Insertion sort is a simple and intuitive sorting algorithm that builds a sorted array one element at a time, and here is a concise C++ implementation:
#include <iostream>
using namespace std;
void insertionSort(int arr[], int n) {
for (int i = 1; i < n; i++) {
int key = arr[i];
int j = i - 1;
while (j >= 0 && arr[j] > key) {
arr[j + 1] = arr[j];
j--;
}
arr[j + 1] = key;
}
}
int main() {
int arr[] = {12, 11, 13, 5, 6};
int n = sizeof(arr)/sizeof(arr[0]);
insertionSort(arr, n);
for (int i = 0; i < n; i++)
cout << arr[i] << " ";
return 0;
}
Understanding Insertion Sort
What is Insertion Sort?
Insertion Sort is a simple and intuitive sorting algorithm that builds a sorted array (or list) one element at a time. It is much like sorting playing cards in your hands. You start with an empty left hand, and you pick up cards one at a time from the table. You then insert each card into the correct position within your hand.
Understanding sorting algorithms, including insertion sort, is fundamental for efficient programming and data management since they play a crucial role in handling data.
How Insertion Sort Works
The working of insertion sort can be broken down into a few concise steps:
- Begin at the second element of the array (consider the first element as sorted).
- Compare the current element (key) with the elements in the sorted portion.
- Shift all elements larger than the key to the right.
- Place the key in the correct position.
- Repeat the process for all elements in the array.
The time complexity of insertion sort is:
- Best Case: O(n) - The array is already sorted.
- Average and Worst Case: O(n²) - The array is reverse sorted or random.
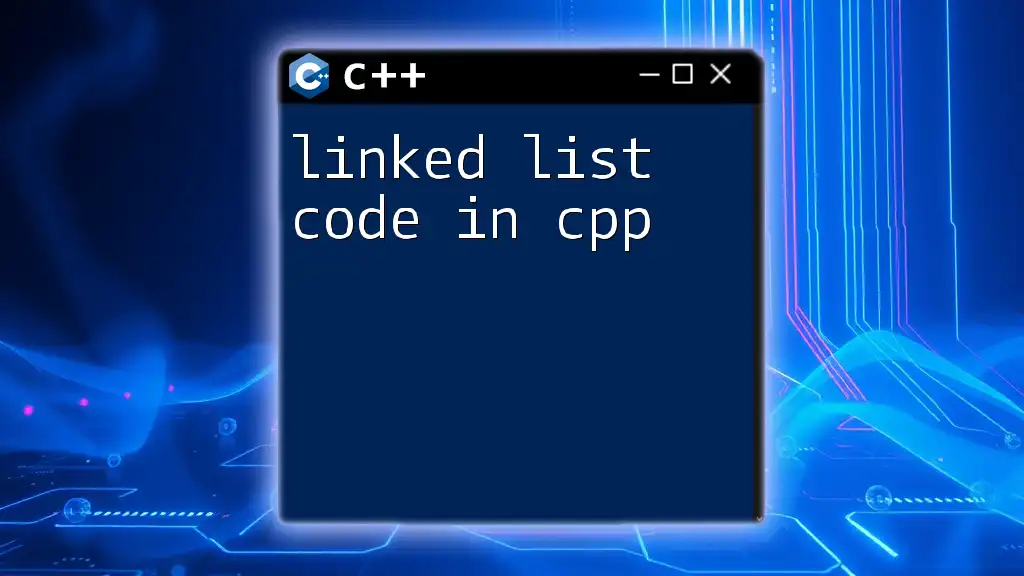
C++ Insertion Sort Code
Insertion Sort in C++: Basic Implementation
The basic structure of a C++ program includes the main function and necessary header files. The insertion sort function itself is designed to sort an array in place. Below is a straightforward implementation:
#include <iostream>
using namespace std;
void insertionSort(int arr[], int n) {
for (int i = 1; i < n; i++) {
int key = arr[i];
int j = i - 1;
// Move elements of arr[0..i-1], that are greater than key,
// to one position ahead of their current position.
while (j >= 0 && arr[j] > key) {
arr[j + 1] = arr[j];
j--;
}
arr[j + 1] = key;
}
}
Detailed Code Explanation
In this code snippet, we initialize a loop starting from the second element (index 1) because the first element is considered as a sorted portion of the array.
- Initialization: The variable `key` is set to the current element we want to insert into the sorted portion.
- Insertion Process: In a nested while loop, we compare the key against the elements in the sorted portion. If an element is greater than the key, it is shifted one position to the right to make space for the key.
- Completion: Once we find the right position, we place the key element in its correct spot.
