In this blog post, we will provide a concise review of essential C++ commands that empower beginners to utilize the language effectively.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding the Basics of C++
What is C++?
C++ is an object-oriented programming language developed by Bjarne Stroustrup in the 1980s. It is an extension of the C programming language, which means it inherits all of C's capabilities while adding features that promote better program organization and efficiency. Some of the key features of C++ include:
- Encapsulation: Bundling data and functions that operate on that data within classes.
- Inheritance: Allowing one class to inherit the properties of another, promoting code reuse.
- Polymorphism: Enabling functions to use entities of different types at different times.
Having a solid grasp of the core concepts in C++ is essential for mastering the language and its commands.
Setting Up Your Environment
Before diving into coding, it is crucial to set up a suitable development environment. Some recommended Integrated Development Environments (IDEs) for C++ include:
- Visual Studio: A comprehensive IDE that provides numerous features including debugging tools and an intuitive interface.
- Code::Blocks: Lightweight and customizable, perfect for beginners.
- Eclipse: A powerful IDE known for its extensibility.
To get started, install the C++ compiler relevant to your operating system. The G++ compiler for Windows, macOS, and Linux, and the Clang compiler are popular choices. Follow the respective installation instructions to ensure your environment is ready for seamless coding.
Your First C++ Program
Creating your first C++ program is a significant milestone. Let's consider a simple "Hello, World!" program:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
In this program:
- `#include <iostream>` includes the Input/Output stream library which allows you to use the `cout` function.
- `using namespace std;` lets you use standard functions without needing to prefix them with `std::`.
- The `main()` function is the entry point of any C++ program.
This simple representation demonstrates the fundamental structure of a C++ program.
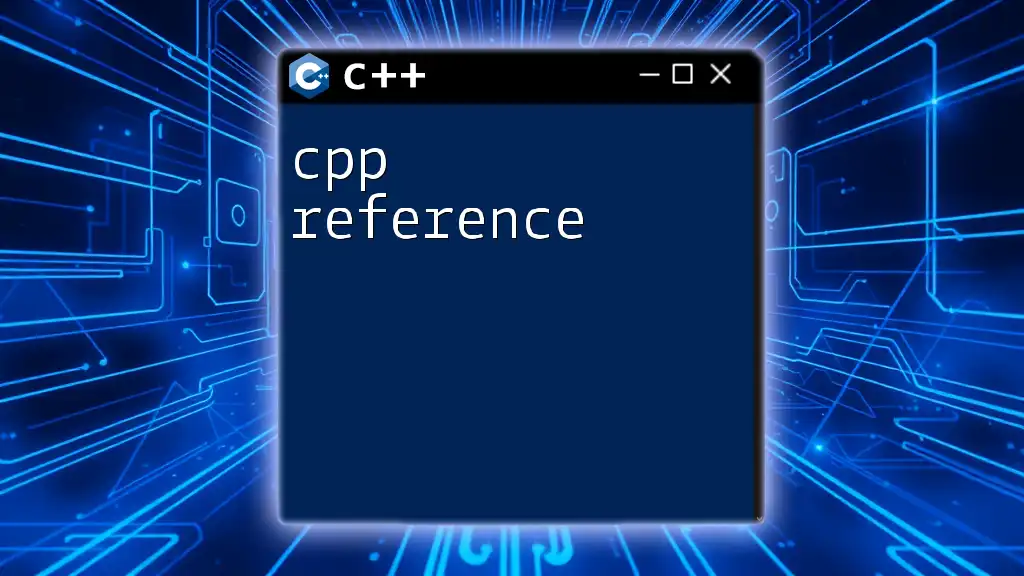
Core C++ Concepts
Variables and Data Types
Understanding variables and data types is fundamental in C++. The basic data types in C++ include:
- int for integer values.
- float for decimal numbers.
- char for single characters.
- string for strings of text.
To declare and initialize these variables, you can do the following:
int age = 30;
float salary = 50000.50;
char grade = 'A';
string name = "John Doe";
By choosing the correct data types, you can optimize your program in terms of memory usage and performance.
