In C++, a null character (`'\0'`) is used to signify the end of a string, allowing functions to determine the length of the string data accurately.
Here's a code snippet demonstrating its use:
#include <iostream>
int main() {
char str[] = "Hello, world!";
std::cout << str << std::endl; // Output: Hello, world!
str[5] = '\0'; // Truncate the string at the null character
std::cout << str << std::endl; // Output: Hello
return 0;
}
Understanding the C++ Null Character
What is a Null Character?
The C++ null char is a special character represented by `'\0'` that signifies the end of a string or character array in memory. In C++ programming, strings are often represented as arrays of characters, and the null character serves as a critical sentinel that denotes where the string ends. This concept originates from C programming, where null-terminated strings are standard.
Representation of the Null Character
The null character is part of the ASCII character set, specifically represented by the value 0. This allows the program to distinguish between the actual content of the string and the point where it terminates. For example, when you write:
char message[] = "Hello";
The underlying representation in memory includes the characters 'H', 'e', 'l', 'l', 'o', followed by a null character:
H e l l o \0
Here, the null char signifies that the string consists of five characters.
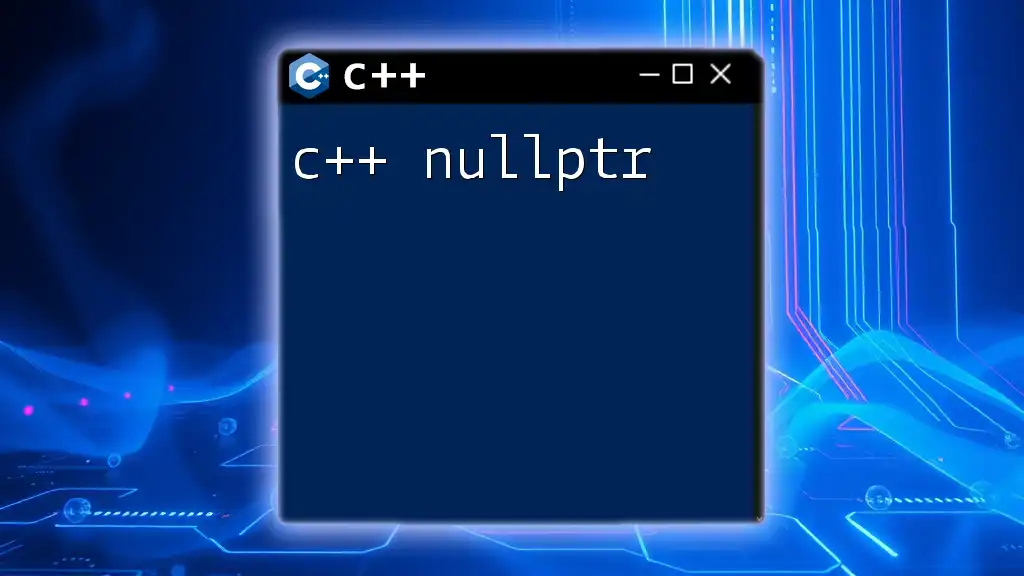
Usage of Null Character in C++
Initializing Strings
When initializing strings, the null char plays a vital role in ensuring that the string is properly formed and terminates correctly. When you explicitly declare an array with a string, the C++ compiler automatically appends a null character.
For instance:
char greeting[10] = "Hi";
This would store 'H', 'i', and then an implicit `'\0'`, resulting in:
H i \0
This way, C++ knows that the string "Hi" ends after the 'i'.
Terminating C-Style Strings
The null character is integral to string length calculations. Functions that handle strings often rely on the null character to determine where the string ends.
For example:
char name[] = "Alice";
std::cout << "Length: " << strlen(name); // Outputs: 5
In this code, the `strlen` function counts the characters in "Alice" before reaching the null character.