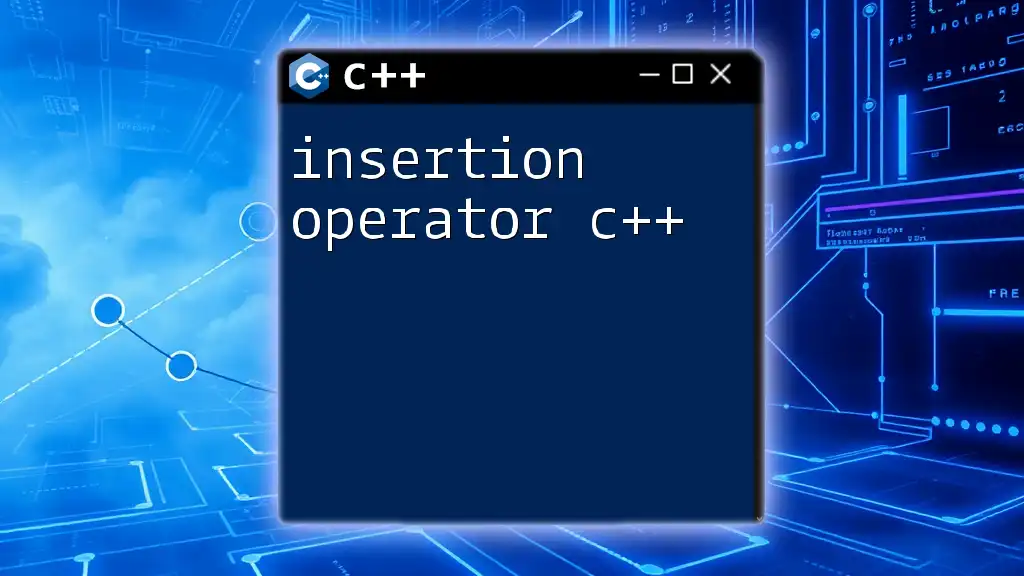
Advanced C++ Insertion Sort Techniques
Optimizing Insertion Sort
While insertion sort is quick for small arrays and nearly sorted data, it can be enhanced through optimizations. One such method is to stop the shifting process if the insertion point is found early, particularly in nearly sorted datasets where fewer shifts are needed.
Recursive Insertion Sort in C++
Recursive insertion sort is an alternative way to implement this algorithm that employs the principles of recursion. The recursive version functions similarly to the iterative approach but is expressed in a different manner:
#include <iostream>
using namespace std;
void recursiveInsertionSort(int arr[], int n) {
if (n <= 1)
return;
recursiveInsertionSort(arr, n - 1);
int last = arr[n - 1];
int j = n - 2;
while (j >= 0 && arr[j] > last) {
arr[j + 1] = arr[j];
j--;
}
arr[j + 1] = last;
}
Efficiency Considerations
The recursive approach may provide clearer logic in some contexts, but remember that it typically comes with additional overhead such as function call costs. It is essential to weigh its use against performance, particularly for larger datasets.
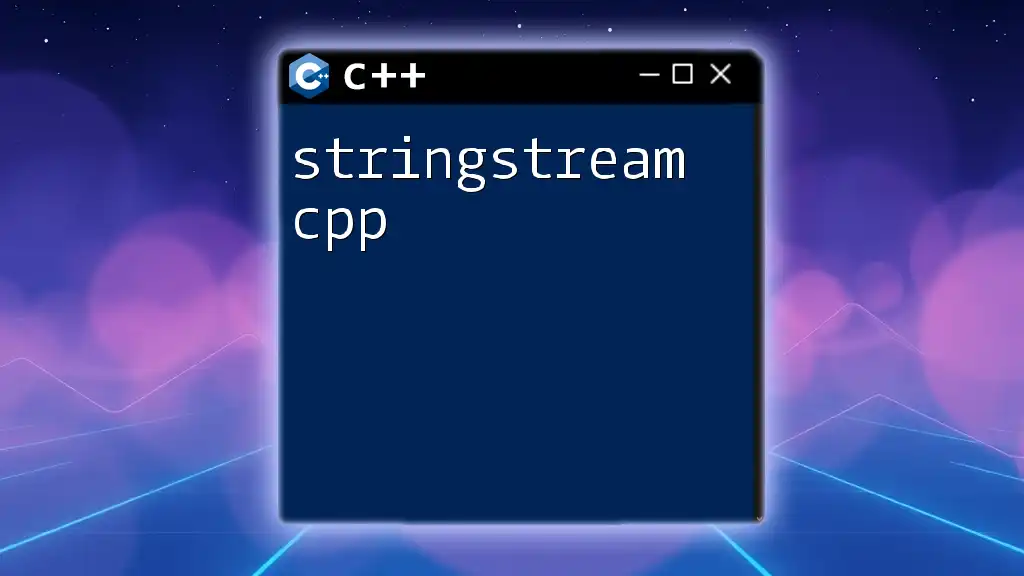
Practical Applications of Insertion Sort in C++
Use Cases for Insertion Sort
Insertion sort is particularly effective in specific scenarios:
- Small datasets: For a small number of items, its performance is often better than more complex algorithms due to lower overhead.
- Nearly sorted data: If you have data that is almost sorted, insertion sort will perform almost in linear time, making it an efficient choice.
When comparing insertion sort to other algorithms such as quicksort or mergesort, it is essential to consider the nature of the data and determine the most suitable algorithm for the task at hand.
Common Pitfalls in C++ Insertion Sort Implementation
While writing insertion sort code in C++, there are common mistakes developers may encounter:
- Off-by-one errors: These typically occur in indexing. It's crucial to ensure that array elements are accessed correctly.
