When comparing C++ and Rust in terms of speed, both languages are designed for performance optimization, but C++ often has a slight edge due to its mature compiler optimizations and extensive libraries; however, Rust's emphasis on safety can lead to faster development cycles and fewer runtime errors.
Here's a simple performance comparison code snippet that demonstrates the speed of both languages in a basic loop:
// C++ code snippet
#include <iostream>
#include <chrono>
int main() {
auto start = std::chrono::high_resolution_clock::now();
int sum = 0;
for (int i = 0; i < 1000000; ++i) {
sum += i;
}
auto end = std::chrono::high_resolution_clock::now();
std::cout << "Sum: " << sum << " Time: " << std::chrono::duration_cast<std::chrono::milliseconds>(end - start).count() << " ms" << std::endl;
return 0;
}
This C++ code snippet measures the time taken to sum numbers from 0 to 999,999, which can be used to benchmark performance against an equivalent Rust implementation.
Understanding Performance in Programming Languages
What Makes a Language Fast?
When it comes to programming languages, various factors contribute to performance.
-
Compiled vs Interpreted Languages: Compiled languages like C++ and Rust translate code to machine language during the compilation process, enabling maximum execution speed. In contrast, interpreted languages perform translation at runtime, which can introduce latency.
-
Memory Management and System Resources: The way a language handles memory—whether through garbage collection, manual allocation, or smart pointers—affects its speed and efficiency significantly.
-
Effects of Language Features on Performance: High-level abstractions in a language can sometimes lead to performance overhead if not optimally compiled.
Benchmarks and Their Importance
Performance is often quantified through benchmarks. These benchmarks provide numerical data that help compare how different language implementations handle the same problem. Common benchmarking tools for C++ and Rust include:
- Google Benchmark for C++
- Criterion for Rust
Using these tools, developers can gain insights into language performance across various scenarios.
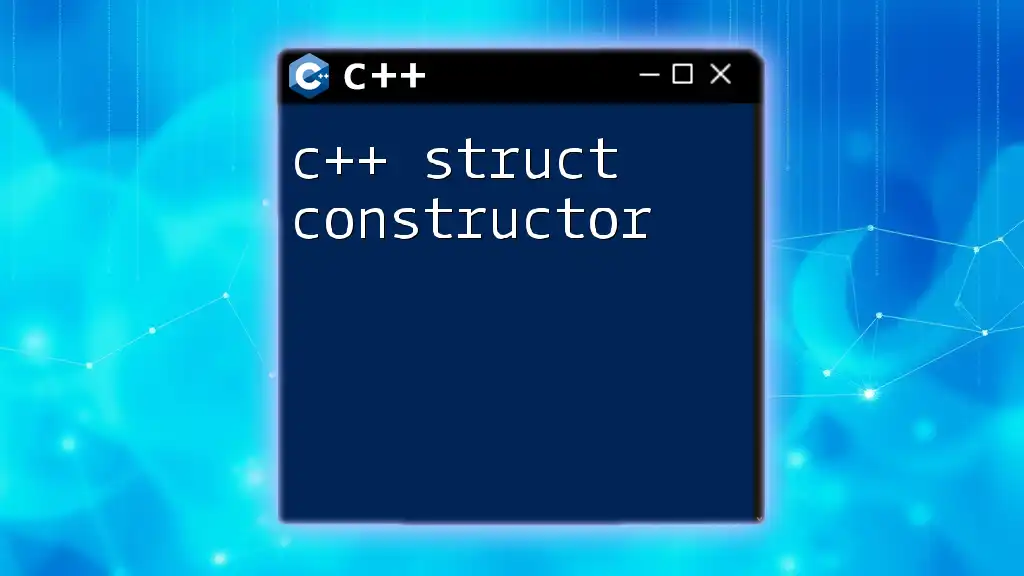
C++: A Closer Look
History and Development
C++ was developed in the early 1980s as an extension of the C programming language. It introduced object-oriented programming features to enhance the capabilities of C, while retaining its power and flexibility.
