The `std::swap` function in C++ exchanges the values of two variables efficiently.
Here's a code snippet demonstrating the usage of `std::swap`:
#include <iostream>
#include <utility> // for std::swap
int main() {
int a = 10, b = 20;
std::swap(a, b);
std::cout << "a: " << a << ", b: " << b << std::endl; // Output: a: 20, b: 10
return 0;
}
Understanding the `swap` Function
What is `swap`?
The `swap` function in C++ is a utility that allows programmers to exchange the values of two variables quickly and efficiently. This operation can be particularly powerful in algorithms and data structures where the arrangement of elements is frequently modified.
Built-in Functionality
C++ provides a built-in version of `swap` in the `<utility>` header, which is optimized for most types. This standard implementation reduces the need to write custom swapping logic, allowing developers to utilize a tried-and-true function to improve their code efficiency.
Syntax of `swap`
The basic syntax for using `swap` is straightforward:
std::swap(a, b);
In this syntax, `a` and `b` refer to the two variables whose values you want to swap. C++ will handle the underlying mechanics of exchanging the values, requiring minimal effort from the coder.
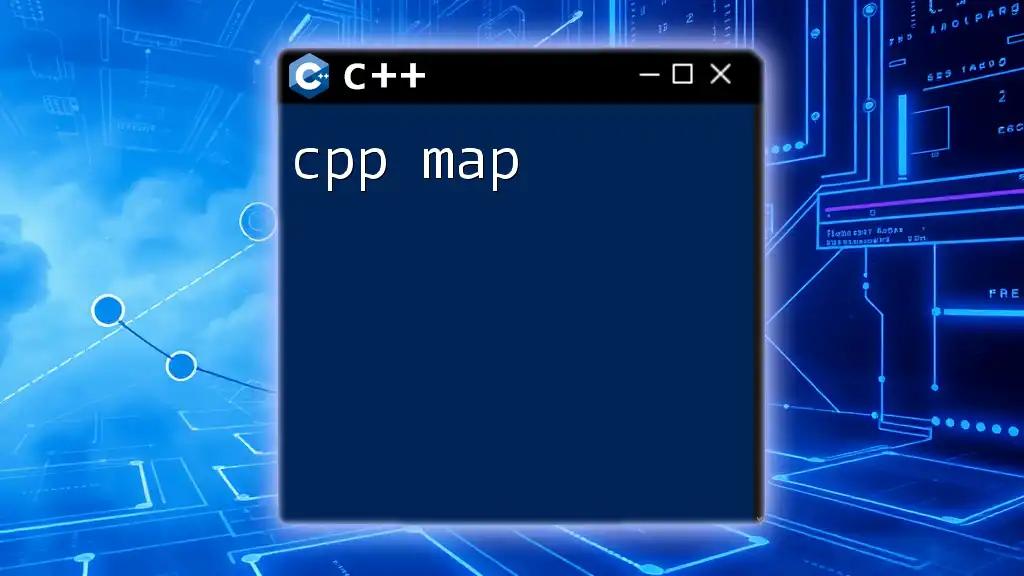
Benefits of Using `swap`
Performance Optimization
Efficiency is one of the standout benefits of the `swap` function. By utilizing `swap`, C++ minimizes the overhead associated with swapping values manually through explicit assignments. Instead of temporarily storing a variable and subsequently rewriting both variables, the `swap` function internally optimizes this process, leading to performance gains—especially within loops or larger data structures.
Simplifying Code Logic
Readability and maintainability are vital qualities of good code. Using `swap` can significantly enhance the clarity of your code. Rather than cluttering your program with multiple variable assignments for a simple swap action, you can express your intentions clearly with a single line of code. This approach not only makes the code easier to understand but also less prone to human error.
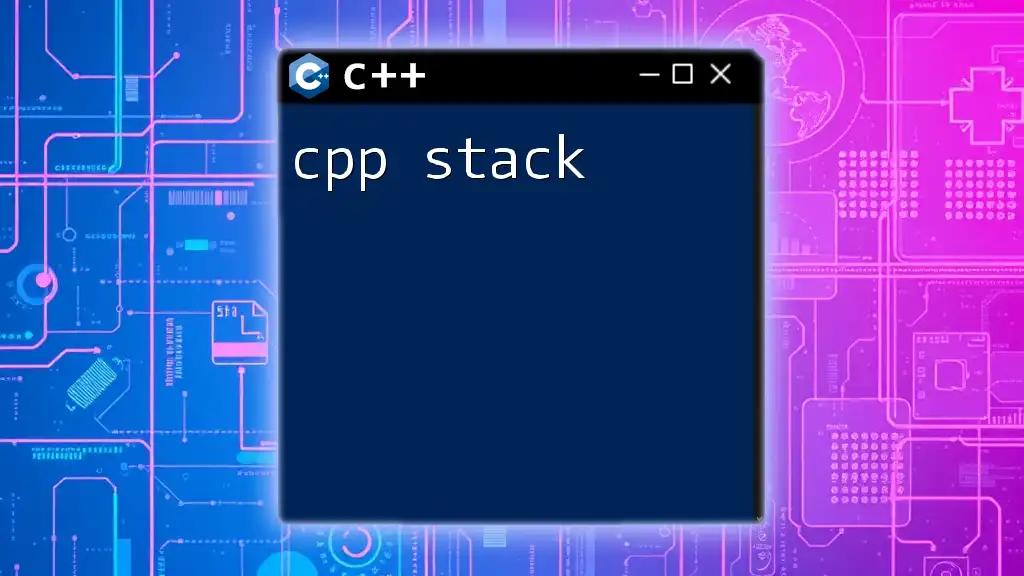
Implementing `swap` in C++
Using Standard Library Function
One of the easiest ways to use `swap` in C++ is utilizing the built-in function from the standard library. Here’s an example to illustrate its use:
#include <iostream>
#include <utility>
int main() {
int a = 5, b = 10;
std::cout << "Before swap: a = " << a << ", b = " << b << std::endl;
std::swap(a, b);
std::cout << "After swap: a = " << a << ", b = " << b << std::endl;
return 0;
}
In the example above, before the swap, `a` holds the value `5`, and `b` has `10`. After calling `std::swap(a, b)`, the values of `a` and `b` are swapped effortlessly, demonstrating how concise and effective this operation can be.