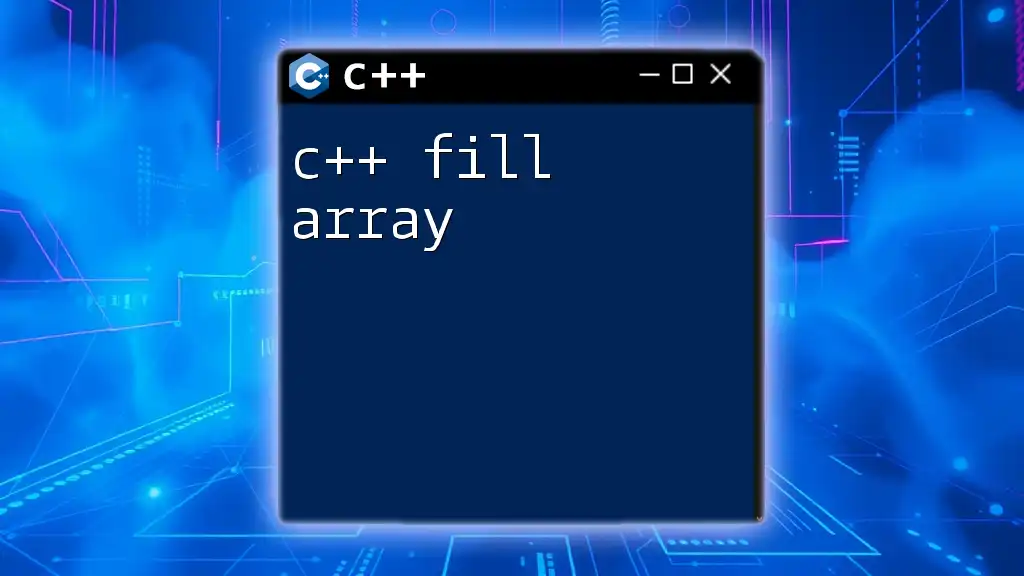
Common Scenarios Involving Null Characters in C++
The Role of Null Characters in Functions
Many C and C++ library functions operate based on null-terminated strings. Functions like `strlen()`, `strcpy()`, and `strcat()` utilize null characters to know where to stop processing.
For example, consider the use of `strcpy()`:
char source[] = "Hello";
char destination[10];
strcpy(destination, source);
std::cout << destination; // Outputs: Hello
In this instance, `strcpy()` copies the characters from `source` to `destination` until it reaches the null character.
Handling Null Characters in Input and Output
When dealing with input and output operations, the null char affects how data is read and printed. For example, when reading a string from the standard input, the input function stops reading as soon as it encounters a null character.
Here’s an example:
char buffer[20];
std::cin >> buffer; // Stops reading at null character
std::cout << buffer; // Will output whatever string was entered
If the input contains a null character, the reading process ends there.
Null Characters in Dynamic Memory Allocation
When dynamically allocating memory, it’s crucial to remember the null character. If you create a character array using `new`, ensure that you allocate enough space for the null terminator.
For instance:
char* dynamicStr = new char[10];
strcpy(dynamicStr, "Hello");
// Ensure to delete to prevent memory leak
delete[] dynamicStr;
In this example, remember to consider space for the null character when performing string operations.
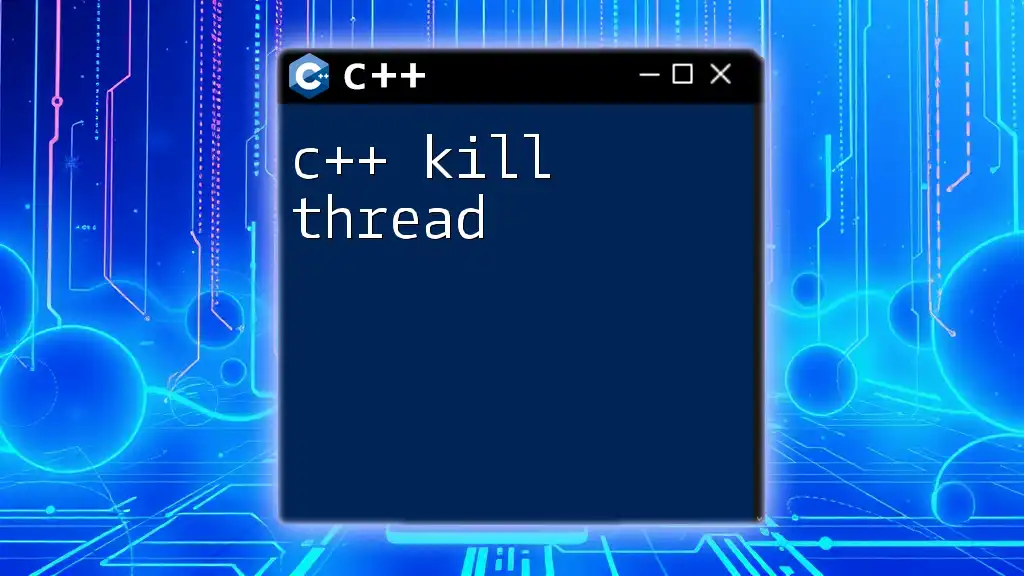
Potential Pitfalls and Errors
Common Mistakes with Null Characters
A frequently encountered error involves neglecting to append a null character at the end of a string. This can lead to undefined behavior if you attempt to treat the extended character array as a string. For instance:
char str[5] = "Hello"; // This causes undefined behavior
Since "Hello" needs 6 spaces (5 for characters plus 1 for the null character), this will corrupt the array.