Control Structures
Control structures dictate the flow of execution in your program.
Conditional Statements
Conditional statements allow you to branch your code based on certain conditions. Here’s how you can utilize `if`, `else if`, and `else` statements:
if (age >= 18) {
cout << "Adult";
} else {
cout << "Minor";
}
This code evaluates the variable `age` and prints whether it is an Adult or a Minor based on the evaluation.
Loops
Loops enable you to repeat a block of code multiple times. Let's explore the `for` loop:
for (int i = 0; i < 5; i++) {
cout << i << endl;
}
This loop starts at zero and increments `i` after each iteration until it reaches 5, printing each value as it goes.
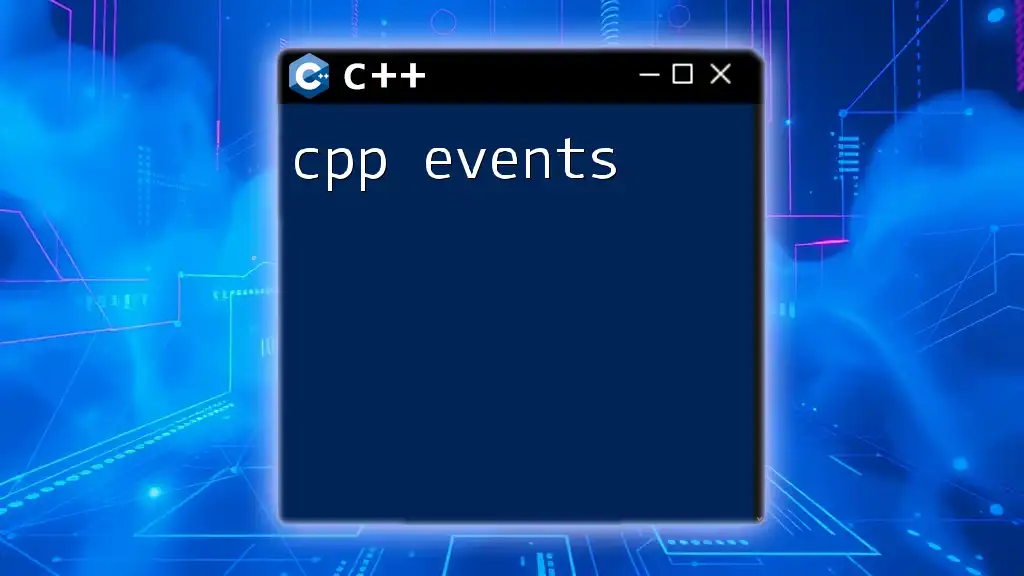
Functions in C++
Defining and Calling Functions
Functions are reusable blocks of code that perform a specific task. Here’s a simple function example:
void greet() {
cout << "Hello!" << endl;
}
You can call this function wherever you need it within your program, promoting cleaner and more organized code.
Function Overloading
One powerful feature of C++ is function overloading, allowing you to have multiple functions with the same name but different parameter types. Consider these examples:
void display(int x) {
cout << "Integer: " << x;
}
void display(string y) {
cout << "String: " << y;
}
Both `display` functions can coexist because their parameter lists differ. This flexibility provides you with options for designing your functions according to the context.
