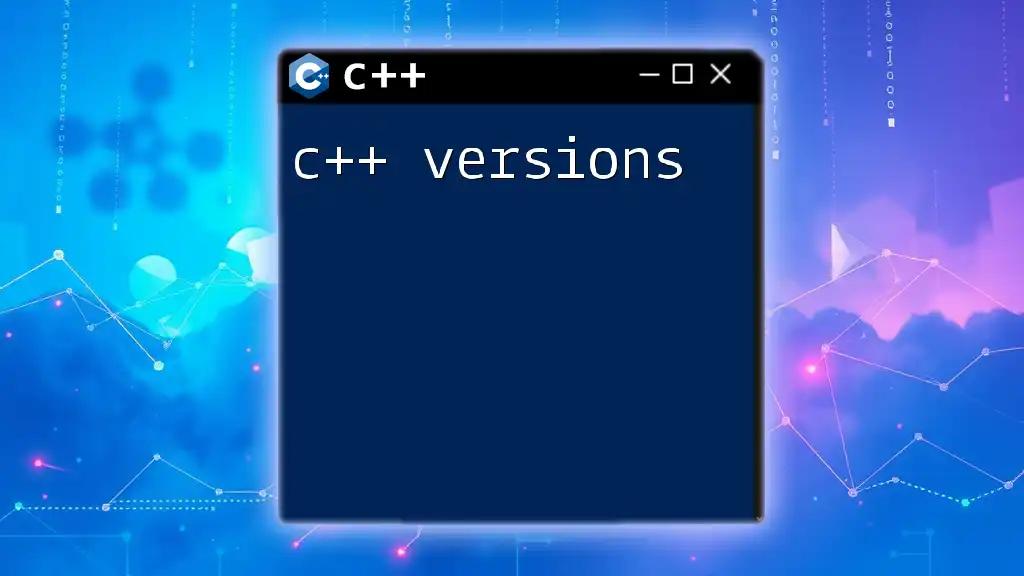
Additional Resources
- [C++ Tutorials](#)
- [Swift Tutorials](#)
- [Recommended Books and Online Courses](#)