Debugging Null Character Issues
Debugging can become challenging when null character-related problems arise. Consider using debugging tools, such as Valgrind, which can help track memory misuse and identify where the null characters are mismanaged.
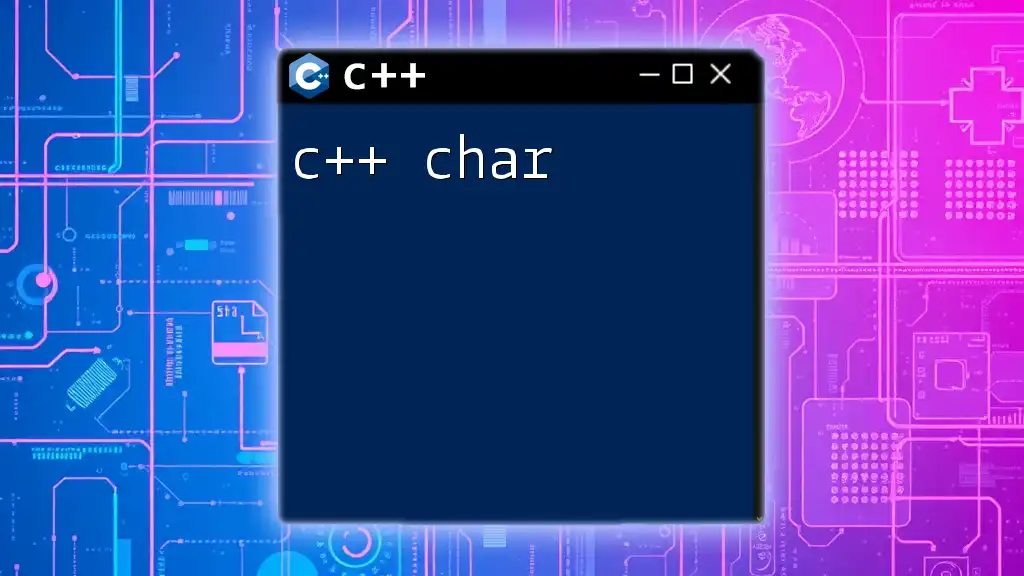
Best Practices for Working with Null Characters
Tips for Safe String Manipulation
Ensure proper initialization and management of your null characters during string operations to help avoid memory issues. Always allocate extra space when dynamically creating strings. A best practice is to use `strncpy()` instead of `strcpy()` to specify the maximum size and avoid buffer overflow.
When to Use C-Style Strings vs. std::string
The choice between using C-style strings and `std::string` often depends on the use case. While C-style strings offer flexibility and control, they are more error-prone. For most modern C++ programs, using `std::string` is recommended due to its ease of use and built-in management of memory and null characters.
For example:
std::string message = "Hello, World!";
std::cout << message; // Automatically manages null character
This demonstrates how `std::string` abstracts the complexities of null characters, allowing programmers to focus on functionality rather than memory management.