Performance Characteristics of C++
-
Memory Management: C++ employs manual memory management, which provides developers with fine-grained control over how memory is allocated and deallocated. This can lead to high performance if managed correctly, but it also increases the risk of memory leaks.
-
Speed: C++ is known for its native execution speed, benefiting from compiler optimizations that can eliminate unnecessary operation overhead.
Sample Code in C++
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers(1000000);
for (int i = 0; i < 1000000; ++i) {
numbers[i] = i * i;
}
std::cout << "Computation complete!" << std::endl;
return 0;
}
In this C++ example, we initialize a vector of one million integers and populate it with their squared values. The simplicity of this code allows the compiler to optimize it effectively, resulting in rapid execution. Such performance benefits are crucial in high-demand environments like gaming or real-time systems.
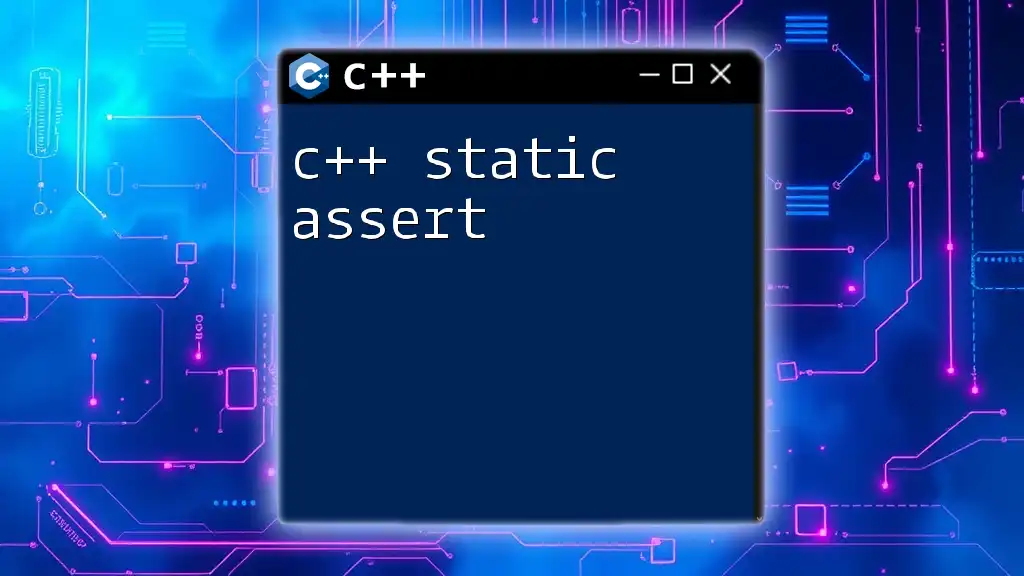
Rust: A Comprehensive Overview
Rust’s Origins and Philosophy
Rust emerged from a desire to create a language that emphasizes memory safety without sacrificing performance. Its development started in 2006 at Mozilla Research, with the guiding principles being safety, concurrency, and performance.
Performance Characteristics of Rust
-
Safety and Concurrency: Rust's ownership model ensures memory safety. The compiler checks for possible issues during compile time, which can prevent runtime errors that cause crashes or memory leaks.
-
Zero-cost Abstractions: Rust provides higher-level abstractions while avoiding performance overhead, a concept often referred to as "zero-cost abstractions." This means that the use of these abstractions does not come at the cost of runtime performance, making it a powerful tool for performance-critical applications.
Hands-on Code Example in Rust
fn main() {
let numbers: Vec<i32> = (0..1_000_000).map(|i| i * i).collect();
println!("Computation complete!");
}
In this Rust code snippet, we create a vector populated with the squares of integers from zero to one million. The code reflects Rust's ability to handle complex operations while ensuring safety and performance through its compiler checks, allowing programmers to focus on logic without much fear of errors related to memory.