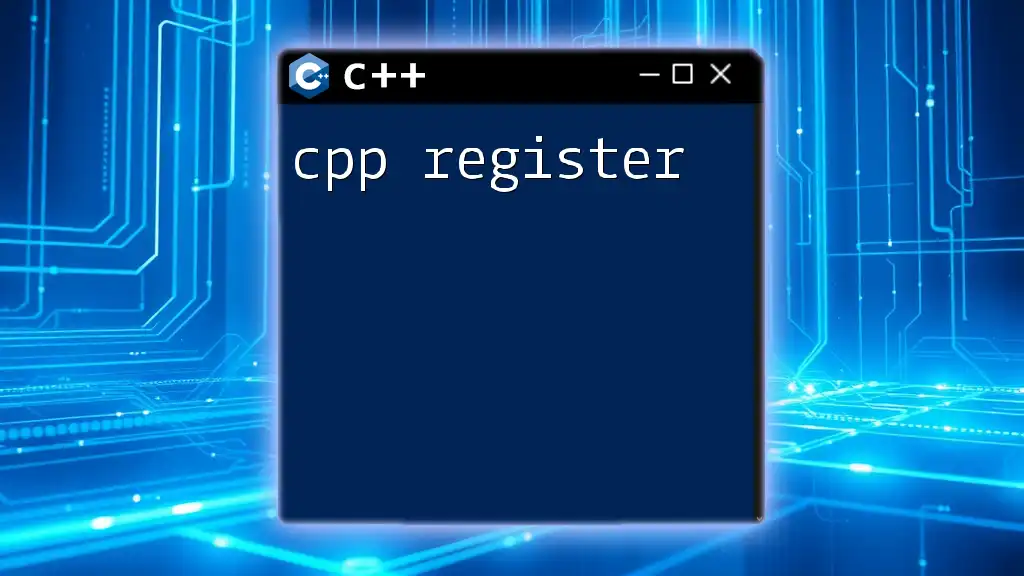
Object-Oriented Programming in C++
Class and Object
C++ brings the concept of classes and objects, which encapsulates data and related functionality. A simple class can be defined as follows:
class Car {
public:
string model;
void displayModel() {
cout << model;
}
};
Here, the class `Car` has a public member `model` and a method `displayModel()` that prints the model’s name. When you create an object of `Car`, you can access its members and methods.
Inheritance
Inheritance is a critical aspect of OOP that allows you to create a new class based on an existing class. Here’s an example:
class Vehicle {
public:
void start() { cout << "Vehicle Starting"; }
};
class Car : public Vehicle {
public:
void drive() { cout << "Car Driving"; }
};
In this scenario, the `Car` class inherits from the `Vehicle` class, gaining its properties and methods while having its own specific methods. This concept aids in code reuse and hierarchy.
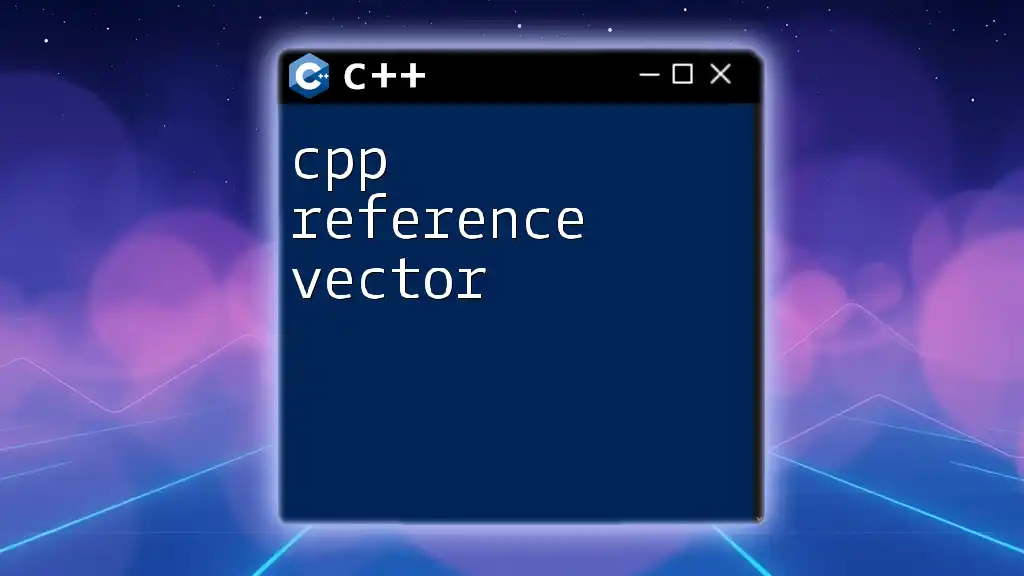
Advanced Concepts
Pointers and References
Pointers are a complex yet powerful feature of C++. A pointer is a variable that stores the memory address of another variable. Here’s how you can use pointers:
int a = 5;
int* ptr = &a;
cout << *ptr; // Output: 5
In this example, `ptr` holds the address of `a`, and using `*ptr` dereferences it to access the value.