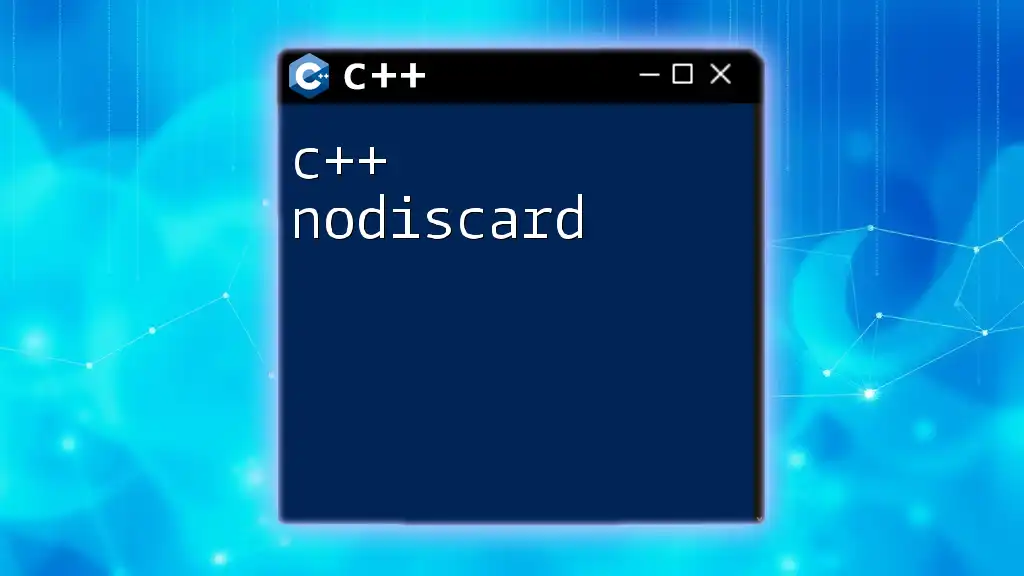
Conclusion
In summary, the C++ null char is a fundamental aspect that plays a critical role in string handling and memory management. By understanding the significance of the null character, developers can better manipulate strings and avoid common pitfalls in their C++ programming. Continuous practice and application of this knowledge will enhance your programming skills and proficiency in C++.
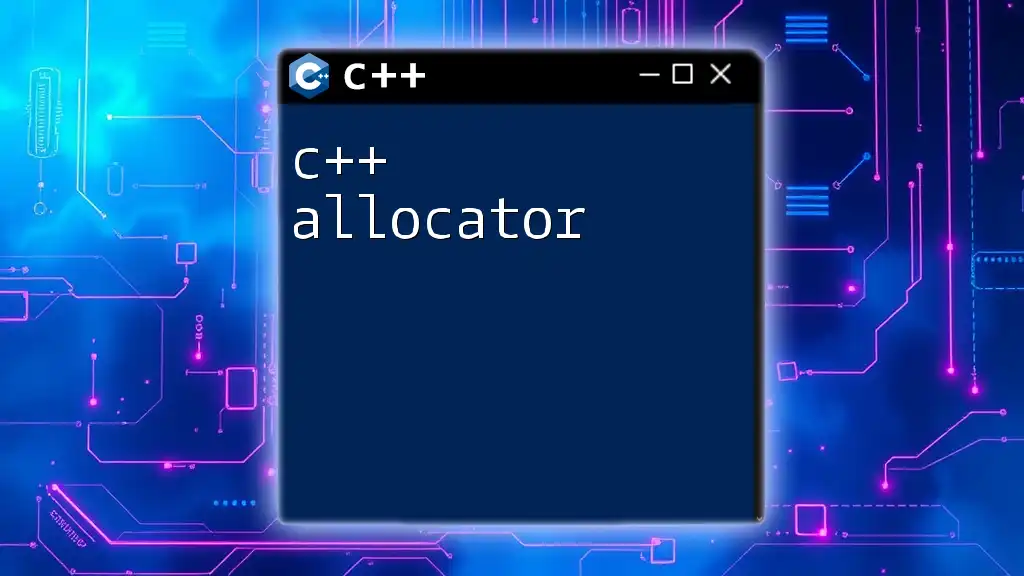
Additional Resources
For further study, consider exploring online courses tailored to C++ programming, as well as textbooks that provide in-depth coverage of string manipulation techniques and memory management in C++.