- Infinite loops: Incorrectly setting conditions for while loops can lead to infinite cycles; thorough testing is vital.
- Performance issues: Inserting too many elements or handling large datasets incorrectly can lead to inefficient runtime.
Debugging and testing your code is critical before deploying it in a real environment to avoid these issues.
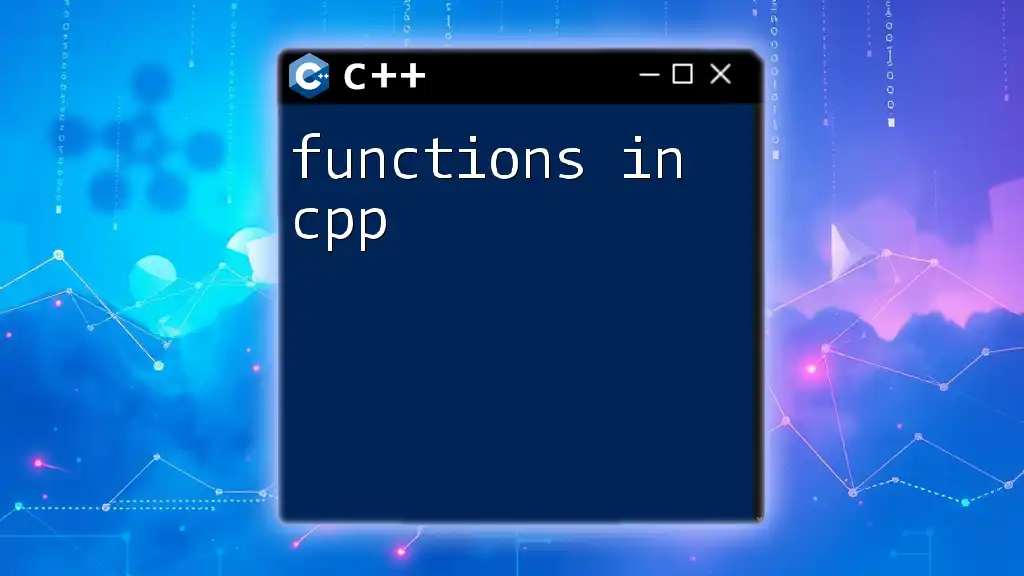
Conclusion: Mastering Insertion Sort in C++
By understanding and implementing the insertion sort code in C++, you've equipped yourself with a fundamental sorting technique that is both intuitive and useful for specific scenarios. This foundational knowledge enables you to handle data more effectively and gives you a stepping stone into deeper topics of data structures and algorithms.
Practice with the examples provided, and feel free to manipulate the code to enhance your understanding further. Engaging with the programming community and sharing your experiences or questions regarding insertion sort will aid in your growth as a C++ developer.
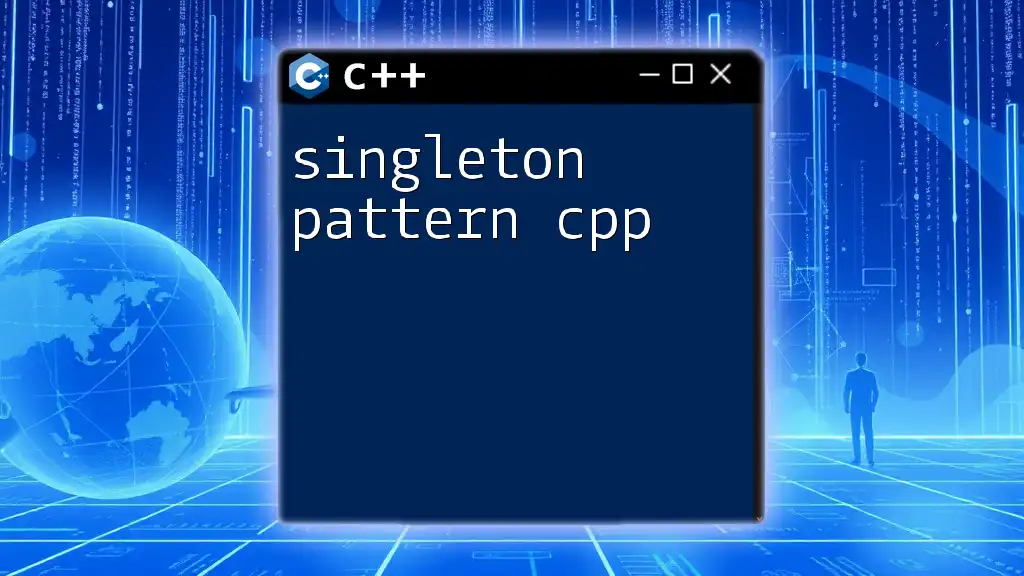
Additional Resources
For those interested in diving deeper into sorting algorithms and C++ programming:
- Explore advanced sorting techniques and their comparative efficiency.
- Participate in online C++ programming tutorials or communities to enhance your understanding.
- See recommended books and dedicated websites that offer comprehensive insights and strategies in mastering C++.