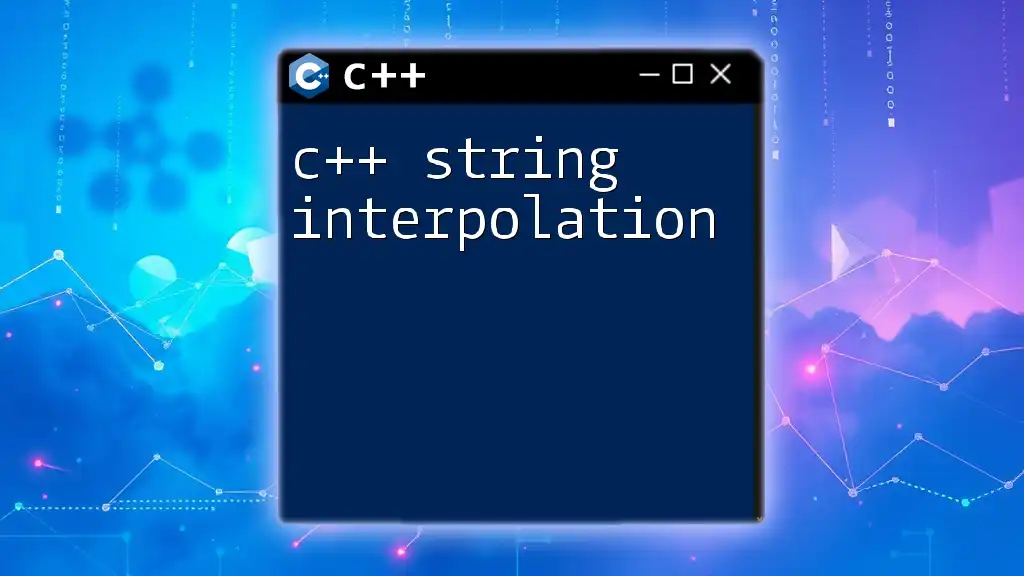
Performance Comparison: C++ vs Rust
Direct Comparisons of Execution Speed
When comparing execution speed between C++ and Rust, benchmarks consistently show that both languages can achieve comparable performance due to their compiled nature. However, the specific implementation details and the optimizations done by compilers can tip the balance in favor of one language over the other in specific scenarios.
Memory Usage and Efficiency
Memory management significantly influences performance. C++ allows developers full control over memory but at the cost of increased complexity and potential mistakes.
In contrast, Rust’s ownership model simplifies memory management while still providing efficient usage patterns, especially in concurrent applications where data races could have severe implications.
Compiling and Optimization
Both C++ and Rust have powerful compilers: GCC and Clang for C++, whereas Rust uses `rustc`. The optimization strategies vary slightly, with C++ often focusing heavily on manual optimizations that the developer must understand deeply, while Rust pushes the compiler to enforce safety constraints that can sometimes lead to optimizations done automatically.
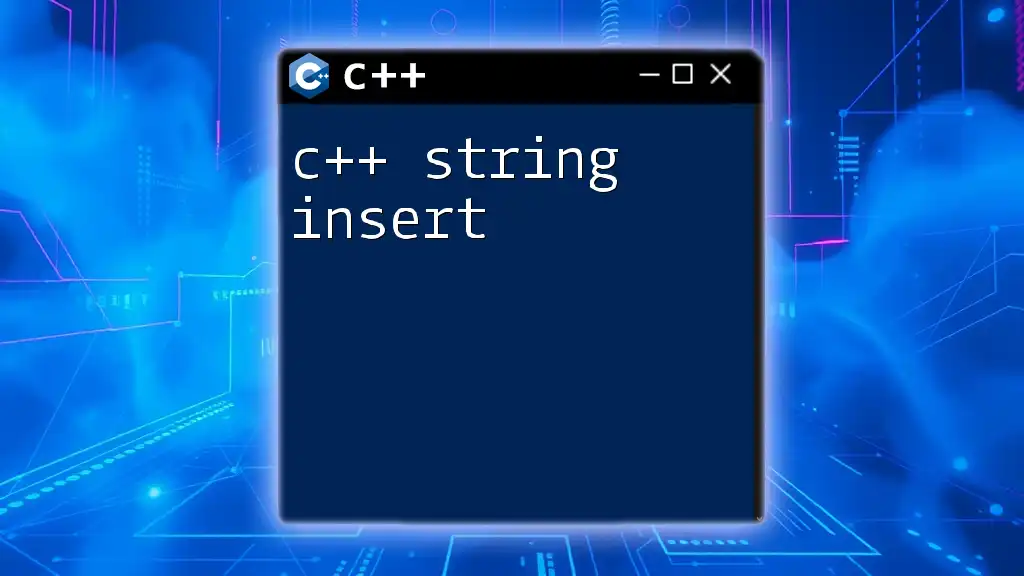
Use Cases: Where Performance Matters
Suitable Scenarios for C++
C++ shines in contexts where performance is paramount. Examples include:
- Gaming Engines: Due to its real-time execution capabilities and fine control over resources.