Templates
Templates allow you to create functions and classes that are generic, meaning they can operate with any data type. For example:
template <typename T>
T add(T a, T b) {
return a + b;
}
This function can accept integers, floats, or any other type that supports the addition operator. Templates promote code reuse, making your functions adaptable to various data types.
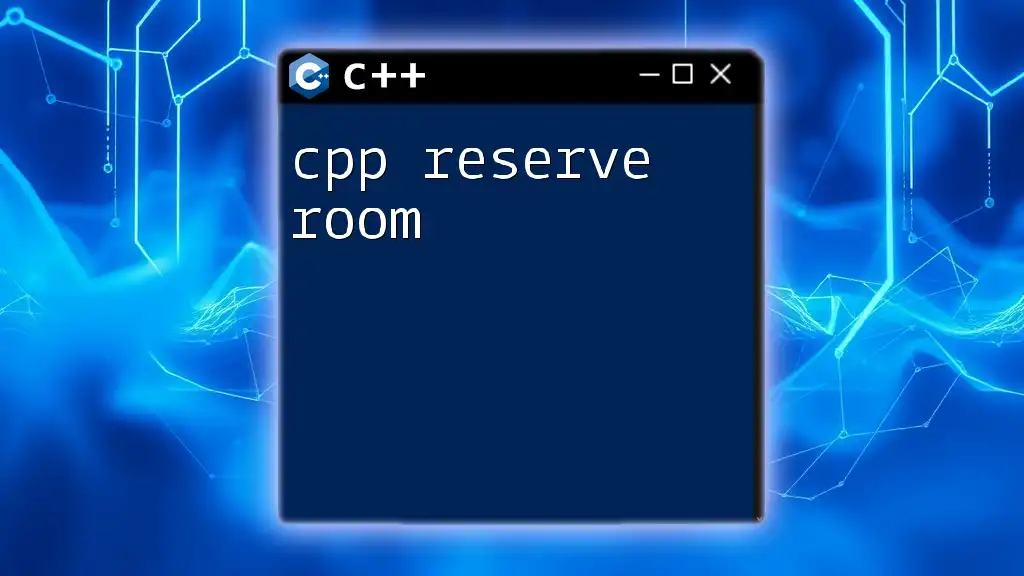
Error Handling in C++
Exceptions
Exception handling allows you to manage runtime errors gracefully. The structure of exception handling includes `try`, `catch`, and `throw`. Here’s a simple demonstration:
try {
throw runtime_error("An error occurred");
} catch (runtime_error &e) {
cout << e.what();
}
In this code, if an error occurs within the `try` block, control is passed to the `catch` block, which handles the error without crashing the program.