Custom Swap Function
When to Use a Custom Swap
While the standard `swap` function is quite powerful, situations may arise where you want to customize it, particularly when dealing with class objects or specific types that the standard implementation may not handle optimally.
Implementing a Custom `swap`
You can create a generic custom swap function using templates to allow for type flexibility:
template<typename T>
void custom_swap(T &x, T &y) {
T temp = x;
x = y;
y = temp;
}
In this implementation, the `custom_swap` function can swap any two variables of the same type, no matter what that type is—thanks to the use of templates. This flexibility is particularly beneficial for complex structures or when dealing with classes.
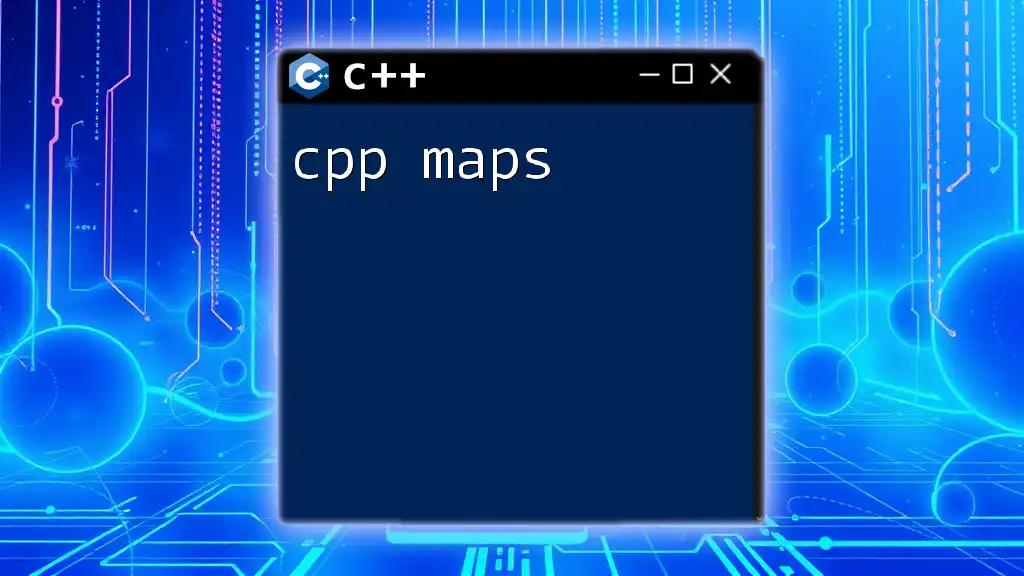
Common Use Cases for `swap`
Swapping Integers
A simple yet common use case involves swapping integer values. The basic operation for integers can be performed directly using `std::swap`:
int a = 1, b = 2;
std::swap(a, b);
This straightforward application showcases its utility in day-to-day programming scenarios.
Swapping Strings
The `swap` functionality extends seamlessly to strings as well. Consider the following example:
#include <iostream>
#include <string>
#include <utility>
int main() {
std::string str1 = "Hello";
std::string str2 = "World";
std::swap(str1, str2);
std::cout << "After swap: str1 = " << str1 << ", str2 = " << str2 << std::endl;
return 0;
}
In this code, the contents of `str1` and `str2` are exchanged, illustrating how `swap` can easily handle different data types.
Swapping Objects
For more advanced usage, `swap` can also be implemented with custom classes. Consider a class called `Box`:
class Box {
public:
int length;
Box(int l) : length(l) {}
};
void swap(Box &b1, Box &b2) {
std::swap(b1.length, b2.length);
}
In this case, the `length` attributes of two `Box` objects are swapped using `std::swap`, demonstrating how this powerful utility can be applied even in custom object manipulation.