- Systems Programming: Such as operating systems or embedded systems that require maximum efficiency and minimal overhead.
Scenarios Where Rust Excels
Rust is particularly advantageous in areas like:
- WebAssembly: Allowing safe and efficient code to run in web environments.
- Concurrent Applications: Its design mitigates problems associated with data races, making it suitable for multithreading tasks.
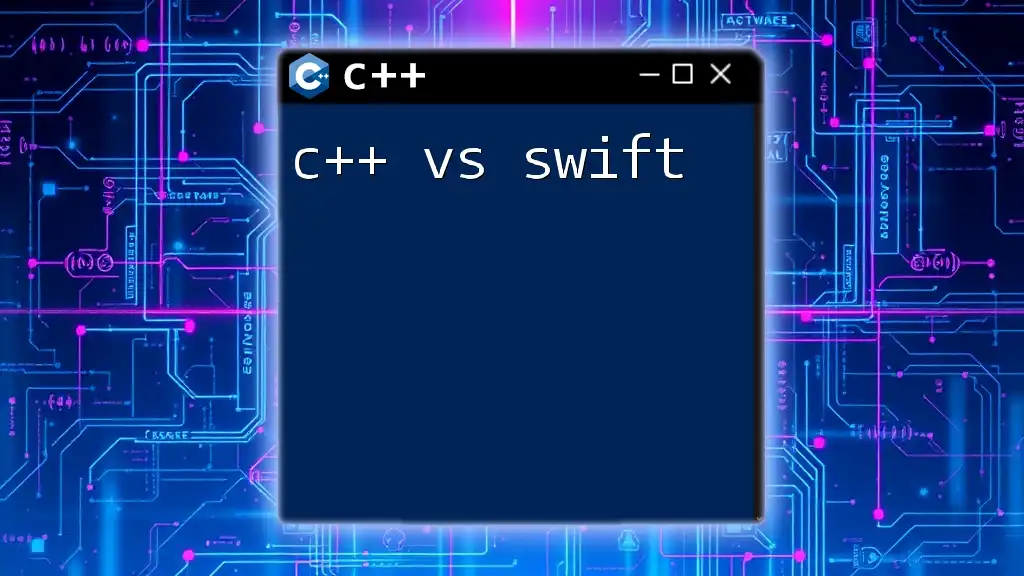
Conclusion
Final Thoughts on C++ vs Rust
Both C++ and Rust are powerful languages, each with unique benefits and performance capabilities. While C++ offers speed through manual control, Rust provides modern memory safety features that can lead to better long-term maintenance and fewer runtime errors.
Is Rust Faster Than C++?
The question of whether Rust is faster than C++ depends heavily on the context of the use case. Properly optimized C++ could outperform Rust in raw execution speed, but Rust's safety features and developer productivity can bring advantages that extend beyond just numbers.