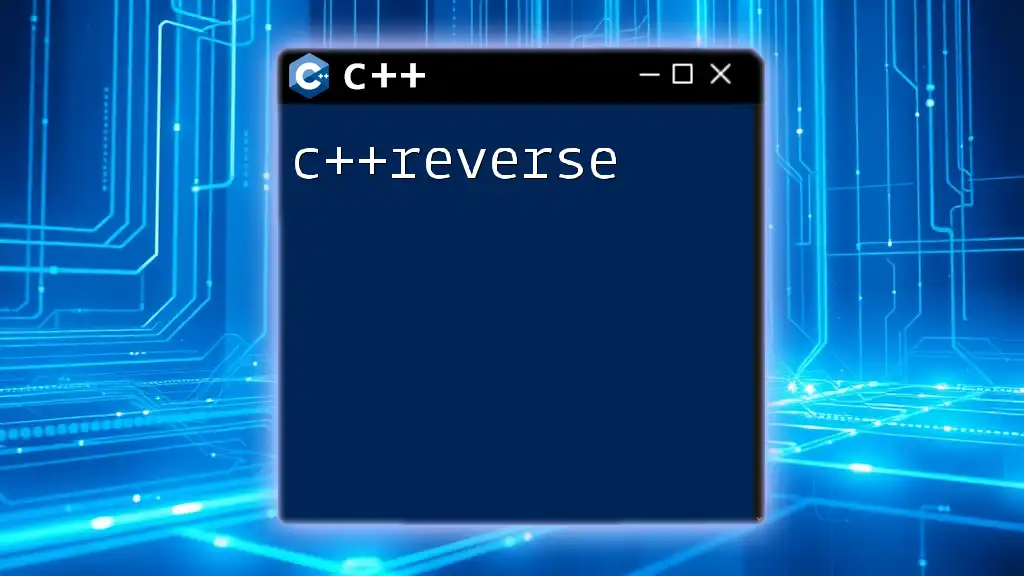
Conclusion
This C++ review covered the essential topics needed to grasp the core aspects of the language—ranging from basic syntax to advanced features like pointers and templates. Mastering these concepts lays the foundation for further exploration and proficiency in C++. As you continue your journey, practice regularly with projects and coding challenges to solidify your understanding.
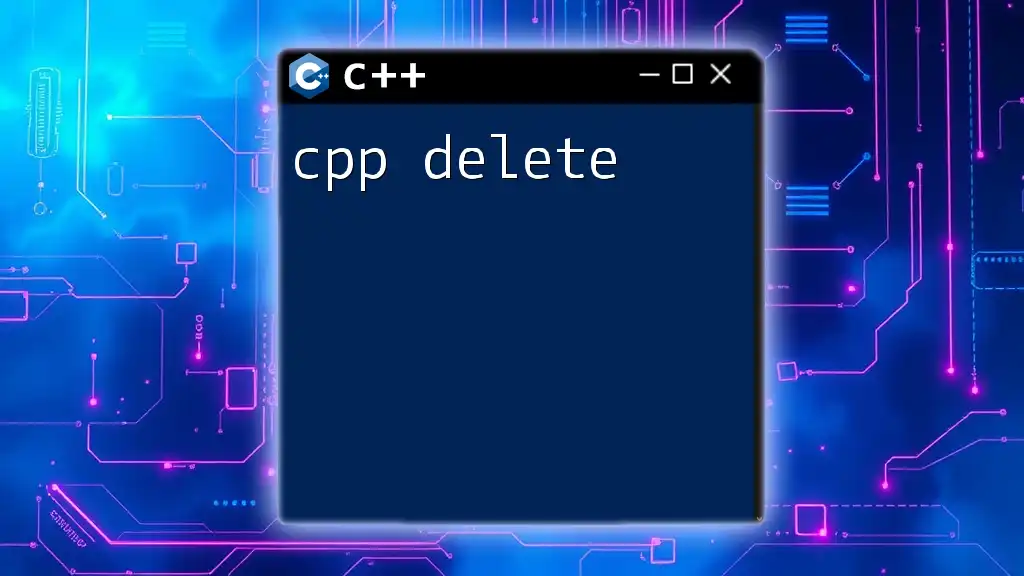
Frequently Asked Questions
What are some common pitfalls for beginners?
New programmers often struggle with pointer arithmetic, memory management, and misunderstanding the nuances of object-oriented principles. It is crucial to invest time in comprehension and avoiding these common mistakes that can lead to frustration.
How can I improve my C++ skills?
Consistent practice is key to mastering C++. Utilize online platforms offering coding challenges, contribute to open-source projects, and collaborate with peers or mentors to gain insights and improve your abilities.
Where can I find C++ projects for practice?
Explore platforms such as GitHub for open-source projects, or undertake personal projects that interest you. Websites like LeetCode or HackerRank offer coding challenges that can deepen your understanding while you solve real-world problems.