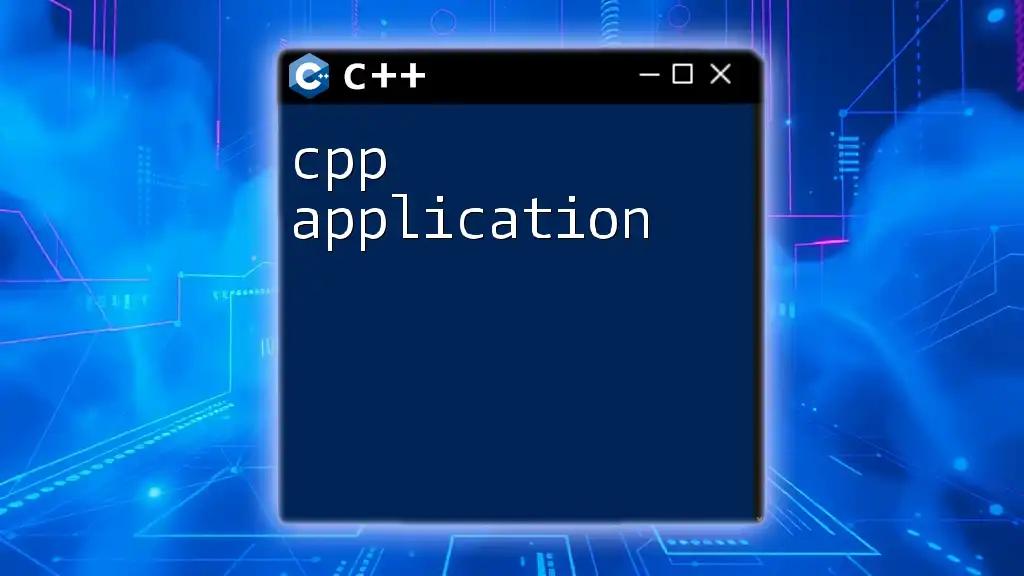
Best Practices for Using `swap`
Avoiding Common Pitfalls
Though `swap` is a straightforward tool, developers must beware of potential pitfalls. One common issue is unintentionally swapping pointers or references without realizing the implications that can have on memory management or data integrity. Being mindful of the types you are swapping and understanding their ownership will help mitigate these problems.
Performance Considerations
When determining whether to use `std::swap` or a custom `swap`, consider the scenario. If you're swapping simple data types, the built-in version is usually sufficient and optimized. However, for complex custom types with ownership semantics or when specific allocations are involved, a customized approach may yield better performance and clarity.
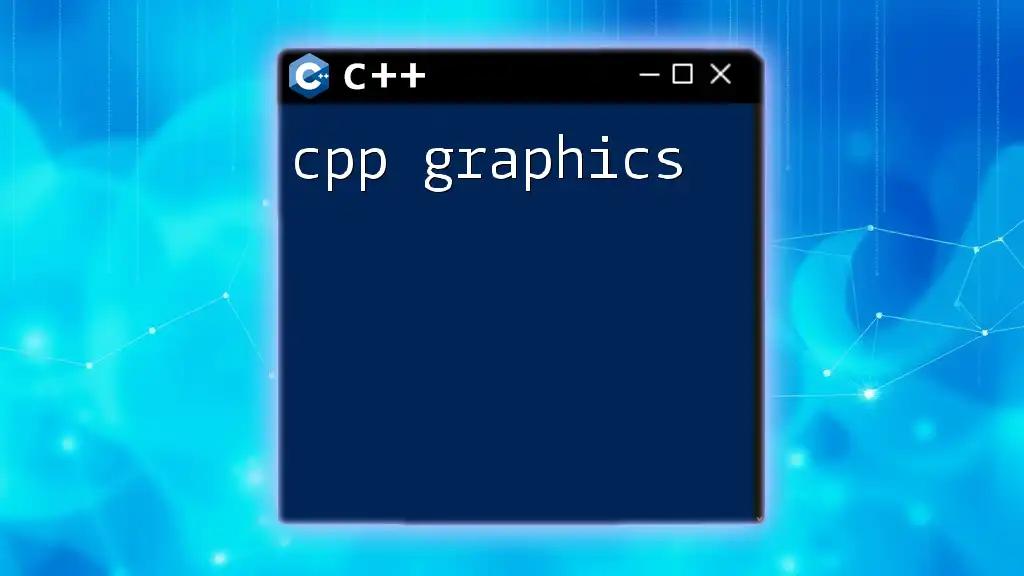
Conclusion
C++'s `swap` functionality is an essential tool in any programmer's toolkit. Its ability to efficiently exchange values while enhancing code readability cannot be overstated. By understanding how to implement and utilize `swap` effectively, you can improve both your coding skills and the performance of your applications. Practice using `swap` in various contexts to become adept at this valuable concept in C++.

Additional Resources
For further reading, consider exploring C++ documentation on the standard library, which provides in-depth details on `std::swap` and its intricate workings. Various online tutorials and community forums can also offer practical insights and examples to deepen your understanding of `swap` and related concepts.