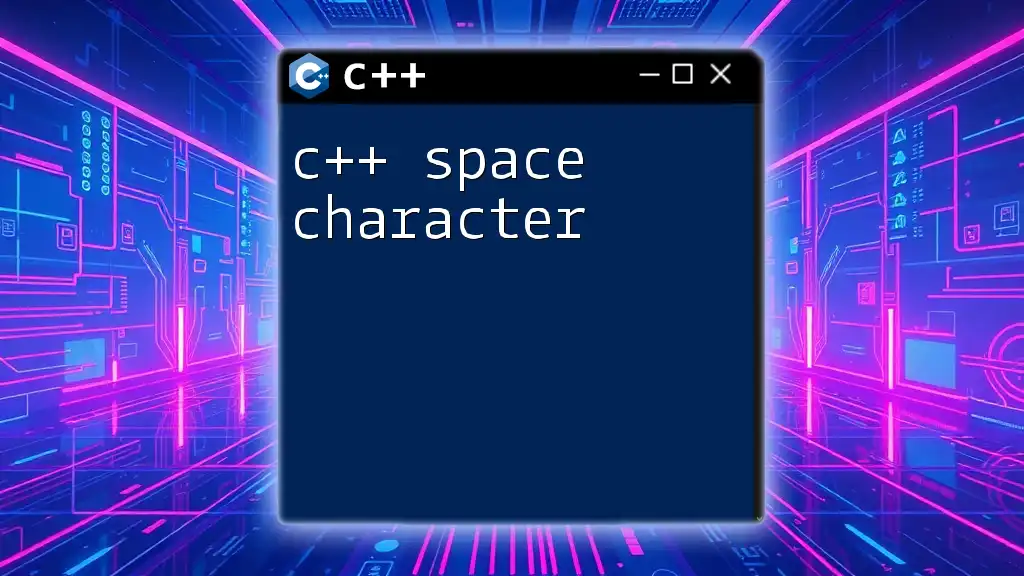
Further Reading and Resources
For those eager to deepen their understanding of C++ and Rust, consider exploring books, online courses, and resources dedicated to both languages. Engaging with community benchmarks and tools will also enhance your comprehension of each language's performance.
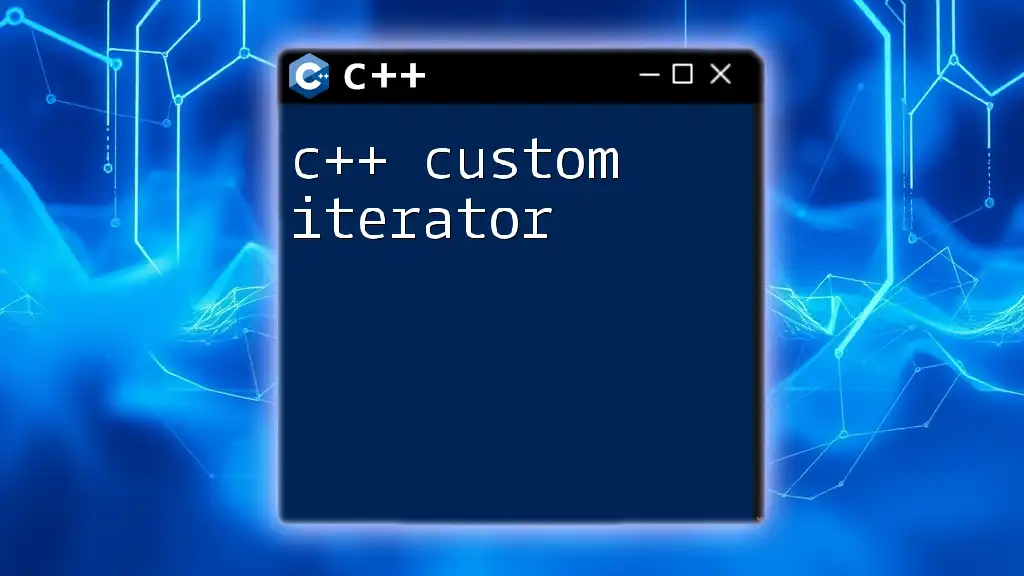
Call to Action
We encourage you to share your experiences with both C++ and Rust. Join our courses to learn more about how you can effectively utilize these languages in your projects, compare performance, and optimize your coding skills for maximum efficiency.